How to Apply Recursion to Solve Complex Problems
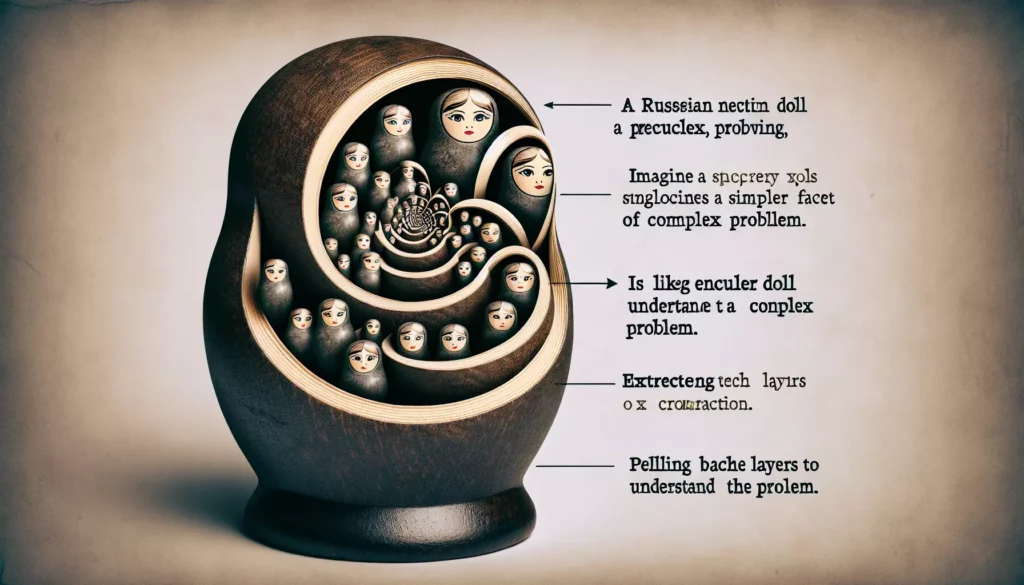
Recursion is a powerful programming technique that allows developers to solve complex problems by breaking them down into smaller, more manageable subproblems. In this comprehensive guide, we’ll explore how to apply recursion effectively to tackle intricate coding challenges, with a focus on preparing for technical interviews at major tech companies.
Understanding Recursion
Before diving into the application of recursion, let’s briefly review what recursion is and how it works:
- Recursion is a method where a function calls itself to solve a problem.
- It involves breaking down a problem into smaller instances of the same problem.
- Every recursive function has a base case (stopping condition) and a recursive case.
The power of recursion lies in its ability to express complex algorithms in a concise and elegant manner. However, it’s crucial to use recursion judiciously, as improper implementation can lead to stack overflow errors or inefficient solutions.
When to Use Recursion
Recursion is particularly useful in scenarios where:
- The problem can be naturally divided into smaller, similar subproblems.
- The solution to the problem depends on solutions to smaller instances of the same problem.
- The problem has a tree-like structure.
- You need to implement backtracking algorithms.
- You’re working with recursive data structures (e.g., linked lists, trees, graphs).
Steps to Apply Recursion
To effectively apply recursion to solve complex problems, follow these steps:
1. Identify the Base Case
The base case is the simplest instance of the problem that can be solved directly without further recursion. It’s crucial to define a correct base case to prevent infinite recursion.
2. Define the Recursive Case
The recursive case describes how to break down the problem into smaller subproblems and how to combine their solutions to solve the original problem.
3. Ensure Progress Towards the Base Case
Make sure that each recursive call moves closer to the base case. This usually involves reducing the size or complexity of the input in some way.
4. Combine Solutions
Determine how to combine the solutions of subproblems to obtain the solution for the original problem.
Examples of Applying Recursion
Let’s explore some classic examples of how to apply recursion to solve complex problems:
1. Fibonacci Sequence
The Fibonacci sequence is a classic example of a problem that can be solved recursively. Each number in the sequence is the sum of the two preceding ones.
def fibonacci(n):
if n <= 1: # Base case
return n
else: # Recursive case
return fibonacci(n-1) + fibonacci(n-2)
# Example usage
print(fibonacci(6)) # Output: 8
In this example, the base case is when n is 0 or 1. The recursive case calculates the sum of the two preceding Fibonacci numbers.
2. Factorial Calculation
Calculating the factorial of a number is another common recursive problem.
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else: # Recursive case
return n * factorial(n-1)
# Example usage
print(factorial(5)) # Output: 120
The base case is when n is 0 or 1, as the factorial of these numbers is 1. For other numbers, we multiply n by the factorial of (n-1).
3. Binary Search
Binary search is an efficient algorithm for searching a sorted array, and it can be implemented recursively.
def binary_search(arr, target, low, high):
if low > high: # Base case: target not found
return -1
mid = (low + high) // 2
if arr[mid] == target: # Base case: target found
return mid
elif arr[mid] > target: # Recursive case: search lower half
return binary_search(arr, target, low, mid - 1)
else: # Recursive case: search upper half
return binary_search(arr, target, mid + 1, high)
# Example usage
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(arr, target, 0, len(arr) - 1)
print(f"Target {target} found at index: {result}") # Output: Target 7 found at index: 3
In this implementation, the base cases are when the target is found or when the search space is exhausted. The recursive cases divide the search space in half based on the comparison with the middle element.
Advanced Recursive Techniques
As you become more comfortable with basic recursion, you can explore more advanced techniques to solve even more complex problems:
1. Divide and Conquer
Divide and conquer is a problem-solving paradigm that involves breaking a problem into smaller subproblems, solving them recursively, and then combining their solutions. Examples include:
- Merge Sort
- Quick Sort
- Strassen’s Matrix Multiplication
2. Dynamic Programming with Memoization
Dynamic programming is an optimization technique that combines recursion with memoization (caching of results) to avoid redundant calculations. This approach is particularly useful for problems with overlapping subproblems.
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
# Example usage
print(fibonacci_memo(100)) # Calculates the 100th Fibonacci number efficiently
3. Backtracking
Backtracking is a recursive technique used to solve problems where you need to find all (or some) solutions to a computational problem. It incrementally builds candidates for the solutions and abandons a candidate as soon as it determines that the candidate cannot lead to a valid solution.
Examples of problems solved using backtracking include:
- N-Queens Problem
- Sudoku Solver
- Generating all permutations of a set
4. Tree and Graph Traversals
Recursion is particularly well-suited for traversing tree and graph data structures. Common recursive traversals include:
- In-order, pre-order, and post-order traversals for binary trees
- Depth-First Search (DFS) for graphs
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val, end=" ")
inorder_traversal(root.right)
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
inorder_traversal(root) # Output: 4 2 5 1 3
Common Pitfalls and How to Avoid Them
While recursion is powerful, it comes with potential pitfalls. Here are some common issues and how to avoid them:
1. Stack Overflow
Recursive calls consume stack space, and deep recursion can lead to stack overflow errors. To mitigate this:
- Use tail recursion optimization when possible.
- Consider iterative solutions for problems with deep recursion.
- Implement your own stack for very deep recursions.
2. Redundant Calculations
Naive recursive implementations can lead to exponential time complexity due to redundant calculations. To avoid this:
- Use memoization or dynamic programming techniques.
- Implement bottom-up iterative solutions for certain problems.
3. Incorrect Base Case
Failing to define the correct base case can result in infinite recursion. Always ensure that:
- The base case is correctly defined and covers all terminating scenarios.
- The recursive case makes progress towards the base case.
4. Excessive Recursion Depth
Some problems may require an excessive number of recursive calls. In such cases:
- Consider tail recursion optimization.
- Explore iterative solutions or hybrid approaches.
Optimizing Recursive Solutions
To make your recursive solutions more efficient and interview-ready, consider these optimization techniques:
1. Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Many modern compilers can optimize tail-recursive functions to use constant stack space.
def factorial_tail(n, acc=1):
if n == 0:
return acc
return factorial_tail(n-1, n*acc)
# Example usage
print(factorial_tail(5)) # Output: 120
2. Memoization
We’ve already seen an example of memoization with the Fibonacci sequence. This technique can dramatically improve the performance of recursive functions with overlapping subproblems.
3. Iterative Solutions
In some cases, converting a recursive solution to an iterative one can improve performance and reduce stack usage. For example, here’s an iterative version of the factorial function:
def factorial_iterative(n):
result = 1
for i in range(1, n+1):
result *= i
return result
# Example usage
print(factorial_iterative(5)) # Output: 120
4. Hybrid Approaches
Sometimes, a combination of recursive and iterative approaches can yield the best results. For example, in quicksort, you might use recursion for the overall algorithm but switch to an iterative sorting method for small subarrays.
Real-world Applications of Recursion
Understanding how recursion is applied in real-world scenarios can help you appreciate its power and relevance. Here are some practical applications:
1. File System Operations
Recursion is often used for operations on hierarchical file systems, such as directory traversal or file search.
2. Parsing and Compilers
Recursive descent parsers use recursion to parse programming languages and other structured text formats.
3. Data Compression
Some compression algorithms, like Huffman coding, use recursive structures to build compression trees.
4. Computer Graphics
Fractal generation and ray tracing algorithms often employ recursive techniques.
5. Machine Learning
Decision trees and certain neural network architectures use recursive structures.
Preparing for Technical Interviews
When preparing for technical interviews, especially for major tech companies, keep these points in mind regarding recursion:
1. Practice Classic Problems
Familiarize yourself with classic recursive problems like tree traversals, backtracking problems, and divide-and-conquer algorithms.
2. Analyze Time and Space Complexity
Be prepared to discuss the time and space complexity of your recursive solutions. Understand how to derive recurrence relations and solve them.
3. Consider Trade-offs
Be ready to discuss the trade-offs between recursive and iterative solutions for a given problem.
4. Optimize Your Solutions
Practice optimizing recursive solutions using techniques like memoization and tail recursion.
5. Explain Your Thought Process
During interviews, clearly explain your thought process when developing a recursive solution. Discuss how you identified the base case and recursive case.
Conclusion
Recursion is a fundamental concept in computer science and a powerful tool for solving complex problems. By understanding when and how to apply recursion, you can write elegant and efficient solutions to a wide range of programming challenges. As you prepare for technical interviews, focus on mastering recursive techniques, optimizing your solutions, and being able to clearly explain your approach.
Remember that while recursion can lead to concise and intuitive solutions, it’s not always the best approach. Always consider the problem at hand and choose the most appropriate solution, whether it’s recursive, iterative, or a combination of both. With practice and a deep understanding of recursion, you’ll be well-equipped to tackle complex problems and excel in technical interviews at top tech companies.