How to Analyze and Explain Your Code in an Interview
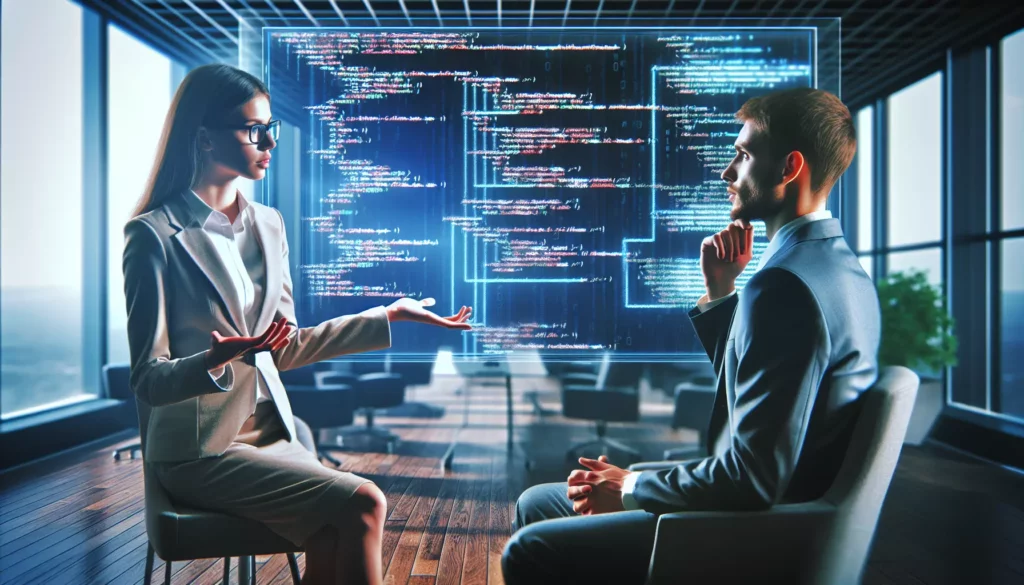
When it comes to technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to analyze and explain your code is just as crucial as writing it. This skill demonstrates not only your technical prowess but also your communication abilities and thought process. In this comprehensive guide, we’ll explore effective strategies to analyze and explain your code during an interview, helping you showcase your skills and stand out from other candidates.
1. Understanding the Importance of Code Analysis and Explanation
Before diving into the specifics, it’s essential to understand why the ability to analyze and explain your code is so valuable to interviewers:
- Demonstrates critical thinking: It shows that you can think critically about your code and understand its implications.
- Reveals communication skills: Clear explanations showcase your ability to communicate complex ideas effectively.
- Highlights problem-solving approach: It gives insight into your problem-solving process and decision-making skills.
- Shows depth of knowledge: Detailed explanations reveal the depth of your understanding of programming concepts.
- Indicates collaboration potential: Good communication skills are crucial for teamwork in professional settings.
2. Preparing for Code Analysis
To effectively analyze and explain your code during an interview, preparation is key. Here are some steps to help you get ready:
2.1. Practice Regularly
Consistent practice is crucial. Regularly solve coding problems and explain your solutions out loud, even when you’re alone. This helps you become comfortable articulating your thoughts and reasoning.
2.2. Understand Common Algorithms and Data Structures
Familiarize yourself with common algorithms and data structures. Be prepared to explain their time and space complexities, use cases, and trade-offs. Some key areas to focus on include:
- Sorting algorithms (e.g., quicksort, mergesort)
- Search algorithms (e.g., binary search)
- Graph algorithms (e.g., BFS, DFS, Dijkstra’s)
- Data structures (e.g., arrays, linked lists, trees, hash tables)
2.3. Review Your Own Code
Regularly review and refactor your own code. This practice helps you identify areas for improvement and reinforces good coding habits.
2.4. Study Code Style and Best Practices
Familiarize yourself with coding best practices and style guidelines for the programming languages you’ll be using in the interview. This knowledge will help you write cleaner, more maintainable code and explain your choices confidently.
3. Structuring Your Code Explanation
When explaining your code during an interview, it’s helpful to follow a structured approach. Here’s a framework you can use:
3.1. Start with the Problem Statement
Begin by restating the problem in your own words. This ensures that you and the interviewer are on the same page and demonstrates your understanding of the task at hand.
3.2. Explain Your Approach
Before diving into the code details, give a high-level overview of your approach. Discuss:
- The algorithm or strategy you chose
- Why you chose this particular approach
- Any alternative approaches you considered
3.3. Walk Through the Code
Go through your code step by step, explaining:
- The purpose of each major section or function
- Key variables and data structures used
- Any important logic or algorithms implemented
3.4. Discuss Time and Space Complexity
Analyze and explain the time and space complexity of your solution. Be prepared to discuss best-case, average-case, and worst-case scenarios.
3.5. Address Potential Edge Cases
Discuss how your code handles edge cases or potential issues, such as invalid input or extreme scenarios.
3.6. Suggest Possible Improvements
If time allows, mention any potential optimizations or alternative approaches that could improve your solution.
4. Tips for Effective Code Explanation
To make your code explanation more effective and engaging, consider the following tips:
4.1. Use Clear and Concise Language
Avoid jargon unless you’re sure the interviewer is familiar with it. Use clear, simple language to explain complex concepts.
4.2. Provide Context
When explaining a specific part of your code, provide context by relating it to the overall problem and your chosen approach.
4.3. Use Analogies
Where appropriate, use analogies to explain complex concepts or algorithms. This can make your explanation more relatable and easier to understand.
4.4. Engage the Interviewer
Make your explanation interactive by asking if the interviewer has any questions or if they’d like more detail on any particular aspect.
4.5. Be Honest About Uncertainties
If you’re unsure about something, it’s better to admit it than to try to bluff. You can say something like, “I’m not entirely sure about this part, but here’s what I think…”
5. Common Pitfalls to Avoid
When analyzing and explaining your code, be aware of these common pitfalls:
5.1. Over-explaining
While thorough explanations are good, avoid getting bogged down in unnecessary details. Focus on the most important aspects of your code.
5.2. Ignoring the Interviewer
Pay attention to the interviewer’s reactions and questions. They may provide valuable cues about what aspects of your code they’re most interested in.
5.3. Defensive Behavior
If the interviewer points out an issue or suggests an improvement, don’t become defensive. Instead, show your ability to accept feedback and iterate on your solution.
5.4. Rushing Through the Explanation
Take your time to explain your code clearly. Rushing can lead to confusion and may give the impression that you don’t fully understand your own solution.
6. Practical Example: Analyzing and Explaining a Binary Search Algorithm
Let’s walk through an example of how to analyze and explain a binary search algorithm implementation during an interview.
6.1. The Code
Here’s a Python implementation of binary search:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
6.2. Explanation Structure
Now, let’s break down how you might explain this code in an interview:
Problem Statement
“The problem we’re solving here is to efficiently find a target value in a sorted array. We’re using the binary search algorithm to accomplish this task.”
Approach Overview
“I’ve chosen to implement the binary search algorithm because it’s highly efficient for searching in sorted arrays, with a time complexity of O(log n). The basic idea is to repeatedly divide the search interval in half, allowing us to eliminate half of the remaining elements in each iteration.”
Code Walkthrough
“Let’s go through the code step by step:
- We start by initializing two pointers, ‘left’ and ‘right’, to the start and end of the array respectively.
- We enter a while loop that continues as long as ‘left’ is less than or equal to ‘right’.
- Inside the loop, we calculate the middle index as the average of ‘left’ and ‘right’.
- We then compare the element at the middle index with our target:
- If they’re equal, we’ve found the target and return its index.
- If the middle element is less than the target, we know the target must be in the right half, so we update ‘left’ to mid + 1.
- If the middle element is greater than the target, we know the target must be in the left half, so we update ‘right’ to mid – 1.
- If we exit the while loop without finding the target, we return -1 to indicate the target is not in the array.”
Time and Space Complexity
“The time complexity of this algorithm is O(log n) because we’re dividing the search space in half with each iteration. In the worst case, we’ll need log2(n) iterations to find the target or determine it’s not in the array.
The space complexity is O(1) because we’re using a constant amount of extra space regardless of the input size. We’re only using a few variables (left, right, mid) that don’t grow with the input.”
Edge Cases
“This implementation handles several edge cases:
- If the array is empty, the initial right value will be -1, and the while loop won’t execute, correctly returning -1.
- If the target is smaller than the smallest element or larger than the largest element, the algorithm will correctly return -1.
- It also correctly handles cases where the target is at the beginning or end of the array.”
Potential Improvements
“One potential improvement could be to use `left + (right – left) // 2` instead of `(left + right) // 2` to calculate the middle index. This prevents potential integer overflow for very large arrays, although it’s not an issue in Python with its arbitrary-precision integers.”
7. Practice Makes Perfect
Analyzing and explaining code effectively is a skill that improves with practice. Here are some ways to hone this skill:
7.1. Participate in Mock Interviews
Practice with friends, mentors, or through online platforms that offer mock technical interviews. This will help you get comfortable explaining your code under pressure.
7.2. Join Coding Communities
Participate in online coding communities where you can share your solutions and explain your approach to others. Platforms like LeetCode, HackerRank, or CodeSignal often have discussion forums where you can engage in such conversations.
7.3. Teach Others
Teaching is an excellent way to reinforce your own understanding. Consider tutoring, mentoring, or creating coding tutorials to practice explaining concepts clearly.
7.4. Record Yourself
Try recording yourself as you solve and explain coding problems. This can help you identify areas for improvement in your explanation style and clarity.
8. Conclusion
The ability to analyze and explain your code effectively is a crucial skill for succeeding in technical interviews, especially for positions at top tech companies. By understanding the importance of this skill, preparing thoroughly, structuring your explanations well, and practicing regularly, you can significantly improve your performance in coding interviews.
Remember, interviewers are not just looking for someone who can write code, but for a potential colleague who can think critically, communicate effectively, and contribute to the team’s success. By mastering the art of code analysis and explanation, you’ll be well-equipped to showcase these qualities and stand out in your next technical interview.
Keep practicing, stay curious, and approach each coding challenge as an opportunity to improve both your coding skills and your ability to articulate your thoughts. With time and effort, you’ll find yourself confidently navigating even the most challenging technical interviews.