How to Adapt Your Solution Based on Feedback During the Interview
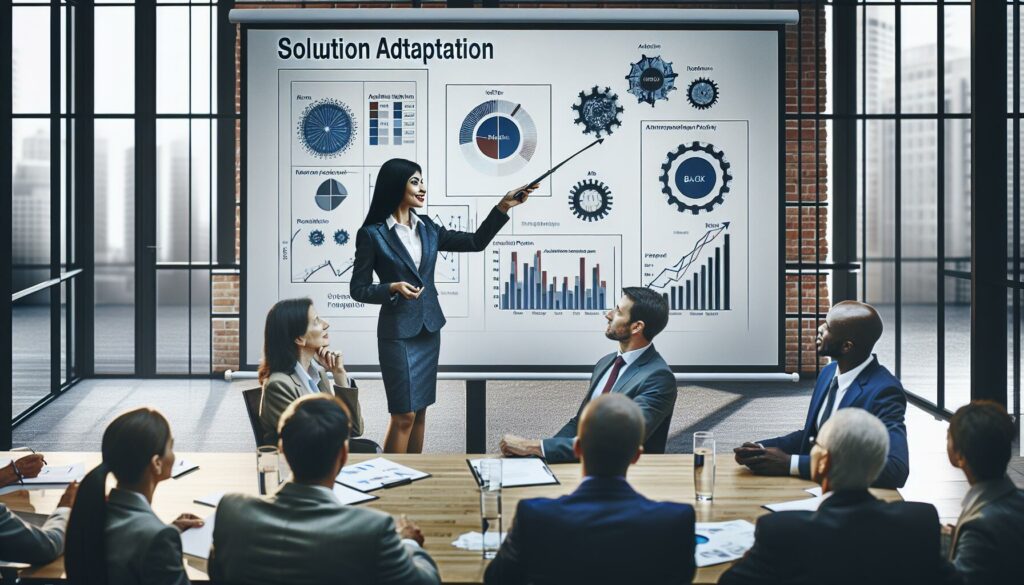
Technical interviews can be nerve-wracking experiences, especially when you’re aiming for positions at prestigious tech companies. One crucial skill that often separates successful candidates from the rest is the ability to adapt and refine their solutions based on interviewer feedback. In this comprehensive guide, we’ll explore the art of remaining flexible during coding interviews, discuss strategies for incorporating hints and suggestions, and highlight the importance of demonstrating your capacity to iterate on ideas.
The Importance of Adaptability in Coding Interviews
Before we dive into specific strategies, let’s understand why adaptability is such a valued trait in the tech industry:
- Real-world problem-solving: In actual development scenarios, requirements often change, and solutions need to evolve. Showing adaptability during an interview demonstrates that you can handle real-world challenges.
- Collaboration skills: The ability to incorporate feedback and work with others’ ideas is crucial in team environments.
- Learning agility: Adapting quickly showcases your capacity to learn and grow, a highly desirable trait in the fast-paced tech world.
- Problem-solving under pressure: Interviews are stressful, and being able to pivot your approach demonstrates grace under pressure.
Strategies for Adapting Your Solution
1. Active Listening
The foundation of adaptability is active listening. When an interviewer provides feedback or hints, it’s crucial to:
- Pay close attention to their words
- Ask clarifying questions if needed
- Take notes if necessary
- Confirm your understanding before proceeding
Example dialogue:
Interviewer: "Have you considered using a hash map to optimize the lookup?"
You: "That's an interesting suggestion. If I understand correctly, you're proposing that a hash map could improve the time complexity for lookups. Can you elaborate on how you envision this being implemented in this context?"
2. Acknowledge the Feedback
Always acknowledge the interviewer’s input, even if you’re not immediately sure how to incorporate it. This shows respect and openness to new ideas.
You: "Thank you for that insight. I hadn't considered using a hash map. Let me think about how we could integrate that into the current solution."
3. Think Aloud
As you process the feedback and consider how to adapt your solution, verbalize your thoughts. This gives the interviewer insight into your problem-solving process and allows them to provide further guidance if needed.
You: "Okay, so if we use a hash map, we could potentially reduce the lookup time from O(n) to O(1). We'd need to consider the trade-off between space complexity and time complexity. Let me walk through how this might change our approach..."
4. Iterate Incrementally
Instead of completely scrapping your original solution, try to incorporate the feedback incrementally. This demonstrates your ability to build upon existing work and refine ideas.
You: "Let's start by implementing the hash map for the most frequent lookups while keeping the rest of the structure intact. Then we can evaluate if further optimizations are necessary."
5. Explain Your Reasoning
As you adapt your solution, explain the rationale behind your changes. This showcases your critical thinking skills and ensures the interviewer understands your thought process.
You: "I'm choosing to use a hash map for the user IDs because we're performing frequent lookups. This will reduce our time complexity from O(n) to O(1) for these operations, which is crucial given the scale of data we're dealing with."
6. Be Open to Multiple Iterations
Sometimes, the first adaptation might not be the final solution. Be prepared to go through multiple iterations based on ongoing feedback.
Interviewer: "That's a good start. Now, how would you handle collisions in the hash map?"
You: "Excellent point. We could implement chaining to handle collisions. Let me adjust the code to incorporate this..."
Handling Different Types of Feedback
Interviewers may provide various types of feedback. Here’s how to handle each:
Direct Hints
When an interviewer gives a direct hint, they’re often pointing you towards a specific optimization or approach they want to see.
Interviewer: "Have you considered using a two-pointer technique?"
You: "Thank you for the suggestion. I hadn't thought of that approach. Let me see how we can apply the two-pointer technique to this problem..."
Subtle Nudges
Sometimes, interviewers provide more subtle guidance. Pay attention to these gentle pushes in a certain direction.
Interviewer: "What if we needed to optimize for space complexity?"
You: "Interesting question. If space complexity is a concern, we might want to reconsider our current approach of storing all elements in memory. Perhaps we could explore a streaming algorithm or..."
Challenging Assumptions
Interviewers might challenge your assumptions to see how you handle edge cases or unexpected scenarios.
Interviewer: "What if the input could include negative numbers?"
You: "That's an important consideration I overlooked. Let me adjust our solution to handle negative inputs. We might need to modify our initial conditions and..."
Performance Concerns
Feedback about performance usually indicates that the interviewer wants to see you optimize your solution.
Interviewer: "How would this solution perform with a million elements?"
You: "You're right to be concerned about scale. With a million elements, our current O(n^2) solution wouldn't be efficient. Let's explore ways to optimize this, perhaps using a more efficient data structure or algorithm..."
Demonstrating Your Ability to Iterate
Showing that you can iterate on your ideas is crucial. Here are some ways to demonstrate this skill:
1. Propose Multiple Solutions
Even before receiving feedback, consider presenting multiple approaches to the problem.
You: "I see two potential solutions here. We could use a brute force approach, which would be simpler but less efficient, or we could optimize using a binary search tree. Let me outline both, and we can discuss which you'd like me to implement."
2. Analyze Trade-offs
When adapting your solution, discuss the trade-offs between different approaches.
You: "By switching to a hash map, we're trading increased space complexity for improved time complexity. In this case, I believe it's worth it because..."
3. Refactor as You Go
Don’t be afraid to refactor your code as you incorporate feedback. This shows your commitment to clean, maintainable code.
You: "Now that we've added this new functionality, I see an opportunity to refactor this section to improve readability. Would you like me to do that?"
4. Test and Debug
As you make changes, continuously test your code and debug any issues. This demonstrates thoroughness and attention to detail.
You: "Let me add a few test cases to ensure our new implementation handles all scenarios correctly."
Handling Challenges in Adaptation
Adapting your solution isn’t always smooth sailing. Here are some challenges you might face and how to handle them:
When You Don’t Understand the Feedback
If you’re unsure about the interviewer’s suggestion, it’s okay to ask for clarification.
You: "I'm not entirely familiar with the technique you've mentioned. Could you provide a bit more context or perhaps point me towards the aspect of the problem where this technique would be most beneficial?"
When You Disagree with the Suggestion
There might be times when you believe your original approach is better. In such cases, discuss it respectfully.
You: "I appreciate your suggestion to use recursion here. I initially chose an iterative approach because I was concerned about stack overflow with large inputs. However, I'm open to exploring the recursive solution if you think it offers advantages I might have overlooked."
When You’re Stuck
If you’re having trouble incorporating the feedback, be honest and think through it step-by-step.
You: "I'm having some difficulty integrating the binary search into our current structure. Let me break this down into smaller steps and think it through aloud if that's okay."
The Role of Practice in Developing Adaptability
Like any skill, adaptability in coding interviews improves with practice. Here are some ways to enhance this ability:
1. Mock Interviews
Engage in mock interviews where your interviewer provides real-time feedback and suggestions. This simulates the actual interview environment and helps you get comfortable with adapting on the fly.
2. Pair Programming
Practice pair programming with peers. This exposes you to different problem-solving approaches and helps you learn to incorporate others’ ideas into your code.
3. Code Reviews
Participate in code reviews, both as a reviewer and as the one being reviewed. This trains you to give and receive constructive feedback and to iterate on solutions based on peer input.
4. Online Coding Platforms
Utilize platforms like AlgoCademy that offer interactive coding tutorials and AI-powered assistance. These tools can provide instant feedback and alternative solutions, helping you learn to adapt quickly.
5. Solve Problems Multiple Ways
When practicing coding problems, challenge yourself to solve each problem in multiple ways. This flexibility will serve you well when you need to pivot during an interview.
Conclusion
Adapting your solution based on interviewer feedback is a critical skill in technical interviews. It demonstrates your flexibility, collaborative spirit, and ability to think on your feet. By actively listening, acknowledging feedback, thinking aloud, and iterating incrementally, you can showcase your adaptability and problem-solving skills.
Remember, the goal isn’t just to solve the problem, but to demonstrate your thought process and ability to work with others’ ideas. Embrace feedback as an opportunity to improve your solution and showcase your skills. With practice and the right mindset, you can turn the challenge of adaptation into one of your strongest assets in coding interviews.
As you continue your journey in coding education and interview preparation, platforms like AlgoCademy can provide valuable resources and practice opportunities. By focusing on algorithmic thinking, problem-solving, and practical coding skills, you’ll be well-prepared to adapt and excel in any technical interview scenario.