How to Ace Your Take-Home Coding Assignment: A Comprehensive Guide
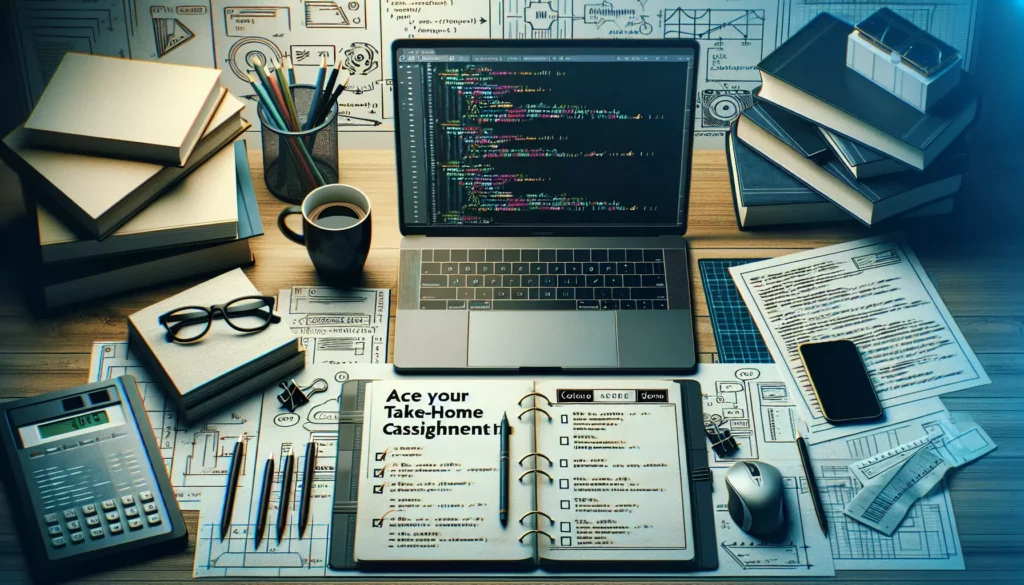
Take-home coding assignments have become an increasingly popular part of the technical interview process for software engineering positions. These assignments offer candidates a chance to showcase their skills in a more relaxed environment compared to on-the-spot coding challenges. However, they also come with their own set of challenges and expectations. In this comprehensive guide, we’ll walk you through everything you need to know to excel in your take-home coding assignment and increase your chances of landing that dream job.
Understanding Take-Home Coding Assignments
Before we dive into the preparation strategies, let’s first understand what take-home coding assignments are and why companies use them:
- Definition: A take-home coding assignment is a programming task given to job candidates to complete on their own time, usually within a specified timeframe (e.g., 24-48 hours or a week).
- Purpose: These assignments allow companies to assess a candidate’s coding skills, problem-solving abilities, and attention to detail in a more realistic setting than whiteboard interviews or time-constrained coding challenges.
- Evaluation criteria: Companies typically look at code quality, functionality, efficiency, and how well the candidate followed the given instructions.
Preparing for Take-Home Coding Assignments
Now that we understand the basics, let’s explore how to prepare effectively for these assignments:
1. Brush Up on Fundamentals
Before you even receive an assignment, it’s crucial to have a solid foundation in programming fundamentals. This includes:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and space complexity analysis
- Object-oriented programming principles
- Design patterns
Platforms like AlgoCademy offer interactive tutorials and resources to help you strengthen these foundational skills. Make use of their AI-powered assistance and step-by-step guidance to master these concepts.
2. Practice Coding Regularly
Consistent practice is key to improving your coding skills and preparing for take-home assignments. Here are some ways to incorporate regular coding practice into your routine:
- Solve coding challenges: Use platforms like LeetCode, HackerRank, or CodeSignal to solve algorithmic problems regularly.
- Build side projects: Work on personal projects to gain hands-on experience with different technologies and frameworks.
- Contribute to open-source: Participating in open-source projects can help you understand large codebases and collaborate with other developers.
3. Familiarize Yourself with Common Tools and Technologies
Take-home assignments often require you to work with specific tools or technologies. Make sure you’re comfortable with:
- Version control systems (e.g., Git)
- Popular IDEs or text editors (e.g., Visual Studio Code, IntelliJ IDEA)
- Command-line interfaces
- Testing frameworks relevant to your primary programming language
- Build tools and package managers (e.g., npm for JavaScript, pip for Python)
4. Study Best Practices and Coding Standards
Companies often evaluate your code based on its readability, maintainability, and adherence to best practices. Familiarize yourself with:
- Clean code principles
- SOLID principles for object-oriented design
- Language-specific style guides (e.g., PEP 8 for Python, Google’s Java Style Guide)
- Code documentation best practices
5. Prepare Your Development Environment
Having a well-set-up development environment can save you time and reduce stress during the assignment. Consider:
- Setting up your preferred IDE or text editor with useful extensions
- Creating project templates for quick start-up
- Configuring your version control system
- Setting up linters and formatters to ensure code quality
Tackling the Take-Home Coding Assignment
Once you receive the assignment, follow these steps to maximize your chances of success:
1. Read and Understand the Requirements
This might seem obvious, but it’s crucial to thoroughly read and understand the assignment requirements before you start coding. Pay attention to:
- The problem statement and desired outcome
- Any specific requirements or constraints
- The evaluation criteria
- The deadline and time expectations
If anything is unclear, don’t hesitate to ask for clarification from the company. It’s better to ask questions upfront than to make incorrect assumptions.
2. Plan Your Approach
Before diving into coding, take some time to plan your approach:
- Break down the problem into smaller, manageable tasks
- Sketch out a high-level design or architecture
- Decide on the technologies and libraries you’ll use
- Create a rough timeline for completing different parts of the assignment
3. Start with a Minimum Viable Product (MVP)
Instead of trying to implement all features at once, start with a basic working version that meets the core requirements. This approach has several benefits:
- It ensures you have something to submit even if you run out of time
- It allows you to get the basic structure in place before adding complexity
- It provides a solid foundation for iterative improvements
4. Write Clean, Well-Documented Code
As you code, focus on writing clean, readable, and well-documented code. This includes:
- Using meaningful variable and function names
- Writing clear comments to explain complex logic or decisions
- Structuring your code into logical modules or classes
- Following consistent formatting and style guidelines
Here’s an example of clean, well-documented code in Python:
def calculate_average(numbers):
"""
Calculate the average of a list of numbers.
Args:
numbers (list): A list of numbers.
Returns:
float: The average of the numbers.
Raises:
ValueError: If the input list is empty.
"""
if not numbers:
raise ValueError("Cannot calculate average of an empty list")
total = sum(numbers)
count = len(numbers)
return total / count
# Example usage
try:
sample_numbers = [1, 2, 3, 4, 5]
average = calculate_average(sample_numbers)
print(f"The average is: {average}")
except ValueError as e:
print(f"Error: {e}")
5. Implement Error Handling and Edge Cases
Don’t forget to handle potential errors and edge cases in your code. This demonstrates your attention to detail and ability to write robust software. Consider:
- Invalid input handling
- Edge cases (e.g., empty lists, boundary values)
- Error messages and logging
- Graceful failure scenarios
6. Write Tests
Including tests with your submission shows that you value code quality and reliability. Write unit tests for your functions and integration tests for your overall solution. Here’s an example of a simple unit test in Python using the unittest
framework:
import unittest
from my_module import calculate_average
class TestCalculateAverage(unittest.TestCase):
def test_calculate_average_normal_case(self):
numbers = [1, 2, 3, 4, 5]
expected_average = 3.0
self.assertEqual(calculate_average(numbers), expected_average)
def test_calculate_average_single_number(self):
numbers = [42]
expected_average = 42.0
self.assertEqual(calculate_average(numbers), expected_average)
def test_calculate_average_empty_list(self):
numbers = []
with self.assertRaises(ValueError):
calculate_average(numbers)
if __name__ == '__main__':
unittest.main()
7. Optimize Your Solution
Once you have a working solution, look for opportunities to optimize it. This could involve:
- Improving time or space complexity
- Refactoring code for better readability or maintainability
- Optimizing database queries or API calls
However, be cautious not to over-optimize at the expense of readability or simplicity. Sometimes, a clear and straightforward solution is preferable to a highly optimized but complex one.
8. Document Your Solution
Provide clear documentation for your solution. This typically includes:
- A README file explaining how to set up and run your project
- Any assumptions or design decisions you made
- Known limitations or areas for improvement
- Instructions for running tests
Here’s an example of what a good README might look like:
# Project Name
Brief description of your project.
## Setup
1. Clone the repository:
```
git clone https://github.com/yourusername/project-name.git
```
2. Install dependencies:
```
pip install -r requirements.txt
```
3. Set up environment variables (if applicable):
```
export API_KEY=your_api_key_here
```
## Running the Application
To run the application, use the following command:
```
python main.py
```
## Running Tests
To run the test suite, use:
```
python -m unittest discover tests
```
## Design Decisions
- Explain any significant design decisions or trade-offs you made.
## Known Limitations
- List any known limitations or areas for improvement.
## Future Enhancements
- Suggest potential future enhancements or features.
9. Review and Refine
Before submitting your assignment, take some time to review and refine your work:
- Run your tests to ensure everything is working as expected
- Review your code for any last-minute improvements or clean-ups
- Check that you’ve met all the requirements specified in the assignment
- Proofread your documentation for clarity and correctness
10. Submit on Time
Make sure to submit your assignment before the deadline. If you’re running short on time, it’s better to submit a working solution with some known limitations than to miss the deadline trying to perfect every detail.
After Submission
Your work doesn’t end with submission. Here are some things to consider after you’ve turned in your assignment:
1. Reflect on Your Work
Take some time to reflect on the assignment. Consider what you learned, what challenges you faced, and how you might approach similar problems in the future.
2. Prepare for Follow-Up Questions
Be ready to discuss your solution in a follow-up interview. You might be asked about:
- Your overall approach and design decisions
- Specific implementation details
- How you might scale your solution for larger datasets
- Potential optimizations or improvements
3. Seek Feedback
Whether you move forward in the interview process or not, try to get feedback on your submission. This can provide valuable insights for future assignments and help you improve your skills.
Common Pitfalls to Avoid
As you prepare for and work on take-home coding assignments, be aware of these common pitfalls:
1. Overengineering
While it’s important to showcase your skills, avoid overengineering your solution. Focus on meeting the requirements efficiently rather than adding unnecessary complexity.
2. Ignoring Instructions
Carefully follow all instructions provided with the assignment. Failing to adhere to specific requirements or constraints can negatively impact your evaluation.
3. Poor Time Management
Plan your time wisely. Don’t spend too much time on one aspect of the assignment at the expense of others. If you’re struggling with a particular part, move on and come back to it later if time allows.
4. Neglecting Error Handling
Don’t forget to handle potential errors and edge cases. Robust error handling demonstrates your ability to write production-ready code.
5. Insufficient Testing
Failing to include tests or submitting code with obvious bugs can be a red flag. Make sure to thoroughly test your solution before submission.
6. Plagiarism
While it’s okay to use libraries or reference documentation, don’t copy entire solutions from online sources. Your submission should be your own work.
Conclusion
Take-home coding assignments can be challenging, but they also offer a great opportunity to showcase your skills and problem-solving abilities. By following the strategies outlined in this guide, you can approach these assignments with confidence and increase your chances of success.
Remember, the key to excelling in take-home coding assignments is a combination of solid preparation, careful planning, clean implementation, and thorough testing. With practice and the right approach, you can turn these assignments into powerful demonstrations of your coding prowess.
As you continue to develop your skills, consider using resources like AlgoCademy to enhance your algorithmic thinking and problem-solving abilities. With its interactive tutorials, AI-powered assistance, and focus on practical coding skills, AlgoCademy can be a valuable tool in your journey to becoming a top-tier software engineer.
Good luck with your next take-home coding assignment!