How Practice Alone Won’t Make You Better: The Role of Reflection in Problem Solving
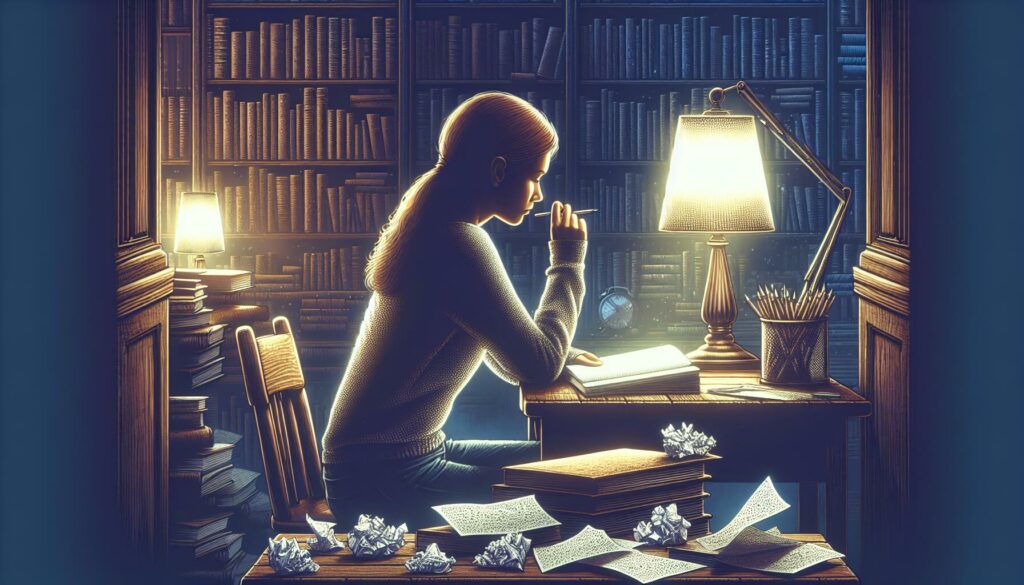
In the world of coding and software development, there’s a common belief that practice makes perfect. While it’s true that consistent practice is crucial for improvement, it’s not the whole story. Many aspiring programmers find themselves stuck in a loop, solving problem after problem without seeing significant progress in their skills. The missing piece of the puzzle? Reflection.
In this article, we’ll explore why practice alone isn’t enough to make you a better problem solver, and how incorporating reflection into your learning process can dramatically enhance your coding skills. We’ll delve into the importance of reviewing and reflecting on your past problem-solving attempts, discuss how writing down insights and mistakes can build long-term problem-solving skills, and provide practical strategies for implementing reflection in your coding journey.
The Limitations of Practice Without Reflection
Before we dive into the role of reflection, let’s first understand why practice alone might not be as effective as we think:
- Reinforcing bad habits: Without reflection, you might unknowingly repeat inefficient or incorrect approaches to problem-solving.
- Lack of deeper understanding: Solving problems mechanically without analyzing the process can prevent you from grasping underlying concepts and patterns.
- Missed learning opportunities: Failing to reflect on your mistakes means missing out on valuable lessons that could improve your future performance.
- Plateau in skill development: You might reach a point where you’re no longer improving, simply because you’re not identifying areas that need work.
These limitations highlight the need for a more thoughtful approach to learning and skill development in programming. This is where reflection comes into play.
The Power of Reflection in Problem Solving
Reflection is the process of thinking deeply about your experiences, analyzing what worked and what didn’t, and drawing insights that can inform future actions. When applied to problem-solving in coding, reflection can be a game-changer. Here’s why:
1. Enhances Understanding of Concepts
When you take the time to reflect on a problem you’ve solved, you’re more likely to understand the underlying concepts and principles at play. This deeper understanding makes it easier to apply similar solutions to different problems in the future.
For example, after solving a problem involving a binary search algorithm, you might reflect on why it’s more efficient than a linear search for certain types of data. This reflection helps solidify your understanding of time complexity and algorithm efficiency, concepts that you can apply broadly in your coding practice.
2. Identifies Patterns in Problem-Solving Approaches
Regular reflection helps you recognize patterns in how you approach different types of problems. You might notice that you tend to struggle with certain concepts or that you excel in particular areas. This self-awareness allows you to tailor your learning and practice to address your specific needs.
3. Improves Decision-Making Skills
By reflecting on past problem-solving experiences, you develop a mental database of strategies and their outcomes. This repository of knowledge informs your decision-making process when faced with new challenges, helping you choose more effective approaches.
4. Builds Metacognitive Skills
Reflection nurtures metacognition – the ability to think about your own thinking processes. This skill is crucial for programmers, as it enables you to monitor your problem-solving strategies in real-time, making adjustments as needed.
5. Enhances Long-Term Memory and Skill Retention
The act of reflection helps transfer knowledge from short-term to long-term memory. By actively processing your experiences, you’re more likely to retain the skills and insights you’ve gained over time.
Implementing Reflection in Your Coding Practice
Now that we understand the importance of reflection, let’s explore practical ways to incorporate it into your coding practice:
1. Keep a Coding Journal
One of the most effective ways to reflect on your problem-solving process is to maintain a coding journal. After solving each problem, take a few minutes to write down:
- The problem statement and your initial thoughts
- The approach you took to solve the problem
- Any challenges you faced and how you overcame them
- Alternative solutions you considered
- What you learned from solving this problem
Here’s an example of what an entry in your coding journal might look like:
Problem: Implement a function to find the longest palindromic substring in a given string.
Initial thoughts: This seems like it could be solved using a brute force approach, but I suspect there's a more efficient solution.
Approach:
1. Started with a brute force solution, checking every possible substring.
2. Realized this was inefficient (O(n^3) time complexity).
3. Researched and learned about the "expand around center" technique.
4. Implemented the more efficient solution (O(n^2) time complexity).
Challenges:
- Initially struggled with handling even-length palindromes.
- Overcame by treating each character and the space between characters as potential centers.
Alternative solutions considered:
- Dynamic programming approach (seemed more complex for this problem).
Learnings:
- The importance of considering edge cases (empty string, single character).
- How to optimize by leveraging the properties of palindromes.
- The "expand around center" technique can be applied to other palindrome-related problems.
2. Conduct Regular Code Reviews
Even if you’re working on solo projects, conducting regular code reviews can be incredibly beneficial. Here’s how to do it:
- Set aside time (e.g., once a week) to review your recent code.
- Pretend you’re explaining your code to someone else. This often reveals areas where your logic might be unclear or overly complex.
- Look for patterns in your coding style and identify areas for improvement.
- Consider how you might refactor the code to make it more efficient or readable.
3. Utilize the Feynman Technique
Named after the famous physicist Richard Feynman, this technique involves explaining a concept in simple terms as if you were teaching it to someone else. Apply this to your coding practice by:
- Choosing a problem or concept you’ve recently worked on.
- Explaining it out loud or writing it down as if you’re teaching it to a beginner.
- Identifying areas where your explanation falters – these are likely gaps in your understanding.
- Revisiting the topic to fill in those gaps.
4. Participate in Code Reviews with Peers
If possible, engage in code reviews with fellow programmers. This can be done through:
- Online coding communities
- Local meetup groups
- Study groups or coding bootcamps
- Open source project contributions
Reviewing others’ code and having your code reviewed provides fresh perspectives and exposes you to different problem-solving approaches.
5. Use Reflection Prompts
Sometimes, getting started with reflection can be challenging. Use these prompts to guide your thinking:
- What was the most challenging part of this problem, and why?
- How does this solution compare to others I’ve implemented for similar problems?
- What assumptions did I make, and were they valid?
- If I had to solve this problem again, what would I do differently?
- How can I apply what I learned from this problem to future challenges?
The Long-Term Benefits of Reflection in Coding
Incorporating reflection into your coding practice isn’t just about improving your problem-solving skills for the next challenge. It has far-reaching benefits that can significantly impact your career as a programmer:
1. Accelerated Skill Development
By consistently reflecting on your problem-solving process, you’ll likely find that you progress faster in your coding skills. You’ll be able to tackle increasingly complex problems with greater confidence and efficiency.
2. Enhanced Problem-Solving Versatility
Reflection helps you build a diverse toolkit of problem-solving strategies. Over time, you’ll become more adept at selecting the right approach for each unique challenge you face.
3. Improved Code Quality
As you reflect on your coding practices, you’ll naturally start to write cleaner, more efficient, and more maintainable code. This improvement in code quality is invaluable in professional settings.
4. Better Preparation for Technical Interviews
The insights gained through reflection can be particularly beneficial when preparing for technical interviews, especially for positions at major tech companies. You’ll be better equipped to explain your thought process, discuss alternative solutions, and analyze the trade-offs of different approaches – all crucial skills for acing coding interviews.
5. Continuous Learning Mindset
Regular reflection fosters a growth mindset and a habit of continuous learning. This mindset is essential in the ever-evolving field of technology, where staying current with new languages, frameworks, and best practices is crucial.
Overcoming Common Challenges in Implementing Reflection
While the benefits of reflection are clear, implementing it consistently can be challenging. Here are some common obstacles and strategies to overcome them:
1. Time Constraints
Challenge: Feeling like you don’t have time to reflect after solving a problem.
Solution: Start small. Even 5-10 minutes of reflection can be beneficial. Gradually increase the time as you see the benefits. Remember, the time invested in reflection often saves time in the long run by preventing repeated mistakes.
2. Lack of Structure
Challenge: Not knowing how to reflect effectively.
Solution: Use structured templates or checklists for your reflection process. For example:
Reflection Template:
1. Problem Summary: [Brief description of the problem]
2. My Approach: [Steps taken to solve the problem]
3. Challenges Faced: [List of difficulties encountered]
4. Key Learnings: [New concepts or techniques learned]
5. Future Applications: [How this learning can be applied to other problems]
6. Areas for Improvement: [Skills or concepts to focus on next]
3. Difficulty in Self-Analysis
Challenge: Finding it hard to critically analyze your own work.
Solution: Practice objectivity by imagining you’re reviewing someone else’s code. Also, consider using tools like code linters or AI-powered code review assistants to provide an initial objective analysis.
4. Focusing Only on Successes
Challenge: Tendency to reflect only on successful problem-solving experiences.
Solution: Make a conscious effort to reflect on both successes and failures. Often, the most valuable lessons come from analyzing where things went wrong.
5. Isolation in Learning
Challenge: Lack of external input or different perspectives.
Solution: Engage with coding communities, either online or in-person. Platforms like Stack Overflow, GitHub, or local coding meetups can provide valuable opportunities for shared reflection and learning.
Integrating Reflection with Coding Platforms and Tools
To make reflection a seamless part of your coding practice, consider integrating it with the tools and platforms you already use. Here are some ideas:
1. Version Control Systems
Use detailed commit messages in Git to document your thought process and key decisions. This creates a natural timeline of your problem-solving journey.
Example of a reflective commit message:
git commit -m "Implement binary search for user lookup
- Chose binary search over linear search for O(log n) time complexity
- Considered hash table but opted for binary search due to sorted data
- TODO: Explore ways to handle duplicate entries in future iterations"
2. IDE Extensions
Many Integrated Development Environments (IDEs) offer extensions or plugins that can aid in reflection. For example:
- Code Time (for VS Code): Tracks your coding activity and provides insights into your productivity patterns.
- TODO Tree (for VS Code): Helps you keep track of areas in your code that need further reflection or improvement.
3. Learning Platforms
Platforms like AlgoCademy often have built-in features that support reflection. Make full use of these tools:
- Progress tracking: Regularly review your progress charts to identify areas of strength and weakness.
- Solution comparisons: After solving a problem, compare your solution with others to gain new perspectives.
- AI-powered feedback: Utilize AI assistants to get insights into your code quality and potential improvements.
4. Digital Note-Taking Tools
Use tools like Notion, Evernote, or OneNote to create a digital coding journal. These platforms allow you to easily organize your reflections, add code snippets, and link related concepts.
Conclusion: Embracing Reflection for Continuous Improvement
As we’ve explored throughout this article, the path to becoming a proficient problem solver and coder isn’t just about relentless practice – it’s about thoughtful, reflective practice. By incorporating reflection into your coding routine, you’re not just solving problems; you’re building a deeper understanding of programming concepts, developing more efficient problem-solving strategies, and cultivating a growth mindset that will serve you well throughout your coding journey.
Remember, reflection is a skill in itself, one that improves with consistent practice. Start small, be patient with yourself, and gradually make reflection an integral part of your learning process. Whether you’re a beginner just starting out or an experienced developer preparing for technical interviews at top tech companies, the habit of reflection will be a powerful tool in your programming arsenal.
As you continue your journey with platforms like AlgoCademy, challenge yourself to not just solve problems, but to truly understand and learn from each coding experience. Embrace the power of reflection, and watch as your problem-solving skills and coding proficiency reach new heights. Happy coding, and even happier reflecting!