How Often Does the Trie Data Structure Appear in Coding Interviews?
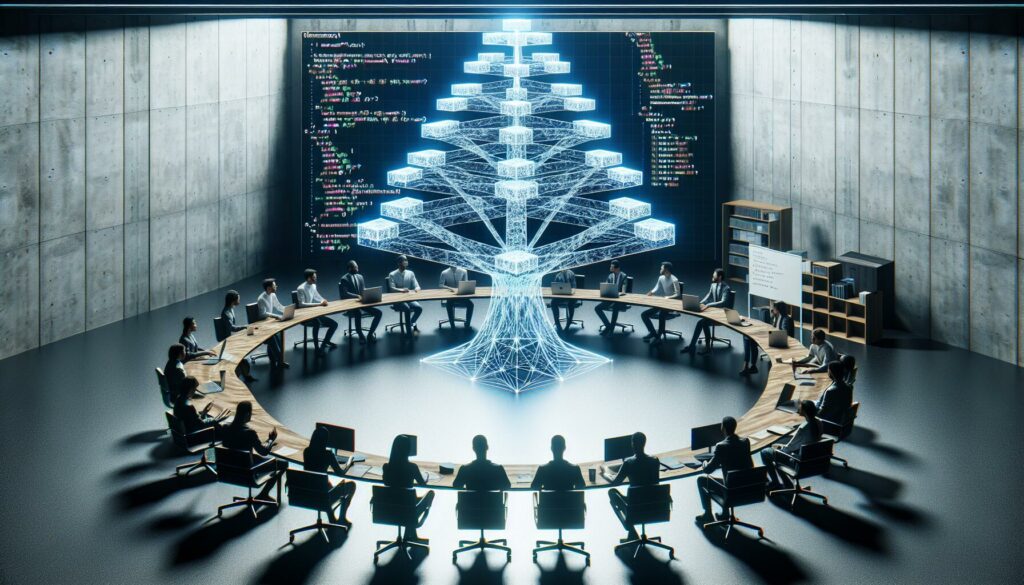
In the world of coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), certain data structures and algorithms tend to appear more frequently than others. One such data structure that often pops up is the Trie, also known as a prefix tree. But just how common is the Trie in coding interviews, and why is it important for aspiring software engineers to understand this structure? Let’s dive deep into the world of Tries and their relevance in technical interviews.
Understanding the Trie Data Structure
Before we discuss its frequency in interviews, let’s briefly recap what a Trie is and why it’s useful.
A Trie is a tree-like data structure used to store and retrieve strings efficiently. The name “Trie” comes from the word “retrieval,” highlighting its primary purpose. Each node in a Trie represents a character, and the path from the root to a node forms a prefix of one or more strings stored in the structure.
Here’s a simple representation of a Trie storing the words “cat,” “car,” and “dog”:
root
/ | \
c d ...
/ \ \
a ... o
/ \ g
t r
Tries are particularly efficient for tasks involving string operations, such as prefix matching, autocomplete suggestions, and spell checking.
Frequency of Trie Questions in Coding Interviews
While it’s challenging to provide an exact percentage, Trie-related questions do appear with moderate frequency in coding interviews, especially at top tech companies. Here’s a breakdown of why and how often you might encounter Trie questions:
1. FAANG and Other Tech Giants
Companies like Google, Amazon, and Facebook are known to ask Trie-related questions in their interviews. These companies deal with massive amounts of data and often require efficient string processing, making Tries a relevant topic. You can expect to see Trie questions in about 10-15% of their algorithm-focused interviews.
2. Startups and Smaller Companies
Startups and smaller tech companies might ask about Tries less frequently, perhaps in 5-10% of their coding interviews. However, this can vary greatly depending on the company’s focus and the specific role you’re applying for.
3. Specific Roles
If you’re interviewing for roles that involve heavy text processing, natural language processing, or search functionality, the likelihood of encountering Trie questions increases significantly, potentially up to 20-25% of algorithm questions.
Why Tries are Important in Coding Interviews
Understanding why interviewers ask about Tries can help you appreciate their importance:
1. Efficiency in String Operations
Tries offer excellent time complexity for operations like insert, search, and delete, typically O(m) where m is the length of the string. This efficiency is crucial when dealing with large datasets of strings.
2. Problem-Solving Skills
Trie questions often require candidates to think creatively about string manipulation and tree traversal, showcasing problem-solving abilities.
3. Real-World Applications
Tries have practical applications in areas like autocomplete systems, spell checkers, and IP routing tables, making them relevant to many tech companies’ products.
4. Algorithmic Knowledge
Understanding Tries demonstrates a broader knowledge of advanced data structures, which is valuable for roles requiring strong algorithmic skills.
Common Trie-Related Interview Questions
To give you a better idea of what to expect, here are some common Trie-related questions that might appear in coding interviews:
- Implement a Trie data structure with insert, search, and startsWith methods.
- Design an autocomplete system using a Trie.
- Find the longest common prefix among a set of strings using a Trie.
- Implement a spell checker using a Trie.
- Design a data structure for adding and searching words, allowing for ‘.’ to match any letter.
- Count the number of strings with a given prefix in a set of strings.
- Implement a word dictionary with add and search functionalities.
Preparing for Trie Questions in Interviews
Given the moderate frequency of Trie questions in coding interviews, it’s wise to be well-prepared. Here are some tips to help you master Tries:
1. Understand the Basics
Make sure you have a solid grasp of the Trie structure, including how to implement it and its basic operations. Here’s a simple implementation in Python:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
2. Practice Implementation
Implement a Trie from scratch in your preferred programming language. This exercise will help you understand the structure deeply and prepare you for coding it during an interview.
3. Solve Related Problems
Work through Trie-related problems on platforms like LeetCode, HackerRank, or AlgoCademy. Start with easier problems and gradually move to more complex ones.
4. Understand Time and Space Complexity
Be prepared to discuss the time and space complexity of Trie operations. For example:
- Insert: O(m) time, where m is the length of the word
- Search: O(m) time
- Space Complexity: O(n * m), where n is the number of words and m is the average length of words
5. Learn About Variations
Familiarize yourself with variations of the Trie, such as compressed Tries (also known as radix trees) and ternary search trees.
6. Understand Real-World Applications
Be ready to discuss practical applications of Tries, such as in autocomplete systems, spell checkers, or IP routing tables.
Trie vs. Other Data Structures
Understanding how Tries compare to other data structures can help you explain why you might choose a Trie in certain scenarios:
Trie vs. Hash Table
While both can be used for string storage and retrieval, Tries excel in prefix-based operations and space efficiency for certain datasets.
- Tries are more efficient for prefix matching and autocomplete scenarios.
- Hash tables might be faster for exact string matching but don’t support prefix operations efficiently.
- Tries can be more space-efficient when storing many strings with common prefixes.
Trie vs. Binary Search Tree (BST)
Both are tree structures, but Tries are optimized for string operations.
- Tries offer O(m) time complexity for string operations, where m is the length of the string.
- BSTs typically have O(m log n) time complexity for string operations, where n is the number of strings.
- Tries are more efficient for prefix matching and lexicographical ordering of strings.
Advanced Trie Concepts
To truly excel in Trie-related interview questions, consider exploring these advanced concepts:
1. Compressed Tries (Radix Trees)
Compressed Tries optimize space usage by merging nodes with single children. This variation is useful when dealing with sparse datasets or long common prefixes.
2. Ternary Search Trees
A hybrid between binary search trees and Tries, ternary search trees can be more space-efficient than standard Tries while maintaining good performance for string operations.
3. Suffix Trees and Suffix Arrays
While not Tries in the strictest sense, these related structures are often used for advanced string matching problems and can sometimes appear in challenging interview questions.
Real-World Applications of Tries
Understanding the practical applications of Tries can help you discuss their relevance in a real-world context during interviews:
1. Autocomplete and Type-Ahead Systems
Tries are excellent for implementing autocomplete functionality in search engines, text editors, and mobile keyboards. They allow for quick retrieval of all words with a given prefix.
2. Spell Checkers
Tries can efficiently store a dictionary of valid words and quickly check if a given word exists or suggest corrections for misspelled words.
3. IP Routing Tables
In computer networking, Tries are used to implement routing tables for IP address lookups, allowing for efficient longest prefix matching.
4. Dictionary Implementation
Tries can be used to implement dictionaries with fast lookup times, especially useful for applications requiring prefix-based searches.
5. Natural Language Processing
In NLP applications, Tries can be used for efficient storage and retrieval of large vocabularies, aiding in tasks like tokenization and word prediction.
Optimizing Trie Implementations
In coding interviews, you might be asked to optimize a basic Trie implementation. Here are some techniques to consider:
1. Memory Optimization
Instead of using a fixed-size array for children nodes, consider using a hash map to save space, especially for sparse datasets.
2. Lazy Expansion
Create child nodes only when needed, rather than initializing all possible children upfront.
3. Path Compression
For long chains of single-child nodes, compress them into a single node to save space.
4. Caching
For frequently accessed prefixes or words, implement a caching mechanism to improve performance.
Conclusion
While Tries may not be the most common data structure in coding interviews, they appear frequently enough to warrant serious attention, especially for roles at major tech companies or positions involving significant text processing. Their efficiency in handling string operations and their practical applications in real-world scenarios make them a valuable tool in any software engineer’s arsenal.
By understanding Tries thoroughly, practicing their implementation, and solving related problems, you’ll be well-prepared to tackle any Trie-related questions that come your way in coding interviews. Remember, the goal isn’t just to memorize the structure but to understand its strengths, weaknesses, and appropriate use cases.
As you continue your journey in coding education and interview preparation, platforms like AlgoCademy can be invaluable resources. They offer interactive coding tutorials, problem-solving exercises, and AI-powered assistance to help you master data structures like Tries and develop the algorithmic thinking necessary to excel in technical interviews.
Keep practicing, stay curious, and approach each problem as an opportunity to showcase your problem-solving skills. With dedication and the right resources, you’ll be well-equipped to handle any Trie question – and indeed, any data structure challenge – that comes your way in your coding interviews.