How Learning a Second Programming Language Changes the Way You Approach Problems
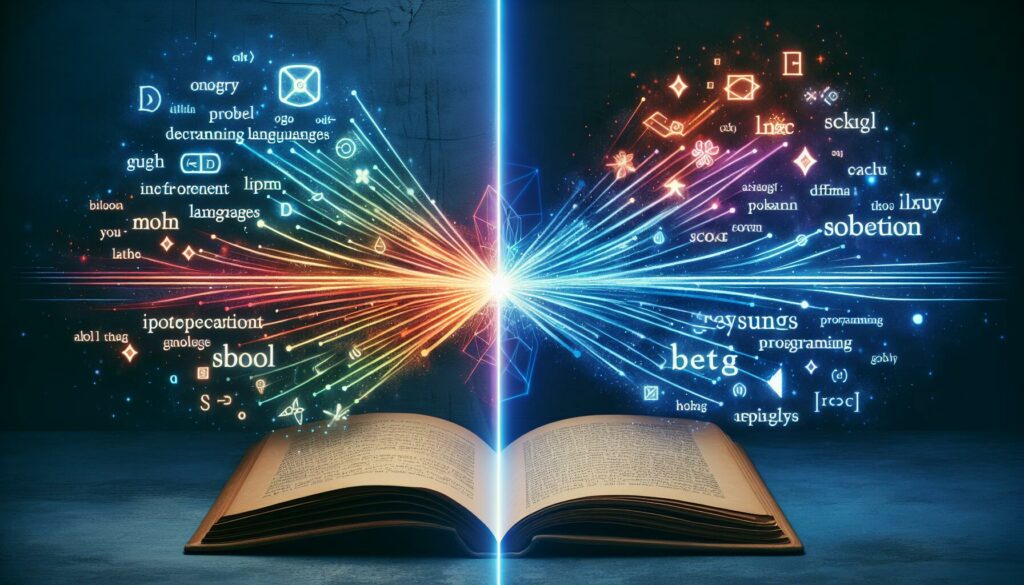
In the ever-evolving world of technology, programming languages serve as the building blocks for innovation and problem-solving. As a coder, mastering one language is an accomplishment, but venturing into the realm of a second programming language can be transformative. It’s not just about adding another tool to your toolkit; it’s about reshaping your entire approach to coding and problem-solving. In this comprehensive guide, we’ll explore how learning a second programming language can dramatically alter your perspective and enhance your capabilities as a developer.
The Importance of Multilingual Programming Skills
Before we dive into the specific ways learning a second language changes your approach, let’s consider why multilingual programming skills are so valuable in today’s tech landscape:
- Versatility: Different languages are optimized for different tasks. Being proficient in multiple languages allows you to choose the best tool for each job.
- Career Opportunities: Many employers seek developers who can work across multiple languages, especially in full-stack development roles.
- Problem-Solving Flexibility: Each language has its own paradigms and best practices, which can inform your approach to problems in novel ways.
- Deeper Understanding: Learning multiple languages helps you grasp fundamental programming concepts that transcend specific syntaxes.
Expanding Your Mental Model of Programming
When you learn your first programming language, you’re not just learning syntax; you’re building a mental model of how programming works. This model is heavily influenced by the paradigms and features of that first language. Learning a second language challenges and expands this mental model in several ways:
1. Paradigm Shifts
Different programming languages often embody different programming paradigms. For example, if you started with an object-oriented language like Java and then learn a functional language like Haskell, you’ll experience a significant paradigm shift. This can lead to:
- A new understanding of data and function relationships
- Different approaches to managing state and side effects
- Novel ways of structuring and organizing code
Consider this simple example of calculating the sum of squares in Java (object-oriented) versus Haskell (functional):
Java:
public class SumOfSquares {
public static int sumOfSquares(int[] numbers) {
int sum = 0;
for (int num : numbers) {
sum += num * num;
}
return sum;
}
}
Haskell:
sumOfSquares :: [Int] -> Int
sumOfSquares numbers = sum (map (^2) numbers)
The Haskell version leverages functional concepts like higher-order functions and lazy evaluation, which can lead to more concise and potentially more efficient code in certain scenarios.
2. Syntax Flexibility
As you become comfortable with multiple syntaxes, you’ll find yourself more adaptable to new languages and frameworks. This flexibility allows you to:
- Pick up new languages more quickly
- Focus more on problem-solving rather than syntax details
- Appreciate the trade-offs between verbosity and conciseness in different languages
3. Abstraction Levels
Different languages operate at different levels of abstraction. Moving between high-level and low-level languages can significantly impact how you think about problems. For instance:
- A high-level language like Python might abstract away memory management
- A low-level language like C requires explicit memory handling
Understanding these differences helps you make more informed decisions about when to use each level of abstraction in your projects.
Enhanced Problem-Solving Techniques
Learning a second programming language doesn’t just change how you write code; it transforms how you approach problems from the outset. Here are some ways your problem-solving techniques may evolve:
1. Multiple Solution Pathways
With knowledge of multiple languages, you’ll start to see multiple potential solutions to a single problem. This can lead to:
- More creative and efficient problem-solving
- The ability to choose the most appropriate solution based on project constraints
- A deeper understanding of algorithmic trade-offs
For example, consider the problem of implementing a web server. In a language like Python, you might use a high-level framework like Flask:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, World!"
if __name__ == "__main__":
app.run()
While in Node.js, you might opt for a more low-level approach using the built-in http module:
const http = require("http");
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader("Content-Type", "text/plain");
res.end("Hello, World!");
});
server.listen(3000, "localhost", () => {
console.log(`Server running at http://localhost:3000/`);
});
Each approach has its merits, and understanding both allows you to make informed decisions based on project requirements.
2. Performance Optimization
Different languages have different performance characteristics. Learning multiple languages helps you:
- Identify potential performance bottlenecks more easily
- Choose the right language for performance-critical parts of your application
- Understand the trade-offs between development speed and runtime performance
3. Design Pattern Recognition
As you encounter similar problems across different languages, you’ll start to recognize common design patterns. This recognition allows you to:
- Apply proven solutions to common problems more quickly
- Communicate ideas more effectively with other developers
- Structure your code in more maintainable and scalable ways
Broadening Your Toolset
Each programming language comes with its own ecosystem of tools, libraries, and frameworks. Learning a second language exposes you to a whole new set of tools, which can significantly impact how you approach development tasks:
1. Diverse Development Environments
Different languages often have different preferred Integrated Development Environments (IDEs) or text editors. Exposure to various development environments can:
- Improve your productivity through advanced IDE features
- Help you understand the importance of tooling in the development process
- Make you more adaptable to different project setups
2. New Testing Frameworks
Testing practices and frameworks can vary significantly between languages. Learning these differences can lead to:
- More robust testing strategies
- A deeper understanding of test-driven development (TDD) principles
- The ability to choose the most appropriate testing approach for each project
For instance, compare the testing approaches in Python using unittest:
import unittest
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual("foo".upper(), "FOO")
if __name__ == "__main__":
unittest.main()
With JavaScript using Jest:
test("string uppercase", () => {
expect("foo".toUpperCase()).toBe("FOO");
});
3. Package Management and Dependency Handling
Different languages have different approaches to managing dependencies and packages. Understanding these differences can help you:
- Make better decisions about project structure and organization
- Manage dependencies more effectively across different projects
- Appreciate the importance of ecosystem health in language choice
Enhancing Code Quality and Maintainability
Learning a second programming language often leads to improvements in overall code quality and maintainability. Here’s how:
1. Best Practices Cross-Pollination
Each language community has its own set of best practices and coding standards. Exposure to multiple communities allows you to:
- Adopt the best practices from each language
- Develop a more nuanced understanding of what makes code “good”
- Apply universal principles of clean code across languages
2. Code Organization and Structure
Different languages often have different conventions for organizing code. Learning these differences can help you:
- Structure your projects more effectively
- Improve code readability and maintainability
- Collaborate more effectively in diverse development teams
3. Documentation Practices
Documentation styles and tools can vary between languages. Exposure to different documentation practices can lead to:
- More comprehensive and useful documentation in your projects
- Better understanding of how to read and use documentation effectively
- Improved communication of code intent and functionality
Cultivating a Growth Mindset
Perhaps one of the most significant changes that comes from learning a second programming language is the cultivation of a growth mindset. This shift in perspective can have far-reaching effects on your development career:
1. Embracing Challenges
The process of learning a new language teaches you to:
- Embrace the discomfort of not knowing everything
- See challenges as opportunities for growth rather than obstacles
- Approach new technologies with curiosity and enthusiasm
2. Continuous Learning
The tech industry is constantly evolving, and learning a second language reinforces the importance of continuous learning. This mindset helps you:
- Stay current with industry trends and best practices
- Adapt more quickly to new technologies and paradigms
- Remain competitive in the job market
3. Broader Perspective
Exposure to multiple programming paradigms and communities broadens your perspective, allowing you to:
- Appreciate the strengths and weaknesses of different approaches
- Contribute more effectively to cross-functional teams
- Make more informed decisions about technology choices
Practical Steps for Learning a Second Language
If you’re convinced of the benefits and ready to embark on learning a second programming language, here are some practical steps to get started:
1. Choose Wisely
Select a language that:
- Complements your current skill set
- Aligns with your career goals or interests
- Introduces you to new programming paradigms
2. Start with the Basics
Even if you’re an experienced programmer, don’t skip the fundamentals. Understanding the basic syntax and structure of the new language is crucial.
3. Build Projects
Apply your new knowledge by building small projects. This hands-on experience is invaluable for solidifying your understanding.
4. Engage with the Community
Join online forums, attend meetups, or contribute to open-source projects in your new language. This engagement can accelerate your learning and provide valuable insights.
5. Compare and Contrast
Actively compare how you would solve problems in your first language versus your new language. This comparative analysis deepens your understanding of both languages.
Conclusion
Learning a second programming language is more than just adding another skill to your resume. It’s a transformative experience that can fundamentally change how you approach problems, write code, and think about software development. The expanded mental model, enhanced problem-solving techniques, and broader toolset you gain can make you a more versatile, effective, and insightful developer.
As you embark on this journey, remember that the goal isn’t just to learn syntax, but to embrace new ways of thinking. Each language you learn opens up new possibilities and perspectives, contributing to your growth as a developer and problem-solver.
In the words of the Zen of Python: “There should be one– and preferably only one –obvious way to do it.” But by learning multiple languages, you’ll discover that there are often multiple “obvious” ways, each with its own merits. This understanding is what truly sets apart versatile, adaptable developers in today’s diverse technological landscape.
So, whether you’re considering learning your second language or your fifth, embrace the challenge. The journey of learning a new programming language is an investment in your future as a developer, opening doors to new opportunities, insights, and ways of solving the complex problems of our digital world.