How Important is Big O Notation in Coding Interviews?
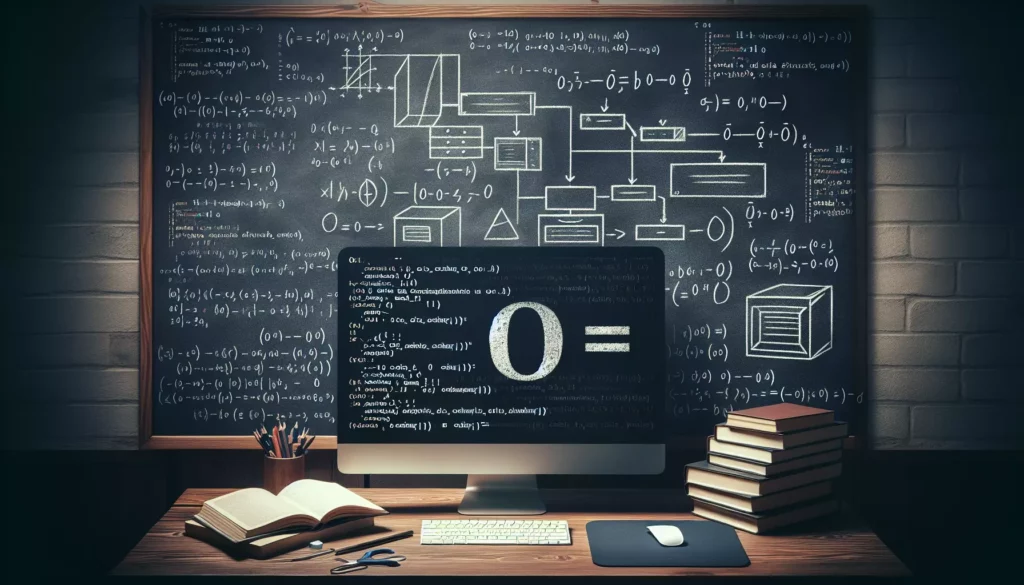
When preparing for coding interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), you’ll often come across the term “Big O notation.” But just how important is this concept, and why do interviewers place such emphasis on it? In this comprehensive guide, we’ll explore the significance of Big O notation in coding interviews, its practical applications, and how you can master this crucial skill to ace your next technical interview.
Understanding Big O Notation
Before we dive into its importance, let’s briefly recap what Big O notation actually is. Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. It specifically describes the worst-case scenario, or the maximum time an algorithm will take to complete.
The “O” in Big O notation stands for “Order of,” which refers to the order of magnitude of complexity. Some common Big O notations include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Each of these notations represents how the runtime of an algorithm grows as the input size increases. For instance, an O(n) algorithm’s runtime grows linearly with the input size, while an O(n^2) algorithm’s runtime grows quadratically.
The Importance of Big O Notation in Coding Interviews
Now that we’ve refreshed our understanding of Big O notation, let’s explore why it’s so crucial in coding interviews:
1. Demonstrates Efficiency Thinking
When interviewers ask about Big O notation, they’re not just testing your memorization skills. They want to see if you can think critically about the efficiency of your code. By discussing the time and space complexity of your solutions, you show that you’re considering the performance implications of your algorithms, which is a crucial skill in real-world software development.
2. Helps in Comparing Algorithms
Big O notation provides a standardized way to compare different algorithms. When you’re able to analyze and discuss the time complexity of various solutions to a problem, you demonstrate your ability to choose the most appropriate algorithm for a given situation. This skill is invaluable in software engineering, where choosing the right algorithm can significantly impact the performance of an application.
3. Indicates Problem-Solving Skills
Understanding Big O notation often goes hand-in-hand with strong problem-solving skills. When you can break down a problem, consider different approaches, and analyze their efficiencies, you’re showcasing your ability to think algorithmically. This is a key skill that interviewers look for, as it translates directly to real-world scenarios where you’ll need to solve complex problems efficiently.
4. Reflects Real-World Concerns
In the real world of software development, efficiency matters. Applications need to handle large amounts of data and perform operations quickly. By demonstrating your understanding of Big O notation, you show that you’re mindful of these real-world concerns and can write code that scales well.
5. Common Language in Tech
Big O notation serves as a common language among software engineers. Being fluent in this “language” shows that you can effectively communicate about algorithm efficiency with your potential future colleagues. This is particularly important in collaborative environments where discussing and optimizing code is a regular occurrence.
Practical Applications of Big O Notation
To further emphasize the importance of Big O notation, let’s look at some practical applications:
1. Optimizing Database Queries
When working with databases, understanding the time complexity of your queries can make a huge difference. For example, knowing that a nested loop join has a time complexity of O(n^2) while a hash join can achieve O(n) can help you write more efficient database operations.
2. Choosing Data Structures
Different data structures have different time complexities for various operations. Understanding these can help you choose the most appropriate structure for your needs. For instance:
- Arrays have O(1) access time but O(n) insertion time (at the beginning)
- Linked lists have O(n) access time but O(1) insertion time (at the beginning)
- Hash tables offer O(1) average case for insertion and lookup
3. Scaling Applications
As applications grow and handle more data, algorithms with poor time complexity can become bottlenecks. Understanding Big O notation helps you anticipate these issues and design solutions that scale well from the start.
4. Optimizing Algorithms
When faced with a performance problem, Big O analysis can help you identify where the bottleneck is and guide you towards more efficient solutions. For example, you might realize that your O(n^2) sorting algorithm is the culprit and opt for a more efficient O(n log n) algorithm instead.
Common Big O Notations and Their Meanings
Let’s dive deeper into some common Big O notations you’re likely to encounter in coding interviews:
O(1) – Constant Time
This is the holy grail of time complexity. It means that the algorithm will always execute in the same time (or space) regardless of the input size. Examples include:
- Accessing an array element by index
- Inserting a node at the beginning of a linked list
- Push and pop operations on a stack
O(log n) – Logarithmic Time
Algorithms with logarithmic time complexity are very efficient, especially for large inputs. They typically involve dividing the problem in half with each step. Examples include:
- Binary search
- Certain divide-and-conquer algorithms
- Balanced binary tree operations
O(n) – Linear Time
Linear time complexity means the runtime grows directly in proportion to the input size. While not as efficient as O(1) or O(log n), it’s still considered reasonably efficient. Examples include:
- Traversing an array or linked list
- Linear search
- Finding the maximum or minimum in an unsorted array
O(n log n) – Linearithmic Time
This time complexity is common in efficient sorting algorithms. It’s more efficient than O(n^2) but less efficient than O(n). Examples include:
- Merge sort
- Heap sort
- Quick sort (average case)
O(n^2) – Quadratic Time
Quadratic time complexity is often a red flag in interviews, especially for large inputs. It typically involves nested iterations over the input. Examples include:
- Bubble sort
- Insertion sort
- Comparing each element in an array to every other element
O(2^n) – Exponential Time
Exponential time complexity is typically seen in algorithms that solve problems through brute-force search of all possibilities. These algorithms are generally impractical for large inputs. Examples include:
- Recursive calculation of Fibonacci numbers
- The traveling salesman problem solved by brute-force
- Generating all subsets of a set
How to Improve Your Big O Analysis Skills
Now that we’ve established the importance of Big O notation in coding interviews, you might be wondering how to improve your skills in this area. Here are some strategies:
1. Practice, Practice, Practice
The more problems you solve and analyze, the better you’ll become at recognizing patterns and quickly determining time complexity. Platforms like AlgoCademy offer a wealth of problems to practice on, often with built-in complexity analysis tools.
2. Analyze Common Algorithms
Familiarize yourself with the time and space complexity of common algorithms and data structures. This includes sorting algorithms, search algorithms, and operations on various data structures like arrays, linked lists, trees, and graphs.
3. Understand the Basics of Algorithm Analysis
Learn the fundamental principles of algorithm analysis. This includes concepts like best case, average case, and worst case scenarios, as well as how to analyze loops, recursive functions, and combined complexities.
4. Use Visualization Tools
There are many online tools and websites that can help you visualize how different algorithms perform with varying input sizes. These can be incredibly helpful in building your intuition about time complexity.
5. Implement and Experiment
Don’t just theorize about Big O notation. Implement algorithms yourself and experiment with different input sizes. This hands-on experience can provide valuable insights into how algorithms perform in practice.
Common Pitfalls in Big O Analysis
As you work on improving your Big O analysis skills, be aware of these common pitfalls:
1. Focusing Only on Time Complexity
While time complexity is crucial, don’t forget about space complexity. Sometimes, an algorithm might be time-efficient but memory-intensive, which can be problematic in certain scenarios.
2. Ignoring Constants
In Big O notation, we typically ignore constants. For example, O(2n) is simplified to O(n). However, in some real-world scenarios, these constants can make a significant difference, especially for smaller input sizes.
3. Overlooking Average Case
Big O notation typically describes the worst-case scenario. However, the average case can be just as important in real-world applications. Make sure you consider both.
4. Misunderstanding Nested Loops
A common mistake is assuming that all nested loops result in O(n^2) complexity. This isn’t always the case. For example, two nested loops where the inner loop runs a constant number of times is still O(n), not O(n^2).
5. Forgetting About Input Size
The efficiency of an algorithm can change dramatically based on the size of the input. An algorithm that’s efficient for small inputs might be impractical for large datasets.
Big O Notation in Different Programming Languages
While the principles of Big O notation are language-agnostic, different programming languages and their standard libraries can affect the actual performance of algorithms. Here’s a brief overview of how Big O notation applies in some popular languages:
Python
Python’s built-in data structures and operations have known time complexities. For example:
- List index access: O(1)
- List append: O(1) amortized
- List sort: O(n log n)
- Dictionary get/set: O(1) average case
Here’s an example of a simple O(n) operation in Python:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
Java
Java’s Collections framework provides different implementations with varying time complexities:
- ArrayList get/set: O(1)
- LinkedList get: O(n), add: O(1)
- HashMap get/put: O(1) average case
- TreeMap get/put: O(log n)
Here’s an example of an O(n^2) operation in Java:
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1]) {
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
JavaScript
JavaScript’s array methods have specific time complexities:
- Array push/pop: O(1)
- Array shift/unshift: O(n)
- Array sort: O(n log n)
- Object property access: O(1) average case
Here’s an example of an O(log n) operation in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Conclusion: Mastering Big O Notation for Interview Success
In the world of coding interviews, particularly for prestigious tech companies, Big O notation is not just important—it’s essential. It’s a fundamental concept that demonstrates your ability to think critically about code efficiency, compare algorithms, and solve problems effectively. Moreover, it reflects real-world concerns in software development and serves as a common language among engineers.
To excel in your coding interviews, invest time in understanding and practicing Big O analysis. Work through a variety of problems, analyze common algorithms, and don’t shy away from implementing and experimenting with different approaches. Remember to consider both time and space complexity, and be aware of the common pitfalls in Big O analysis.
While mastering Big O notation is crucial, it’s just one piece of the puzzle. Combine this knowledge with strong problem-solving skills, a solid understanding of data structures, and proficiency in your chosen programming language. Platforms like AlgoCademy can be invaluable in this journey, offering a structured approach to learning these concepts and applying them to interview-style problems.
With dedication and practice, you can develop the skills needed to confidently discuss algorithm efficiency in your next coding interview. Remember, the goal isn’t just to recite Big O notations, but to demonstrate a deep understanding of how to write efficient, scalable code. This skill will not only help you ace your interviews but will also make you a more effective software engineer in your future career.
So, embrace the challenge of mastering Big O notation. It’s a powerful tool that will serve you well throughout your coding journey, from interview rooms to real-world software development projects. Happy coding, and best of luck in your future interviews!