High-Performance Computing (HPC): Optimizing Computational Tasks for Speed and Efficiency
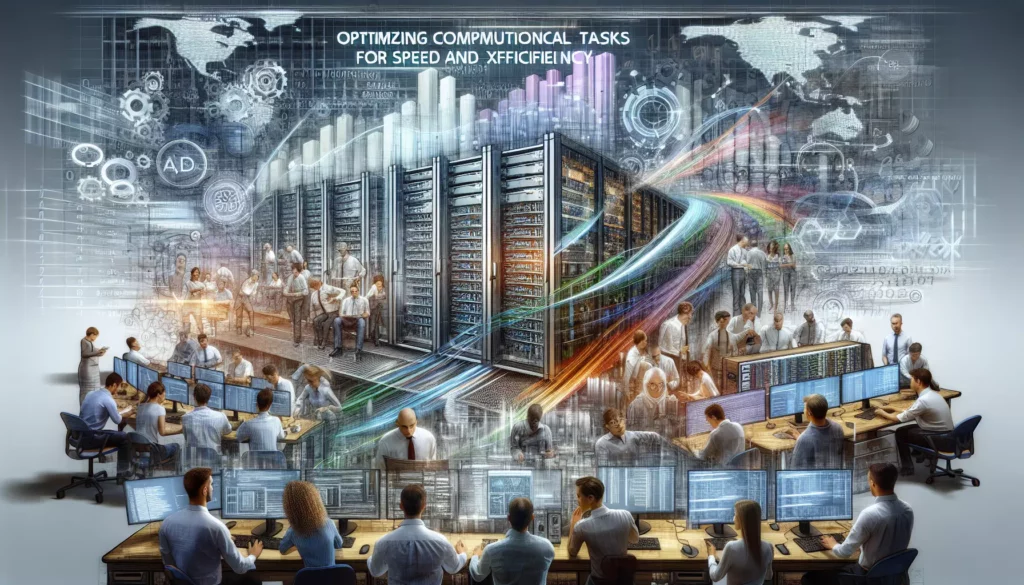
In today’s data-driven world, the ability to process vast amounts of information quickly and efficiently is crucial. This is where High-Performance Computing (HPC) comes into play. HPC is not just about having powerful hardware; it’s about optimizing computational tasks to achieve maximum speed and efficiency. In this comprehensive guide, we’ll dive deep into the world of HPC, exploring its principles, applications, and how you can leverage it to enhance your coding skills and solve complex problems.
What is High-Performance Computing?
High-Performance Computing refers to the practice of aggregating computing power to deliver much higher performance than a typical desktop computer or workstation. This is done to solve large problems in science, engineering, or business. HPC systems are typically composed of thousands of compute nodes working in parallel to complete tasks.
The key aspects of HPC include:
- Parallel processing
- Distributed computing
- Optimized algorithms
- Efficient resource management
- Scalability
Why is HPC Important?
HPC is crucial in many fields, including:
- Scientific research (e.g., climate modeling, genomics)
- Engineering simulations (e.g., aerospace, automotive)
- Financial modeling
- Big data analytics
- Artificial Intelligence and Machine Learning
- Cryptography
For programmers and software developers, understanding HPC principles can lead to more efficient code, better resource utilization, and the ability to tackle larger, more complex problems.
Key Concepts in High-Performance Computing
1. Parallel Processing
Parallel processing is at the heart of HPC. It involves breaking down a problem into smaller parts that can be solved simultaneously. There are different levels of parallelism:
- Bit-level parallelism
- Instruction-level parallelism
- Data parallelism
- Task parallelism
Understanding these concepts helps in designing algorithms that can effectively utilize parallel computing resources.
2. Distributed Computing
Distributed computing involves spreading computations across multiple machines. This is essential for tackling problems that are too large for a single computer. Concepts like Message Passing Interface (MPI) and MapReduce are fundamental to distributed computing in HPC.
3. Scalability
Scalability refers to a system’s ability to handle increased workload by adding resources. In HPC, we often talk about:
- Strong scaling: Solving a fixed-size problem faster by using more processors
- Weak scaling: Solving a larger problem in the same amount of time by using more processors
4. Performance Optimization
Optimizing code for performance is crucial in HPC. This includes:
- Algorithmic optimization
- Memory optimization
- I/O optimization
- Compiler optimizations
HPC Architecture and Infrastructure
1. Supercomputers
Supercomputers are the pinnacle of HPC. They are massive systems with thousands of interconnected nodes, each containing multiple processors. Examples include IBM Summit and Fugaku.
2. Clusters
Clusters are groups of computers working together as a single system. They are more accessible and scalable than supercomputers, making them popular in many organizations.
3. Grid Computing
Grid computing involves connecting geographically distributed resources to solve large-scale problems. It’s like a “virtual supercomputer” created by networked, loosely coupled computers.
4. Cloud HPC
Cloud platforms now offer HPC capabilities, allowing organizations to access high-performance resources without investing in expensive hardware. Services like Amazon EC2, Google Cloud Platform, and Microsoft Azure provide scalable HPC solutions.
Programming for HPC
1. Parallel Programming Models
Several models and APIs are used for parallel programming in HPC:
- MPI (Message Passing Interface)
- OpenMP
- CUDA (for GPU computing)
- OpenCL
2. Languages for HPC
While any language can be used for HPC, some are more common due to their performance characteristics:
- C and C++
- Fortran
- Python (with libraries like NumPy and SciPy)
- Julia
3. Example: Parallel Matrix Multiplication in C with OpenMP
Here’s a simple example of how you might implement parallel matrix multiplication using OpenMP in C:
#include <stdio.h>
#include <omp.h>
#define N 1000
void matrix_multiply(int A[N][N], int B[N][N], int C[N][N]) {
#pragma omp parallel for
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
C[i][j] = 0;
for (int k = 0; k < N; k++) {
C[i][j] += A[i][k] * B[k][j];
}
}
}
}
int main() {
int A[N][N], B[N][N], C[N][N];
// Initialize matrices A and B (omitted for brevity)
double start_time = omp_get_wtime();
matrix_multiply(A, B, C);
double end_time = omp_get_wtime();
printf("Time taken: %f seconds\n", end_time - start_time);
return 0;
}
This example uses OpenMP to parallelize the outer loop of the matrix multiplication algorithm, potentially offering significant speedup on multi-core systems.
Optimizing Algorithms for HPC
When working with HPC, it’s crucial to optimize your algorithms for parallel execution. Here are some strategies:
1. Data Partitioning
Divide your data into chunks that can be processed independently. This is the foundation of data parallelism.
2. Load Balancing
Ensure that work is distributed evenly across all available processors to maximize efficiency.
3. Minimizing Communication
In distributed systems, communication between nodes can be a bottleneck. Design your algorithms to minimize necessary data transfer.
4. Vectorization
Use vector operations where possible to take advantage of SIMD (Single Instruction, Multiple Data) capabilities of modern processors.
5. Memory Access Optimization
Optimize data structures and access patterns to make efficient use of cache and reduce memory latency.
HPC in Practice: Real-World Applications
1. Weather Forecasting
Weather models require processing vast amounts of data and performing complex calculations. HPC systems allow meteorologists to create more accurate and timely forecasts.
2. Genomics
Sequencing and analyzing genomes involves processing terabytes of data. HPC accelerates this process, enabling breakthroughs in personalized medicine.
3. Financial Modeling
Banks and financial institutions use HPC for risk analysis, fraud detection, and high-frequency trading algorithms.
4. Film Animation
Rendering complex 3D animations requires immense computational power. Studios use HPC clusters to generate stunning visual effects.
5. Drug Discovery
Pharmaceutical companies use HPC to simulate molecular interactions, speeding up the process of identifying potential new drugs.
Challenges in HPC
1. Power Consumption
HPC systems consume enormous amounts of energy. Developing more energy-efficient systems is an ongoing challenge.
2. Heat Dissipation
With great power comes great heat. Cooling HPC systems efficiently is a significant engineering challenge.
3. Programming Complexity
Writing efficient parallel code is challenging and requires specialized skills.
4. Data Management
Managing and moving large datasets efficiently is a constant challenge in HPC environments.
Future Trends in HPC
1. Exascale Computing
The next frontier in HPC is exascale computing – systems capable of performing a quintillion (10^18) calculations per second.
2. Quantum Computing
Quantum computers promise to solve certain problems exponentially faster than classical computers, potentially revolutionizing fields like cryptography and molecular simulation.
3. AI and HPC Convergence
The lines between HPC and AI are blurring, with many HPC applications incorporating machine learning techniques and vice versa.
4. Edge HPC
Bringing HPC capabilities closer to the data source, especially for IoT and real-time applications.
Getting Started with HPC
If you’re interested in exploring HPC further, here are some steps you can take:
- Learn parallel programming concepts and models (MPI, OpenMP)
- Practice with multi-threading in your preferred programming language
- Experiment with GPU computing using CUDA or OpenCL
- Set up a small cluster using Raspberry Pis or cloud instances
- Participate in online courses or workshops focused on HPC
- Contribute to open-source HPC projects
Conclusion
High-Performance Computing is a fascinating field at the intersection of computer science, engineering, and numerous application domains. As we continue to generate and process ever-increasing amounts of data, the importance of HPC will only grow. Whether you’re a student, a professional developer, or a researcher, understanding HPC principles can significantly enhance your ability to solve complex computational problems efficiently.
By mastering HPC concepts and techniques, you’ll be well-equipped to tackle the most challenging computational tasks of today and tomorrow. Remember, the journey into HPC is ongoing – technology evolves rapidly, and there’s always something new to learn. Keep exploring, experimenting, and pushing the boundaries of what’s computationally possible!