Happy Numbers: A Joyful Journey Through Mathematical Curiosities
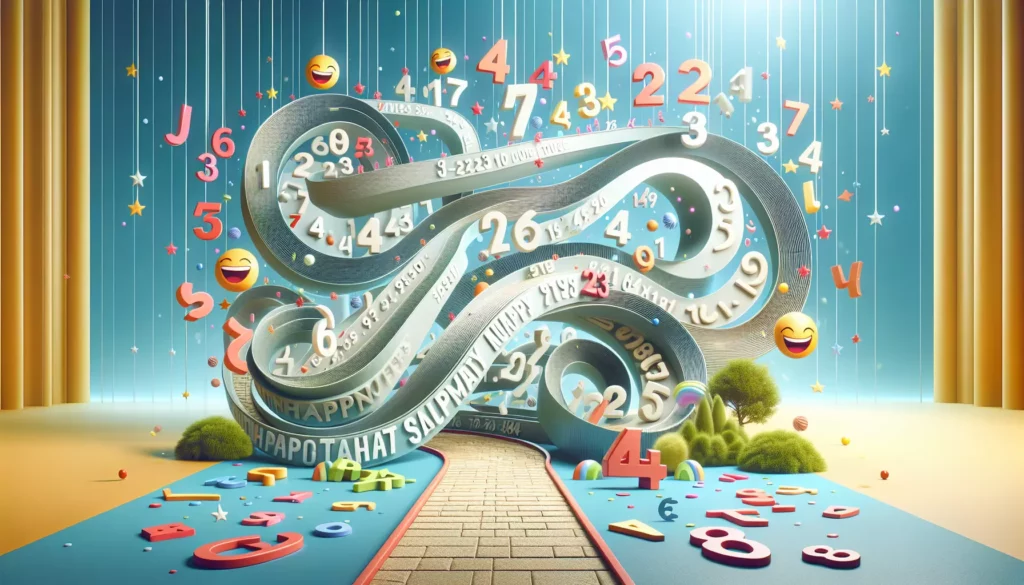
In the vast landscape of mathematics, there are countless intriguing concepts that capture our imagination. One such delightful notion is that of “happy numbers.” These numerical entities, with their quirky behavior and fascinating properties, offer a perfect blend of mathematical intrigue and programming challenge. In this comprehensive exploration, we’ll dive deep into the world of happy numbers, unraveling their mysteries and implementing algorithms to detect them.
What Are Happy Numbers?
Happy numbers are a special class of positive integers that follow a particular pattern when subjected to a specific process. The concept was introduced by R. K. Guy and J. H. Conway in the 1980s, and since then, it has been a source of fascination for mathematicians and programmers alike.
A number is considered “happy” if, when you repeatedly replace it with the sum of the squares of its digits, you eventually reach the number 1. If this process results in an endless cycle that does not include 1, then the number is called an “unhappy number” or a “sad number.”
The Process of Determining a Happy Number
Let’s break down the process step-by-step:
- Start with any positive integer.
- Calculate the sum of the squares of its digits.
- Replace the original number with this sum.
- Repeat steps 2 and 3 until either:
- The number becomes 1 (in which case it’s a happy number), or
- The process enters a cycle that does not include 1 (in which case it’s an unhappy number).
An Example: Is 19 a Happy Number?
Let’s apply this process to the number 19:
- 12 + 92 = 1 + 81 = 82
- 82 + 22 = 64 + 4 = 68
- 62 + 82 = 36 + 64 = 100
- 12 + 02 + 02 = 1 + 0 + 0 = 1
Since we’ve reached 1, we can conclude that 19 is indeed a happy number!
Implementing a Happy Number Detector in Python
Now that we understand what happy numbers are, let’s implement an algorithm to detect them. We’ll use Python for this implementation, as its simplicity and readability make it an excellent choice for demonstrating algorithmic concepts.
The Basic Algorithm
Here’s a straightforward implementation of a happy number detector:
def is_happy(n):
seen = set()
while n != 1:
n = sum(int(digit)**2 for digit in str(n))
if n in seen:
return False
seen.add(n)
return True
# Test the function
print(is_happy(19)) # Should print True
print(is_happy(4)) # Should print False
Let’s break down this implementation:
- We define a function
is_happy
that takes a numbern
as input. - We use a set
seen
to keep track of numbers we’ve encountered in the process. This helps us detect cycles. - We enter a while loop that continues until we either reach 1 or detect a cycle.
- Inside the loop, we calculate the sum of squares of digits using a list comprehension and the
sum
function. - We check if we’ve seen this number before. If so, we’ve entered a cycle, and the number is unhappy.
- If we haven’t seen the number, we add it to our set and continue the process.
- If we exit the loop (by reaching 1), the number is happy.
Optimizing the Algorithm
While the above implementation works correctly, we can optimize it in several ways:
- Avoiding string conversion: Instead of converting the number to a string to iterate over its digits, we can use integer division and modulo operations.
- Using Floyd’s cycle-finding algorithm: Also known as the “tortoise and hare” algorithm, this can detect cycles more efficiently.
Here’s an optimized version incorporating these improvements:
def sum_of_squares_of_digits(n):
total = 0
while n > 0:
digit = n % 10
total += digit ** 2
n //= 10
return total
def is_happy(n):
slow = fast = n
while True:
slow = sum_of_squares_of_digits(slow)
fast = sum_of_squares_of_digits(sum_of_squares_of_digits(fast))
if slow == fast:
return slow == 1
# Test the function
print(is_happy(19)) # Should print True
print(is_happy(4)) # Should print False
This optimized version uses Floyd’s cycle-finding algorithm, which uses two pointers moving at different speeds to detect cycles. It’s more space-efficient as it doesn’t need to store previously seen numbers.
Properties of Happy Numbers
Happy numbers have several interesting properties that make them a fascinating subject of study:
1. All Happy Numbers End in the Same Cycle
Regardless of the starting number, if a number is happy, it will always end up in the cycle: 1 → 1. This is why our algorithm stops when it reaches 1.
2. Unhappy Numbers End in the “Sad” Cycle
All unhappy numbers eventually enter a cycle that doesn’t include 1. This cycle is:
4 → 16 → 37 → 58 → 89 → 145 → 42 → 20 → 4
3. Happy Numbers in Different Bases
The concept of happy numbers can be extended to different number bases. Interestingly, 7 is the only number that is happy in all bases from 2 to 10.
4. Distribution of Happy Numbers
Happy numbers become increasingly sparse as numbers get larger. However, there are infinitely many happy numbers.
Applications and Extensions of Happy Numbers
While happy numbers might seem like a purely recreational mathematical concept, they have several interesting applications and extensions:
1. Cryptography and Random Number Generation
The process of generating happy numbers can be used as part of cryptographic algorithms or random number generators, as it introduces an element of unpredictability.
2. Teaching Programming Concepts
Happy numbers serve as an excellent example for teaching various programming concepts:
- Loop structures
- Recursion
- Cycle detection algorithms
- Optimizing code for performance
3. Mathematical Research
Happy numbers open up several avenues for mathematical research, including:
- Studying the distribution of happy numbers
- Investigating happy numbers in different bases
- Exploring variations of the happy number concept
4. Puzzle Creation
Happy numbers can be used to create engaging mathematical puzzles and brain teasers, making them a valuable tool in recreational mathematics.
Variations on Happy Numbers
The concept of happy numbers has inspired several variations and related concepts:
1. Happy Primes
A happy prime is a number that is both happy and prime. The first few happy primes are 7, 13, 19, 23, 31, 79, 97, 103, 109, 139, 167, 193, 239, 263, 293, …
2. Harshad Numbers
A Harshad number (or Niven number) is an integer that is divisible by the sum of its digits. While not directly related to happy numbers, they share the property of being defined by operations on their digits.
3. Narcissistic Numbers
A narcissistic number is a number that is the sum of its own digits each raised to the power of the number of digits. For example, 153 = 13 + 53 + 33.
Implementing a Happy Prime Detector
As an extension to our happy number detector, let’s implement a function to find happy primes. This will combine our happy number detection with a primality test:
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def is_happy_prime(n):
return is_happy(n) and is_prime(n)
# Find the first 10 happy primes
count = 0
num = 2
happy_primes = []
while count < 10:
if is_happy_prime(num):
happy_primes.append(num)
count += 1
num += 1
print(f"The first 10 happy primes are: {happy_primes}")
This code combines our previously defined is_happy
function with a simple primality test to identify happy primes.
The Educational Value of Happy Numbers
Happy numbers offer a wealth of educational opportunities, making them an excellent topic for coding education platforms like AlgoCademy:
1. Algorithmic Thinking
The process of detecting happy numbers encourages students to think algorithmically, breaking down a problem into clear, logical steps.
2. Code Optimization
As we saw with our optimized implementation, happy numbers provide a great context for discussing and applying code optimization techniques.
3. Mathematical Reasoning
Exploring the properties and variations of happy numbers helps develop mathematical reasoning skills, encouraging students to look for patterns and make logical deductions.
4. Problem-Solving Skills
Implementing functions to detect happy numbers, happy primes, and other variations hones problem-solving skills, a crucial ability for any programmer.
5. Interdisciplinary Connections
Happy numbers bridge the gap between mathematics and computer science, showcasing how abstract mathematical concepts can be explored and applied through programming.
Conclusion
Happy numbers, with their intriguing properties and the algorithmic challenges they present, offer a joyful journey through the intersection of mathematics and programming. From basic implementations to optimized algorithms, from theoretical properties to practical applications, happy numbers provide a rich landscape for exploration and learning.
For platforms like AlgoCademy, focusing on coding education and programming skills development, happy numbers serve as an excellent topic. They encapsulate many fundamental programming concepts, offer opportunities for optimization and extension, and provide a concrete example of how mathematical curiosities can be explored through code.
As we’ve seen, what starts as a simple numerical quirk can lead to deep dives into algorithmic efficiency, cycle detection, primality testing, and beyond. Happy numbers remind us that in the world of mathematics and programming, joy and learning often go hand in hand, with each new discovery opening doors to further exploration and understanding.
So the next time you encounter a number, why not take a moment to check if it’s happy? You might just find yourself embarking on a fascinating journey through the delightful world of mathematical curiosities and algorithmic exploration.