Handling Unexpected Roadblocks: How to Recover When Your Solution Doesn’t Work
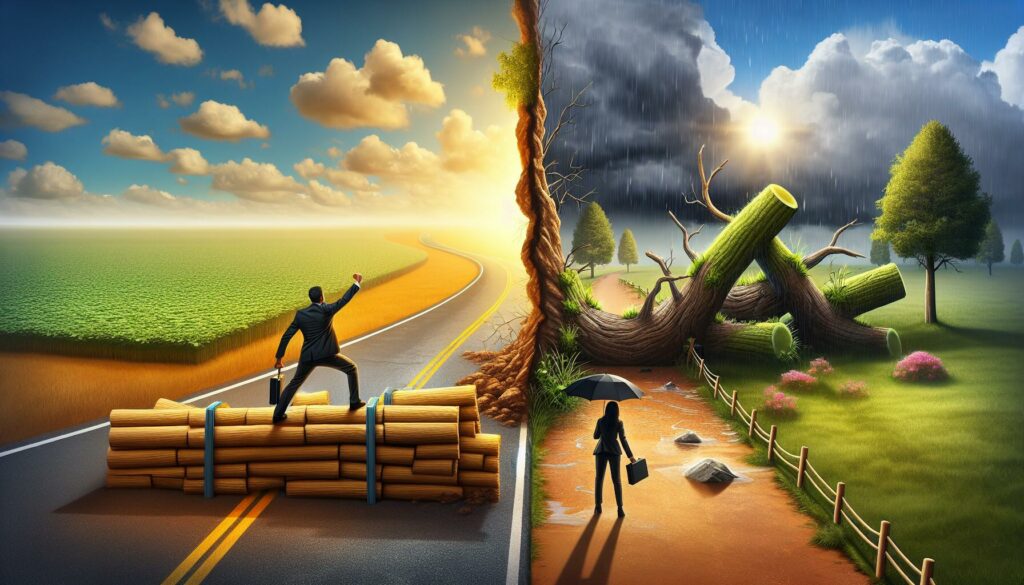
As aspiring programmers and seasoned developers alike can attest, the journey of coding is rarely a smooth, straight path. One of the most common and frustrating experiences in programming is when your carefully crafted solution doesn’t work as expected. These moments of unexpected roadblocks can be disheartening, but they’re also invaluable opportunities for growth and learning. In this comprehensive guide, we’ll explore strategies for handling these situations, recovering from setbacks, and ultimately becoming a more resilient and skilled programmer.
Understanding the Nature of Coding Challenges
Before we dive into specific strategies for handling unexpected roadblocks, it’s crucial to understand the nature of coding challenges. Programming is inherently a problem-solving discipline, and with problem-solving comes the inevitability of encountering obstacles.
Coding challenges can arise from various sources:
- Logical errors in your algorithm
- Syntax errors in your code
- Misunderstanding of the problem requirements
- Edge cases not considered in your initial solution
- Performance issues with your implementation
- Compatibility problems with different environments or systems
Recognizing that these challenges are a normal part of the coding process is the first step in developing resilience and the ability to recover when your solution doesn’t work.
The Importance of a Growth Mindset
Adopting a growth mindset is crucial when facing coding challenges. This mindset, popularized by psychologist Carol Dweck, is the belief that abilities and intelligence can be developed through dedication and hard work. In the context of programming, this means viewing each roadblock not as a failure, but as an opportunity to learn and improve your skills.
Here are some ways to cultivate a growth mindset in your coding journey:
- Embrace challenges as opportunities for growth
- View effort as a path to mastery
- Learn from criticism and feedback
- Find inspiration in the success of others
- Persist in the face of setbacks
By adopting this mindset, you’ll be better equipped to handle unexpected roadblocks and turn them into stepping stones for your development as a programmer.
Strategies for Diagnosing the Problem
When your solution doesn’t work as expected, the first step is to diagnose the problem. Here are some strategies to help you identify what’s going wrong:
1. Read the Error Messages
Error messages are your first line of defense. They often provide valuable information about what’s going wrong and where the issue is occurring. Don’t skip over them! Take the time to read and understand the error messages thoroughly.
2. Use Print Statements or Logging
One of the simplest yet most effective debugging techniques is to use print statements or logging to track the flow of your program and the values of variables at different points. This can help you identify where the program’s behavior deviates from your expectations.
For example, in Python:
def calculate_sum(numbers):
total = 0
for num in numbers:
print(f"Current number: {num}, Total before addition: {total}")
total += num
print(f"Total after addition: {total}")
return total
result = calculate_sum([1, 2, 3, 4, 5])
print(f"Final result: {result}")
3. Use a Debugger
Debuggers are powerful tools that allow you to step through your code line by line, inspect variable values, and set breakpoints. Learning to use a debugger effectively can significantly speed up your problem-solving process.
4. Rubber Duck Debugging
This technique involves explaining your code, line by line, to an inanimate object (traditionally a rubber duck). The act of verbalizing your code often helps you spot errors or inconsistencies in your logic.
5. Divide and Conquer
If you’re working on a complex problem, try to isolate the issue by breaking your code into smaller parts and testing each part individually. This can help you pinpoint exactly where the problem is occurring.
Strategies for Recovering and Moving Forward
Once you’ve identified the problem, it’s time to work on a solution. Here are some strategies to help you recover and move forward:
1. Take a Step Back
Sometimes, the best thing you can do when faced with a persistent problem is to take a break. Step away from your code for a while. This can help clear your mind and allow you to approach the problem with fresh eyes when you return.
2. Review the Problem Requirements
Go back to the original problem statement or requirements. Make sure you haven’t misunderstood or overlooked any crucial details. It’s not uncommon for programmers to realize they’ve been solving the wrong problem altogether!
3. Research and Learn
If you’re stuck on a particular concept or error, don’t hesitate to research and learn more about it. Utilize resources like documentation, Stack Overflow, or programming forums. Remember, every experienced programmer was once a beginner, and learning from others is a crucial part of the coding journey.
4. Implement a Different Approach
If your current approach isn’t working, consider implementing a different solution. There’s often more than one way to solve a problem in programming. Exploring alternative approaches can broaden your understanding and problem-solving skills.
5. Start from Scratch
Sometimes, starting over with a clean slate can be the most effective approach. This doesn’t mean you’ve wasted time – the process of writing the first solution, even if it didn’t work, has likely given you valuable insights into the problem.
6. Seek Help
Don’t be afraid to ask for help. Whether it’s a colleague, a mentor, or an online community, getting a fresh perspective can often lead to breakthroughs. Just be sure to explain what you’ve tried so far and what specific issue you’re facing.
Learning from the Experience
Once you’ve successfully overcome the roadblock, it’s important to take time to reflect on the experience and extract valuable lessons. Here are some ways to make the most of the learning opportunity:
1. Document Your Process
Keep a record of the problem you faced, the steps you took to diagnose and solve it, and the ultimate solution. This documentation can be invaluable when you face similar issues in the future.
2. Analyze Your Mistakes
Take an honest look at what went wrong. Did you make any assumptions that turned out to be incorrect? Did you overlook any important details? Understanding your mistakes can help prevent similar errors in the future.
3. Identify Knowledge Gaps
Did the problem reveal any gaps in your knowledge or skills? Use this insight to guide your future learning and development efforts.
4. Share Your Experience
Consider sharing your experience with others, whether through a blog post, a presentation to your team, or a contribution to an online community. Teaching others can reinforce your own learning and potentially help someone else facing a similar challenge.
Developing Resilience in the Face of Coding Challenges
Resilience is a key trait for successful programmers. It’s the ability to bounce back from setbacks, learn from failures, and persist in the face of challenges. Here are some tips for developing resilience in your coding journey:
1. Embrace the Learning Process
Remember that every bug you encounter, every error you make, is an opportunity to learn something new. Embrace these opportunities rather than dreading them.
2. Celebrate Small Wins
Don’t wait for the big breakthrough to feel a sense of accomplishment. Celebrate small wins along the way, like figuring out a tricky part of the problem or successfully implementing a new concept.
3. Develop a Problem-Solving Toolkit
As you encounter and overcome various challenges, add the strategies and techniques you’ve learned to your personal problem-solving toolkit. This could include debugging techniques, research methods, or specific coding patterns that have proven useful.
4. Practice Regularly
Like any skill, resilience in coding improves with practice. Regularly challenging yourself with coding problems, whether through platforms like AlgoCademy, coding competitions, or personal projects, can help build your problem-solving muscles.
5. Maintain a Positive Attitude
While it’s natural to feel frustrated when facing persistent problems, try to maintain a positive attitude. Remember that every experienced programmer has been where you are now, and that overcoming these challenges is part of your growth as a developer.
Leveraging Tools and Resources
In today’s digital age, programmers have access to a wealth of tools and resources that can aid in problem-solving and recovery when solutions don’t work. Here are some key resources to consider:
1. Version Control Systems
Tools like Git allow you to track changes in your code over time. This can be invaluable when you need to revert to a previous working version or understand when and where a bug was introduced.
2. Integrated Development Environments (IDEs)
Modern IDEs offer features like intelligent code completion, real-time error detection, and integrated debugging tools. Familiarizing yourself with these features can significantly speed up your problem-solving process.
3. Online Coding Platforms
Platforms like AlgoCademy provide structured learning paths, interactive coding environments, and AI-powered assistance. These can be excellent resources for practicing problem-solving skills and getting immediate feedback on your solutions.
4. Documentation and API References
Official documentation for programming languages, libraries, and frameworks is often the most reliable source of information. Make a habit of referring to these resources when you’re unsure about how to use a particular feature or function.
5. Code Analysis Tools
Static code analysis tools can help identify potential issues in your code before you even run it. These tools can catch common mistakes, style issues, and even potential security vulnerabilities.
The Role of Collaboration in Problem-Solving
While individual problem-solving skills are crucial, collaboration can often lead to faster and more effective solutions. Here’s how collaboration can help when your solution doesn’t work:
1. Code Reviews
Having others review your code can help catch errors you might have missed and provide new perspectives on the problem. Even if you’re working on a personal project, consider finding a coding buddy or participating in online code review communities.
2. Pair Programming
Pair programming, where two developers work together on the same code, can be an effective way to tackle complex problems. One person can focus on writing the code while the other reviews it in real-time, catching potential issues early.
3. Leveraging Team Knowledge
In a team environment, don’t hesitate to tap into the collective knowledge and experience of your colleagues. Someone on your team may have faced a similar issue before and can provide valuable insights.
4. Online Communities
Platforms like Stack Overflow, Reddit’s programming communities, and language-specific forums can be excellent resources for getting help with specific problems. Just be sure to do your research first and provide a clear, concise description of your issue when asking for help.
Conclusion: Embracing the Journey of Continuous Improvement
Handling unexpected roadblocks and recovering when your solution doesn’t work are essential skills for any programmer. By adopting a growth mindset, developing effective problem-solving strategies, learning from your experiences, and leveraging available tools and resources, you can turn these challenges into opportunities for growth and improvement.
Remember, every experienced programmer has faced similar challenges on their journey. What sets successful developers apart is not the absence of problems, but their ability to persist, learn, and grow from these experiences. Embrace the process, celebrate your progress, and keep pushing forward. With each challenge you overcome, you’re not just solving a problem – you’re becoming a better, more resilient programmer.
As you continue your coding journey, platforms like AlgoCademy can provide structured guidance, interactive practice, and AI-powered assistance to help you develop your problem-solving skills and prepare for real-world coding challenges. Remember, the path to becoming a skilled programmer is a marathon, not a sprint. Embrace the journey, and keep coding!