Handling Pressure: How to Stay Focused When the Interviewer Is Watching You Code
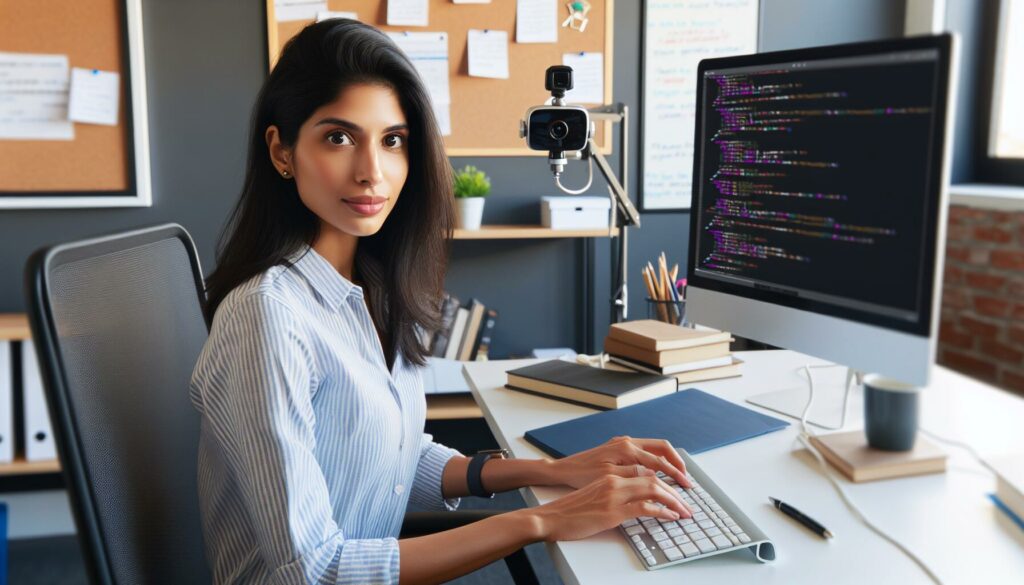
Technical interviews can be nerve-wracking experiences, especially when you’re asked to code on the spot while an interviewer observes your every move. The pressure of performing under scrutiny can cause even the most skilled developers to falter. However, with the right strategies and mindset, you can maintain your composure and showcase your true abilities. In this comprehensive guide, we’ll explore effective techniques to help you stay focused and confident when coding under pressure during interviews.
Understanding the Challenge
Before diving into strategies, it’s essential to understand why coding interviews can be so challenging:
- Time constraints: You’re often given complex problems to solve in a limited time frame.
- Performance anxiety: The presence of an observer can heighten nervousness and self-doubt.
- Unfamiliar environment: You may be using an unfamiliar IDE or coding platform.
- High stakes: The outcome of the interview can significantly impact your career prospects.
Recognizing these factors is the first step in developing coping mechanisms to overcome them.
Strategies for Maintaining Composure
1. Prepare Thoroughly
The best defense against interview anxiety is thorough preparation. This includes:
- Practicing coding problems regularly
- Familiarizing yourself with common algorithms and data structures
- Simulating interview conditions during your practice sessions
- Reviewing fundamental concepts in your preferred programming language
By building a strong foundation, you’ll feel more confident in your abilities when faced with a challenging problem during the interview.
2. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can help you stay on track even when you’re feeling pressured. Consider adopting the following framework:
- Clarify the problem: Ask questions to ensure you fully understand the requirements.
- Break down the problem: Identify smaller, manageable sub-problems.
- Plan your approach: Outline your solution strategy before coding.
- Implement incrementally: Start with a basic solution and build upon it.
- Test and refine: Continuously check your code and optimize as needed.
By following a systematic approach, you’ll be less likely to get overwhelmed or lose focus during the interview.
3. Practice Mindfulness and Breathing Techniques
Mindfulness can help you stay present and focused, even in high-pressure situations. Try these techniques:
- Deep breathing: Take slow, deep breaths to calm your nerves.
- Grounding exercises: Focus on your physical sensations to anchor yourself in the present moment.
- Positive self-talk: Use encouraging internal dialogue to boost your confidence.
Incorporating these practices into your daily routine can make them more natural to employ during stressful interviews.
4. Communicate Effectively
Clear communication is crucial during coding interviews. It helps you:
- Demonstrate your thought process
- Seek clarification when needed
- Build rapport with the interviewer
Practice verbalizing your thoughts while coding, and don’t hesitate to ask questions or think aloud during the interview.
5. Embrace the “Think-Aloud” Protocol
The “think-aloud” protocol involves vocally expressing your thoughts and reasoning as you work through a problem. This technique serves multiple purposes:
- It helps the interviewer understand your problem-solving approach.
- It can slow down your thought process, reducing the likelihood of overlooking important details.
- It may prompt the interviewer to provide hints or guidance if you’re heading in the wrong direction.
To effectively use this technique:
- Explain your understanding of the problem.
- Describe potential approaches and their trade-offs.
- Verbalize your decision-making process as you code.
- Discuss any assumptions or constraints you’re considering.
Remember, it’s okay to take brief pauses to collect your thoughts. The goal is to provide insight into your problem-solving skills, not to maintain a constant stream of speech.
6. Manage Your Time Effectively
Time management is critical during coding interviews. Here are some strategies to help you make the most of your allotted time:
- Ask about time constraints upfront.
- Allocate time for each phase of your problem-solving framework.
- Use a simple solution first, then optimize if time allows.
- Set mental checkpoints to assess your progress.
If you’re running out of time, communicate your awareness to the interviewer and discuss how you would proceed if given more time.
7. Handle Moments of Uncertainty
It’s natural to encounter moments of uncertainty during a coding interview. Here’s how to navigate them without losing focus or confidence:
- Acknowledge the challenge: It’s okay to admit when you’re unsure about something.
- Break the problem down further: Try to identify smaller, more manageable parts of the problem you can solve.
- Use pseudo-code: If you’re stuck on implementation details, write out the logic in plain language or pseudo-code.
- Draw diagrams: Visual representations can help clarify your thoughts and potentially reveal solutions.
- Revisit your assumptions: Sometimes, uncertainty stems from misunderstanding the problem. Double-check the requirements.
Remember, interviewers are often more interested in your problem-solving approach than in seeing a perfect solution.
8. Practice Resilience and Adaptability
Interviews rarely go perfectly, and that’s okay. Cultivate resilience by:
- Viewing challenges as opportunities for growth
- Learning from mistakes rather than dwelling on them
- Adapting quickly when your initial approach doesn’t work
These qualities not only help during the interview but are also valuable traits that employers seek in potential hires.
Practical Examples: Navigating Uncertain Moments
Let’s explore some common scenarios you might encounter during a coding interview and strategies to handle them:
Scenario 1: You Can’t Remember a Specific Method or Syntax
Example: You’re implementing a sorting algorithm but can’t recall the exact syntax for swapping elements in an array.
How to Handle It:
- Acknowledge the gap: “I’m having trouble remembering the exact syntax for swapping elements, but I know the logic.”
- Explain the logic: “What I want to do is temporarily store one element, replace it with another, and then assign the stored value to the second position.”
- Use pseudo-code or a close approximation:
// Pseudo-code for swapping elements temp = array[i] array[i] = array[j] array[j] = temp
- Ask if it’s okay to use this approach: “Is it alright if I proceed with this logic, and we can correct the syntax later if needed?”
Scenario 2: You’re Unsure About the Time Complexity of Your Solution
Example: You’ve implemented a solution using nested loops, but you’re not confident about its efficiency.
How to Handle It:
- Express your concern: “I’ve implemented a solution, but I’m not entirely sure about its time complexity. Let me think through it.”
- Break down your analysis:
- “The outer loop runs n times.”
- “For each iteration of the outer loop, the inner loop also runs up to n times.”
- “This suggests it might be O(n^2), but I’d like to consider if there’s any way to optimize it.”
- Discuss potential optimizations: “We might be able to improve this by using a different data structure, like a hash map, to reduce the nested iterations.”
- Ask for feedback: “What are your thoughts on this analysis? Are there any aspects I’m overlooking?”
Scenario 3: You Realize Your Initial Approach Is Flawed
Example: Midway through implementing a solution, you realize your approach won’t handle all edge cases.
How to Handle It:
- Stop and communicate: “I’ve just realized that my current approach has a flaw. It won’t handle the case where…”
- Explain the issue: Clearly articulate why your current solution is problematic.
- Propose alternatives: “I think we could address this by modifying our approach to…”
- Ask for guidance: “Before I make significant changes, do you think this new direction makes sense, or do you have any suggestions?”
Leveraging Tools and Resources
While your core coding skills are crucial, it’s also important to be familiar with the tools and resources that can support you during a coding interview:
1. Online IDEs and Coding Platforms
Many companies use online coding platforms for remote interviews. Familiarize yourself with common platforms like:
- HackerRank
- LeetCode
- CoderPad
Practice using these platforms to get comfortable with their interfaces and features.
2. Debugging Tools
Know how to effectively use debugging tools within your chosen programming environment. This might include:
- Setting breakpoints
- Stepping through code
- Inspecting variables
Being proficient with these tools can help you quickly identify and fix issues during the interview.
3. Documentation and Language References
While you shouldn’t rely heavily on external resources during an interview, it’s often acceptable to quickly reference documentation for specific syntax or method names. Ask your interviewer if it’s okay to do so, and use this option sparingly.
Post-Interview Reflection and Growth
Regardless of the outcome, each interview is a valuable learning experience. After the interview:
- Reflect on what went well and areas for improvement.
- Review any problems you struggled with and research optimal solutions.
- Consider how you handled pressure and identify strategies that worked best for you.
- Seek feedback if possible, either from the interviewer or through your recruiter.
Use these insights to refine your preparation strategy for future interviews.
Conclusion
Handling pressure during coding interviews is a skill that improves with practice and preparation. By developing a structured problem-solving approach, honing your communication skills, and learning to manage stress effectively, you can significantly enhance your performance under observation.
Remember that interviewers are not just evaluating your coding skills, but also your ability to think critically, communicate clearly, and perform under pressure. These are all valuable traits in real-world development scenarios.
Stay calm, trust in your preparation, and view each interview as an opportunity to showcase your skills and learn something new. With time and experience, you’ll find that the pressure of coding while being observed becomes more manageable, allowing your true abilities to shine through.
Keep practicing, stay curious, and approach each challenge with a growth mindset. Your journey in mastering the art of coding interviews is an integral part of your overall development as a programmer. Embrace the process, and you’ll not only excel in interviews but also become a more confident and capable developer in your professional career.