Handling Multiple Approaches: How to Choose the Best Solution During a Coding Interview
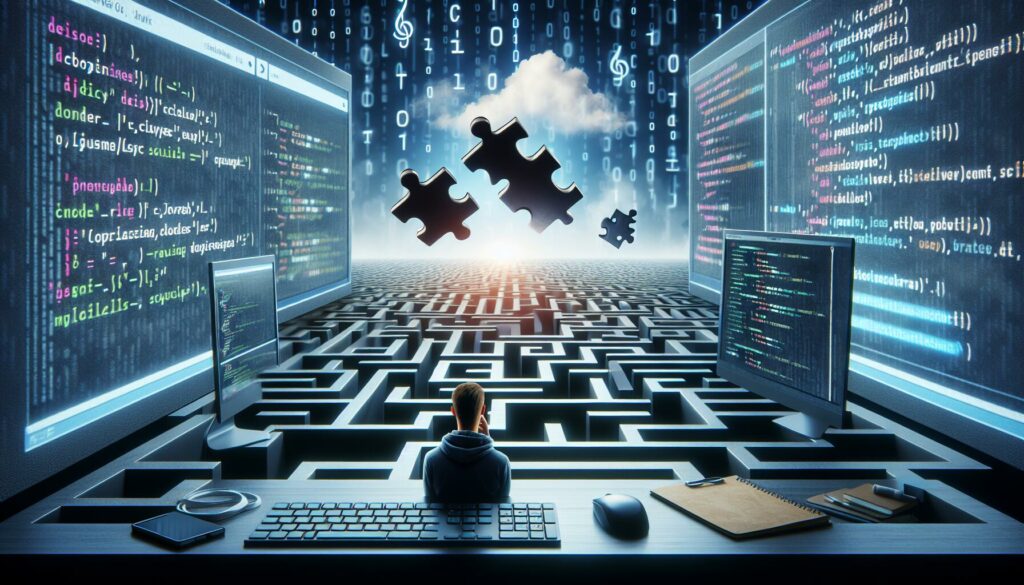
When facing a coding challenge during an interview, you’ll often find yourself considering multiple approaches to solve the problem at hand. The ability to evaluate different solutions and choose the most appropriate one is a crucial skill that interviewers look for in candidates. In this comprehensive guide, we’ll explore how to handle multiple approaches, weigh their pros and cons, and make informed decisions about which solution to implement during a coding interview.
Understanding the Importance of Approach Selection
Before diving into the specifics of evaluating different approaches, it’s essential to understand why this skill is so valuable in the eyes of interviewers:
- Problem-solving ability: Your approach to solving a problem reveals your thought process and problem-solving skills.
- Analytical thinking: Evaluating multiple solutions demonstrates your ability to think critically and analyze trade-offs.
- Adaptability: Showing that you can consider various options indicates flexibility and openness to different perspectives.
- Real-world relevance: In actual software development, choosing the right approach is crucial for creating efficient and maintainable code.
Common Types of Approaches in Coding Interviews
Before we delve into how to choose between different approaches, let’s review some common types of solutions you might encounter or consider during a coding interview:
1. Brute Force Approach
The brute force approach involves solving the problem in the most straightforward way possible, often by exhaustively checking all possible solutions.
- Pros: Simple to implement and understand; guaranteed to find the correct solution (if one exists).
- Cons: Often inefficient, especially for large inputs; may not meet time or space complexity requirements.
2. Optimized Approach
An optimized approach improves upon the brute force method by reducing time complexity, space complexity, or both.
- Pros: More efficient than brute force; demonstrates advanced problem-solving skills.
- Cons: May be more complex to implement; requires deeper understanding of algorithms and data structures.
3. Dynamic Programming
Dynamic programming involves breaking down a problem into smaller subproblems and storing their solutions to avoid redundant computations.
- Pros: Efficient for problems with overlapping subproblems; can significantly reduce time complexity.
- Cons: May be overkill for simpler problems; can be challenging to implement correctly under time pressure.
4. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, aiming to find a global optimum.
- Pros: Often simple and intuitive; can be very efficient for certain problem types.
- Cons: May not always lead to the globally optimal solution; requires proof of correctness.
5. Divide and Conquer
This approach involves breaking the problem into smaller subproblems, solving them independently, and then combining the results.
- Pros: Can be very efficient for certain problem types; often leads to elegant recursive solutions.
- Cons: May introduce overhead for simple problems; can be complex to implement correctly.
Evaluating Different Approaches
Now that we’ve covered some common types of approaches, let’s explore how to evaluate them effectively during a coding interview:
1. Analyze Time and Space Complexity
One of the most critical factors in choosing an approach is its efficiency in terms of time and space complexity.
- Calculate the Big O notation for each approach.
- Consider the expected input size and how it affects performance.
- Evaluate whether the approach meets the given constraints or requirements.
For example, if you’re dealing with a large dataset, a solution with O(n^2) time complexity might be impractical, even if it’s simpler to implement than an O(n log n) solution.
2. Consider Implementation Complexity
While efficiency is crucial, you also need to factor in how complex the implementation will be, especially given the time constraints of an interview.
- Assess how long it will take you to code each approach.
- Consider the likelihood of introducing bugs or edge case errors.
- Evaluate your comfort level with the required algorithms or data structures.
Sometimes, a slightly less efficient but easier-to-implement solution might be preferable if you’re confident you can code it correctly within the given time.
3. Evaluate Scalability and Maintainability
Think beyond the immediate problem and consider how well the solution would scale or be maintained in a real-world scenario.
- Consider how the approach would handle larger inputs or additional requirements.
- Assess the readability and maintainability of the resulting code.
- Think about potential future optimizations or extensions.
Demonstrating this kind of forward-thinking can impress interviewers and show that you consider the bigger picture.
4. Assess Trade-offs
Often, there’s no perfect solution, and you’ll need to make trade-offs. Be prepared to explain your reasoning.
- Compare time complexity vs. space complexity trade-offs.
- Weigh implementation simplicity against runtime efficiency.
- Consider the balance between code readability and performance optimizations.
Being able to articulate these trade-offs shows depth of understanding and critical thinking skills.
5. Consider the Problem Context
The nature of the problem and any additional context provided can influence which approach is most suitable.
- Look for clues in the problem statement that might suggest a particular approach.
- Consider any constraints or requirements mentioned by the interviewer.
- Think about how the solution might fit into a larger system or use case.
For instance, if the problem involves real-time processing of streaming data, an approach with constant space complexity might be preferred over one that’s slightly faster but requires more memory.
Making the Decision: Choosing the Best Approach
After evaluating the different approaches, you’ll need to make a decision about which one to implement. Here’s a step-by-step process to help you choose:
1. Eliminate Clearly Inferior Options
Start by ruling out any approaches that are clearly unsuitable. These might include:
- Solutions that don’t meet the given time or space complexity requirements.
- Approaches that are too complex to implement within the interview time frame.
- Methods that don’t address all aspects of the problem or have significant drawbacks.
2. Rank the Remaining Approaches
For the approaches that remain viable, create a mental ranking based on the following criteria:
- Efficiency (time and space complexity)
- Ease of implementation
- Clarity and maintainability of the resulting code
- Scalability and potential for future improvements
3. Discuss Your Thoughts with the Interviewer
Before committing to an approach, it’s often beneficial to discuss your thoughts with the interviewer. This accomplishes several things:
- It demonstrates your ability to communicate technical ideas clearly.
- It allows you to get feedback or additional insights from the interviewer.
- It shows that you’re thoughtful and considerate in your decision-making process.
You might say something like: “I’m considering two main approaches here. The first is a brute force solution with O(n^2) time complexity, which would be straightforward to implement. The second is an optimized approach using a hash map, which would bring the time complexity down to O(n) but might be slightly more complex to code. Given the large input size mentioned in the problem, I’m leaning towards the optimized approach. What are your thoughts on this?”
4. Make a Decision and Commit
Based on your evaluation and any feedback from the interviewer, make a decision on which approach to implement. Once you’ve decided, commit to it and start coding. Remember, it’s better to have a working solution using a less optimal approach than to get stuck trying to implement the perfect solution.
5. Be Prepared to Pivot if Necessary
As you start implementing your chosen approach, remain open to the possibility that you might need to change course. If you encounter unexpected difficulties or realize a flaw in your initial approach, be prepared to reassess and potentially switch to a different method.
Balancing Speed of Implementation and Efficiency
One of the most challenging aspects of coding interviews is balancing the speed of implementation with the efficiency of the solution. Here are some strategies to help you navigate this trade-off:
1. Start with a Simple Solution
If time allows, consider starting with a simpler, perhaps less efficient solution. This approach has several benefits:
- It allows you to have a working solution quickly, which you can then optimize if time permits.
- It demonstrates your ability to iterate and improve on your code.
- It provides a fallback option if you struggle with implementing a more optimized approach.
You might say to the interviewer: “I’m going to start with a straightforward implementation to ensure we have a working solution. Then, if we have time, I’ll optimize it to improve the time complexity.”
2. Implement in Stages
For more complex problems, consider implementing your solution in stages:
- Start with the core logic or algorithm.
- Add necessary data structures or helper functions.
- Implement edge case handling and error checking.
- Optimize and refactor if time allows.
This approach allows you to have a partially working solution at various stages of the interview, which is better than having nothing if you run out of time.
3. Communicate Your Intention to Optimize
If you decide to start with a less efficient solution, clearly communicate your intention to optimize it later. This shows the interviewer that you’re aware of the trade-offs and have a plan for improvement.
For example: “I’m going to implement a brute force solution first to ensure correctness. Once that’s working, I’ll optimize it using a sliding window technique to reduce the time complexity from O(n^2) to O(n).”
4. Use Pseudocode for Complex Parts
For particularly complex or time-consuming parts of your solution, consider using pseudocode to outline your approach before diving into the actual implementation. This can help you:
- Organize your thoughts and plan your implementation.
- Quickly convey your intended approach to the interviewer.
- Identify potential issues or optimizations before committing to code.
5. Know When to Optimize
Be strategic about when to focus on optimization. Consider the following guidelines:
- If your initial solution meets all requirements, including time and space complexity, you may not need to optimize further.
- If your solution works but doesn’t meet the stated complexity requirements, prioritize optimization.
- If you’re running out of time, focus on having a complete, working solution rather than partial optimizations.
Example: Evaluating Approaches in Practice
Let’s walk through an example of how you might evaluate different approaches during an interview. Consider the following problem:
Given an array of integers, find the two numbers that add up to a specific target number. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Here’s how you might approach this problem:
Approach 1: Brute Force
function twoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return [];
}
- Time Complexity: O(n^2)
- Space Complexity: O(1)
- Pros: Simple to implement and understand.
- Cons: Inefficient for large inputs.
Approach 2: Hash Map
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
- Time Complexity: O(n)
- Space Complexity: O(n)
- Pros: Efficient, single pass through the array.
- Cons: Uses additional space for the hash map.
Evaluation and Decision
In this case, you might reason as follows:
- The brute force approach is simpler but has a time complexity of O(n^2), which could be problematic for large inputs.
- The hash map approach improves time complexity to O(n) at the cost of O(n) space complexity.
- Given that the problem doesn’t specify any space constraints, the hash map approach seems preferable due to its better time complexity.
- The hash map solution is still relatively simple to implement and explain.
Based on this evaluation, you might choose to implement the hash map approach. However, you could also mention the brute force method to the interviewer, explaining why you prefer the optimized solution:
“I’m considering two approaches here. The first is a brute force method that would check all pairs of numbers, which is simple but has O(n^2) time complexity. The second approach uses a hash map to achieve O(n) time complexity with O(n) space. Given that we’re not constrained on space and efficiency is important, I’m going to implement the hash map solution. Does that sound reasonable to you?”
Conclusion
Handling multiple approaches and choosing the best solution during a coding interview is a skill that comes with practice and experience. By systematically evaluating different approaches based on their time and space complexity, implementation difficulty, and other relevant factors, you can make informed decisions that demonstrate your problem-solving abilities to interviewers.
Remember these key points:
- Always consider multiple approaches before settling on one.
- Analyze the trade-offs between different solutions.
- Communicate your thought process clearly to the interviewer.
- Be prepared to implement a simpler solution first if time is a concern.
- Stay flexible and be ready to adapt your approach if needed.
By mastering the art of approach selection, you’ll not only perform better in coding interviews but also develop valuable skills that will serve you well throughout your software development career. Keep practicing, stay curious about different problem-solving techniques, and always strive to understand the why behind your choices. With time and dedication, you’ll become proficient at navigating the complex landscape of algorithmic problem-solving.