Handling Multi-Part Questions in Interviews: A Comprehensive Guide
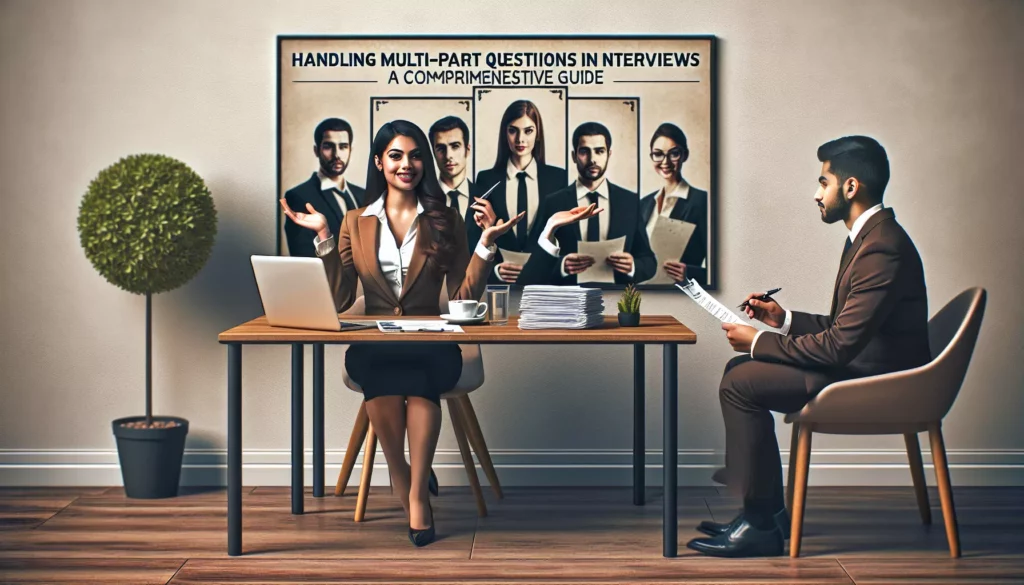
In the world of technical interviews, especially those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), candidates often face complex, multi-part questions. These questions are designed to test not only your coding skills but also your problem-solving abilities, attention to detail, and communication skills. In this comprehensive guide, we’ll explore strategies for effectively handling multi-part questions in interviews, helping you navigate these challenging scenarios with confidence.
Understanding Multi-Part Questions
Multi-part questions are interview problems that consist of several interconnected components or stages. They’re often used to assess a candidate’s ability to break down complex problems, manage multiple requirements, and demonstrate a range of skills within a single problem-solving exercise.
Here’s an example of a multi-part question you might encounter:
Design a system to manage a parking lot:
1. Implement a class to represent a parking spot.
2. Create a class for the parking lot that can add and remove cars.
3. Implement a method to find the nearest available parking spot.
4. Add a feature to track how long each car has been parked.
5. Implement a billing system based on parking duration.
As you can see, this question covers multiple aspects of system design, object-oriented programming, data structures, and even a bit of algorithm design. Let’s dive into strategies for tackling such questions effectively.
1. Break Down the Question
The first step in handling a multi-part question is to break it down into its constituent parts. This process, often called “problem decomposition,” is crucial for managing complexity and ensuring you address all aspects of the question.
Strategy:
- Read the entire question carefully
- Identify each distinct part or requirement
- List out these parts, possibly numbering them
- Understand how these parts relate to each other
For our parking lot example, you might break it down like this:
- Parking Spot class
- Parking Lot class with add/remove functionality
- Nearest spot finding algorithm
- Parking duration tracking
- Billing system
2. Clarify Requirements
Before diving into the solution, it’s crucial to clarify any ambiguities in the question. This demonstrates your attention to detail and ensures you’re solving the right problem.
Questions to ask:
- What specific attributes should the parking spot class have?
- Should the parking lot have a fixed size or be expandable?
- What criteria define the “nearest” spot? (e.g., physical distance, ease of access)
- How precise should the parking duration tracking be? (e.g., minutes, hours)
- What’s the pricing model for the billing system? (e.g., hourly rate, flat fee)
Remember, interviewers appreciate candidates who ask thoughtful questions. It shows you’re thinking critically about the problem.
3. Plan Your Approach
With a clear understanding of the problem, it’s time to plan your approach. This step is crucial for managing time effectively and ensuring you cover all parts of the question.
Steps to consider:
- Decide on the order in which you’ll tackle each part
- Estimate roughly how much time you’ll spend on each part
- Consider dependencies between parts
- Think about which data structures and algorithms you might use
For our parking lot example, a possible approach could be:
- Start with the Parking Spot class (5 minutes)
- Move on to the Parking Lot class (10 minutes)
- Implement the nearest spot algorithm (15 minutes)
- Add parking duration tracking (10 minutes)
- Finally, implement the billing system (10 minutes)
4. Implement Incrementally
When dealing with multi-part questions, it’s often best to implement your solution incrementally. This approach allows you to make progress steadily and demonstrate your thought process throughout the interview.
Benefits of incremental implementation:
- Allows you to show progress quickly
- Easier to debug and test as you go
- Provides opportunities for the interviewer to give feedback
- Helps manage time more effectively
Let’s look at how we might start implementing our parking lot system incrementally:
class ParkingSpot:
def __init__(self, spot_number, vehicle=None):
self.spot_number = spot_number
self.vehicle = vehicle
self.is_occupied = False
def park_vehicle(self, vehicle):
if not self.is_occupied:
self.vehicle = vehicle
self.is_occupied = True
return True
return False
def remove_vehicle(self):
if self.is_occupied:
self.vehicle = None
self.is_occupied = False
return True
return False
class ParkingLot:
def __init__(self, total_spots):
self.total_spots = total_spots
self.spots = [ParkingSpot(i) for i in range(total_spots)]
def park_car(self, vehicle):
for spot in self.spots:
if spot.park_vehicle(vehicle):
return spot.spot_number
return None
def remove_car(self, spot_number):
if 0 <= spot_number < self.total_spots:
return self.spots[spot_number].remove_vehicle()
return False
This implementation covers the first two parts of our multi-part question. We can now move on to implementing the nearest spot algorithm, duration tracking, and billing system.
5. Communicate Throughout
Clear communication is crucial when handling multi-part questions. As you work through each part, explain your thought process, decisions, and any assumptions you’re making.
Tips for effective communication:
- Think out loud as you work through the problem
- Explain the rationale behind your design choices
- Discuss trade-offs between different approaches
- Ask for feedback after completing each part
For example, when implementing the nearest spot algorithm, you might say something like:
“For finding the nearest available spot, I’m considering two approaches. We could use a simple linear search, which would be O(n) time complexity but straightforward to implement. Alternatively, we could maintain a min-heap of available spots based on their distance from the entrance, which would give us O(log n) time for finding the nearest spot but require more complex code and additional space. Given the scale of a typical parking lot, I think the simpler linear search would be sufficient, but I’m open to discussing if you think we need the more optimized approach.”
6. Manage Your Time
Time management is crucial when dealing with multi-part questions. You need to ensure you have enough time to address all parts of the question, even if some parts are implemented at a higher level.
Time management strategies:
- Keep an eye on the clock
- Stick to your planned time allocations as much as possible
- If you’re running behind, consider simplifying your approach for remaining parts
- Don’t get bogged down in optimizing one part at the expense of others
If you find yourself running out of time, it’s better to provide a high-level description of how you’d implement the remaining parts rather than leaving them completely unaddressed.
7. Test Your Solution
As you complete each part of the question, take time to test your implementation. This demonstrates your attention to detail and commitment to producing reliable code.
Testing strategies:
- Write unit tests for individual components
- Test edge cases and boundary conditions
- Consider how different parts of the system interact
- Discuss potential scalability issues
Here’s an example of how you might test the parking lot implementation:
def test_parking_lot():
lot = ParkingLot(5)
# Test parking
assert lot.park_car("Car1") == 0
assert lot.park_car("Car2") == 1
assert lot.park_car("Car3") == 2
# Test removing
assert lot.remove_car(1) == True
assert lot.remove_car(1) == False # Already removed
# Test parking in a previously occupied spot
assert lot.park_car("Car4") == 1
# Test full lot
lot.park_car("Car5")
assert lot.park_car("Car6") == None # Lot is full
print("All tests passed!")
8. Optimize and Refine
If you have time after implementing all parts of the question, look for opportunities to optimize or refine your solution. This could involve improving the efficiency of your algorithms, enhancing the design for better scalability, or adding error handling and edge case management.
Areas to consider for optimization:
- Time complexity of critical operations
- Space efficiency
- Code readability and maintainability
- Scalability for larger inputs or concurrent users
For our parking lot system, we might optimize the nearest spot finding algorithm:
import heapq
class ParkingLot:
def __init__(self, total_spots):
self.total_spots = total_spots
self.spots = [ParkingSpot(i) for i in range(total_spots)]
self.available_spots = [(spot.distance_from_entrance, i) for i, spot in enumerate(self.spots)]
heapq.heapify(self.available_spots)
def find_nearest_spot(self):
if self.available_spots:
_, spot_number = heapq.heappop(self.available_spots)
return spot_number
return None
def park_car(self, vehicle):
spot_number = self.find_nearest_spot()
if spot_number is not None:
self.spots[spot_number].park_vehicle(vehicle)
return spot_number
return None
def remove_car(self, spot_number):
if 0 <= spot_number < self.total_spots and self.spots[spot_number].remove_vehicle():
heapq.heappush(self.available_spots, (self.spots[spot_number].distance_from_entrance, spot_number))
return True
return False
This optimization uses a min-heap to efficiently find the nearest available spot, improving the time complexity from O(n) to O(log n) for this operation.
9. Reflect on Trade-offs
As you near the end of your solution, take a moment to reflect on the trade-offs in your design and implementation. This demonstrates your ability to think critically about software design and shows maturity in your approach to problem-solving.
Points to consider:
- Time complexity vs. space complexity trade-offs
- Simplicity vs. efficiency
- Flexibility for future changes vs. current optimizations
- Performance vs. maintainability
For our parking lot system, you might discuss:
“By using a min-heap for available spots, we’ve improved the time complexity of finding the nearest spot from O(n) to O(log n). However, this comes at the cost of additional space complexity and slightly more complex code. For a small parking lot, a simple array might have been sufficient and more straightforward. The heap approach would show its benefits more clearly for larger parking lots or in scenarios where finding the nearest spot is a frequent operation.”
10. Summarize Your Solution
At the end of the interview, provide a concise summary of your solution. This helps reinforce your understanding of the problem and gives the interviewer a clear picture of what you’ve accomplished.
Elements to include in your summary:
- Brief overview of your approach
- Key design decisions and their rationale
- Main algorithms and data structures used
- Any significant optimizations or trade-offs
- Potential areas for future improvement
For our parking lot system, a summary might look like this:
“To solve this multi-part question, I implemented a ParkingSpot class to represent individual spots and a ParkingLot class to manage the overall system. The ParkingLot uses a min-heap to efficiently find the nearest available spot, offering O(log n) time complexity for this operation. I implemented car parking and removal functionality, along with a simple duration tracking system using timestamps. The billing system calculates fees based on the duration of stay. Key trade-offs included using more memory with the heap structure to gain better time efficiency in finding spots. Areas for future improvement could include implementing a more sophisticated pricing model or adding support for different types of vehicles.”
Conclusion
Handling multi-part questions in technical interviews can be challenging, but with the right approach, they offer an excellent opportunity to showcase your skills. By breaking down the problem, clarifying requirements, planning your approach, implementing incrementally, communicating clearly, managing your time, testing thoroughly, optimizing where possible, reflecting on trade-offs, and summarizing your solution, you can navigate these complex questions with confidence.
Remember, the goal isn’t just to solve the problem, but to demonstrate your problem-solving process, coding skills, and ability to handle complex scenarios. With practice and the strategies outlined in this guide, you’ll be well-equipped to tackle multi-part questions in your next technical interview.
Keep coding, keep learning, and best of luck in your interviews!