Handling Edge Cases: Why It’s Critical to Think Beyond the Obvious in Coding Interviews
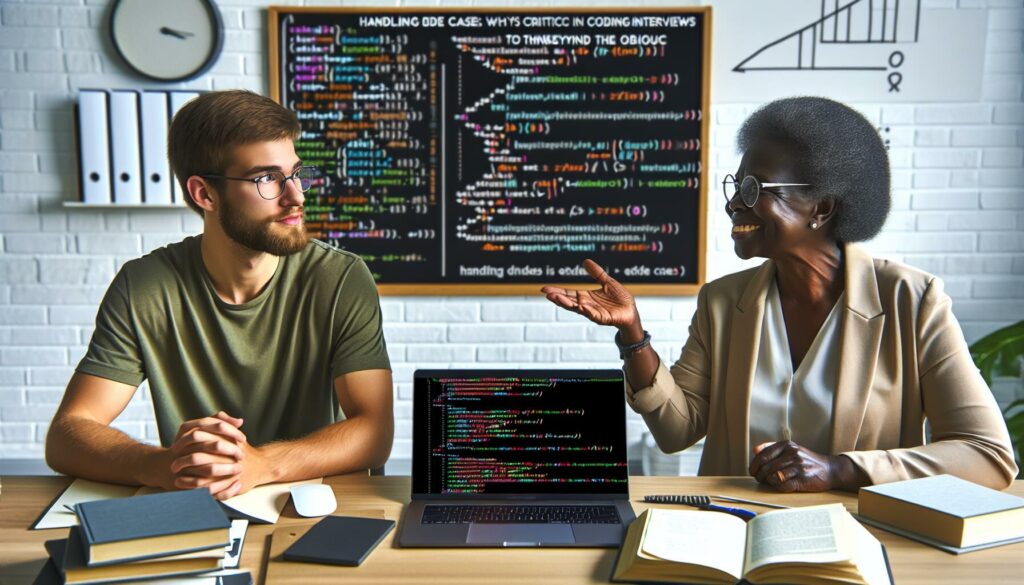
When it comes to coding interviews, particularly those at prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), one skill that often separates successful candidates from the rest is the ability to handle edge cases. Edge cases are those scenarios that occur at the extremes of possible inputs or conditions for a given problem. They’re the situations that might be rare or unexpected but can cause significant issues if not properly addressed.
In this comprehensive guide, we’ll dive deep into the world of edge cases, exploring why they’re crucial in coding interviews, how to identify them, and strategies for handling them effectively. By the end of this article, you’ll have a solid understanding of edge cases and be better prepared to tackle them in your next coding interview.
Table of Contents
- What Are Edge Cases?
- The Importance of Handling Edge Cases in Coding Interviews
- How to Identify Edge Cases
- Common Edge Cases and How to Handle Them
- Strategies for Effectively Handling Edge Cases
- Real-World Examples of Edge Case Handling
- Best Practices for Edge Case Management
- Conclusion
1. What Are Edge Cases?
Edge cases are inputs or scenarios that test the boundaries of your code. They’re often unexpected or unusual situations that can cause your program to behave incorrectly if not properly handled. Some common examples of edge cases include:
- Empty inputs
- Extremely large or small inputs
- Inputs at the minimum or maximum allowed values
- Inputs with unexpected formats or types
- Boundary conditions where behavior changes
Understanding and accounting for edge cases is crucial for writing robust, reliable code that can handle a wide range of real-world scenarios.
2. The Importance of Handling Edge Cases in Coding Interviews
In coding interviews, particularly at top tech companies, the ability to handle edge cases is highly valued for several reasons:
Demonstrates Thorough Problem-Solving Skills
When you consider and handle edge cases, you show that you can think beyond the obvious and anticipate potential issues. This demonstrates a level of thoroughness and attention to detail that is crucial in professional software development.
Reflects Real-World Coding Practices
In production environments, edge cases often lead to critical bugs or security vulnerabilities. By addressing these in your interview solutions, you’re showing that you understand the importance of writing robust, production-ready code.
Shows Technical Maturity
Considering edge cases indicates that you have experience dealing with complex systems and understand the nuances of software development. It shows that you’re not just focused on making something work for the happy path, but that you’re thinking about all possible scenarios.
Differentiates You from Other Candidates
Many candidates might be able to solve the main problem, but those who also handle edge cases effectively stand out. It can be a deciding factor in competitive interview processes.
3. How to Identify Edge Cases
Identifying edge cases is a skill that improves with practice. Here are some strategies to help you spot potential edge cases:
Consider the Extremes
Think about the minimum and maximum possible values for your inputs. What happens if you get the smallest or largest allowed input?
Think About Empty or Null Inputs
Always consider what should happen if the input is empty, null, or undefined.
Look for Type Mismatches
What if the input is not of the expected type? For example, what if a function expecting a number receives a string?
Consider Boundary Conditions
Are there any points where the behavior of your algorithm changes? These transition points are often sources of edge cases.
Think About Scale
How does your solution behave with very small inputs? What about very large inputs?
Consider Invalid or Unexpected Inputs
What should happen if the input is invalid or in an unexpected format?
4. Common Edge Cases and How to Handle Them
Let’s look at some common edge cases you might encounter in coding interviews and how to handle them:
Empty Inputs
Empty inputs are one of the most common edge cases. Always consider what should happen if your function receives an empty string, array, or other data structure.
Example:
function findMax(arr) {
if (arr.length === 0) {
throw new Error("Cannot find maximum of an empty array");
}
return Math.max(...arr);
}
Null or Undefined Inputs
Check for null or undefined inputs at the beginning of your function and handle them appropriately.
Example:
function processData(data) {
if (data === null || data === undefined) {
return "No data provided";
}
// Process the data
}
Large Inputs
Consider how your algorithm performs with very large inputs. This is especially important for problems involving arrays or strings.
Example:
function sumArray(arr) {
return arr.reduce((sum, num) => {
// Use a BigInt for large numbers to avoid overflow
return BigInt(sum) + BigInt(num);
}, 0n);
}
Boundary Values
Test your code with inputs at the boundaries of valid ranges.
Example:
function getDayOfWeek(day) {
const days = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
if (day < 1 || day > 7) {
throw new Error("Invalid day number");
}
return days[day - 1];
}
Type Mismatches
Handle cases where the input might not be of the expected type.
Example:
function square(num) {
if (typeof num !== "number") {
throw new TypeError("Input must be a number");
}
return num * num;
}
5. Strategies for Effectively Handling Edge Cases
Now that we’ve covered some common edge cases, let’s discuss strategies for effectively handling them in your code:
Start with Input Validation
Always validate your inputs at the beginning of your function. This helps catch edge cases early and prevents unexpected behavior later in your code.
Use Defensive Programming
Assume that things can go wrong and code defensively. This means checking for potential issues and handling them gracefully.
Write Clear Error Messages
When handling edge cases, provide clear and informative error messages. This helps with debugging and improves the overall quality of your code.
Use Type Checking
In languages that support it, use type checking to ensure that inputs are of the expected type. In JavaScript, you can use the typeof operator or instanceof for more complex types.
Consider Using Default Values
For some edge cases, it might be appropriate to use default values instead of throwing an error. This can make your function more flexible and easier to use.
Test Thoroughly
Write test cases that specifically target edge cases. This helps ensure that your code handles these scenarios correctly.
6. Real-World Examples of Edge Case Handling
Let’s look at some real-world examples of how edge cases might be handled in coding interview questions:
Example 1: Binary Search
In a binary search algorithm, we need to consider several edge cases:
function binarySearch(arr, target) {
if (arr.length === 0) {
return -1; // Edge case: empty array
}
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Edge case: target not found
}
In this example, we handle the edge cases of an empty array and the target not being found in the array.
Example 2: Reversing a Linked List
When reversing a linked list, we need to consider the cases of an empty list and a list with only one node:
class ListNode {
constructor(val = 0, next = null) {
this.val = val;
this.next = next;
}
}
function reverseLinkedList(head) {
if (head === null || head.next === null) {
return head; // Edge cases: empty list or single node
}
let prev = null;
let current = head;
while (current !== null) {
const nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
Here, we handle the edge cases of an empty list and a list with only one node by returning the head immediately in these cases.
Example 3: Finding the Maximum Subarray Sum
In the maximum subarray sum problem (Kadane’s algorithm), we need to consider the case where all numbers are negative:
function maxSubarraySum(arr) {
if (arr.length === 0) {
throw new Error("Array is empty"); // Edge case: empty array
}
let maxSum = arr[0];
let currentSum = arr[0];
for (let i = 1; i < arr.length; i++) {
currentSum = Math.max(arr[i], currentSum + arr[i]);
maxSum = Math.max(maxSum, currentSum);
}
return maxSum;
}
This implementation handles the edge cases of an empty array and an array containing all negative numbers correctly.
7. Best Practices for Edge Case Management
To effectively manage edge cases in your coding interviews and beyond, consider the following best practices:
Document Your Assumptions
Clearly state any assumptions you’re making about the input or problem constraints. This shows that you’ve thought about potential edge cases and helps clarify your approach.
Communicate with the Interviewer
If you’re unsure about how to handle certain edge cases, don’t hesitate to ask the interviewer for clarification. This shows that you’re considering these scenarios and can lead to valuable discussions.
Prioritize Edge Cases
Not all edge cases are equally important. Focus on the most critical ones first, especially those that could cause your program to crash or produce incorrect results.
Use Assertions
In languages that support them, use assertions to check for conditions that should always be true. This can help catch edge cases early in development.
Write Unit Tests
Develop a suite of unit tests that specifically target edge cases. This ensures that your code continues to handle these scenarios correctly as you make changes.
Refactor for Readability
As you add edge case handling, make sure your code remains readable. Consider extracting complex conditions into well-named helper functions.
Consider Performance
While it’s important to handle edge cases, be mindful of the performance impact. Strive for a balance between robustness and efficiency.
8. Conclusion
Handling edge cases is a critical skill for any programmer, and it’s especially important in coding interviews. By demonstrating your ability to think beyond the obvious and account for unusual or extreme scenarios, you show that you’re capable of writing robust, production-ready code.
Remember, the key to effectively handling edge cases is to:
- Identify potential edge cases by considering extremes, empty inputs, type mismatches, and boundary conditions.
- Implement strategies to handle these cases, such as input validation, defensive programming, and clear error handling.
- Practice with real-world examples and coding interview questions to improve your edge case detection and handling skills.
- Follow best practices like documenting assumptions, communicating clearly, and writing thorough unit tests.
By mastering the art of handling edge cases, you’ll not only perform better in coding interviews but also become a more skilled and reliable programmer overall. This attention to detail and thoroughness is what sets apart great developers and is highly valued in the software industry.
As you continue your journey in coding education and interview preparation, remember that platforms like AlgoCademy can provide valuable resources and practice opportunities to hone your edge case handling skills. Keep practicing, stay curious, and always think beyond the obvious. Happy coding!