Hamiltonian Path and Circuit: Mastering Advanced Graph Algorithms
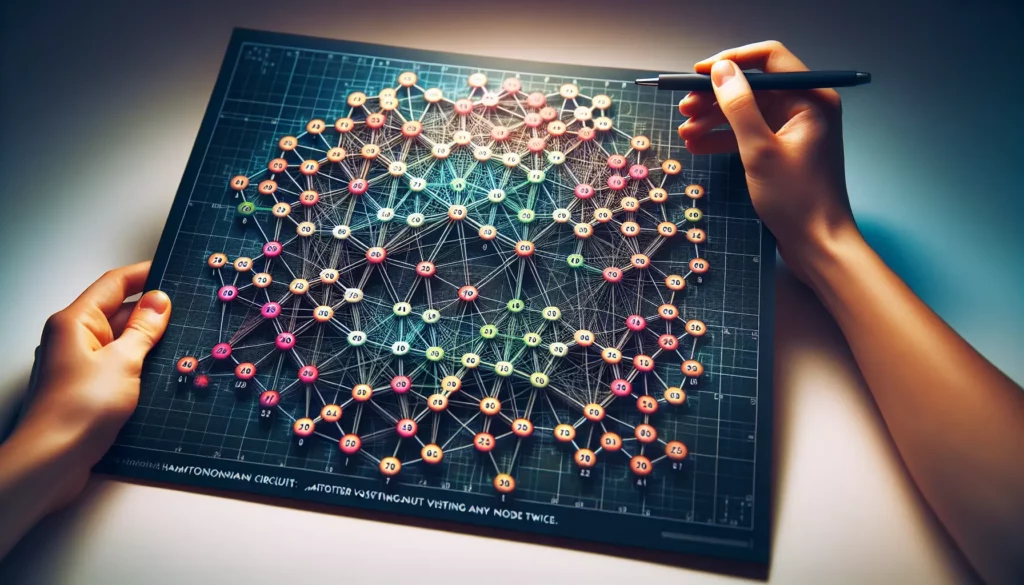
Welcome to another in-depth exploration of graph algorithms on AlgoCademy! Today, we’re diving into the fascinating world of Hamiltonian paths and circuits. These concepts are not only crucial for advancing your understanding of graph theory but also play a significant role in solving real-world problems and acing technical interviews at top tech companies.
What are Hamiltonian Paths and Circuits?
Before we delve into the intricacies of Hamiltonian paths and circuits, let’s start with their definitions:
- Hamiltonian Path: A path in an undirected or directed graph that visits each vertex exactly once.
- Hamiltonian Circuit: A Hamiltonian path that is a cycle, i.e., it starts and ends at the same vertex.
These concepts are named after the renowned mathematician William Rowan Hamilton, who invented the Icosian game, which involves finding a Hamiltonian cycle along the edges of a dodecahedron.
The Importance of Hamiltonian Paths and Circuits
Understanding Hamiltonian paths and circuits is crucial for several reasons:
- Problem-Solving: They are fundamental to solving various real-world problems, such as optimizing delivery routes or planning efficient travel itineraries.
- Algorithm Design: Mastering these concepts enhances your ability to design and analyze complex algorithms.
- Interview Preparation: Questions related to Hamiltonian paths and circuits are common in technical interviews, especially at FAANG companies.
- Graph Theory: They provide deeper insights into graph structures and properties.
Properties of Hamiltonian Paths and Circuits
Before we dive into algorithms and implementations, let’s discuss some key properties:
- Not all graphs have Hamiltonian paths or circuits.
- A graph that contains a Hamiltonian circuit also contains a Hamiltonian path, but the reverse is not always true.
- The problem of determining whether a graph contains a Hamiltonian path or circuit is NP-complete, meaning there’s no known polynomial-time algorithm for solving it in all cases.
- Certain graph properties can guarantee the existence of a Hamiltonian circuit (e.g., Dirac’s theorem and Ore’s theorem).
Algorithms for Finding Hamiltonian Paths and Circuits
Now, let’s explore some algorithms for finding Hamiltonian paths and circuits:
1. Brute Force Approach
The simplest (but least efficient) method is to generate all possible permutations of vertices and check if they form a valid Hamiltonian path or circuit.
def is_hamiltonian_path(graph, path):
if len(path) != len(graph):
return False
for i in range(len(path) - 1):
if path[i+1] not in graph[path[i]]:
return False
return True
def find_hamiltonian_path(graph):
vertices = list(graph.keys())
for path in itertools.permutations(vertices):
if is_hamiltonian_path(graph, path):
return path
return None
This approach has a time complexity of O(n!), making it impractical for large graphs.
2. Backtracking Algorithm
A more efficient approach is to use backtracking, which builds the solution incrementally and abandons paths that cannot lead to a solution.
def hamiltonian_path_util(graph, path, pos):
if pos == len(graph):
return True
for v in graph:
if v not in path and (not path or v in graph[path[-1]]):
path.append(v)
if hamiltonian_path_util(graph, path, pos + 1):
return True
path.pop()
return False
def find_hamiltonian_path(graph):
path = []
if hamiltonian_path_util(graph, path, 0):
return path
return None
While still having a worst-case time complexity of O(n!), backtracking often performs much better in practice.
3. Dynamic Programming Approach
For small to medium-sized graphs, a dynamic programming approach can be more efficient:
def find_hamiltonian_path_dp(graph):
n = len(graph)
dp = [[False] * n for _ in range(1 << n)]
for i in range(n):
dp[1 << i][i] = True
for mask in range(1, 1 << n):
for v in range(n):
if mask & (1 << v):
for u in range(n):
if mask & (1 << u) and graph[u][v] and u != v:
if dp[mask ^ (1 << v)][u]:
dp[mask][v] = True
break
full_mask = (1 << n) - 1
if not any(dp[full_mask]):
return None
path = []
mask = full_mask
last = next(v for v in range(n) if dp[mask][v])
for i in range(n-1, -1, -1):
path.append(last)
new_last = next(u for u in range(n) if u != last and (mask & (1 << u)) and graph[u][last] and dp[mask ^ (1 << last)][u])
mask ^= (1 << last)
last = new_last
return path[::-1]
This approach has a time complexity of O(n^2 * 2^n), which is better than O(n!) for smaller values of n.
Real-World Applications
Hamiltonian paths and circuits have numerous practical applications:
- Traveling Salesman Problem: Finding the shortest possible route that visits each city exactly once and returns to the starting city.
- DNA Fragment Assembly: In bioinformatics, reconstructing DNA sequences from smaller fragments.
- Game Design: Creating puzzle games where the player must visit every location exactly once.
- Circuit Design: Optimizing the layout of electronic circuits to minimize wire length.
- Logistics and Transportation: Planning efficient delivery routes or transportation schedules.
Interview Strategies for Hamiltonian Path Problems
When tackling Hamiltonian path problems in technical interviews, consider the following strategies:
- Understand the Problem: Clarify whether you’re looking for a Hamiltonian path or circuit, and if any additional constraints exist.
- Consider Graph Properties: Check if the graph satisfies any conditions that guarantee the existence of a Hamiltonian path or circuit.
- Start Simple: Begin with a brute-force approach to demonstrate your problem-solving process, then optimize.
- Use Visualization: Draw the graph and potential paths to better understand the problem and explain your thought process.
- Discuss Trade-offs: Explain the time and space complexity trade-offs between different approaches (brute-force, backtracking, dynamic programming).
- Optimize for the Specific Case: If the graph is small, a simpler algorithm might suffice. For larger graphs, discuss more advanced techniques or approximation algorithms.
Common Pitfalls and How to Avoid Them
When working with Hamiltonian paths and circuits, be aware of these common pitfalls:
- Confusing Paths and Circuits: Always clarify whether you’re looking for a path (which doesn’t need to return to the start) or a circuit (which does).
- Overlooking Edge Cases: Consider graphs with no solutions, single-vertex graphs, and disconnected graphs.
- Inefficient Implementations: Be mindful of unnecessary computations, especially in recursive solutions.
- Ignoring Graph Properties: Failing to check for properties that might simplify the problem or guarantee a solution.
- Misunderstanding Time Complexity: Remember that finding a Hamiltonian path is NP-complete, so exponential time algorithms are often unavoidable for exact solutions.
Advanced Topics and Variations
As you progress in your understanding of Hamiltonian paths and circuits, consider exploring these advanced topics:
1. Approximation Algorithms
For large graphs where finding an exact solution is impractical, approximation algorithms can find near-optimal solutions in polynomial time. The Christofides algorithm, for example, provides a 1.5-approximation for the metric Traveling Salesman Problem.
2. Hamiltonian Decomposition
This involves partitioning the edges of a graph into disjoint Hamiltonian circuits. It’s related to the famous Lovász conjecture in graph theory.
3. Gray Code
A Gray code is a sequence of binary strings where each pair of adjacent strings differs in exactly one bit. Generating a Gray code can be viewed as finding a Hamiltonian path in a hypercube graph.
4. Pancake Sorting
This problem, where you sort a stack of pancakes by flipping prefixes, can be modeled as finding a Hamiltonian path in a specific graph.
Implementing a Hamiltonian Path Finder in Python
Let’s implement a more comprehensive Hamiltonian path finder that combines backtracking with some optimizations:
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[0 for _ in range(vertices)] for _ in range(vertices)]
def add_edge(self, u, v):
self.graph[u][v] = 1
self.graph[v][u] = 1
def is_safe(self, v, pos, path):
if self.graph[path[pos-1]][v] == 0:
return False
if v in path:
return False
return True
def hamiltonian_util(self, path, pos):
if pos == self.V:
if self.graph[path[pos-1]][path[0]] == 1:
return True
else:
return False
for v in range(1, self.V):
if self.is_safe(v, pos, path):
path[pos] = v
if self.hamiltonian_util(path, pos + 1):
return True
path[pos] = -1
return False
def hamiltonian_cycle(self):
path = [-1] * self.V
path[0] = 0
if not self.hamiltonian_util(path, 1):
print("No Hamiltonian cycle exists")
return False
self.print_solution(path)
return True
def print_solution(self, path):
print("Hamiltonian cycle found:")
for vertex in path:
print(vertex, end=" ")
print(path[0])
# Example usage
g = Graph(5)
g.add_edge(0, 1)
g.add_edge(0, 3)
g.add_edge(1, 2)
g.add_edge(1, 3)
g.add_edge(1, 4)
g.add_edge(2, 4)
g.add_edge(3, 4)
g.hamiltonian_cycle()
This implementation uses backtracking with some optimizations, such as checking for edge existence and avoiding revisiting vertices. It’s more efficient than the brute-force approach but still has exponential time complexity in the worst case.
Conclusion
Mastering Hamiltonian paths and circuits is a crucial step in your journey to becoming a proficient algorithm designer and problem solver. These concepts not only enhance your understanding of graph theory but also prepare you for challenging technical interviews at top tech companies.
Remember, while finding Hamiltonian paths and circuits is computationally hard in general, understanding the underlying principles and various approaches to solve these problems will significantly boost your algorithmic thinking skills. Keep practicing with different graph structures and sizes to solidify your understanding and improve your problem-solving speed.
As you continue your learning journey with AlgoCademy, don’t hesitate to explore related topics such as graph coloring, maximum flow problems, and advanced dynamic programming techniques. These will further enhance your ability to tackle complex algorithmic challenges and excel in your coding career.
Happy coding, and may your paths always be Hamiltonian!