Grokking the Coding Interview: A Comprehensive Guide to Mastering Technical Interviews
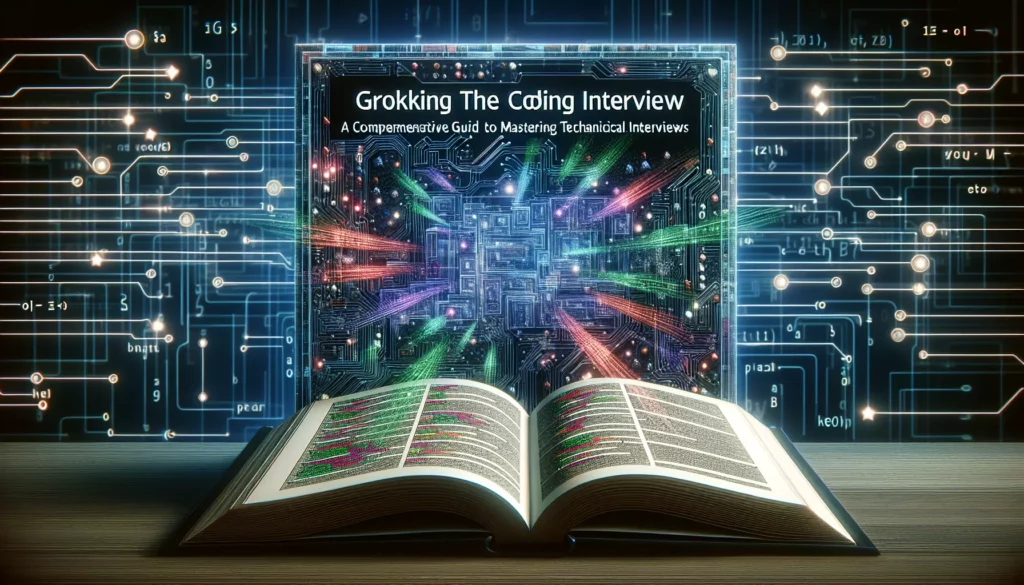
In the competitive world of software engineering, landing a job at a top tech company often hinges on your performance in coding interviews. These interviews can be daunting, requiring a deep understanding of algorithms, data structures, and problem-solving techniques. Enter “Grokking the Coding Interview” – a concept that has gained immense popularity among aspiring developers and seasoned programmers alike. In this comprehensive guide, we’ll explore what it means to “grok” the coding interview, why it’s crucial for your career, and how you can master this essential skill.
What Does “Grokking” Mean?
Before we dive into the specifics of coding interviews, let’s understand what “grokking” actually means. The term “grok” was coined by Robert A. Heinlein in his 1961 science fiction novel “Stranger in a Strange Land.” It means to understand something so thoroughly that it becomes a part of you. In the context of coding interviews, grokking implies not just memorizing solutions but truly comprehending the underlying principles and patterns that make those solutions work.
Why is Grokking the Coding Interview Important?
Mastering coding interviews is crucial for several reasons:
- Career Advancement: Top tech companies like Google, Facebook, Amazon, Apple, and Netflix (often referred to as FAANG) use coding interviews as a primary method of evaluating candidates.
- Problem-Solving Skills: The skills you develop while preparing for coding interviews are directly applicable to real-world software development challenges.
- Algorithmic Thinking: Grokking coding interviews enhances your ability to think algorithmically, which is essential for writing efficient and scalable code.
- Confidence Boost: Being well-prepared for coding interviews can significantly boost your confidence, helping you perform better under pressure.
Key Components of Coding Interviews
To truly grok coding interviews, you need to understand their key components:
1. Data Structures
A solid grasp of fundamental data structures is crucial. These include:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Understanding when and how to use these data structures efficiently is key to solving many coding problems.
2. Algorithms
Familiarity with common algorithms and their applications is essential. Some important categories include:
- Sorting and Searching
- Recursion and Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
- Graph Algorithms
3. Time and Space Complexity Analysis
Being able to analyze the efficiency of your solutions in terms of time and space complexity is crucial. This involves understanding Big O notation and being able to optimize your code for better performance.
4. Problem-Solving Techniques
Developing a systematic approach to problem-solving is vital. This includes:
- Understanding the problem statement
- Identifying edge cases
- Developing a solution strategy
- Implementing the solution
- Testing and debugging
Strategies for Grokking the Coding Interview
Now that we understand the components, let’s explore strategies to truly grok coding interviews:
1. Practice, Practice, Practice
Consistent practice is the key to mastering coding interviews. Utilize platforms like LeetCode, HackerRank, or AlgoCademy to solve a variety of problems regularly. Aim to solve at least one problem a day, gradually increasing the difficulty level.
2. Study Pattern Recognition
Many coding interview questions follow certain patterns. Learning to recognize these patterns can significantly speed up your problem-solving process. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a LinkedList
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
3. Implement Data Structures from Scratch
To truly understand data structures, try implementing them from scratch. This exercise will give you a deeper understanding of how they work internally and when to use them effectively.
4. Analyze Multiple Solutions
For each problem you solve, don’t stop at finding a working solution. Explore multiple approaches and analyze their trade-offs in terms of time and space complexity. This practice will help you develop the ability to quickly evaluate different solutions during an interview.
5. Mock Interviews
Participate in mock interviews with peers or use platforms that offer mock interview services. This will help you get comfortable with explaining your thought process out loud and working under time pressure.
6. Review and Reflect
After solving a problem or participating in a mock interview, take time to review and reflect on your performance. Identify areas for improvement and make a plan to address them.
Common Coding Interview Patterns and Examples
Let’s dive deeper into some common coding interview patterns with examples to illustrate how they work:
1. Two Pointers
The two pointers technique involves using two pointers to solve a problem, often moving them towards each other or in the same direction.
Example: Reverse a String
function reverseString(s: string[]): void {
let left = 0;
let right = s.length - 1;
while (left < right) {
// Swap characters
[s[left], s[right]] = [s[right], s[left]];
left++;
right--;
}
}
2. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or string.
Example: Find the maximum sum subarray of size K
function maxSubarraySum(arr: number[], k: number): number {
let maxSum = 0;
let windowSum = 0;
for (let i = 0; i < arr.length; i++) {
windowSum += arr[i];
if (i >= k - 1) {
maxSum = Math.max(maxSum, windowSum);
windowSum -= arr[i - (k - 1)];
}
}
return maxSum;
}
3. Fast and Slow Pointers
This technique uses two pointers moving at different speeds to solve problems, often used in linked list problems.
Example: Detect a cycle in a linked list
class ListNode {
val: number;
next: ListNode | null;
constructor(val?: number, next?: ListNode | null) {
this.val = (val===undefined ? 0 : val);
this.next = (next===undefined ? null : next);
}
}
function hasCycle(head: ListNode | null): boolean {
if (!head || !head.next) return false;
let slow = head;
let fast = head.next;
while (slow !== fast) {
if (!fast || !fast.next) return false;
slow = slow.next!;
fast = fast.next.next;
}
return true;
}
4. Merge Intervals
This pattern deals with problems involving overlapping intervals.
Example: Merge overlapping intervals
function mergeIntervals(intervals: number[][]): number[][] {
if (intervals.length <= 1) return intervals;
intervals.sort((a, b) => a[0] - b[0]);
const result: number[][] = [intervals[0]];
for (let i = 1; i < intervals.length; i++) {
const currentInterval = intervals[i];
const lastMergedInterval = result[result.length - 1];
if (currentInterval[0] <= lastMergedInterval[1]) {
lastMergedInterval[1] = Math.max(lastMergedInterval[1], currentInterval[1]);
} else {
result.push(currentInterval);
}
}
return result;
}
5. Tree Depth-First Search (DFS)
DFS is used to traverse or search tree or graph data structures by exploring as far as possible along each branch before backtracking.
Example: Inorder traversal of a binary tree
class TreeNode {
val: number;
left: TreeNode | null;
right: TreeNode | null;
constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) {
this.val = (val===undefined ? 0 : val);
this.left = (left===undefined ? null : left);
this.right = (right===undefined ? null : right);
}
}
function inorderTraversal(root: TreeNode | null): number[] {
const result: number[] = [];
function dfs(node: TreeNode | null) {
if (!node) return;
dfs(node.left);
result.push(node.val);
dfs(node.right);
}
dfs(root);
return result;
}
Advanced Topics in Coding Interviews
As you progress in your journey of grokking the coding interview, you’ll encounter more advanced topics. Here are some areas to focus on:
1. Dynamic Programming
Dynamic Programming (DP) is a powerful technique used to solve optimization problems by breaking them down into simpler subproblems. It’s often used when the problem has overlapping subproblems and optimal substructure.
Example: Fibonacci Sequence with Memoization
function fibonacci(n: number, memo: Record<number, number> = {}): number {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
2. Graph Algorithms
Understanding graph algorithms is crucial for many complex problems. Key algorithms include:
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Topological Sort
Example: Implementing BFS
function bfs(graph: Record<string, string[]>, start: string): string[] {
const visited: Set<string> = new Set();
const queue: string[] = [start];
const result: string[] = [];
while (queue.length > 0) {
const vertex = queue.shift()!;
if (!visited.has(vertex)) {
visited.add(vertex);
result.push(vertex);
queue.push(...(graph[vertex] || []));
}
}
return result;
}
3. Bit Manipulation
Bit manipulation can lead to highly optimized solutions for certain problems. Key operations include:
- AND, OR, XOR
- Left and Right Shifts
- Setting, Clearing, and Toggling Bits
Example: Counting set bits in an integer
function countSetBits(n: number): number {
let count = 0;
while (n) {
count += n & 1;
n >>>= 1;
}
return count;
}
4. System Design
For more senior roles, system design questions are common. These test your ability to design large-scale distributed systems. Key areas to study include:
- Scalability
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
Common Pitfalls and How to Avoid Them
Even with extensive preparation, there are common pitfalls that candidates often fall into during coding interviews. Here’s how to avoid them:
1. Jumping to Code Too Quickly
Pitfall: Starting to code without fully understanding the problem or considering different approaches.
How to Avoid: Take time to clarify the problem, discuss potential approaches with your interviewer, and outline your solution before coding.
2. Neglecting Edge Cases
Pitfall: Focusing only on the happy path and forgetting to handle edge cases.
How to Avoid: Always consider edge cases like empty inputs, negative numbers, or boundary conditions before finalizing your solution.
3. Poor Communication
Pitfall: Coding silently without explaining your thought process.
How to Avoid: Practice thinking out loud. Explain your approach, why you’re making certain decisions, and any trade-offs you’re considering.
4. Ignoring Time and Space Complexity
Pitfall: Providing a working solution without considering its efficiency.
How to Avoid: Always analyze and discuss the time and space complexity of your solution. Be prepared to optimize if needed.
5. Getting Stuck Without Making Progress
Pitfall: Spending too much time stuck on one approach without making progress.
How to Avoid: If you’re stuck, communicate this to your interviewer. They may provide hints or guide you towards a solution. Don’t be afraid to start with a brute force approach and then optimize.
The Role of Soft Skills in Coding Interviews
While technical skills are crucial, soft skills play a significant role in coding interviews. Here’s why they matter and how to improve them:
1. Communication
Why it Matters: Clear communication helps interviewers understand your thought process and problem-solving approach.
How to Improve: Practice explaining your solutions to others. Participate in coding meetups or study groups where you can discuss problems and solutions.
2. Collaboration
Why it Matters: Many interviews include pair programming or collaborative problem-solving to assess how well you work with others.
How to Improve: Engage in pair programming sessions with peers. Contribute to open-source projects to gain experience working with others on code.
3. Handling Pressure
Why it Matters: Coding interviews can be stressful, and companies want to see how you perform under pressure.
How to Improve: Practice with timed coding challenges. Participate in coding competitions to get used to solving problems under time constraints.
4. Adaptability
Why it Matters: Interviewers often introduce new constraints or ask you to optimize your solution, testing your ability to adapt.
How to Improve: After solving a problem, challenge yourself to solve it in a different way or with additional constraints.
Leveraging Tools and Resources
To effectively grok the coding interview, it’s important to leverage the right tools and resources. Here are some recommendations:
1. Online Coding Platforms
- LeetCode: Offers a wide range of coding problems with difficulty levels and company tags.
- HackerRank: Provides coding challenges and contests, often used by companies for initial screenings.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
2. Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “The Algorithm Design Manual” by Steven S. Skiena
3. Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare’s “Introduction to Algorithms”
- Udacity’s “Data Structures and Algorithms Nanodegree”
4. GitHub Repositories
- javascript-algorithms: Algorithms and data structures implemented in JavaScript with explanations and links to further readings
- interviews: A repository containing everything you need to prepare for your technical interview
Conclusion: The Journey to Mastery
Grokking the coding interview is not just about passing a test or landing a job; it’s about developing a deep understanding of computer science fundamentals and problem-solving techniques that will serve you throughout your career. It’s a journey that requires persistence, practice, and continuous learning.
Remember that every problem you solve, every algorithm you study, and every data structure you implement brings you one step closer to mastery. Embrace the challenge, stay curious, and don’t be discouraged by setbacks. With dedication and the right approach, you can not only pass coding interviews but excel in them.
As you progress in your journey, consider how you can contribute back to the community. Share your knowledge, mentor others, and continue to push the boundaries of your understanding. The field of computer science is vast and ever-evolving, offering endless opportunities for growth and discovery.
Finally, keep in mind that while technical skills are crucial, they are just one part of what makes a great software engineer. Cultivate your soft skills, embrace teamwork, and always strive to write clean, maintainable code. By doing so, you’ll not only ace your coding interviews but also set yourself up for a successful and fulfilling career in software development.
Happy coding, and may your journey of grokking the coding interview be as rewarding as the destination!