Greedy Algorithms: Efficient Problem-Solving Techniques for Programmers
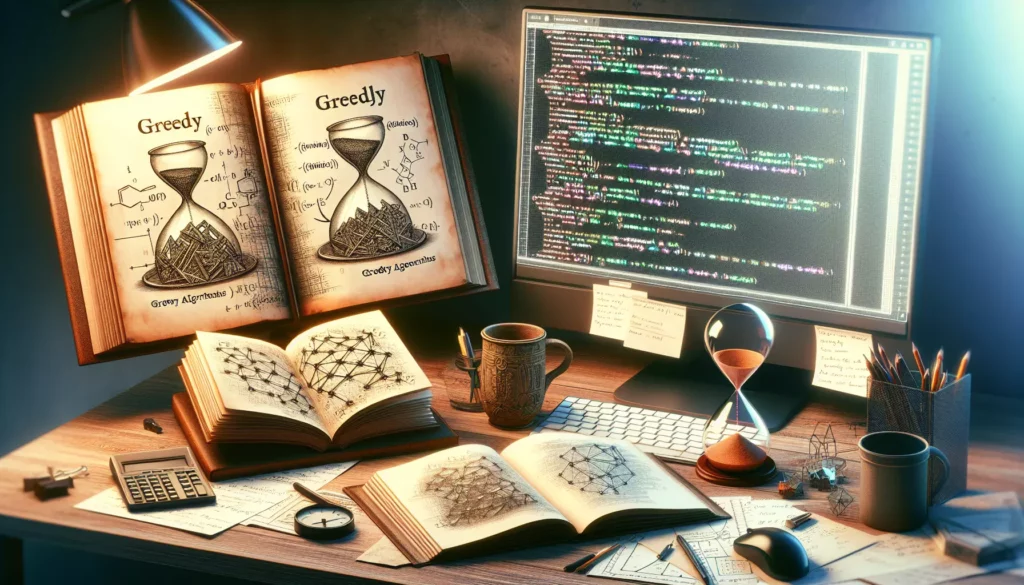
In the world of computer science and programming, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and solve complex problems with minimal computational resources. One powerful technique that has proven invaluable in this quest for efficiency is the use of greedy algorithms. In this comprehensive guide, we’ll dive deep into the world of greedy algorithms, exploring their principles, applications, strengths, and limitations.
What Are Greedy Algorithms?
Greedy algorithms are a class of algorithms that follow the problem-solving heuristic of making the locally optimal choice at each step, with the hope of finding a global optimum. In other words, a greedy algorithm always makes the choice that seems best at the moment, without considering the bigger picture or future consequences of that choice.
The key characteristics of greedy algorithms include:
- Making locally optimal choices at each step
- Never reconsidering or backtracking on previous decisions
- Aiming to find an approximate global optimum in a reasonable amount of time
While this approach doesn’t always lead to the best overall solution, it often provides a good approximation of the optimal solution and can be implemented with relative ease and efficiency.
The Greedy Choice Property
The fundamental principle behind greedy algorithms is the greedy choice property. This property states that a globally optimal solution can be reached by making locally optimal choices. In other words, the best solution to the overall problem can be constructed by always choosing the best immediate, or local, solution.
It’s important to note that not all problems exhibit the greedy choice property. For problems that do, greedy algorithms can be an excellent choice. However, for problems that don’t have this property, greedy algorithms may fail to find the optimal solution.
When to Use Greedy Algorithms
Greedy algorithms are particularly useful in optimization problems where we need to maximize or minimize some value. They are often applied in scenarios where:
- The problem has optimal substructure (i.e., an optimal solution to the problem contains optimal solutions to sub-problems)
- The problem has the greedy choice property
- Local optimal choices lead to a global optimal solution
Some common problem domains where greedy algorithms excel include:
- Scheduling problems
- Resource allocation
- Data compression
- Graph algorithms (e.g., minimum spanning tree, shortest path)
- Huffman coding
Advantages of Greedy Algorithms
Greedy algorithms offer several advantages that make them attractive to programmers and computer scientists:
- Simplicity: Greedy algorithms are often straightforward to design and implement, making them accessible to programmers of all skill levels.
- Efficiency: In many cases, greedy algorithms run in linear or near-linear time, making them highly efficient for large-scale problems.
- Approximation: Even when they don’t provide the optimal solution, greedy algorithms often produce good approximations of the optimal solution.
- Incremental approach: Greedy algorithms make decisions incrementally, which can be beneficial in scenarios where not all input is available at once or when dealing with streaming data.
Limitations of Greedy Algorithms
While greedy algorithms have many strengths, they also come with some limitations:
- Suboptimal solutions: Greedy algorithms don’t always find the globally optimal solution, as they make locally optimal choices without considering the overall problem state.
- Lack of flexibility: Once a choice is made, a greedy algorithm never reconsiders it, which can lead to suboptimal solutions in problems with complex dependencies.
- Problem-specific: The effectiveness of a greedy algorithm often depends on the specific problem and its structure. A greedy approach that works well for one problem may fail for a similar but slightly different problem.
Common Greedy Algorithm Examples
Let’s explore some classic examples of greedy algorithms to better understand their application and implementation.
1. Coin Change Problem
The coin change problem is a classic example of where a greedy algorithm can be applied. The goal is to find the minimum number of coins needed to make a certain amount of change, given a set of coin denominations.
Here’s a Python implementation of a greedy algorithm for the coin change problem:
def coin_change(coins, amount):
coins.sort(reverse=True) # Sort coins in descending order
coin_count = 0
for coin in coins:
while amount >= coin:
amount -= coin
coin_count += 1
return coin_count if amount == 0 else -1
# Example usage
coins = [25, 10, 5, 1] # Quarter, dime, nickel, penny
amount = 67
result = coin_change(coins, amount)
print(f"Minimum number of coins needed: {result}")
In this example, the greedy choice is to always use the largest coin possible. While this works for the US coin system, it’s worth noting that this greedy approach doesn’t always yield the optimal solution for all coin systems.
2. Activity Selection Problem
The activity selection problem involves selecting the maximum number of non-overlapping activities that can be performed by a single person, given their start and finish times.
Here’s a Python implementation of a greedy algorithm for the activity selection problem:
def activity_selection(activities):
# Sort activities based on finish time
activities.sort(key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for activity in activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
result = activity_selection(activities)
print(f"Selected activities: {result}")
In this problem, the greedy choice is to always select the next activity with the earliest finish time that doesn’t overlap with the previously selected activity.
3. Huffman Coding
Huffman coding is a greedy algorithm used for lossless data compression. It assigns variable-length codes to characters based on their frequencies, with more frequent characters getting shorter codes.
Here’s a simplified Python implementation of Huffman coding:
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
# Count frequency of each character
freq = defaultdict(int)
for char in text:
freq[char] += 1
# Create a priority queue of nodes
heap = [Node(char, frequency) for char, frequency in freq.items()]
heapq.heapify(heap)
# Build the Huffman tree
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
internal = Node(None, left.freq + right.freq)
internal.left = left
internal.right = right
heapq.heappush(heap, internal)
return heap[0]
def generate_codes(root, code='', mapping=None):
if mapping is None:
mapping = {}
if root is None:
return
if root.char is not None:
mapping[root.char] = code
generate_codes(root.left, code + '0', mapping)
generate_codes(root.right, code + '1', mapping)
return mapping
# Example usage
text = "this is an example for huffman encoding"
root = build_huffman_tree(text)
codes = generate_codes(root)
print("Huffman Codes:")
for char, code in codes.items():
print(f"{char}: {code}")
In Huffman coding, the greedy choice is to repeatedly combine the two nodes with the lowest frequencies to build the Huffman tree.
Greedy vs. Dynamic Programming
While greedy algorithms and dynamic programming are both optimization techniques, they differ in their approach and applicability:
- Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. They are typically faster and use less memory, but may not always find the optimal solution.
- Dynamic programming breaks down a problem into smaller subproblems, solves each subproblem only once, and stores the solutions to subproblems to avoid redundant computations. Dynamic programming is generally more versatile and guarantees finding the optimal solution, but it can be slower and more memory-intensive than greedy algorithms.
The choice between greedy and dynamic programming often depends on the specific problem and its requirements. Some problems can be solved optimally with a greedy approach, while others require the more comprehensive approach of dynamic programming.
Proving the Correctness of Greedy Algorithms
Proving that a greedy algorithm produces the optimal solution for a given problem is crucial. There are two main techniques for proving the correctness of greedy algorithms:
- Greedy Choice Property: Prove that a greedy choice at each step leads to an optimal solution.
- Optimal Substructure: Show that an optimal solution to the problem contains optimal solutions to subproblems.
To prove the correctness of a greedy algorithm, you typically need to:
- Show that the greedy choice is always safe, i.e., there exists an optimal solution consistent with the greedy choice.
- Prove that after making the greedy choice, the remaining subproblem has the same structure as the original problem.
- Demonstrate that combining the greedy choice with the optimal solution to the subproblem yields an optimal solution to the original problem.
Common Pitfalls and How to Avoid Them
When working with greedy algorithms, be aware of these common pitfalls:
- Assuming greedy always works: Not all problems can be solved optimally with a greedy approach. Always verify that the problem has the greedy choice property and optimal substructure.
- Overlooking edge cases: Greedy algorithms can sometimes fail on specific edge cases. Be sure to thoroughly test your algorithm with a variety of inputs, including edge cases.
- Incorrect greedy choice: Choosing the wrong criteria for the greedy choice can lead to suboptimal solutions. Carefully analyze the problem to determine the correct greedy choice.
- Neglecting to prove correctness: Always attempt to prove the correctness of your greedy algorithm, either formally or through rigorous testing.
Greedy Algorithms in Technical Interviews
Greedy algorithms are a popular topic in technical interviews, especially for positions at major tech companies. Here are some tips for tackling greedy algorithm problems in interviews:
- Identify the problem type: Recognize when a problem might be suitable for a greedy approach.
- Articulate your greedy choice: Clearly explain what criteria you’re using for making the greedy choice at each step.
- Consider alternatives: Discuss why a greedy approach might be better than other methods (e.g., dynamic programming, brute force) for the given problem.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your greedy solution.
- Test your solution: Walk through your algorithm with example inputs to demonstrate its correctness and catch any potential issues.
Conclusion
Greedy algorithms are a powerful tool in a programmer’s arsenal, offering efficient solutions to a wide range of optimization problems. While they may not always provide the globally optimal solution, their simplicity and efficiency make them an attractive choice for many real-world applications.
As you continue your journey in algorithm design and problem-solving, remember that mastering greedy algorithms is just one step towards becoming a well-rounded programmer. Keep practicing, exploring different problem-solving techniques, and always strive to understand the underlying principles behind each algorithm you encounter.
By developing a deep understanding of greedy algorithms and other algorithmic paradigms, you’ll be well-equipped to tackle complex coding challenges, optimize your solutions, and excel in technical interviews at top tech companies. Happy coding!