Grasping the Basics of File Input and Output Operations
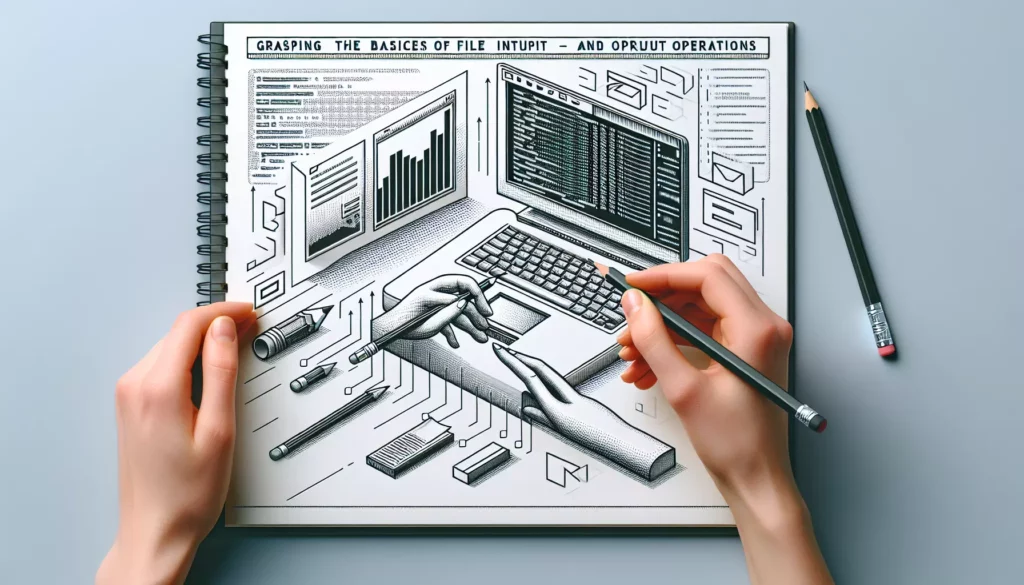
File input and output (I/O) operations are fundamental concepts in programming that allow developers to interact with files on a computer system. These operations enable reading data from files and writing data to files, which is crucial for many applications, from simple text processing to complex data storage and retrieval systems. In this comprehensive guide, we’ll explore the basics of file I/O operations, their importance in programming, and how to implement them in various programming languages.
Why File I/O Operations Matter
Before diving into the specifics, it’s essential to understand why file I/O operations are so important in programming:
- Data Persistence: File I/O allows programs to store data permanently, ensuring that information persists even after the program terminates.
- Data Exchange: Files serve as a medium for exchanging data between different programs or systems.
- Configuration Management: Many applications use configuration files to store settings and preferences.
- Logging: File I/O is crucial for creating and maintaining log files, which are essential for debugging and monitoring applications.
- Large Data Processing: When dealing with large datasets that don’t fit into memory, file I/O becomes necessary for processing data in chunks.
Basic Concepts of File I/O
To effectively work with file I/O, you need to understand several key concepts:
1. File Streams
A file stream is an abstraction that represents a sequence of bytes flowing between a program and a file. There are typically three types of streams:
- Input Stream: Used for reading data from a file.
- Output Stream: Used for writing data to a file.
- Bidirectional Stream: Allows both reading from and writing to a file.
2. File Pointers
A file pointer is a marker that indicates the current position in a file where reading or writing operations will occur. It moves as you read from or write to the file.
3. File Modes
When opening a file, you specify a mode that determines how the file can be accessed:
- Read Mode: Opens a file for reading only.
- Write Mode: Opens a file for writing, typically creating a new file or overwriting an existing one.
- Append Mode: Opens a file for writing, but adds new data at the end of the file.
- Binary Mode: Opens a file in binary format, as opposed to text format.
4. File Handling Steps
The general process for working with files includes:
- Opening the file
- Reading from or writing to the file
- Closing the file
Implementing File I/O in Different Programming Languages
Now, let’s look at how to implement basic file I/O operations in some popular programming languages.
Python
Python provides a simple and intuitive way to handle file I/O operations.
Reading from a File:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
Writing to a File:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
The with
statement in Python ensures that the file is properly closed after the operations are completed.
Java
Java offers several classes for file I/O operations. Here’s a basic example using FileReader
and FileWriter
.
Reading from a File:
import java.io.FileReader;
import java.io.BufferedReader;
import java.io.IOException;
try (BufferedReader reader = new BufferedReader(new FileReader("example.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Writing to a File:
import java.io.FileWriter;
import java.io.IOException;
try (FileWriter writer = new FileWriter("example.txt")) {
writer.write("Hello, World!");
} catch (IOException e) {
e.printStackTrace();
}
C++
C++ uses the <fstream>
library for file I/O operations.
Reading from a File:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
}
return 0;
}
Writing to a File:
#include <iostream>
#include <fstream>
int main() {
std::ofstream file("example.txt");
if (file.is_open()) {
file << "Hello, World!";
file.close();
}
return 0;
}
Advanced File I/O Concepts
As you become more proficient with basic file I/O, you’ll encounter more advanced concepts and techniques:
1. Random Access
Random access allows you to read from or write to specific positions in a file without sequentially processing all the data before it. This is particularly useful when working with large files or structured data.
Example in Python:
with open('example.txt', 'r+b') as file:
file.seek(10) # Move to the 10th byte
data = file.read(5) # Read 5 bytes
file.seek(-5, 2) # Move 5 bytes before the end of the file
file.write(b'Hello') # Write 'Hello' at that position
2. Buffered I/O
Buffered I/O involves using a memory buffer to hold data temporarily before writing it to a file or after reading it from a file. This can significantly improve performance by reducing the number of actual I/O operations.
Example in Java:
import java.io.*;
try (BufferedWriter writer = new BufferedWriter(new FileWriter("example.txt"))) {
writer.write("This is a buffered write operation.");
writer.newLine();
writer.write("It's more efficient for multiple writes.");
} catch (IOException e) {
e.printStackTrace();
}
3. Memory-Mapped Files
Memory-mapped files allow you to map a file or a portion of a file directly to memory, which can provide faster access for large files.
Example in C++:
#include <iostream>
#include <fstream>
#include <sys/mman.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("example.txt", O_RDONLY);
if (fd == -1) {
std::cerr << "Error opening file" << std::endl;
return 1;
}
// Get file size
off_t file_size = lseek(fd, 0, SEEK_END);
lseek(fd, 0, SEEK_SET);
// Map file to memory
char* mapped_data = (char*)mmap(NULL, file_size, PROT_READ, MAP_PRIVATE, fd, 0);
if (mapped_data == MAP_FAILED) {
std::cerr << "Error mapping file" << std::endl;
close(fd);
return 1;
}
// Read and print the contents
std::cout << std::string(mapped_data, file_size) << std::endl;
// Unmap and close
munmap(mapped_data, file_size);
close(fd);
return 0;
}
4. Asynchronous I/O
Asynchronous I/O allows a program to initiate I/O operations that run in the background, enabling the program to continue executing other tasks while waiting for I/O to complete.
Example in Python (using asyncio):
import asyncio
import aiofiles
async def read_file(filename):
async with aiofiles.open(filename, mode='r') as file:
content = await file.read()
print(f"Content of {filename}: {content}")
async def main():
await asyncio.gather(
read_file('file1.txt'),
read_file('file2.txt'),
read_file('file3.txt')
)
asyncio.run(main())
Best Practices for File I/O
To ensure efficient and reliable file I/O operations, consider the following best practices:
- Always close files: Failing to close files can lead to resource leaks. Use language constructs like Python’s
with
statement or Java’s try-with-resources to automatically handle file closing. - Handle exceptions: File operations can fail for various reasons (e.g., file not found, permission denied). Always include proper exception handling.
- Use appropriate buffer sizes: When working with buffered I/O, choose an appropriate buffer size based on your application’s needs and the characteristics of the data being processed.
- Consider file locking: In multi-threaded or multi-process applications, use file locking mechanisms to prevent concurrent access issues.
- Validate input: When writing user input to files, always validate and sanitize the input to prevent security vulnerabilities.
- Use appropriate file modes: Choose the correct file mode (read, write, append, etc.) based on your specific requirements to avoid unintended data loss or corruption.
- Be mindful of performance: For large files or frequent I/O operations, consider using techniques like buffering, memory mapping, or asynchronous I/O to improve performance.
Common File I/O Challenges and Solutions
As you work with file I/O, you may encounter several common challenges. Here are some solutions to these issues:
1. Dealing with Large Files
When working with very large files, reading the entire file into memory may not be feasible. Instead, process the file in chunks:
def process_large_file(filename):
chunk_size = 1024 # 1 KB chunks
with open(filename, 'rb') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
# Process the chunk here
process_chunk(chunk)
2. Cross-Platform Compatibility
Different operating systems use different line endings. Use universal newline mode in Python or appropriate libraries in other languages to handle this:
with open('example.txt', 'r', newline=None) as file:
content = file.read()
3. Encoding Issues
When dealing with text files, always specify the correct encoding to avoid character encoding problems:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
4. Concurrent Access
Use file locking mechanisms to handle concurrent access in multi-threaded or multi-process applications:
import fcntl
def lock_file(file):
fcntl.flock(file.fileno(), fcntl.LOCK_EX)
def unlock_file(file):
fcntl.flock(file.fileno(), fcntl.LOCK_UN)
with open('example.txt', 'r+') as file:
lock_file(file)
# Perform operations on the file
unlock_file(file)
File I/O in Real-World Applications
Understanding file I/O is crucial for many real-world applications. Here are some examples of how file I/O is used in various domains:
1. Log Management Systems
Log management systems heavily rely on file I/O for writing log entries and reading logs for analysis. They often need to handle large volumes of data efficiently.
2. Database Systems
While databases abstract away much of the low-level file operations, understanding file I/O is crucial for database developers, especially when optimizing performance or implementing custom storage engines.
3. Content Management Systems (CMS)
CMS platforms use file I/O for managing media files, caching, and storing configuration data.
4. Data Processing Pipelines
ETL (Extract, Transform, Load) processes and data processing pipelines often involve reading data from files, processing it, and writing results to other files.
5. Version Control Systems
Version control systems like Git use sophisticated file I/O operations to manage file versions, merges, and diffs efficiently.
Conclusion
File input and output operations are a fundamental aspect of programming that every developer should master. From basic reading and writing operations to advanced concepts like memory-mapped files and asynchronous I/O, understanding file I/O opens up a world of possibilities for data persistence, processing, and exchange.
As you continue to develop your programming skills, make sure to practice file I/O operations in various scenarios. Experiment with different file formats, large datasets, and concurrent access situations. Remember to always follow best practices, handle exceptions properly, and consider performance implications when working with files.
By mastering file I/O, you’ll be better equipped to tackle a wide range of programming challenges and build more robust, efficient applications. Whether you’re developing a simple script or a complex distributed system, the ability to effectively manage file operations will be an invaluable skill in your programming toolkit.