GraphQL: A Powerful Alternative to REST for Building Flexible APIs
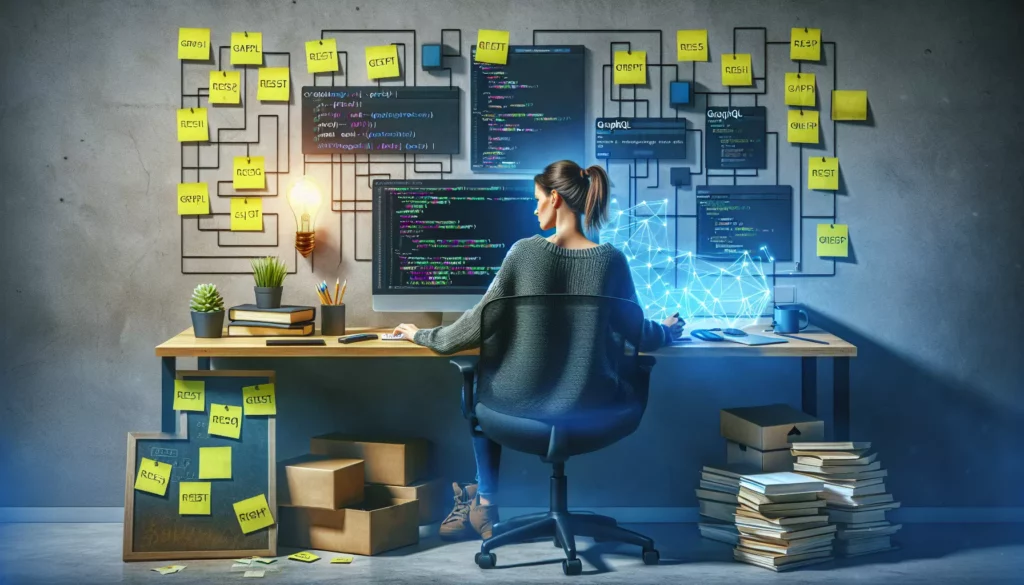
In the ever-evolving landscape of web development, APIs (Application Programming Interfaces) play a crucial role in enabling communication between different software systems. For years, REST (Representational State Transfer) has been the go-to architectural style for designing APIs. However, a new player has emerged on the scene, offering a more flexible and efficient approach to data querying: GraphQL.
In this comprehensive guide, we’ll dive deep into GraphQL, exploring its features, benefits, and how it compares to REST. Whether you’re a seasoned developer or just starting your coding journey, understanding GraphQL can significantly enhance your ability to build modern, efficient APIs.
Table of Contents
- What is GraphQL?
- GraphQL vs. REST: A Comparison
- Key Features of GraphQL
- How GraphQL Works
- Advantages of Using GraphQL
- Challenges and Considerations
- Getting Started with GraphQL
- Best Practices for GraphQL Implementation
- Real-World Examples of GraphQL in Action
- The Future of GraphQL
1. What is GraphQL?
GraphQL is a query language and runtime for APIs that was developed by Facebook in 2012 and open-sourced in 2015. It provides a more efficient, powerful, and flexible alternative to REST. With GraphQL, clients can request exactly the data they need, and nothing more, allowing for more precise and efficient data fetching.
At its core, GraphQL operates on a few key principles:
- Declarative Data Fetching: Clients specify exactly what data they need in their queries.
- Hierarchical: GraphQL queries mirror the shape of the data they return, making it intuitive to work with nested information.
- Strongly Typed: GraphQL APIs are built on a strong type system, enhancing reliability and providing clear contracts between client and server.
- Protocol, Not Storage: GraphQL is transport-layer agnostic and can work with any backend database or service.
2. GraphQL vs. REST: A Comparison
To understand the value proposition of GraphQL, it’s helpful to compare it with REST, the traditional approach to API design:
Data Fetching
- REST: Typically requires multiple endpoints for different resources. Clients often have to make several requests to gather all needed data.
- GraphQL: Uses a single endpoint. Clients can request multiple resources in a single query, reducing network overhead.
Over-fetching and Under-fetching
- REST: Often returns more data than needed (over-fetching) or requires additional requests for related data (under-fetching).
- GraphQL: Clients specify exactly what they need, eliminating over-fetching and reducing under-fetching.
Versioning
- REST: Often requires versioning of endpoints as the API evolves.
- GraphQL: Can add new fields and types without impacting existing queries, reducing the need for versioning.
Documentation
- REST: Requires external tools for documentation, which can become outdated.
- GraphQL: Provides built-in introspection, allowing for always up-to-date documentation.
3. Key Features of GraphQL
GraphQL comes with a rich set of features that make it a powerful tool for API development:
3.1 Schema Definition Language (SDL)
GraphQL uses a strong type system to define the schema of an API. This schema serves as a contract between the client and the server, describing the functionality available to the client.
Here’s an example of a simple GraphQL schema:
type Query {
user(id: ID!): User
}
type User {
id: ID!
name: String!
email: String!
posts: [Post!]!
}
type Post {
id: ID!
title: String!
content: String!
author: User!
}
3.2 Queries
Queries in GraphQL allow clients to request specific data. They resemble the structure of the data being returned.
Example query:
query {
user(id: "123") {
name
email
posts {
title
}
}
}
3.3 Mutations
Mutations are used to modify server-side data. They are similar to queries but are used for write operations.
Example mutation:
mutation {
createPost(title: "Hello GraphQL", content: "This is my first post") {
id
title
}
}
3.4 Subscriptions
Subscriptions allow clients to receive real-time updates when specific events occur on the server.
Example subscription:
subscription {
newPost {
id
title
author {
name
}
}
}
4. How GraphQL Works
Understanding the inner workings of GraphQL can help developers leverage its full potential:
4.1 Request Processing
- Client sends a query: The client formulates a GraphQL query specifying the desired data.
- Server receives the query: The GraphQL server parses and validates the query against the schema.
- Resolvers are executed: The server calls resolver functions for each field in the query.
- Data is collected: Resolvers fetch data from various sources (databases, APIs, etc.).
- Response is constructed: The server assembles the data into the shape specified by the query.
- Response is sent back: The client receives exactly the data it requested.
4.2 Resolvers
Resolvers are functions that define how to fetch the data for each field in your schema. They are the bridge between the GraphQL operation and your data source.
Example resolver:
const resolvers = {
Query: {
user: (parent, args, context, info) => {
return database.getUserById(args.id);
},
},
User: {
posts: (parent, args, context, info) => {
return database.getPostsByUserId(parent.id);
},
},
};
5. Advantages of Using GraphQL
GraphQL offers numerous benefits that make it an attractive choice for API development:
5.1 Efficient Data Loading
By allowing clients to request exactly what they need, GraphQL eliminates over-fetching and reduces under-fetching, leading to more efficient network usage and faster load times.
5.2 Flexible Data Querying
Clients have the power to ask for precisely the data they need, which is particularly useful for applications with diverse data requirements or those supporting multiple platforms.
5.3 Strong Typing and Introspection
The GraphQL type system provides clear contracts between client and server, reducing errors and enabling powerful developer tools through introspection.
5.4 Easier Evolution of APIs
New fields and types can be added to the GraphQL API without impacting existing queries, making it easier to evolve the API over time.
5.5 Improved Developer Experience
GraphQL’s clear and predictable specification, along with excellent tooling support, can significantly enhance developer productivity and satisfaction.
6. Challenges and Considerations
While GraphQL offers many advantages, it’s important to be aware of potential challenges:
6.1 Learning Curve
For teams accustomed to REST, there may be a learning curve in adopting GraphQL concepts and best practices.
6.2 Complexity in Caching
The flexibility of GraphQL queries can make caching more complex compared to REST endpoints.
6.3 Performance Concerns
Without proper constraints, clients could potentially construct very complex queries that may impact server performance.
6.4 Security Considerations
The power given to clients to query data as they see fit requires careful consideration of security measures to prevent abuse.
7. Getting Started with GraphQL
To begin working with GraphQL, follow these steps:
7.1 Choose a GraphQL Server
There are several GraphQL server implementations available for different programming languages. Some popular options include:
- Apollo Server (JavaScript)
- GraphQL-Java (Java)
- Graphene (Python)
- Absinthe (Elixir)
7.2 Define Your Schema
Start by defining your GraphQL schema using the Schema Definition Language (SDL). This will outline the types, queries, mutations, and subscriptions available in your API.
7.3 Implement Resolvers
Create resolver functions for each field in your schema. These functions will determine how to fetch or compute the data for each field.
7.4 Set Up a GraphQL Client
For the client-side, you can use libraries like Apollo Client (for JavaScript) or Relay (for React applications) to interact with your GraphQL API.
7.5 Write Your First Query
With your server and client set up, you can start writing GraphQL queries to fetch data from your API.
8. Best Practices for GraphQL Implementation
To make the most of GraphQL, consider these best practices:
8.1 Design Your Schema Thoughtfully
Your schema is the foundation of your GraphQL API. Spend time designing it to accurately represent your domain and anticipate future needs.
8.2 Use Fragments for Reusable Queries
Fragments allow you to define reusable pieces of queries, promoting code reuse and maintainability.
8.3 Implement Pagination
For large datasets, implement pagination to limit the amount of data returned in a single request.
8.4 Leverage Batching and Caching
Use techniques like DataLoader to batch and cache database queries, improving performance.
8.5 Monitor and Optimize Performance
Use tools like Apollo Engine to monitor query performance and identify areas for optimization.
9. Real-World Examples of GraphQL in Action
Many prominent companies have adopted GraphQL for their APIs. Here are a few examples:
9.1 GitHub
GitHub’s API v4 is built entirely on GraphQL, allowing developers to create more precise and efficient integrations with the platform.
9.2 Facebook
As the creators of GraphQL, Facebook uses it extensively in their mobile apps to efficiently load complex, interconnected data.
9.3 Shopify
Shopify’s Admin API and Storefront API both use GraphQL, providing developers with flexible tools to build e-commerce applications.
9.4 Airbnb
Airbnb uses GraphQL to power its search functionality, allowing for complex, multi-faceted queries.
10. The Future of GraphQL
As GraphQL continues to gain adoption, several trends and developments are shaping its future:
10.1 Increased Adoption
More companies are likely to adopt GraphQL as its benefits become widely recognized and tooling improves.
10.2 Enhanced Tooling
Expect to see continued improvement in GraphQL development tools, IDEs, and testing frameworks.
10.3 Serverless and Edge Computing
GraphQL is well-suited for serverless architectures and edge computing scenarios, areas likely to see growth.
10.4 Federation and Microservices
GraphQL federation, allowing for the composition of multiple GraphQL services into a single graph, is likely to become more prevalent in microservices architectures.
10.5 AI and Machine Learning Integration
As AI becomes more integrated into applications, GraphQL’s flexibility may prove valuable in querying and manipulating AI-generated data.
Conclusion
GraphQL represents a significant evolution in API design, offering developers a more flexible and efficient way to query and manipulate data. Its ability to solve common issues with REST APIs, such as over-fetching and under-fetching of data, makes it an attractive option for modern web and mobile applications.
While GraphQL does come with its own set of challenges, particularly around caching and potential performance issues with complex queries, its benefits often outweigh these concerns. The strong typing, introspection capabilities, and the ability to evolve APIs without versioning are particularly valuable in today’s fast-paced development environments.
As you continue your journey in software development, whether you’re just starting out or you’re an experienced developer looking to expand your skillset, understanding and mastering GraphQL can be a valuable addition to your toolkit. It aligns well with modern development practices and can significantly enhance your ability to build efficient, flexible, and powerful APIs.
Remember, like any technology, the key to success with GraphQL lies in understanding not just how to use it, but when and why to use it. As you gain experience with GraphQL, you’ll develop a better sense of when it’s the right tool for the job and how to leverage its strengths to build better software.
Happy coding, and may your queries be ever efficient!