Graph Traversal Techniques: BFS and DFS Explained
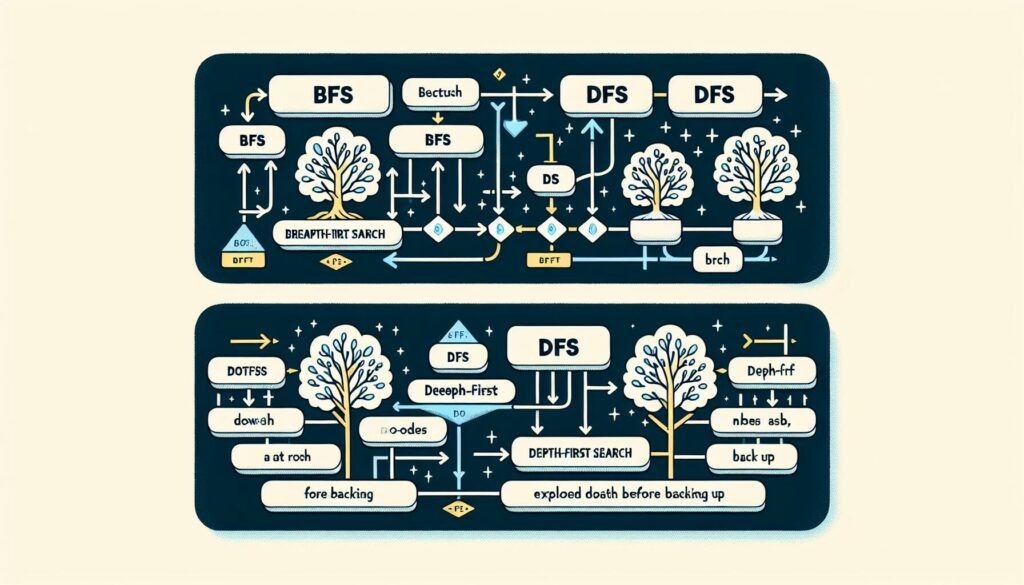
Graph traversal is a fundamental concept in computer science and a crucial skill for any programmer to master. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your problem-solving abilities, understanding graph traversal techniques is essential. In this comprehensive guide, we’ll dive deep into two of the most important graph traversal algorithms: Breadth-First Search (BFS) and Depth-First Search (DFS).
What is Graph Traversal?
Before we delve into the specific algorithms, let’s first understand what graph traversal means. Graph traversal, also known as graph search, is the process of visiting (checking and/or updating) each vertex in a graph. The order in which the vertices are visited defines the traversal technique.
Graphs are versatile data structures used to represent complex relationships between objects. They consist of vertices (also called nodes) and edges that connect these vertices. Graphs can be found in various real-world applications, such as:
- Social networks (where users are vertices and connections are edges)
- Road networks (where intersections are vertices and roads are edges)
- Computer networks (where devices are vertices and connections are edges)
- Web page linking structures (where pages are vertices and hyperlinks are edges)
Now that we understand the importance of graphs, let’s explore the two primary graph traversal techniques: BFS and DFS.
Breadth-First Search (BFS)
Breadth-First Search is a graph traversal algorithm that explores a graph level by level. It starts at a chosen source vertex and explores all the neighboring vertices at the present depth before moving on to the vertices at the next depth level.
How BFS Works
- Start by choosing a source vertex.
- Visit the source vertex and mark it as visited.
- Enqueue the source vertex into a queue.
- While the queue is not empty:
- Dequeue a vertex from the queue.
- Explore all the adjacent vertices of the dequeued vertex.
- For each unvisited adjacent vertex:
- Mark it as visited.
- Enqueue it into the queue.
- Repeat step 4 until the queue is empty.
BFS Implementation in Python
Here’s a Python implementation of the BFS algorithm:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal starting from vertex 'A':")
bfs(graph, 'A')
This implementation uses a queue (implemented using Python’s deque
for efficiency) to keep track of vertices to be visited. The visited
set ensures that each vertex is visited only once.
Time and Space Complexity of BFS
The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because in the worst case, we need to visit all vertices and edges.
The space complexity is O(V), as in the worst case, all vertices might be in the queue simultaneously.
Applications of BFS
- Shortest path in an unweighted graph
- Web crawlers
- Social networking features (e.g., finding people within a certain degree of connection)
- GPS navigation systems
- Puzzle solving
Depth-First Search (DFS)
Depth-First Search is another graph traversal algorithm that explores as far as possible along each branch before backtracking. Unlike BFS, which explores breadth-wise, DFS goes deep into the graph structure before backtracking.
How DFS Works
- Start by choosing a source vertex.
- Visit the source vertex and mark it as visited.
- Recursively visit any unvisited adjacent vertex.
- If all adjacent vertices have been visited, backtrack to the previous vertex.
- Repeat steps 3-4 until all vertices have been visited.
DFS Implementation in Python
Here’s a Python implementation of the DFS algorithm using recursion:
def dfs(graph, vertex, visited=None):
if visited is None:
visited = set()
visited.add(vertex)
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal starting from vertex 'A':")
dfs(graph, 'A')
This implementation uses recursion to traverse the graph. The visited
set keeps track of vertices that have been visited to avoid cycles and repeated visits.
Time and Space Complexity of DFS
The time complexity of DFS is also O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because we visit each vertex and explore each edge once.
The space complexity is O(V) in the worst case, due to the recursion stack. In a skewed graph, the recursion depth could be equal to the number of vertices.
Applications of DFS
- Cycle detection in graphs
- Topological sorting
- Solving maze puzzles
- Finding connected components in a graph
- Generating game trees (e.g., for chess or tic-tac-toe)
BFS vs DFS: When to Use Which?
Both BFS and DFS have their strengths and are suited for different types of problems. Here’s a comparison to help you choose the right algorithm for your needs:
Use BFS when:
- You need to find the shortest path in an unweighted graph
- You’re searching for something that’s likely to be closer to the starting point
- The graph is very wide rather than deep
- You need to visit nodes in order of their distance from the start
Use DFS when:
- You need to search the entire graph (e.g., for connected components)
- You’re solving maze-like puzzles
- You need to perform topological sorting
- The solution is known to be far from the root
- The graph is very deep and solutions are rare
Advanced Graph Traversal Techniques
While BFS and DFS form the foundation of graph traversal, there are several advanced techniques and variations that build upon these basic algorithms. Let’s explore some of them:
1. Bidirectional Search
Bidirectional search is an optimization technique used to find the shortest path between a source and destination node. It runs two simultaneous BFS searches: one forward from the source and one backward from the destination. The search terminates when the two searches meet in the middle.
2. Iterative Deepening Depth-First Search (IDDFS)
IDDFS is a state space/graph search strategy that combines depth-first search’s space-efficiency and breadth-first search’s completeness. It repeatedly performs depth-limited searches, increasing the depth limit with each iteration until the goal is found.
3. A* Search Algorithm
A* is a best-first search algorithm that finds the least-cost path from a given initial node to a goal node. It uses a heuristic function to estimate the cost from any vertex to the goal, which guides the search towards the most promising paths.
4. Dijkstra’s Algorithm
Dijkstra’s algorithm is used to find the shortest path between nodes in a graph with non-negative edge weights. It’s essentially a more sophisticated version of BFS that takes edge weights into account.
Practical Applications of Graph Traversal
Understanding graph traversal techniques is crucial for solving a wide range of real-world problems. Here are some practical applications:
1. Social Network Analysis
Both BFS and DFS can be used to analyze social networks. For example, BFS can be used to find the shortest chain of connections between two users, while DFS can be used to explore deep relationships or find all users within a certain number of connections.
2. Web Crawling
Web crawlers use graph traversal techniques to navigate through web pages. BFS is often preferred as it allows the crawler to explore pages level by level, which is useful for indexing purposes.
3. GPS Navigation
Graph traversal algorithms, particularly those that consider edge weights (like Dijkstra’s algorithm), are fundamental to GPS navigation systems for finding the shortest or fastest route between two points.
4. Network Routing Protocols
Routing protocols in computer networks often use graph traversal techniques to find the most efficient path for data packets to travel from source to destination.
5. Recommendation Systems
Graph-based recommendation systems use traversal techniques to explore relationships between users and items to generate personalized recommendations.
Optimizing Graph Traversal Algorithms
As you work with larger and more complex graphs, optimizing your traversal algorithms becomes crucial. Here are some tips to improve the performance of your BFS and DFS implementations:
1. Use Appropriate Data Structures
Choose the right data structures for your specific use case. For example, using a queue implemented with a deque in Python for BFS can provide better performance than a regular list.
2. Avoid Unnecessary Visits
Keep track of visited nodes efficiently. Using a set for visited nodes in Python provides O(1) lookup time, which is faster than checking a list.
3. Consider Iterative Implementations
While recursive implementations of DFS are elegant, they can lead to stack overflow errors for very deep graphs. An iterative implementation using a stack can be more robust for large graphs.
4. Use Adjacency Lists for Sparse Graphs
For sparse graphs (graphs with relatively few edges), using an adjacency list representation can be more memory-efficient than an adjacency matrix.
5. Parallelize When Possible
For very large graphs, consider parallelizing your traversal algorithms. This can significantly speed up processing, especially for independent subgraphs.
Common Interview Questions on Graph Traversal
Graph traversal is a popular topic in technical interviews, especially for roles at major tech companies. Here are some common interview questions related to BFS and DFS:
- Implement BFS and DFS for a given graph.
- Find the shortest path between two nodes in an unweighted graph.
- Detect a cycle in a directed graph.
- Implement topological sorting of a directed acyclic graph (DAG).
- Find all connected components in an undirected graph.
- Determine if a path exists between two vertices in a graph.
- Find the number of islands in a 2D grid (where 1 represents land and 0 represents water).
- Implement a word ladder problem (transforming one word to another by changing one letter at a time).
- Find the shortest distance from a guard to all rooms in a 2D grid (multi-source BFS).
- Implement a graph coloring algorithm.
To excel in these interviews, it’s crucial to not only understand the algorithms but also be able to implement them efficiently and explain your thought process clearly.
Conclusion
Graph traversal techniques like BFS and DFS are fundamental algorithms in computer science with wide-ranging applications. Whether you’re preparing for technical interviews, working on a complex software project, or simply looking to enhance your problem-solving skills, a solid understanding of these algorithms is invaluable.
Remember, the key to mastering these techniques is practice. Implement these algorithms from scratch, solve various graph problems, and try to apply them to real-world scenarios. As you gain more experience, you’ll develop an intuition for when to use each algorithm and how to optimize them for different types of graphs and problems.
Keep exploring, keep coding, and happy graph traversing!