Graph Theory Simplified for Interviews: A Comprehensive Guide
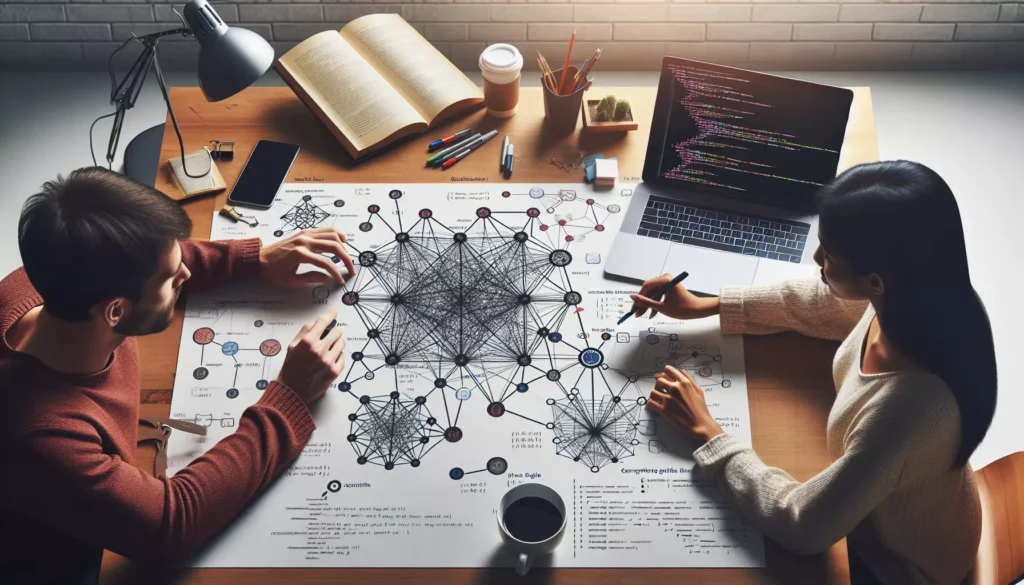
Welcome to our comprehensive guide on Graph Theory, tailored specifically for technical interviews. If you’re preparing for coding interviews, especially with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding graph theory is crucial. This guide will break down complex concepts into digestible chunks, providing you with the knowledge and confidence to tackle graph-related problems in your upcoming interviews.
Table of Contents
- Introduction to Graph Theory
- Graph Representation
- Graph Traversal Algorithms
- Shortest Path Algorithms
- Minimum Spanning Tree
- Topological Sorting
- Advanced Graph Concepts
- Practice Problems and Solutions
- Interview Tips for Graph Problems
- Conclusion
1. Introduction to Graph Theory
Graph theory is a fundamental concept in computer science and mathematics that deals with the study of graphs. In the context of coding interviews, a graph is a data structure consisting of a finite set of vertices (or nodes) and a set of edges that connect these vertices.
Key Concepts:
- Vertex (Node): A fundamental unit of which graphs are formed.
- Edge: A connection between two vertices in a graph.
- Directed Graph (Digraph): A graph where edges have directions.
- Undirected Graph: A graph where edges do not have directions.
- Weighted Graph: A graph where each edge has an associated weight or cost.
- Cyclic Graph: A graph containing at least one cycle.
- Acyclic Graph: A graph with no cycles.
Understanding these basic concepts is crucial as they form the foundation for more complex graph algorithms and problems you might encounter in interviews.
2. Graph Representation
In coding interviews, you’ll often need to implement graphs. There are two primary ways to represent graphs in code:
Adjacency Matrix
An adjacency matrix is a 2D array where matrix[i][j] represents an edge from vertex i to vertex j. This representation is efficient for dense graphs but can be memory-intensive for sparse graphs.
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_matrix = [[0] * num_vertices for _ in range(num_vertices)]
def add_edge(self, v1, v2):
self.adj_matrix[v1][v2] = 1
self.adj_matrix[v2][v1] = 1 # For undirected graph
Adjacency List
An adjacency list uses a list (or dictionary) where each index (or key) represents a vertex, and the corresponding value is a list of vertices adjacent to it. This representation is more space-efficient for sparse graphs.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, v1, v2):
self.graph[v1].append(v2)
self.graph[v2].append(v1) # For undirected graph
Choosing the right representation depends on the problem at hand and the operations you need to perform on the graph.
3. Graph Traversal Algorithms
Graph traversal is the process of visiting all the vertices in a graph. The two primary traversal algorithms are Depth-First Search (DFS) and Breadth-First Search (BFS).
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It’s often implemented using recursion or a stack.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
Breadth-First Search (BFS)
BFS explores all vertices at the present depth before moving to vertices at the next depth level. It’s typically implemented using a queue.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
Both DFS and BFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges.
4. Shortest Path Algorithms
Finding the shortest path between vertices is a common graph problem in interviews. Here are two important algorithms:
Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path from a starting node to all other nodes in a weighted graph with non-negative edge weights.
import heapq
def dijkstra(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_vertex = heapq.heappop(pq)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
Bellman-Ford Algorithm
The Bellman-Ford algorithm can handle graphs with negative edge weights and detect negative cycles.
def bellman_ford(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
for _ in range(len(graph) - 1):
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distances[vertex] + weight < distances[neighbor]:
distances[neighbor] = distances[vertex] + weight
# Check for negative cycles
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distances[vertex] + weight < distances[neighbor]:
print("Graph contains a negative cycle")
return None
return distances
5. Minimum Spanning Tree
A Minimum Spanning Tree (MST) is a subset of edges in a weighted, undirected graph that connects all vertices together with the minimum possible total edge weight. Two popular algorithms for finding MSTs are:
Kruskal’s Algorithm
Kruskal’s algorithm builds the MST by adding edges in order of increasing weight, skipping edges that would create a cycle.
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal(graph):
edges = [(w, u, v) for u in graph for v, w in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
ds = DisjointSet(vertices)
mst = []
for w, u, v in edges:
if ds.find(u) != ds.find(v):
ds.union(u, v)
mst.append((u, v, w))
return mst
Prim’s Algorithm
Prim’s algorithm starts with a single vertex and grows the MST one edge at a time, always adding the lowest-weight edge that connects a vertex in the MST to a vertex outside the MST.
import heapq
def prim(graph):
start_vertex = next(iter(graph))
mst = []
visited = set([start_vertex])
edges = [(w, start_vertex, v) for v, w in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
w, u, v = heapq.heappop(edges)
if v not in visited:
visited.add(v)
mst.append((u, v, w))
for next_v, next_w in graph[v].items():
if next_v not in visited:
heapq.heappush(edges, (next_w, v, next_v))
return mst
6. Topological Sorting
Topological sorting is used for ordering tasks with dependencies. It’s applicable only to Directed Acyclic Graphs (DAGs). Here’s an implementation using DFS:
from collections import defaultdict
def topological_sort(graph):
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
visited = set()
stack = []
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1]
# Example usage
graph = defaultdict(list)
graph[0].extend([1, 2])
graph[1].append(3)
graph[2].append(3)
graph[3].append(4)
graph[4].append(5)
print(topological_sort(graph)) # Output: [0, 2, 1, 3, 4, 5]
7. Advanced Graph Concepts
As you progress in your interview preparation, you may encounter more advanced graph concepts. Here are a few you should be familiar with:
Strongly Connected Components (SCCs)
SCCs are subgraphs where every vertex is reachable from every other vertex. Kosaraju’s algorithm is commonly used to find SCCs:
from collections import defaultdict
def kosaraju(graph):
def dfs(v, visited, stack):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor, visited, stack)
stack.append(v)
def reverse_graph(graph):
reversed_graph = defaultdict(list)
for v in graph:
for neighbor in graph[v]:
reversed_graph[neighbor].append(v)
return reversed_graph
def dfs_scc(v, visited, scc):
visited.add(v)
scc.append(v)
for neighbor in reversed_graph[v]:
if neighbor not in visited:
dfs_scc(neighbor, visited, scc)
stack = []
visited = set()
for v in graph:
if v not in visited:
dfs(v, visited, stack)
reversed_graph = reverse_graph(graph)
visited.clear()
sccs = []
while stack:
v = stack.pop()
if v not in visited:
scc = []
dfs_scc(v, visited, scc)
sccs.append(scc)
return sccs
Articulation Points and Bridges
Articulation points (or cut vertices) are vertices whose removal increases the number of connected components in a graph. Bridges are edges whose removal does the same. These concepts are important in network reliability.
Network Flow
Network flow problems involve finding the maximum flow through a flow network. The Ford-Fulkerson algorithm and its improved version, the Edmonds-Karp algorithm, are commonly used for solving max flow problems.
8. Practice Problems and Solutions
To solidify your understanding of graph theory, it’s crucial to practice with real interview-style problems. Here are a few examples with solutions:
Problem 1: Number of Islands (LeetCode 200)
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), count the number of islands.
def numIslands(grid):
if not grid:
return 0
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == '1':
dfs(grid, i, j)
count += 1
return count
def dfs(grid, i, j):
if i<0 or j<0 or i>=len(grid) or j>=len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '0'
dfs(grid, i+1, j)
dfs(grid, i-1, j)
dfs(grid, i, j+1)
dfs(grid, i, j-1)
Problem 2: Course Schedule (LeetCode 207)
Problem: There are a total of n courses you have to take, labeled from 0 to n-1. Some courses may have prerequisites. Determine if it is possible to finish all courses.
from collections import defaultdict
def canFinish(numCourses, prerequisites):
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
visited = [0] * numCourses
def dfs(course):
if visited[course] == -1:
return False
if visited[course] == 1:
return True
visited[course] = -1
for prereq in graph[course]:
if not dfs(prereq):
return False
visited[course] = 1
return True
for course in range(numCourses):
if not dfs(course):
return False
return True
Problem 3: Network Delay Time (LeetCode 743)
Problem: Given a network of n nodes, labeled from 1 to n. You are given times, a list of travel times as directed edges times[i] = (u, v, w), where u is the source node, v is the target node, and w is the time it takes for a signal to travel from source to target. We will send a signal from a given node k. Return the time it takes for all the n nodes to receive the signal. If it is impossible for all the n nodes to receive the signal, return -1.
import heapq
def networkDelayTime(times, n, k):
graph = defaultdict(list)
for u, v, w in times:
graph[u].append((v, w))
distances = {node: float('inf') for node in range(1, n+1)}
distances[k] = 0
pq = [(0, k)]
while pq:
time, node = heapq.heappop(pq)
if time > distances[node]:
continue
for neighbor, weight in graph[node]:
new_time = time + weight
if new_time < distances[neighbor]:
distances[neighbor] = new_time
heapq.heappush(pq, (new_time, neighbor))
max_time = max(distances.values())
return max_time if max_time < float('inf') else -1
9. Interview Tips for Graph Problems
When tackling graph problems in interviews, keep these tips in mind:
- Clarify the input: Ask about the graph representation (adjacency list, matrix, etc.) and any constraints on the input.
- Consider edge cases: Empty graph, single node, disconnected components, cycles, etc.
- Choose the right algorithm: Based on the problem, decide whether DFS, BFS, or a more specialized algorithm is appropriate.
- Optimize space: Consider using in-place modifications if allowed, to save space.
- Time complexity: Be prepared to discuss the time and space complexity of your solution.
- Test your code: Walk through your code with a small example to catch any bugs.
- Explain your approach: Clearly communicate your thought process and reasoning behind your solution.
10. Conclusion
Graph theory is a vast and crucial topic in computer science, especially for coding interviews. This guide has covered the fundamental concepts, common algorithms, and practical problem-solving techniques related to graphs. Remember, the key to mastering graph problems is consistent practice and a solid understanding of the underlying principles.
As you continue your interview preparation journey, make sure to:
- Regularly practice graph problems from various sources.
- Implement the algorithms from scratch to deepen your understanding.
- Analyze different approaches to solving the same problem.
- Stay updated with new graph-related questions appearing in recent interviews.
With dedication and the knowledge gained from this guide, you’ll be well-equipped to tackle graph problems in your upcoming technical interviews. Good luck with your preparation!