Google Interview Questions: A Comprehensive Guide to Acing Your Technical Interview
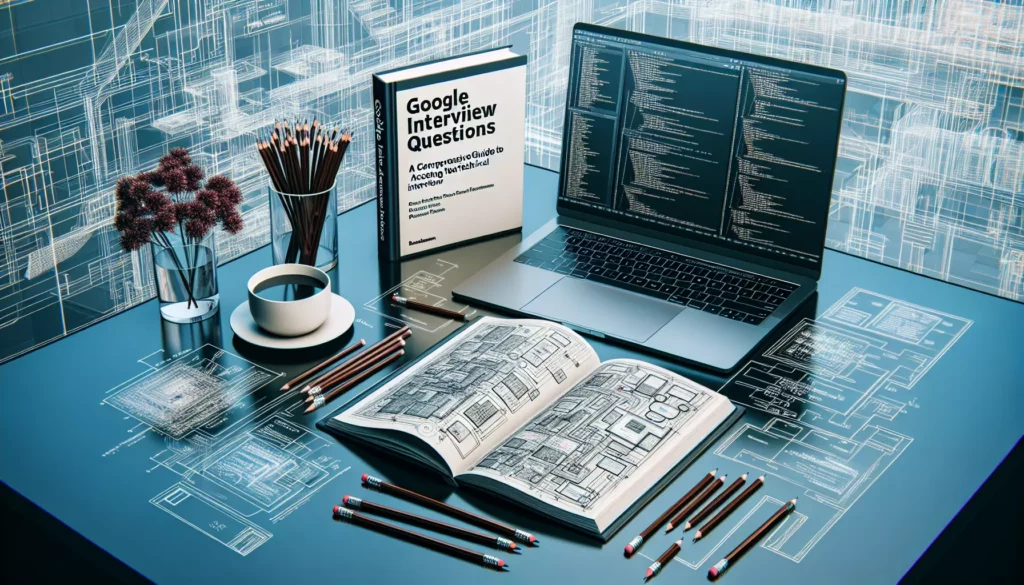
Are you preparing for a Google interview? You’re not alone. Every year, thousands of hopeful candidates vie for positions at one of the world’s most prestigious tech companies. Google is known for its rigorous interview process, particularly when it comes to technical roles. In this comprehensive guide, we’ll dive deep into Google interview questions, providing you with invaluable insights, tips, and strategies to help you succeed.
Table of Contents
- Understanding Google Interviews
- Types of Google Interview Questions
- Common Google Interview Questions
- Coding Challenges
- System Design Questions
- Behavioral Questions
- Tips for Success
- Resources for Preparation
- Conclusion
1. Understanding Google Interviews
Before diving into specific questions, it’s crucial to understand the structure and philosophy behind Google’s interview process. Google’s interviews are designed to assess not just your technical skills, but also your problem-solving abilities, creativity, and cultural fit.
The typical Google interview process includes:
- Phone or video screening
- Online coding assessment
- On-site interviews (4-5 rounds)
- Team matching (for some roles)
Each stage is designed to evaluate different aspects of your abilities and potential fit within the company. Google places a high value on candidates who can think critically, communicate effectively, and demonstrate a passion for technology and innovation.
2. Types of Google Interview Questions
Google interview questions can be broadly categorized into three main types:
- Technical/Coding Questions: These assess your programming skills, algorithmic thinking, and problem-solving abilities.
- System Design Questions: These evaluate your ability to design large-scale systems and understand complex architectural concepts.
- Behavioral Questions: These gauge your soft skills, leadership potential, and cultural fit within Google.
Let’s explore each of these categories in more detail.
3. Common Google Interview Questions
While the specific questions you’ll encounter in a Google interview can vary widely, there are some common themes and problem types that frequently appear. Here are some examples:
Technical Questions:
- Implement a function to reverse a linked list.
- Find the longest palindromic substring in a given string.
- Design an algorithm to detect a cycle in a graph.
- Implement a LRU (Least Recently Used) cache.
- Write a function to find the kth largest element in an unsorted array.
System Design Questions:
- Design a URL shortening service like bit.ly.
- How would you design Google’s search autocomplete feature?
- Design a distributed key-value store.
- How would you implement Google Maps?
- Design a system for real-time chat messaging.
Behavioral Questions:
- Tell me about a time when you had to work with a difficult team member.
- Describe a situation where you had to make a decision with incomplete information.
- How do you stay updated with the latest technology trends?
- Tell me about a project you’re particularly proud of.
- How do you handle criticism or feedback on your work?
4. Coding Challenges
Coding challenges are a crucial part of the Google interview process. These questions are designed to test your ability to write clean, efficient, and correct code under pressure. Let’s look at a sample coding challenge and walk through a solution:
Sample Problem: Two Sum
Problem Statement: Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
# Test the function
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices: {result}")
This solution uses a hash map (dictionary in Python) to achieve O(n) time complexity. It iterates through the array once, checking if the complement (target – current number) exists in the hash map. If it does, we’ve found our pair. If not, we add the current number and its index to the hash map.
Tips for Coding Challenges:
- Clarify the problem: Make sure you understand all requirements and constraints before starting to code.
- Think out loud: Explain your thought process as you work through the problem.
- Consider edge cases: Think about potential edge cases and how your solution handles them.
- Optimize: After implementing a working solution, consider ways to optimize it for better time or space complexity.
- Test your code: Write test cases to verify your solution works correctly.
5. System Design Questions
System design questions are particularly important for senior and lead engineering roles at Google. These questions assess your ability to design scalable, reliable, and efficient systems. Let’s look at a sample system design question:
Sample Problem: Design a URL Shortener
Problem Statement: Design a URL shortening service similar to bit.ly. The service should take a long URL and return a shortened URL, redirecting users to the original URL when the short URL is accessed.
High-level approach:
- API Design:
- POST /shorten – Accept a long URL, return a short URL
- GET /{short_code} – Redirect to the original long URL
- URL Shortening Algorithm:
- Generate a unique short code for each URL
- Options: MD5 hash, base62 encoding, or counter-based approach
- Data Storage:
- Use a database to store mappings of short codes to long URLs
- Consider using a cache for frequently accessed URLs
- Scalability Considerations:
- Use a load balancer to distribute traffic
- Implement database sharding for horizontal scaling
- Use a distributed cache (e.g., Redis) for better performance
- Analytics and Monitoring:
- Track click statistics and implement rate limiting
- Set up monitoring and alerting for system health
Tips for System Design Questions:
- Clarify requirements: Ask questions to understand the scale, features, and constraints of the system.
- Start with a high-level design: Sketch out the main components before diving into details.
- Consider scalability: Discuss how your design would handle increasing load and data volume.
- Think about trade-offs: Be prepared to discuss pros and cons of different design choices.
- Cover all aspects: Don’t forget about security, monitoring, and maintenance of the system.
6. Behavioral Questions
Behavioral questions are an essential part of Google interviews, as they help assess your soft skills, leadership potential, and cultural fit. Google looks for candidates who embody their core values and can work effectively in their collaborative environment.
Sample Behavioral Questions:
- Tell me about a time when you had to deal with a team conflict. How did you handle it?
- Describe a situation where you had to make a difficult decision with limited information.
- How do you stay motivated when working on challenging, long-term projects?
- Give an example of a time when you failed at something. What did you learn from it?
- How do you approach learning new technologies or skills?
Tips for Behavioral Questions:
- Use the STAR method: Structure your answers using the Situation, Task, Action, Result format.
- Be specific: Provide concrete examples rather than general statements.
- Highlight your role: Clearly explain your contribution and impact in each situation.
- Show growth: Demonstrate how you’ve learned and improved from your experiences.
- Align with Google’s values: Familiarize yourself with Google’s culture and values, and show how you embody them.
7. Tips for Success
To increase your chances of success in a Google interview, consider the following tips:
- Practice, practice, practice: Regularly solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Study core computer science concepts: Review data structures, algorithms, and system design principles.
- Mock interviews: Conduct mock interviews with friends or use services like Pramp to get comfortable with the interview format.
- Time management: Practice solving problems within time constraints to improve your efficiency.
- Communicate clearly: Explain your thought process clearly and concisely during the interview.
- Ask questions: Don’t hesitate to ask for clarification or discuss trade-offs with your interviewer.
- Stay calm: Remember that it’s okay to take a moment to think before answering questions.
- Show enthusiasm: Demonstrate your passion for technology and your interest in working at Google.
8. Resources for Preparation
To help you prepare for your Google interview, consider using the following resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Designing Data-Intensive Applications” by Martin Kleppmann
- Online Platforms:
- LeetCode
- HackerRank
- AlgoCademy
- Pramp
- Courses:
- Coursera’s “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare’s “Introduction to Algorithms”
- YouTube Channels:
- Back To Back SWE
- Tech Dummies Narendra L
- Gaurav Sen
9. Conclusion
Preparing for a Google interview can be a challenging but rewarding experience. By understanding the types of questions you might encounter, practicing regularly, and honing your problem-solving skills, you can significantly increase your chances of success. Remember that the interview process is not just about getting the right answers, but also about demonstrating your thought process, communication skills, and passion for technology.
As you prepare, make use of resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to mastering advanced algorithms and data structures. With dedication, practice, and the right mindset, you can approach your Google interview with confidence and showcase your best self to the interviewers.
Good luck with your preparation, and may your journey to becoming a Googler be both exciting and successful!