Git Rebase: Streamlining Your Development Workflow
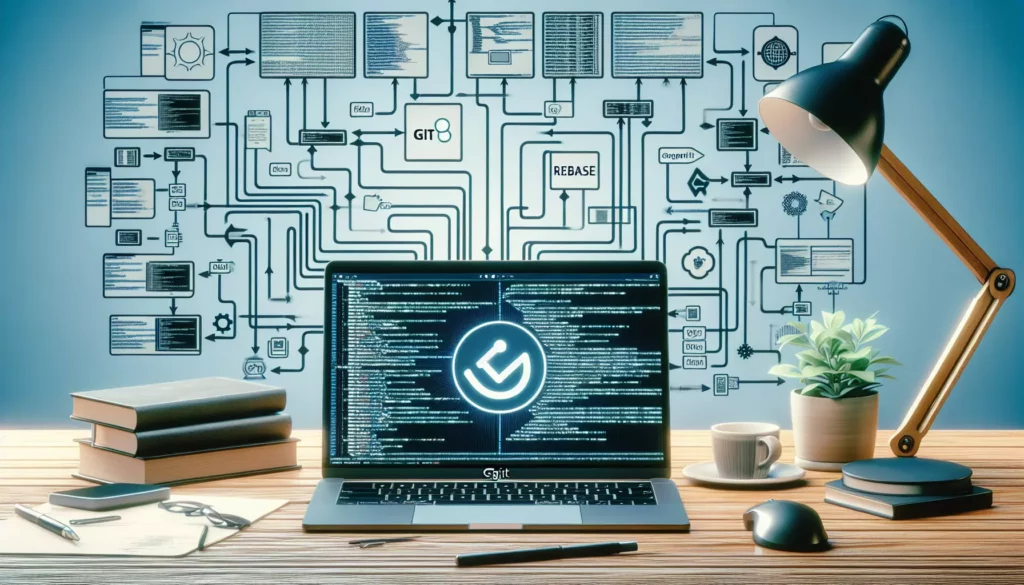
In the world of software development, version control is an essential tool for managing code changes and collaborating with team members. Git, one of the most popular version control systems, offers a powerful feature called “rebase” that can significantly enhance your development workflow. In this comprehensive guide, we’ll explore Git rebase, its benefits, potential pitfalls, and how to use it effectively in your projects.
What is Git Rebase?
Git rebase is a command that allows you to modify the commit history of your project. It essentially “replays” a series of commits on top of another base commit, creating a linear history. This process can be used to integrate changes from one branch into another, clean up commit history, or resolve conflicts before merging.
The basic syntax for Git rebase is:
git rebase <base>
Where <base>
is the branch or commit you want to rebase onto.
Why Use Git Rebase?
There are several reasons why developers choose to use Git rebase:
- Cleaner commit history: Rebase can help create a more linear and organized commit history, making it easier to understand the project’s evolution.
- Easier code reviews: A clean commit history facilitates easier code reviews, as changes are presented in a more logical order.
- Conflict resolution: Rebase allows you to resolve conflicts on a commit-by-commit basis, which can be more manageable than dealing with all conflicts at once during a merge.
- Keeping feature branches up-to-date: Rebasing a feature branch onto the latest main branch ensures that your changes are compatible with the most recent codebase.
Git Rebase vs. Git Merge
To understand the benefits of rebase, it’s helpful to compare it with the more commonly used Git merge command:
Git Merge
When you use Git merge, it creates a new “merge commit” that combines the changes from both branches. This preserves the entire history of both branches but can result in a more complex commit graph.
A---B---C topic
\
D---E---F---G master
# After merge:
A---B---C---H topic, master
\ /
D---E---F---G
Git Rebase
Git rebase, on the other hand, moves the entire feature branch to begin on the tip of the master branch, effectively incorporating all of the new commits. Rebase re-writes the project history by creating new commits for each commit in the original branch.
A---B---C topic
\
D---E---F---G master
# After rebase:
A'--B'--C' topic
/
D---E---F---G master
As you can see, the resulting history is much cleaner and linear.
How to Use Git Rebase
Let’s walk through a typical rebase workflow:
1. Update your local master branch
First, make sure your local master branch is up-to-date with the remote repository:
git checkout master
git pull origin master
2. Start the rebase
Switch to your feature branch and start the rebase:
git checkout feature-branch
git rebase master
3. Resolve conflicts (if any)
If there are any conflicts during the rebase process, Git will pause and allow you to resolve them. After resolving conflicts in a file, stage it and continue the rebase:
git add <conflicted-file>
git rebase --continue
4. Force push to remote (if necessary)
If you’ve already pushed your feature branch to a remote repository, you’ll need to force push the rebased branch:
git push origin feature-branch --force
Note: Be cautious when using force push, especially on shared branches, as it can overwrite the remote history.
Advanced Git Rebase Techniques
Interactive Rebase
Interactive rebase allows you to modify commits as they are replayed onto the new base. This is useful for cleaning up your commit history before merging or submitting a pull request.
To start an interactive rebase:
git rebase -i <base>
This will open your default text editor with a list of commits and actions you can perform on each commit:
pick f7f3f6d Change button color
pick 310154e Update header text
pick a5f4a0d Add new feature
# Rebase 710f0f8..a5f4a0d onto 710f0f8
#
# Commands:
# p, pick <commit> = use commit
# r, reword <commit> = use commit, but edit the commit message
# e, edit <commit> = use commit, but stop for amending
# s, squash <commit> = use commit, but meld into previous commit
# f, fixup <commit> = like "squash", but discard this commit's log message
# x, exec <command> = run command (the rest of the line) using shell
# b, break = stop here (continue rebase later with 'git rebase --continue')
# d, drop <commit> = remove commit
# l, label <label> = label current HEAD with a name
# t, reset <label> = reset HEAD to a label
# m, merge [-C <commit> | -c <commit>] <label> [# <oneline>]
# . create a merge commit using the original merge commit's
# . message (or the oneline, if no original merge commit was
# . specified). Use -c <commit> to reword the commit message.
#
# These lines can be re-ordered; they are executed from top to bottom.
#
# If you remove a line here THAT COMMIT WILL BE LOST.
#
# However, if you remove everything, the rebase will be aborted.
#
# Note that empty commits are commented out
You can then modify this file to change the order of commits, squash multiple commits together, or even drop commits entirely.
Rebasing with Autosquash
When working on a feature branch, you might make small commits to fix typos or add minor changes. Before merging, you can use the autosquash feature to automatically squash these commits into their parent commits.
To use autosquash, first make your fixup commits with the --fixup
option:
git commit --fixup <commit-hash>
Then, when you’re ready to rebase, use the --autosquash
option:
git rebase -i --autosquash <base>
Git will automatically reorder and mark the fixup commits to be squashed into their parent commits.
Best Practices for Git Rebase
While Git rebase is a powerful tool, it’s important to use it responsibly. Here are some best practices to keep in mind:
- Don’t rebase shared branches: Rebasing changes the commit history, which can cause problems for other developers who have based their work on the original branch. As a general rule, don’t rebase branches that other people are working on.
- Communicate with your team: If you do need to rebase a shared branch, make sure to communicate with your team and coordinate the process.
- Use feature branches: Work on features in separate branches and only rebase those branches. This keeps your main branch clean and reduces the risk of conflicts.
- Understand the golden rule of rebasing: Never rebase commits that have been pushed to a public repository unless you’re sure no one else has based their work on those commits.
- Regularly rebase long-running feature branches: If you’re working on a long-running feature branch, periodically rebase it onto the latest main branch to keep it up-to-date and reduce the likelihood of major conflicts later.
- Use interactive rebase to clean up your commit history: Before merging a feature branch, use interactive rebase to clean up your commit history, squashing related commits and ensuring each commit represents a logical unit of work.
- Be cautious with force pushing: When you rebase a branch that has already been pushed to a remote repository, you’ll need to force push. Be very careful when doing this, as it can overwrite changes on the remote branch.
Common Pitfalls and How to Avoid Them
1. Losing commits
If you’re not careful, it’s possible to lose commits during a rebase. To avoid this:
- Always create a backup branch before performing a complex rebase.
- Use
git reflog
to recover lost commits if necessary.
2. Merge conflicts
Rebasing can sometimes lead to numerous merge conflicts, especially if you’re rebasing a long-running feature branch. To minimize this:
- Rebase your feature branch onto the main branch regularly.
- Break large features into smaller, more manageable chunks.
3. Rebasing the wrong branch
Make sure you’re on the correct branch before rebasing. Always double-check your current branch with git branch
before starting a rebase.
4. Force pushing to the wrong branch
When force pushing after a rebase, make absolutely sure you’re pushing to the correct branch. Consider using the --force-with-lease
option instead of --force
for an extra layer of safety.
Git Rebase in Practice: A Real-World Scenario
Let’s walk through a common scenario where Git rebase can be particularly useful:
Imagine you’re working on a feature branch called new-login-page
. You’ve made several commits over the past few days:
A---B---C---D---E new-login-page
\
F---G---H master
Where:
- A: Initial commit for the new login page
- B: Add form validation
- C: Implement password strength meter
- D: Fix typo in error message
- E: Adjust button styling
Meanwhile, the master branch has progressed with commits F, G, and H. You want to incorporate these changes into your feature branch and clean up your commit history before creating a pull request.
Here’s how you can use Git rebase to accomplish this:
Step 1: Update your local master branch
git checkout master
git pull origin master
Step 2: Start an interactive rebase
git checkout new-login-page
git rebase -i master
This will open your text editor with something like this:
pick a1b2c3d Initial commit for the new login page
pick e4f5g6h Add form validation
pick i7j8k9l Implement password strength meter
pick m0n1o2p Fix typo in error message
pick q3r4s5t Adjust button styling
# Rebase 710f0f8..a5f4a0d onto 710f0f8
#
# Commands:
# p, pick <commit> = use commit
# r, reword <commit> = use commit, but edit the commit message
# e, edit <commit> = use commit, but stop for amending
# s, squash <commit> = use commit, but meld into previous commit
# f, fixup <commit> = like "squash", but discard this commit's log message
# x, exec <command> = run command (the rest of the line) using shell
# d, drop <commit> = remove commit
# l, label <label> = label current HEAD with a name
# t, reset <label> = reset HEAD to a label
# m, merge [-C <commit> | -c <commit>] <label> [# <oneline>]
# . create a merge commit using the original merge commit's
# . message (or the oneline, if no original merge commit was
# . specified). Use -c <commit> to reword the commit message.
#
# These lines can be re-ordered; they are executed from top to bottom.
#
# If you remove a line here THAT COMMIT WILL BE LOST.
#
# However, if you remove everything, the rebase will be aborted.
#
# Note that empty commits are commented out
Step 3: Clean up the commit history
Let’s say you want to squash the typo fix and button styling adjustments into their parent commits. You can modify the file like this:
pick a1b2c3d Initial commit for the new login page
pick e4f5g6h Add form validation
pick i7j8k9l Implement password strength meter
f m0n1o2p Fix typo in error message
f q3r4s5t Adjust button styling
Here, we’ve changed “pick” to “f” (fixup) for the last two commits. This will incorporate these changes into their parent commits without keeping the commit messages.
Step 4: Save and close the editor
Git will now apply these changes and rebase your branch onto the latest master.
Step 5: Resolve any conflicts
If there are any conflicts during the rebase process, Git will pause and allow you to resolve them. After resolving conflicts in a file, stage it and continue the rebase:
git add <conflicted-file>
git rebase --continue
Step 6: Force push the changes
Once the rebase is complete, you’ll need to force push your changes to update the remote branch:
git push origin new-login-page --force-with-lease
The --force-with-lease
option is a safer alternative to --force
as it will abort the push if there are any upstream changes that you haven’t incorporated.
Conclusion
Git rebase is a powerful tool that can help you maintain a clean and linear project history. By understanding how to use rebase effectively, you can streamline your development workflow, make code reviews easier, and keep your feature branches up-to-date with the latest changes from the main branch.
However, it’s crucial to use rebase responsibly, especially when working with shared branches. Always communicate with your team, follow best practices, and be cautious when rewriting history that has already been shared.
As you become more comfortable with Git rebase, you’ll find that it’s an invaluable tool in your development toolkit. It allows you to craft a clear and meaningful commit history that accurately reflects the evolution of your project, making it easier for you and your team to understand and maintain your codebase over time.
Remember, like any powerful tool, Git rebase requires practice to master. Don’t be afraid to experiment in a safe environment, such as a personal project or a throwaway branch, to get comfortable with its features and potential pitfalls. With time and experience, you’ll be able to leverage Git rebase to its full potential, enhancing your productivity and the overall quality of your version control practices.