Getting Started with Programming: A Comprehensive Guide for Beginners
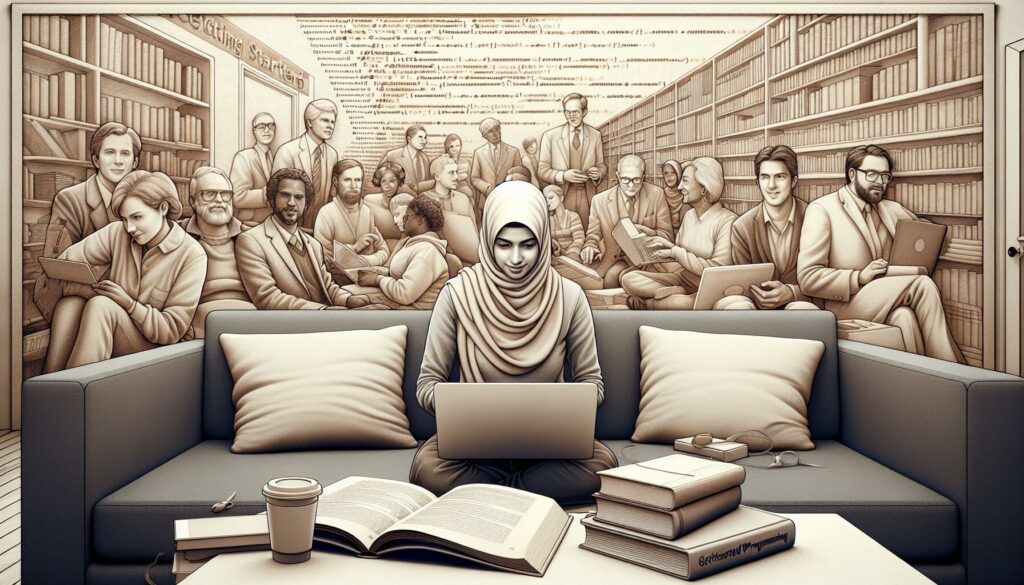
In today’s digital age, programming has become an essential skill that opens up a world of opportunities. Whether you’re looking to start a career in tech, enhance your current job prospects, or simply explore a new hobby, learning to code is an excellent investment of your time and energy. This comprehensive guide will walk you through the basics of getting started with programming, providing you with a solid foundation to build upon.
Why Learn Programming?
Before diving into the how-to’s, let’s briefly explore why programming is such a valuable skill:
- Career Opportunities: The tech industry is booming, with high demand for skilled programmers across various sectors.
- Problem-Solving Skills: Programming teaches you to think logically and approach problems systematically.
- Creativity: Coding allows you to bring your ideas to life, whether it’s building websites, apps, or software.
- Automation: You can create tools to automate repetitive tasks, increasing efficiency in various aspects of life and work.
- Understanding Technology: Learning to code gives you a deeper understanding of the technology that surrounds us.
Choosing Your First Programming Language
One of the first decisions you’ll need to make is which programming language to learn. While there are numerous options, some languages are more beginner-friendly than others. Here are a few popular choices for beginners:
1. Python
Python is widely regarded as one of the best languages for beginners due to its simple, readable syntax and versatility. It’s used in web development, data analysis, artificial intelligence, and more.
2. JavaScript
If you’re interested in web development, JavaScript is an excellent choice. It’s essential for front-end web development and can also be used for back-end development with Node.js.
3. Java
Java is a popular, versatile language used in Android app development, enterprise software, and more. While it has a steeper learning curve than Python, it’s still accessible for beginners.
4. C#
C# (pronounced C-sharp) is a good choice if you’re interested in developing Windows applications or games using the Unity engine.
Remember, the best language to start with is often the one that aligns with your goals and interests. Don’t stress too much about this decision – many programming concepts are transferable between languages, and it’s common for programmers to learn multiple languages throughout their careers.
Setting Up Your Development Environment
Once you’ve chosen a language, you’ll need to set up your development environment. This typically involves installing a text editor or Integrated Development Environment (IDE) and the necessary tools for your chosen language.
Text Editors
Text editors are lightweight applications for writing code. Some popular options include:
- Visual Studio Code (VS Code)
- Sublime Text
- Atom
Integrated Development Environments (IDEs)
IDEs are more comprehensive tools that include features like code completion, debugging, and project management. Some popular IDEs include:
- PyCharm (for Python)
- IntelliJ IDEA (for Java)
- Visual Studio (for C# and other languages)
Installing Your Chosen Language
You’ll also need to install the necessary software for your chosen language. For example:
- For Python: Download and install Python from python.org
- For JavaScript: Install Node.js from nodejs.org
- For Java: Download and install the Java Development Kit (JDK) from oracle.com
Learning the Basics
With your environment set up, it’s time to start learning. Here are some fundamental concepts you’ll encounter in most programming languages:
1. Variables and Data Types
Variables are used to store data in your programs. Different types of data (like numbers, text, or true/false values) are stored in different data types.
Example in Python:
age = 25 # Integer
name = "John" # String
is_student = True # Boolean
2. Control Structures
Control structures allow you to control the flow of your program. The most common are if-else statements and loops.
Example of an if-else statement in Python:
if age >= 18:
print("You are an adult")
else:
print("You are a minor")
Example of a for loop in Python:
for i in range(5):
print(i)
3. Functions
Functions are reusable blocks of code that perform specific tasks. They help organize your code and make it more modular.
Example of a function in Python:
def greet(name):
return f"Hello, {name}!"
message = greet("Alice")
print(message) # Outputs: Hello, Alice!
4. Data Structures
Data structures are ways of organizing and storing data. Common data structures include lists (or arrays), dictionaries (or hash maps), and sets.
Example of a list in Python:
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Outputs: apple
5. Object-Oriented Programming (OOP)
OOP is a programming paradigm that uses objects (instances of classes) to structure code. It’s a more advanced concept but fundamental to many programming languages.
Example of a simple class in Python:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says Woof!"
my_dog = Dog("Buddy")
print(my_dog.bark()) # Outputs: Buddy says Woof!
Learning Resources
There are numerous resources available for learning to code, many of them free. Here are some popular options:
Online Courses and Tutorials
- Codecademy: Offers interactive coding lessons in various languages.
- freeCodeCamp: Provides free coding courses with certifications.
- Coursera and edX: Offer university-level computer science courses.
- YouTube channels like Traversy Media, The Net Ninja, and CS50.
Books
- “Python Crash Course” by Eric Matthes
- “JavaScript: The Good Parts” by Douglas Crockford
- “Head First Java” by Kathy Sierra and Bert Bates
Coding Challenges and Practice
- HackerRank: Offers coding challenges and competitions.
- LeetCode: Provides algorithm challenges often used in technical interviews.
- Project Euler: Offers mathematical/computer programming problems.
Building Projects
One of the best ways to learn programming is by working on projects. Start with small, manageable projects and gradually increase complexity as you gain confidence. Here are some project ideas for beginners:
1. Calculator
Create a simple calculator that can perform basic arithmetic operations. This project will help you practice working with user input, functions, and basic math operations.
2. To-Do List Application
Build a program that allows users to add, remove, and view tasks. This project will introduce you to working with data structures and basic CRUD (Create, Read, Update, Delete) operations.
3. Guess the Number Game
Create a game where the computer generates a random number, and the player tries to guess it. This project will help you practice using random number generation, loops, and conditional statements.
4. Weather App
Build an application that fetches weather data from an API and displays it to the user. This project will introduce you to working with APIs and parsing JSON data.
5. Personal Website
If you’re learning web development, creating a personal website is an excellent project. It will help you practice HTML, CSS, and potentially JavaScript.
Best Practices for Learning Programming
As you embark on your programming journey, keep these best practices in mind:
1. Consistency is Key
Try to code a little bit every day, even if it’s just for 30 minutes. Consistent practice is more effective than sporadic, longer sessions.
2. Learn by Doing
Don’t just read about programming – write code! The more you practice, the more comfortable you’ll become with the concepts.
3. Embrace Errors
Don’t get discouraged by errors in your code. They’re a normal part of programming and an opportunity to learn. Learn to read error messages and use them to debug your code.
4. Use Version Control
Learn to use Git and GitHub. Version control is crucial for tracking changes in your code and collaborating with others.
5. Join a Community
Engage with other learners and experienced programmers. Join coding forums, attend local meetups, or participate in online communities like Stack Overflow.
6. Read Other People’s Code
Reading well-written code can help you learn best practices and new techniques. Explore open-source projects on GitHub to see how experienced developers structure their code.
7. Take Breaks
Programming can be mentally taxing. Remember to take regular breaks to avoid burnout and maintain productivity.
Common Challenges for Beginners
As you start your programming journey, you may encounter some common challenges. Here’s how to overcome them:
1. Information Overload
There’s a vast amount of information available, which can be overwhelming. Focus on mastering the basics before moving on to more advanced topics. Follow a structured curriculum or course to avoid getting lost in the sea of information.
2. Imposter Syndrome
It’s common to feel like you’re not good enough or that you’re falling behind. Remember that everyone starts as a beginner, and learning to code is a journey. Celebrate your small victories and focus on your progress rather than comparing yourself to others.
3. Debugging Frustration
Debugging can be frustrating, especially for beginners. Develop a systematic approach to debugging: read error messages carefully, use print statements to understand what’s happening in your code, and learn to use debugging tools in your IDE.
4. Lack of Problem-Solving Skills
Programming is essentially problem-solving. Improve your problem-solving skills by breaking down complex problems into smaller, manageable parts. Practice algorithmic thinking and work on coding challenges regularly.
5. Difficulty in Applying Concepts
Sometimes, it’s hard to apply what you’ve learned to real-world problems. Bridge this gap by working on projects that interest you and gradually increasing their complexity as you learn new concepts.
Advanced Topics to Explore
Once you’ve mastered the basics, there are many advanced topics you can explore to deepen your programming knowledge:
1. Data Structures and Algorithms
Understanding complex data structures (like trees and graphs) and algorithms is crucial for writing efficient code and succeeding in technical interviews.
2. Database Management
Learn how to work with databases, including SQL for relational databases and NoSQL for non-relational databases.
3. Web Development Frameworks
Explore popular web development frameworks like React, Angular, or Vue.js for front-end development, and Express.js, Django, or Ruby on Rails for back-end development.
4. Cloud Computing
Familiarize yourself with cloud platforms like AWS, Google Cloud, or Microsoft Azure. Cloud computing skills are increasingly in demand in the tech industry.
5. Machine Learning and Artificial Intelligence
If you’re interested in AI, start learning about machine learning algorithms, neural networks, and popular libraries like TensorFlow or PyTorch.
6. Mobile App Development
Learn how to develop mobile applications for iOS or Android platforms, or explore cross-platform development with frameworks like React Native or Flutter.
Conclusion
Starting your programming journey can seem daunting, but remember that every expert was once a beginner. With patience, persistence, and consistent practice, you can develop strong programming skills. Embrace the learning process, celebrate your progress, and don’t be afraid to make mistakes – they’re an essential part of learning.
As you continue your journey, you’ll discover that programming is not just about writing code; it’s about problem-solving, creativity, and continuous learning. The field of technology is ever-evolving, offering endless opportunities to learn and grow.
Whether you’re aiming for a career change, looking to enhance your current job skills, or simply exploring a new hobby, learning to code is a valuable investment in yourself. So, take that first step, write your first line of code, and welcome to the exciting world of programming!
Remember, the key to success in programming is persistence and practice. Keep coding, keep learning, and most importantly, enjoy the process. Happy coding!