Genetic Algorithms and Evolutionary Computation: Harnessing Nature’s Wisdom in Code
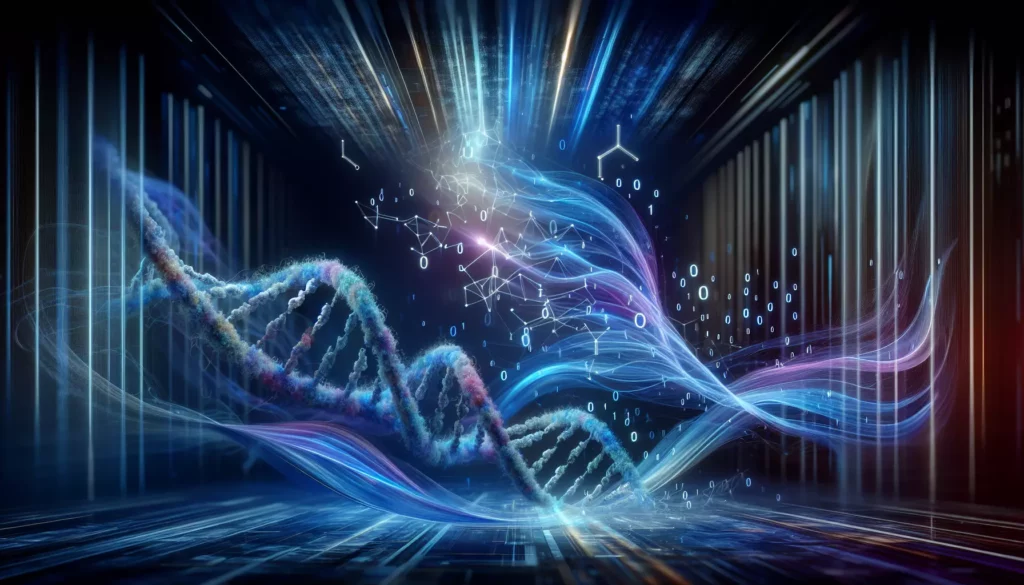
In the vast landscape of computer science and artificial intelligence, there’s a fascinating field that draws inspiration from one of the most powerful forces in nature: evolution. This field, known as Genetic Algorithms (GAs) and Evolutionary Computation (EC), applies the principles of natural selection and genetics to solve complex optimization problems and develop innovative solutions. In this comprehensive guide, we’ll dive deep into the world of genetic algorithms and evolutionary computation, exploring their concepts, applications, and implementation in programming.
Understanding Genetic Algorithms and Evolutionary Computation
Before we delve into the intricacies of genetic algorithms and evolutionary computation, let’s start with a basic understanding of what these terms mean and how they relate to the broader field of artificial intelligence and computer science.
What are Genetic Algorithms?
Genetic Algorithms are a subset of evolutionary algorithms inspired by the process of natural selection. They are used to find optimal or near-optimal solutions to complex problems that would be difficult or impossible to solve using traditional methods. GAs mimic the biological processes of reproduction, mutation, crossover, and selection to evolve a population of potential solutions over multiple generations.
What is Evolutionary Computation?
Evolutionary Computation is a broader term that encompasses various population-based optimization algorithms inspired by biological evolution. This includes genetic algorithms, evolutionary programming, evolution strategies, and genetic programming. EC techniques are particularly useful for solving problems where the search space is large, complex, or poorly understood.
Key Concepts in Genetic Algorithms
To understand how genetic algorithms work, it’s essential to familiarize yourself with some key concepts and terminology:
1. Population
In a genetic algorithm, a population is a set of potential solutions to the problem at hand. Each individual in the population represents a possible solution and is typically encoded as a string of genes (often binary digits).
2. Chromosome
A chromosome is an individual solution within the population. It contains a set of genes that encode the characteristics of the solution.
3. Gene
A gene is a single unit of information within a chromosome. In binary encoding, each gene is represented by a 0 or 1.
4. Fitness Function
The fitness function evaluates how well a particular solution (chromosome) solves the problem. It assigns a fitness score to each individual in the population, determining their likelihood of being selected for reproduction.
5. Selection
Selection is the process of choosing individuals from the population to be parents for the next generation. Individuals with higher fitness scores have a higher probability of being selected.
6. Crossover
Crossover is the process of combining genetic information from two parent chromosomes to create one or more offspring. This operation allows the algorithm to explore new areas of the solution space.
7. Mutation
Mutation introduces small, random changes to individual genes in a chromosome. This helps maintain genetic diversity in the population and prevents premature convergence to suboptimal solutions.
The Genetic Algorithm Process
Now that we’ve covered the basic concepts, let’s walk through the typical steps involved in a genetic algorithm:
- Initialization: Create an initial population of random individuals.
- Fitness Evaluation: Calculate the fitness of each individual in the population.
- Selection: Select individuals to be parents based on their fitness scores.
- Crossover: Create offspring by combining genetic information from selected parents.
- Mutation: Introduce small random changes to some individuals in the new population.
- Replacement: Replace the old population with the new generation of individuals.
- Termination Check: If the termination criteria are met (e.g., a satisfactory solution is found or a maximum number of generations is reached), stop the algorithm. Otherwise, go back to step 2.
Implementing a Simple Genetic Algorithm in Python
To better understand how genetic algorithms work in practice, let’s implement a simple GA to solve a basic optimization problem: finding the maximum value of the function f(x) = x^2 in the range [0, 31].
import random
# Define the fitness function
def fitness_function(x):
return x**2
# Create a random individual (chromosome)
def create_individual():
return random.randint(0, 31)
# Create the initial population
def create_population(pop_size):
return [create_individual() for _ in range(pop_size)]
# Select parents using tournament selection
def tournament_selection(population, tournament_size):
tournament = random.sample(population, tournament_size)
return max(tournament, key=fitness_function)
# Perform crossover (single-point)
def crossover(parent1, parent2):
crossover_point = random.randint(0, 4) # 5-bit representation
mask = (1 << crossover_point) - 1
child = (parent1 & mask) | (parent2 & ~mask)
return child
# Perform mutation
def mutate(individual, mutation_rate):
if random.random() < mutation_rate:
mutation_point = random.randint(0, 4)
individual ^= (1 << mutation_point)
return individual
# Main genetic algorithm function
def genetic_algorithm(pop_size, generations, tournament_size, mutation_rate):
population = create_population(pop_size)
for generation in range(generations):
new_population = []
for _ in range(pop_size):
parent1 = tournament_selection(population, tournament_size)
parent2 = tournament_selection(population, tournament_size)
child = crossover(parent1, parent2)
child = mutate(child, mutation_rate)
new_population.append(child)
population = new_population
best_individual = max(population, key=fitness_function)
print(f"Generation {generation + 1}: Best fitness = {fitness_function(best_individual)}, x = {best_individual}")
return max(population, key=fitness_function)
# Run the genetic algorithm
best_solution = genetic_algorithm(pop_size=50, generations=20, tournament_size=3, mutation_rate=0.1)
print(f"Best solution: x = {best_solution}, f(x) = {fitness_function(best_solution)}")
This simple implementation demonstrates the core concepts of genetic algorithms. When you run this code, you’ll see how the population evolves over generations, gradually converging towards the optimal solution (x = 31, f(x) = 961).
Applications of Genetic Algorithms and Evolutionary Computation
Genetic algorithms and evolutionary computation techniques have found applications in various fields due to their ability to solve complex optimization problems. Some notable applications include:
1. Optimization Problems
GAs are excellent at solving optimization problems in various domains, such as:
- Route optimization for logistics and transportation
- Resource allocation in project management
- Financial portfolio optimization
- Engineering design optimization
2. Machine Learning and Artificial Intelligence
Evolutionary computation techniques are used in machine learning for:
- Feature selection and hyperparameter tuning
- Evolving neural network architectures
- Reinforcement learning policy optimization
3. Bioinformatics
In the field of bioinformatics, GAs are applied to:
- Protein structure prediction
- Gene expression analysis
- DNA sequence alignment
4. Game Development and AI
Evolutionary algorithms are used in game development for:
- Evolving game strategies and AI opponents
- Procedural content generation
- Balancing game mechanics
5. Robotics
In robotics, evolutionary computation is applied to:
- Robot motion planning
- Evolving robot control systems
- Optimizing robot designs
Advantages and Limitations of Genetic Algorithms
Like any algorithmic approach, genetic algorithms have their strengths and weaknesses. Understanding these can help you decide when to use GAs in your projects.
Advantages:
- Versatility: GAs can be applied to a wide range of problems, including those with complex or unknown search spaces.
- Parallelization: The population-based nature of GAs makes them easily parallelizable, allowing for efficient implementation on multi-core processors or distributed systems.
- Handling noisy or incomplete data: GAs are robust to noise and can work with incomplete or imperfect information.
- Finding multiple solutions: GAs can maintain a diverse population, potentially finding multiple good solutions to a problem.
- No need for derivative information: Unlike some optimization techniques, GAs don’t require gradient information, making them suitable for non-differentiable or discontinuous problems.
Limitations:
- Computational intensity: GAs often require many fitness function evaluations, which can be computationally expensive for complex problems.
- Parameter tuning: The performance of GAs can be sensitive to parameter settings (e.g., population size, mutation rate), which may require careful tuning.
- No guarantee of optimality: While GAs can find good solutions, they don’t guarantee finding the global optimum.
- Difficulty in constraint handling: Incorporating constraints into the problem formulation can be challenging with GAs.
- Premature convergence: GAs may converge to suboptimal solutions if not properly designed or if genetic diversity is lost too quickly.
Advanced Concepts in Evolutionary Computation
As you delve deeper into the field of evolutionary computation, you’ll encounter more advanced concepts and variations of genetic algorithms. Some of these include:
1. Multi-Objective Optimization
Many real-world problems involve multiple, often conflicting objectives. Multi-objective evolutionary algorithms (MOEAs) are designed to find a set of Pareto-optimal solutions that represent different trade-offs between objectives.
2. Coevolution
Coevolutionary algorithms involve multiple populations that evolve simultaneously, often in competition or cooperation with each other. This approach is particularly useful for problems where the fitness of an individual depends on other evolving entities.
3. Memetic Algorithms
Memetic algorithms combine genetic algorithms with local search techniques. This hybrid approach can improve the efficiency of the search process by allowing individuals to improve themselves through local optimization.
4. Genetic Programming
Genetic Programming (GP) is a specialized form of genetic algorithm where the individuals in the population are computer programs rather than fixed-length strings. GP is used to evolve programs or expressions that perform a specific task.
5. Differential Evolution
Differential Evolution (DE) is a population-based optimization algorithm that uses vector differences for perturbing the population vectors. It’s particularly effective for continuous optimization problems.
Implementing Genetic Algorithms in Practice
When implementing genetic algorithms for real-world problems, consider the following best practices:
- Problem Encoding: Choose an appropriate representation for your problem. The encoding should allow for easy manipulation of solutions and efficient evaluation of the fitness function.
- Population Diversity: Maintain genetic diversity in your population to prevent premature convergence. This can be achieved through appropriate selection methods, mutation rates, and population management techniques.
- Fitness Function Design: Carefully design your fitness function to accurately reflect the quality of solutions. Consider using techniques like fitness scaling or ranking to maintain selection pressure throughout the evolution process.
- Parameter Tuning: Experiment with different parameter settings (population size, crossover rate, mutation rate) to find the best configuration for your specific problem.
- Constraint Handling: If your problem involves constraints, consider using penalty functions, repair operators, or specialized constraint-handling techniques.
- Hybridization: Consider combining GAs with other optimization techniques or problem-specific heuristics to improve performance.
- Performance Evaluation: Use appropriate metrics to evaluate the performance of your GA, and compare it against other optimization methods when possible.
Conclusion
Genetic Algorithms and Evolutionary Computation represent a powerful and flexible approach to problem-solving inspired by the principles of natural selection. By harnessing the power of evolution in code, we can tackle complex optimization problems and develop innovative solutions across various domains.
As you continue your journey in programming and artificial intelligence, exploring genetic algorithms and evolutionary computation can provide you with valuable insights into optimization techniques and nature-inspired computing. These concepts not only enhance your problem-solving skills but also open up new possibilities for creative and efficient solution design.
Whether you’re preparing for technical interviews at major tech companies or simply looking to expand your coding repertoire, understanding and implementing genetic algorithms can be a valuable addition to your skill set. As you practice and experiment with these techniques, you’ll gain a deeper appreciation for the elegance and power of evolutionary approaches in computer science.
Remember, the key to mastering genetic algorithms lies in hands-on experience. Start with simple problems, gradually increase complexity, and don’t be afraid to experiment with different variations and parameters. Happy evolving!