Garbage Collection in Programming: Keeping Memory Clean and Efficient
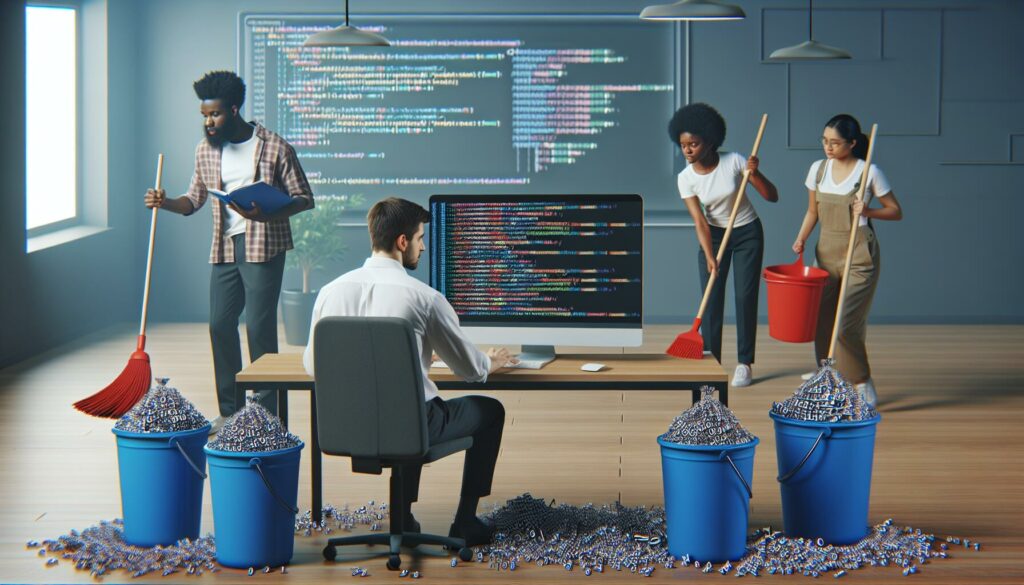
In the world of programming, memory management is a crucial aspect that can significantly impact the performance and stability of applications. One of the most important concepts in this realm is garbage collection. This automatic memory management mechanism has revolutionized how developers write code and manage resources. In this comprehensive guide, we’ll dive deep into the world of garbage collection, exploring its importance, mechanisms, and impact on modern programming languages.
Table of Contents
- What is Garbage Collection?
- The Importance of Garbage Collection
- How Garbage Collection Works
- Common Garbage Collection Algorithms
- Programming Languages with Garbage Collection
- Advantages and Disadvantages of Garbage Collection
- Best Practices for Working with Garbage Collection
- Garbage Collection in Technical Interviews
- The Future of Garbage Collection
- Conclusion
1. What is Garbage Collection?
Garbage collection is an automatic memory management feature in many modern programming languages. It’s a process that identifies and removes objects from memory that are no longer being used by the program. This eliminates the need for manual memory deallocation, which was a common source of errors in languages like C and C++.
The term “garbage” refers to memory occupied by objects that are no longer accessible or useful to the program. The garbage collector’s job is to find this garbage and reclaim the memory, making it available for future allocations.
2. The Importance of Garbage Collection
Garbage collection plays a crucial role in modern software development for several reasons:
- Memory Leak Prevention: It helps prevent memory leaks by automatically freeing up memory that’s no longer in use.
- Simplified Development: Developers can focus on core application logic instead of worrying about memory management.
- Increased Productivity: By eliminating manual memory management, it reduces development time and debugging efforts.
- Enhanced Security: It helps prevent certain types of security vulnerabilities related to memory mismanagement.
- Improved Stability: Automatic memory management reduces the risk of crashes due to memory-related errors.
3. How Garbage Collection Works
The process of garbage collection typically involves three main steps:
3.1. Marking
In this phase, the garbage collector identifies which objects in memory are still in use (reachable) and which are no longer needed (unreachable). It starts from the “root set” of objects (global variables, stack variables, etc.) and traverses through all references to mark live objects.
3.2. Sweeping
After marking, the garbage collector sweeps through the heap, identifying unmarked (unreachable) objects. These are considered garbage and their memory is ready to be reclaimed.
3.3. Compacting (Optional)
Some garbage collectors perform an additional step called compaction. This involves moving all the surviving objects to one end of the heap, leaving a large contiguous block of free memory. This helps reduce memory fragmentation and improves allocation efficiency.
4. Common Garbage Collection Algorithms
Several algorithms have been developed to implement garbage collection efficiently. Here are some of the most common ones:
4.1. Mark-and-Sweep
This is one of the simplest garbage collection algorithms. It follows the basic process described earlier: marking live objects, then sweeping through memory to reclaim space occupied by unmarked objects.
4.2. Copying Collection
This algorithm divides the heap into two equal halves. It copies live objects from one half to the other, then swaps their roles. This effectively compacts memory but requires twice the memory space.
4.3. Generational Collection
Based on the observation that most objects die young, this algorithm divides objects into generations. Younger generations are collected more frequently, while older generations are collected less often, improving overall efficiency.
4.4. Incremental Collection
Instead of stopping the program entirely during garbage collection, incremental collectors perform the collection in small increments, reducing pause times and improving responsiveness.
4.5. Concurrent Collection
This approach allows the garbage collector to run concurrently with the main program, further reducing pause times. However, it requires careful synchronization to prevent conflicts.
5. Programming Languages with Garbage Collection
Many modern programming languages include garbage collection as a core feature. Here are some popular examples:
- Java: Uses a generational garbage collector with different algorithms for different generations.
- Python: Employs reference counting with a cyclic garbage collector to handle reference cycles.
- JavaScript: Most implementations use a mark-and-sweep collector, often with incremental or concurrent collection.
- C#: Uses a generational garbage collector similar to Java’s.
- Go: Features a concurrent, tri-color mark-and-sweep collector.
- Ruby: Implements a generational garbage collector with incremental collection.
Let’s look at a simple example of how garbage collection works in Python:
def create_objects():
x = [1, 2, 3] # Create a list
y = [4, 5, 6] # Create another list
return x # Return x, y goes out of scope
result = create_objects()
# At this point, the list [4, 5, 6] is garbage and will be collected
# The list [1, 2, 3] is still referenced by 'result' and won't be collected
result = None # Remove the reference to [1, 2, 3]
# Now [1, 2, 3] is also garbage and will be collected
In this example, when create_objects()
returns, the list [4, 5, 6]
becomes unreachable and is eligible for garbage collection. Later, when we set result
to None
, the list [1, 2, 3]
also becomes unreachable and will be collected.
6. Advantages and Disadvantages of Garbage Collection
Advantages:
- Automatic Memory Management: Developers don’t need to manually free memory, reducing the risk of memory leaks and dangling pointers.
- Increased Productivity: Less time spent on memory management means more time for core application development.
- Improved Safety: Eliminates entire classes of bugs related to manual memory management.
- Better Abstraction: Allows for higher-level programming paradigms without worrying about low-level memory details.
Disadvantages:
- Performance Overhead: Garbage collection consumes CPU time and can cause pauses in program execution.
- Unpredictable Timing: It’s not always clear when garbage collection will occur, which can be problematic for real-time systems.
- Memory Overhead: Garbage collectors often require extra memory to operate efficiently.
- Lack of Control: Developers have less direct control over memory management, which can be a disadvantage in certain scenarios.
7. Best Practices for Working with Garbage Collection
While garbage collection automates memory management, developers should still follow best practices to ensure optimal performance:
- Minimize Object Creation: Create fewer objects to reduce the workload on the garbage collector.
- Reuse Objects: When possible, reuse existing objects instead of creating new ones.
- Use Value Types: In languages that support them, use value types for small, simple objects to reduce heap allocations.
- Implement Dispose Patterns: For large or resource-intensive objects, implement dispose patterns to release resources explicitly.
- Be Aware of Large Object Allocations: Large objects can impact garbage collection performance, so handle them carefully.
- Profile Your Application: Use profiling tools to understand your application’s memory usage and garbage collection patterns.
- Consider Weak References: Use weak references for caching scenarios to allow the garbage collector to reclaim memory when needed.
Here’s an example of implementing a dispose pattern in C#:
public class ResourceHandler : IDisposable
{
private bool disposed = false;
private IntPtr handle;
public ResourceHandler()
{
handle = // Acquire a resource
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!disposed)
{
if (disposing)
{
// Dispose managed resources
}
// Dispose unmanaged resources
if (handle != IntPtr.Zero)
{
// Release the handle
handle = IntPtr.Zero;
}
disposed = true;
}
}
~ResourceHandler()
{
Dispose(false);
}
}
8. Garbage Collection in Technical Interviews
Understanding garbage collection is crucial for technical interviews, especially for positions involving performance-critical applications or systems programming. Here are some topics related to garbage collection that you might encounter in interviews:
- Garbage Collection Algorithms: Be prepared to explain different garbage collection algorithms and their trade-offs.
- Memory Leaks: Understand how garbage collection helps prevent memory leaks and scenarios where leaks can still occur.
- Performance Implications: Be able to discuss the performance impact of garbage collection and strategies to minimize it.
- Language-Specific Implementations: Know the details of garbage collection in the primary language(s) you work with.
- Manual vs. Automatic Memory Management: Understand the pros and cons of each approach and when one might be preferred over the other.
- Weak References and Finalization: Be familiar with these concepts and how they relate to garbage collection.
A common interview question might be to explain how garbage collection works in a specific language. For example, if asked about Java’s garbage collection, you might respond:
“Java uses a generational garbage collection system. It divides the heap into multiple generations: young generation (further divided into Eden space and two survivor spaces), old generation, and permanent generation (or Metaspace in newer versions).
New objects are allocated in the Eden space. When Eden fills up, a minor garbage collection occurs, moving surviving objects to one of the survivor spaces. Objects that survive multiple minor collections are promoted to the old generation.
When the old generation fills up, a major garbage collection occurs, which can be more time-consuming. Java offers different garbage collector implementations, such as the Parallel GC, Concurrent Mark Sweep (CMS), and G1 GC, each with its own strengths and use cases.
The choice of garbage collector can significantly impact application performance, especially for large-scale or performance-critical applications.”
9. The Future of Garbage Collection
As software systems grow more complex and performance requirements become more stringent, garbage collection continues to evolve. Some emerging trends and areas of research include:
- Real-Time Garbage Collection: Developing garbage collectors with guaranteed pause times for real-time systems.
- Machine Learning-Assisted GC: Using machine learning to predict optimal times for garbage collection or to tune GC parameters automatically.
- Hardware-Assisted GC: Leveraging specialized hardware to accelerate garbage collection processes.
- Region-Based Memory Management: Exploring alternatives to traditional garbage collection, such as region-based memory management systems.
- Improved Concurrent and Parallel GC: Further reducing pause times and improving scalability for multi-core systems.
One interesting development is the concept of a pauseless garbage collector, as implemented in Azul’s C4 (Continuously Concurrent Compacting Collector) algorithm. This approach aims to eliminate stop-the-world pauses entirely, making garbage collection more suitable for latency-sensitive applications.
10. Conclusion
Garbage collection is a fundamental concept in modern programming that has significantly simplified memory management for developers. By automatically reclaiming unused memory, it allows programmers to focus on application logic rather than the intricacies of memory allocation and deallocation.
While garbage collection brings numerous benefits, it’s not without its challenges. Understanding how garbage collection works, its implications for performance, and best practices for working with garbage-collected languages is crucial for writing efficient and robust software.
As you continue your journey in software development, whether you’re preparing for technical interviews or building complex systems, a solid grasp of garbage collection will serve you well. It’s a topic that bridges the gap between high-level application development and low-level system operations, providing insights into both the conveniences of modern languages and the underlying mechanisms that make them possible.
Remember, while garbage collection handles much of the memory management for you, writing efficient code that works well with the garbage collector is still an important skill. By applying the best practices discussed in this article and staying informed about advancements in garbage collection technology, you’ll be well-equipped to develop high-performance, memory-efficient applications in any garbage-collected language.