Functions and Methods Explained: The Basics You Need to Know
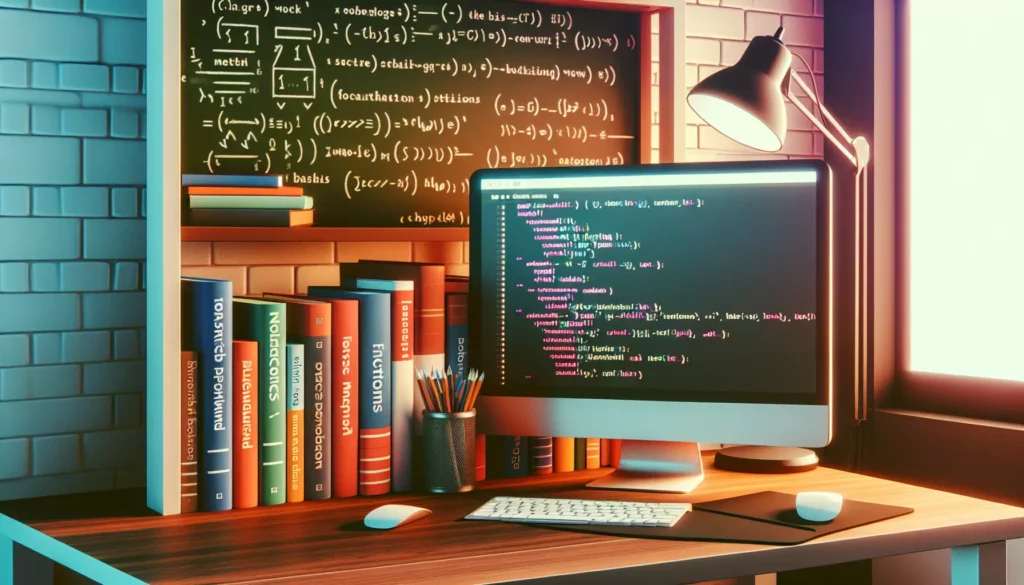
In the world of programming, functions and methods are fundamental building blocks that allow developers to create organized, reusable, and efficient code. Whether you’re just starting your coding journey or looking to solidify your understanding, this comprehensive guide will walk you through the essentials of functions and methods, their differences, and how to use them effectively in your programs.
What Are Functions?
Functions are self-contained blocks of code that perform a specific task. They are designed to be reusable, which means you can call them multiple times throughout your program without having to rewrite the same code over and over again. Functions help in organizing code, making it more readable, and reducing redundancy.
Anatomy of a Function
A typical function consists of the following parts:
- Function Name: A descriptive identifier for the function
- Parameters: Input values that the function can accept (optional)
- Function Body: The code that defines what the function does
- Return Statement: The value that the function sends back (optional)
Here’s a basic example of a function in Python:
def greet(name):
return f"Hello, {name}!"
# Calling the function
message = greet("Alice")
print(message) # Output: Hello, Alice!
Why Use Functions?
Functions offer several advantages in programming:
- Code Reusability: Write once, use many times
- Modularity: Break down complex problems into smaller, manageable parts
- Abstraction: Hide complex implementation details behind a simple interface
- Maintainability: Easier to update and debug isolated blocks of code
- Readability: Well-named functions make code self-documenting
What Are Methods?
Methods are similar to functions, but they are associated with objects or classes in object-oriented programming (OOP). In essence, a method is a function that belongs to a class or an object. Methods define the behavior of objects and allow them to interact with other parts of the program.
Methods vs. Functions
The main difference between methods and functions lies in their association:
- Functions are standalone blocks of code that can be called independently.
- Methods are functions that are bound to a specific class or object and operate on the data within that class or object.
Here’s an example to illustrate the difference:
# Function
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result) # Output: 8
# Method (part of a class)
class Calculator:
def add_numbers(self, a, b):
return a + b
calc = Calculator()
result = calc.add_numbers(5, 3)
print(result) # Output: 8
In the example above, add_numbers
is first defined as a standalone function. Then, it’s defined as a method within the Calculator
class. The method version is called on an instance of the class (calc
).
Types of Functions
Functions can be categorized in several ways based on their characteristics and behavior:
1. Built-in Functions
These are functions that come pre-defined in a programming language. They are ready to use without any additional import or definition. Examples in Python include print()
, len()
, and max()
.
numbers = [1, 2, 3, 4, 5]
print(len(numbers)) # Output: 5
print(max(numbers)) # Output: 5
2. User-defined Functions
These are functions created by the programmer to perform specific tasks. They allow for customization and extension of the language’s capabilities.
def calculate_area(length, width):
return length * width
area = calculate_area(5, 3)
print(f"The area is: {area}") # Output: The area is: 15
3. Anonymous Functions (Lambda Functions)
These are small, unnamed functions that can have any number of arguments but can only have one expression. They are often used for short-term use.
square = lambda x: x ** 2
print(square(4)) # Output: 16
4. Recursive Functions
These are functions that call themselves within their own body. They are useful for solving problems that can be broken down into smaller, similar sub-problems.
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
Function Parameters and Arguments
Parameters and arguments are crucial concepts in understanding how functions work:
Parameters
Parameters are the variables listed in the function definition. They act as placeholders for the values that will be passed to the function when it’s called.
Arguments
Arguments are the actual values passed to the function when it’s called. They correspond to the parameters defined in the function.
def greet(name, greeting): # name and greeting are parameters
return f"{greeting}, {name}!"
message = greet("Alice", "Hello") # "Alice" and "Hello" are arguments
print(message) # Output: Hello, Alice!
Types of Function Arguments
- Positional Arguments: Matched to parameters based on their position
- Keyword Arguments: Matched to parameters based on parameter names
- Default Arguments: Parameters with pre-defined default values
- Variable-length Arguments: Allow a function to accept any number of arguments
def example_function(a, b=2, *args, **kwargs):
print(f"a: {a}, b: {b}")
print(f"args: {args}")
print(f"kwargs: {kwargs}")
example_function(1, 3, 4, 5, x=6, y=7)
# Output:
# a: 1, b: 3
# args: (4, 5)
# kwargs: {'x': 6, 'y': 7}
Return Values
Functions can send back a value to the caller using the return
statement. This allows functions to compute and provide results that can be used elsewhere in the program.
def multiply(a, b):
return a * b
result = multiply(4, 5)
print(result) # Output: 20
Functions can return multiple values as well:
def min_max(numbers):
return min(numbers), max(numbers)
lowest, highest = min_max([1, 2, 3, 4, 5])
print(f"Lowest: {lowest}, Highest: {highest}")
# Output: Lowest: 1, Highest: 5
Scope and Lifetime of Variables
Understanding variable scope is crucial when working with functions:
Local Scope
Variables defined inside a function have a local scope and are only accessible within that function.
Global Scope
Variables defined outside of any function have a global scope and can be accessed throughout the program.
global_var = 10
def example_function():
local_var = 20
print(f"Inside function - global_var: {global_var}, local_var: {local_var}")
example_function()
print(f"Outside function - global_var: {global_var}")
# Trying to access local_var here would raise an error
Methods in Object-Oriented Programming
In object-oriented programming, methods are functions associated with classes. They define the behavior of objects created from those classes.
Types of Methods
- Instance Methods: Operate on individual object instances
- Class Methods: Operate on the class itself, rather than instances
- Static Methods: Don’t operate on instances or the class, but are related to the class in some way
class Example:
class_variable = 0
def __init__(self, value):
self.instance_variable = value
def instance_method(self):
return f"Instance value: {self.instance_variable}"
@classmethod
def class_method(cls):
return f"Class value: {cls.class_variable}"
@staticmethod
def static_method():
return "This is a static method"
# Usage
obj = Example(42)
print(obj.instance_method()) # Output: Instance value: 42
print(Example.class_method()) # Output: Class value: 0
print(Example.static_method()) # Output: This is a static method
Best Practices for Writing Functions and Methods
- Single Responsibility Principle: Each function or method should have a single, well-defined purpose.
- Keep it Short: Aim for functions that are short and focused. If a function gets too long, consider breaking it into smaller functions.
- Use Descriptive Names: Choose names that clearly describe what the function or method does.
- Document Your Code: Use comments and docstrings to explain the purpose, parameters, and return values of your functions and methods.
- Handle Errors: Use try-except blocks to handle potential errors and provide meaningful error messages.
- Avoid Side Effects: Try to make your functions “pure” by avoiding changes to variables outside the function’s scope.
- Use Type Hints: In languages that support it, use type hints to make your code more readable and catch potential type-related errors early.
Common Pitfalls and How to Avoid Them
- Overusing Global Variables: Instead of relying on global variables, pass necessary data as parameters to your functions.
- Not Handling Edge Cases: Consider and handle all possible input scenarios, including edge cases and invalid inputs.
- Ignoring Return Values: Always consider what your function should return and ensure it returns an appropriate value in all cases.
- Modifying Mutable Parameters: Be cautious when modifying mutable objects passed as arguments, as this can lead to unexpected side effects.
- Recursive Functions Without Base Case: Always include a base case in recursive functions to prevent infinite recursion.
Advanced Concepts
Closures
Closures are functions that remember and have access to variables from their outer (enclosing) scope, even after the outer function has finished executing.
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
closure = outer_function(10)
result = closure(5)
print(result) # Output: 15
Decorators
Decorators are a way to modify or enhance functions without changing their source code. They are often used for logging, timing functions, or adding authentication checks.
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
Generator Functions
Generator functions allow you to declare a function that behaves like an iterator. They can be used to work with large datasets efficiently by yielding one item at a time.
def countdown(n):
while n > 0:
yield n
n -= 1
for number in countdown(5):
print(number)
# Output:
# 5
# 4
# 3
# 2
# 1
Conclusion
Functions and methods are powerful tools in a programmer’s toolkit. They allow for code reuse, improve readability, and help in organizing complex programs into manageable pieces. By mastering these concepts, you’ll be well on your way to writing cleaner, more efficient, and more maintainable code.
Remember, practice is key to truly understanding and effectively using functions and methods. Try to incorporate them into your projects, experiment with different types of functions, and always look for opportunities to refactor your code using these principles.
As you continue your journey in programming, you’ll discover even more advanced uses of functions and methods. Keep exploring, keep learning, and most importantly, keep coding!