Functional Programming vs OOP: Understanding the Key Differences
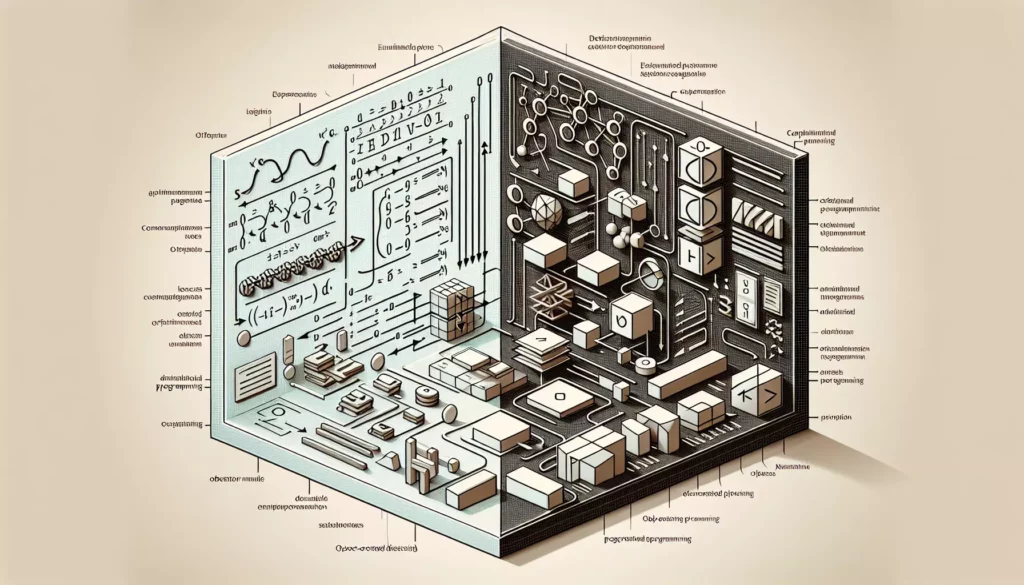
In the ever-evolving landscape of software development, two programming paradigms stand out as pillars of modern coding practices: Functional Programming (FP) and Object-Oriented Programming (OOP). As aspiring developers and seasoned programmers alike navigate the complexities of coding, understanding these paradigms becomes crucial for writing efficient, maintainable, and scalable code. In this comprehensive guide, we’ll delve deep into the world of functional programming, explore its core principles, and compare it with the widely-used object-oriented approach.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state. In essence, functional programming is based on the following key principles:
- Immutability: Data cannot be changed once created.
- Pure Functions: Functions always produce the same output for the same input and have no side effects.
- First-Class and Higher-Order Functions: Functions can be assigned to variables, passed as arguments, and returned from other functions.
- Recursion: Iterative processes are often implemented through recursive function calls.
- Declarative Programming: Code focuses on what to do, rather than how to do it.
Core Concepts of Functional Programming
1. Immutability
In functional programming, once a variable is created, its value cannot be changed. Instead of modifying existing data structures, new ones are created with the desired changes. This approach helps prevent unexpected side effects and makes the code easier to reason about.
Example in JavaScript:
// Immutable approach
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(num => num * 2);
console.log(numbers); // [1, 2, 3, 4, 5]
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
2. Pure Functions
Pure functions are functions that always produce the same output for the same input and have no side effects. They don’t modify external state or depend on it, making them predictable and easy to test.
Example of a pure function:
function add(a, b) {
return a + b;
}
console.log(add(2, 3)); // Always returns 5
3. First-Class and Higher-Order Functions
In functional programming, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned from functions. Higher-order functions are functions that can take other functions as arguments or return them.
Example of a higher-order function:
function multiplyBy(factor) {
return function(number) {
return number * factor;
}
}
const double = multiplyBy(2);
console.log(double(5)); // 10
4. Recursion
Recursion is a technique where a function calls itself to solve a problem. In functional programming, recursion is often used instead of loops to iterate over data structures or perform repetitive tasks.
Example of a recursive function to calculate factorial:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
console.log(factorial(5)); // 120
5. Declarative Programming
Functional programming promotes a declarative style of coding, where you describe what you want to achieve rather than specifying the exact steps to achieve it. This often results in more concise and readable code.
Example of declarative vs imperative style:
// Imperative approach
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
// Declarative approach
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
Object-Oriented Programming (OOP): A Brief Overview
Before we dive into the differences between functional programming and OOP, let’s briefly review the key concepts of object-oriented programming:
- Classes and Objects: OOP is based on the concept of “objects” that contain data and code. Objects are instances of classes, which serve as blueprints for creating objects.
- Encapsulation: Data and methods that operate on that data are bundled together in objects, hiding internal details.
- Inheritance: Classes can inherit properties and methods from other classes, promoting code reuse.
- Polymorphism: Objects of different classes can be treated as objects of a common superclass, allowing for flexible and extensible code.
Key Differences Between Functional Programming and OOP
1. State Management
Functional Programming: Emphasizes immutability and avoids changing state. Data transformations create new data structures instead of modifying existing ones.
OOP: Relies on mutable state, where objects can change their internal state over time.
2. Code Organization
Functional Programming: Organizes code around functions and their compositions.
OOP: Organizes code around objects and their interactions.
3. Data and Behavior
Functional Programming: Separates data and behavior. Functions operate on data, but data structures are typically separate from the functions that use them.
OOP: Combines data and behavior within objects. Methods (functions) are typically associated with specific objects or classes.
4. Inheritance vs. Composition
Functional Programming: Favors composition over inheritance. Complex behaviors are built by combining simpler functions.
OOP: Often relies on inheritance hierarchies to share behavior between classes.
5. Side Effects
Functional Programming: Aims to minimize side effects by using pure functions and immutable data.
OOP: Side effects are more common, as objects can modify their own state or the state of other objects.
6. Concurrency
Functional Programming: Easier to parallelize due to the absence of shared state and side effects.
OOP: Requires careful management of shared state in concurrent scenarios.
When to Use Functional Programming vs. OOP
Choosing between functional programming and OOP depends on various factors, including the nature of the problem, team expertise, and project requirements. Here are some guidelines:
Consider Functional Programming When:
- You’re working on data processing or transformation tasks.
- You need to ensure predictability and avoid side effects.
- Your application requires high concurrency or parallelism.
- You’re dealing with complex mathematical or algorithmic problems.
- You want to maximize code reusability through function composition.
Consider OOP When:
- You’re modeling real-world objects or entities with clear behaviors and attributes.
- You need to maintain a complex state that changes over time.
- You’re working on a large-scale application with many interacting components.
- You want to leverage inheritance for code reuse and polymorphism for flexibility.
- Your team is more familiar with OOP concepts and design patterns.
Hybrid Approaches: The Best of Both Worlds
In practice, many modern programming languages and frameworks support both functional and object-oriented paradigms. This has led to the emergence of hybrid approaches that combine elements of both styles:
1. Functional-First Languages with OOP Support
Languages like Scala and F# are primarily functional but also support object-oriented features. This allows developers to use the most appropriate paradigm for different parts of their application.
2. OOP Languages with Functional Features
Traditional OOP languages like Java and C# have introduced functional programming concepts in recent versions. For example, Java 8 introduced lambda expressions and the Stream API, enabling more functional-style programming within an OOP context.
3. Functional Programming in OOP Design Patterns
Some design patterns traditionally associated with OOP can be implemented using functional programming concepts. For instance, the Strategy pattern can be implemented using higher-order functions instead of separate strategy classes.
Learning Functional Programming: Tips for OOP Developers
If you’re coming from an OOP background and want to explore functional programming, here are some tips to get started:
- Embrace Immutability: Start by avoiding mutable state and side effects in your code. Use const declarations and methods that return new objects instead of modifying existing ones.
- Practice with Pure Functions: Try to write functions that depend only on their inputs and produce consistent outputs without side effects.
- Explore Higher-Order Functions: Familiarize yourself with functions like map, filter, and reduce, which are cornerstones of functional programming.
- Learn Functional Data Structures: Study immutable data structures like linked lists and trees, and how to work with them efficiently.
- Experiment with Recursion: Practice solving problems using recursive approaches instead of imperative loops.
- Study Functional Design Patterns: Learn about functional design patterns like monads, functors, and applicatives.
- Explore Functional Languages: Consider learning a purely functional language like Haskell or a multi-paradigm language with strong functional support like Scala or F#.
Conclusion: Embracing Diversity in Programming Paradigms
Functional programming and object-oriented programming are both powerful paradigms with their own strengths and use cases. Understanding both approaches expands your toolkit as a developer and allows you to choose the most appropriate solution for each problem you encounter.
As the software development landscape continues to evolve, the lines between different programming paradigms are blurring. Many modern codebases incorporate elements of both functional and object-oriented programming, leveraging the strengths of each approach where they’re most beneficial.
By mastering both paradigms, you’ll be better equipped to write clean, efficient, and maintainable code across a wide range of projects and domains. Whether you’re building data-intensive applications, modeling complex systems, or developing high-concurrency services, having a deep understanding of both functional and object-oriented programming will make you a more versatile and effective developer.
Remember, the goal is not to rigidly adhere to a single paradigm, but to have the flexibility to choose the right tool for the job. As you continue your journey in software development, strive to understand the principles behind different programming paradigms and how they can be applied to solve real-world problems effectively.