Functional Programming vs Object-Oriented Programming: A Comprehensive Guide
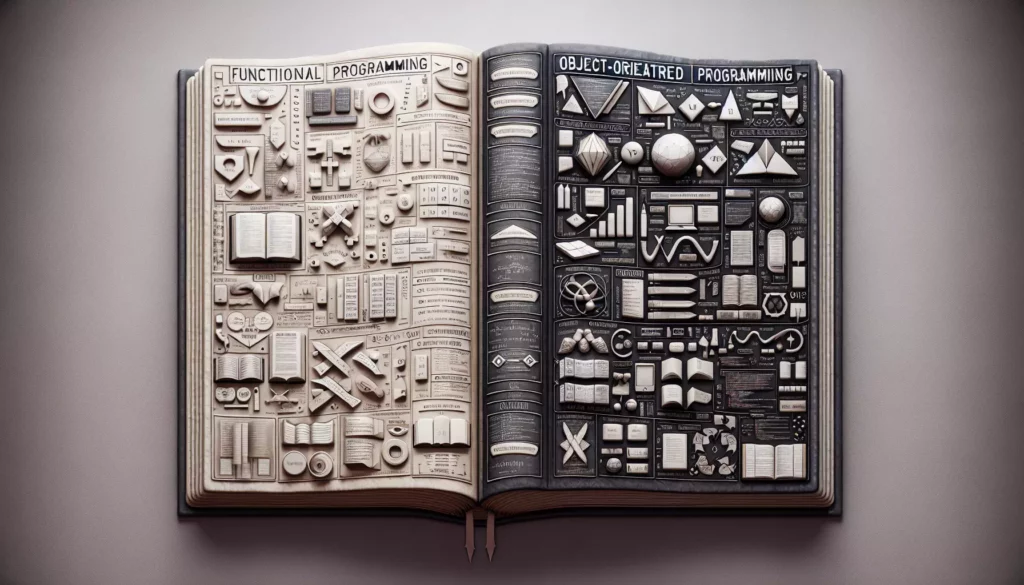
In the world of software development, there are various programming paradigms that developers can choose from when building applications. Two of the most popular and widely discussed paradigms are Functional Programming (FP) and Object-Oriented Programming (OOP). Both approaches have their strengths and weaknesses, and understanding the differences between them can help you make informed decisions about which paradigm to use for your projects. In this comprehensive guide, we’ll explore functional programming, how it differs from OOP, and when to use each paradigm.
Table of Contents
- What is Functional Programming?
- Key Concepts of Functional Programming
- What is Object-Oriented Programming?
- Key Concepts of OOP
- Differences Between FP and OOP
- Advantages of Functional Programming
- Advantages of OOP
- When to Use Functional Programming
- When to Use OOP
- Combining FP and OOP
- Learning FP and OOP
- Conclusion
What is Functional Programming?
Functional Programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state. In other words, functional programming is about writing programs by composing pure functions, avoiding side effects, and using immutable data.
The roots of functional programming can be traced back to lambda calculus, a formal system developed in the 1930s by Alonzo Church. Many functional programming languages, such as Haskell, Lisp, and Erlang, have been influenced by lambda calculus.
Key Concepts of Functional Programming
To better understand functional programming, let’s explore some of its key concepts:
1. Pure Functions
Pure functions are functions that always produce the same output for the same input and have no side effects. They don’t modify any external state or data. This property makes pure functions predictable and easy to test.
Example of a pure function in JavaScript:
const add = (a, b) => a + b;
console.log(add(2, 3)); // Always outputs 5
2. Immutability
Immutability is the practice of not changing data once it’s created. Instead of modifying existing data, functional programming creates new data structures with the desired changes. This approach helps prevent unexpected side effects and makes it easier to reason about the program’s state.
Example of immutability in JavaScript:
const originalArray = [1, 2, 3];
const newArray = [...originalArray, 4]; // Creates a new array instead of modifying the original
console.log(originalArray); // [1, 2, 3]
console.log(newArray); // [1, 2, 3, 4]
3. First-Class and Higher-Order Functions
In functional programming, functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned from functions. Higher-order functions are functions that can take other functions as arguments or return functions.
Example of a higher-order function in JavaScript:
const multiplyBy = (factor) => (number) => number * factor;
const double = multiplyBy(2);
const triple = multiplyBy(3);
console.log(double(5)); // 10
console.log(triple(5)); // 15
4. Recursion
Recursion is a technique where a function calls itself to solve a problem. It’s often used in functional programming as an alternative to imperative loops. Recursive functions can be more elegant and easier to reason about for certain types of problems.
Example of recursion in JavaScript:
const factorial = (n) => {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
};
console.log(factorial(5)); // 120
5. Function Composition
Function composition is the process of combining two or more functions to create a new function. This allows for building complex operations from simpler ones, promoting code reuse and modularity.
Example of function composition in JavaScript:
const compose = (f, g) => (x) => f(g(x));
const double = (x) => x * 2;
const increment = (x) => x + 1;
const doubleAndIncrement = compose(increment, double);
console.log(doubleAndIncrement(5)); // 11
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of “objects,” which can contain data in the form of fields (attributes or properties) and code in the form of procedures (methods). OOP uses the concepts of classes and objects to organize and structure code, promoting modularity, reusability, and encapsulation.
The origins of OOP can be traced back to the 1960s, with the development of the Simula programming language. However, it gained widespread popularity in the 1980s and 1990s with languages like C++ and Java.
Key Concepts of OOP
Let’s explore the main concepts that define object-oriented programming:
1. Classes and Objects
A class is a blueprint for creating objects. It defines the attributes and methods that the objects of that class will have. An object is an instance of a class, representing a specific entity with its own set of data and behaviors.
Example of a class and object in Python:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
return f"{self.make} {self.model}"
my_car = Car("Toyota", "Corolla")
print(my_car.display_info()) # Toyota Corolla
2. Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit (object). It also involves restricting direct access to some of an object’s components, which is often done using access modifiers like public, private, and protected.
Example of encapsulation in Java:
public class BankAccount {
private double balance;
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
public double getBalance() {
return balance;
}
}
3. Inheritance
Inheritance is a mechanism that allows a class to inherit properties and methods from another class. It promotes code reuse and establishes a relationship between parent and child classes.
Example of inheritance in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Buddy says Woof!
print(cat.speak()) # Whiskers says Meow!
4. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common base class. It enables you to write code that can work with objects of multiple types, as long as they share a common interface or base class.
Example of polymorphism in Java:
interface Shape {
double getArea();
}
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
}
class Rectangle implements Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return width * height;
}
}
public class Main {
public static void printArea(Shape shape) {
System.out.println("Area: " + shape.getArea());
}
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
printArea(circle); // Area: 78.53981633974483
printArea(rectangle); // Area: 24.0
}
}
Differences Between FP and OOP
Now that we’ve explored the key concepts of both functional programming and object-oriented programming, let’s compare them side by side to highlight their main differences:
Aspect | Functional Programming | Object-Oriented Programming |
---|---|---|
Basic Unit | Functions | Objects (Classes) |
Data and Behavior | Separated (Data is immutable) | Combined (Encapsulated in objects) |
State Management | Avoids mutable state | Manages state within objects |
Side Effects | Minimizes side effects | Allows side effects |
Code Organization | Organized around functions | Organized around objects and classes |
Inheritance | Typically uses composition | Uses class-based inheritance |
Polymorphism | Achieved through higher-order functions | Achieved through interfaces and method overriding |
Typical Use Cases | Data processing, parallel processing, mathematical computations | GUI applications, simulations, complex systems modeling |
Advantages of Functional Programming
- Predictability: Pure functions always produce the same output for the same input, making the code more predictable and easier to test.
- Modularity: Functions can be easily composed and reused, promoting modular design.
- Concurrency: Immutable data and lack of side effects make it easier to write concurrent and parallel programs.
- Debugging: With pure functions and immutable data, it’s easier to trace the flow of data and identify issues.
- Mathematical reasoning: FP’s roots in mathematical concepts make it suitable for certain types of problem-solving and algorithm design.
Advantages of OOP
- Intuitive modeling: OOP allows for intuitive modeling of real-world entities and their relationships.
- Encapsulation: Data and behavior are bundled together, promoting better organization and data protection.
- Code reuse: Inheritance and polymorphism facilitate code reuse and extensibility.
- Maintainability: OOP’s modular structure can make large codebases easier to maintain and update.
- Widely adopted: OOP is widely used in industry, with many established design patterns and best practices.
When to Use Functional Programming
Functional programming is particularly well-suited for:
- Data processing and transformation pipelines
- Parallel and concurrent programming
- Mathematical and scientific computing
- Building robust and predictable APIs
- Scenarios where immutability and lack of side effects are crucial
- Developing compact, reusable utility functions
When to Use OOP
Object-Oriented Programming is often the better choice for:
- Modeling complex systems with many interacting entities
- Developing graphical user interfaces (GUIs)
- Game development
- Large-scale enterprise applications
- Scenarios where the relationship between data and behavior is tightly coupled
- Projects that require a clear hierarchical structure
Combining FP and OOP
It’s worth noting that functional programming and object-oriented programming are not mutually exclusive. Many modern programming languages and frameworks allow developers to use both paradigms together, leveraging the strengths of each approach where appropriate. This hybrid approach can lead to more flexible and powerful software designs.
For example:
- You can use functional programming concepts like immutability and pure functions within an object-oriented codebase to improve predictability and testability.
- You can apply object-oriented design principles to organize and structure a primarily functional codebase.
- Some languages, like Scala, are designed to support both OOP and FP paradigms seamlessly.
Here’s an example of combining FP and OOP concepts in Python:
from functools import reduce
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, item):
self.items.append(item)
def get_total(self):
return reduce(lambda total, item: total + item.price, self.items, 0)
class Item:
def __init__(self, name, price):
self.name = name
self.price = price
# Using the classes
cart = ShoppingCart()
cart.add_item(Item("Book", 15))
cart.add_item(Item("Pen", 2))
cart.add_item(Item("Notebook", 5))
print(f"Total: ${cart.get_total()}") # Total: $22
In this example, we use OOP to define the structure of our `ShoppingCart` and `Item` classes, while employing functional programming concepts like `reduce` and lambda functions to calculate the total price.
Learning FP and OOP
For aspiring developers and those looking to expand their skills, it’s valuable to learn both functional programming and object-oriented programming. Understanding these paradigms will make you a more versatile programmer and enable you to choose the best approach for each project or problem you encounter.
To get started with learning FP and OOP:
- Choose a language: Pick a language that supports both paradigms, such as Python, JavaScript, or Scala.
- Study the fundamentals: Start with the basic concepts of each paradigm and practice implementing them.
- Work on projects: Apply your knowledge to real-world projects, experimenting with both FP and OOP approaches.
- Analyze existing codebases: Study open-source projects that use FP, OOP, or a combination of both to see how they’re applied in practice.
- Participate in coding challenges: Platforms like LeetCode, HackerRank, or CodeWars offer problems that can be solved using various paradigms.
- Join communities: Engage with other developers through forums, local meetups, or online communities to share knowledge and experiences.
Conclusion
Functional programming and object-oriented programming are two powerful paradigms in the world of software development. While they have different approaches to organizing code and managing data, both have their strengths and are suited to different types of problems.
Functional programming emphasizes immutability, pure functions, and the avoidance of side effects, making it excellent for data processing, concurrent programming, and scenarios where predictability is crucial. Object-oriented programming, on the other hand, excels at modeling complex systems, creating intuitive hierarchies, and managing state within encapsulated objects.
As a developer, having a strong understanding of both paradigms will allow you to choose the right tool for the job and even combine them when appropriate. By mastering both FP and OOP, you’ll be well-equipped to tackle a wide range of programming challenges and create more efficient, maintainable, and robust software solutions.
Remember, the choice between FP and OOP (or a combination of both) should be based on the specific requirements of your project, your team’s expertise, and the problem domain you’re working in. As you gain experience with both paradigms, you’ll develop a intuition for when to apply each approach to achieve the best results.