Frontend vs Backend Development: Understanding the Key Differences
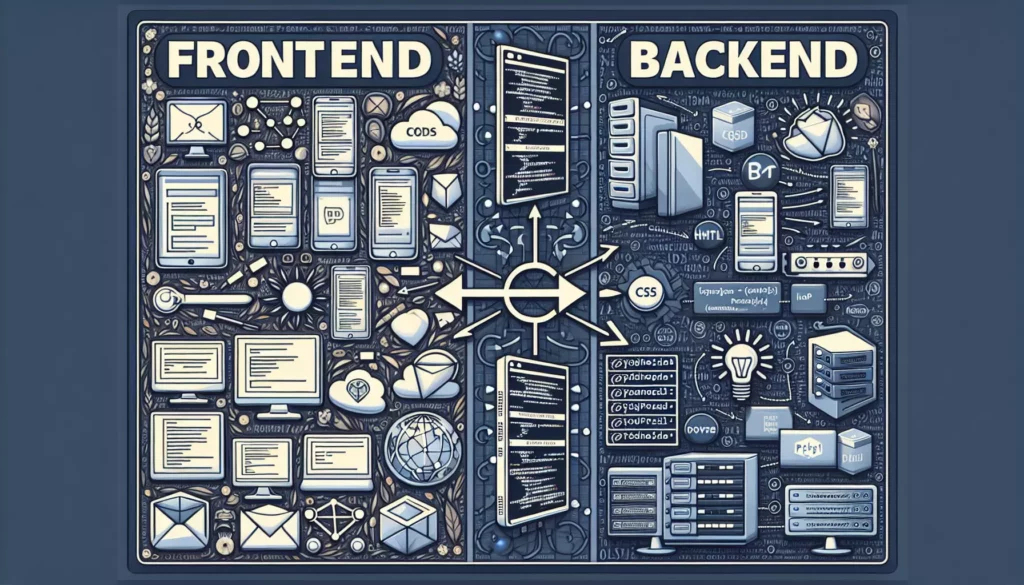
In the world of web development, two primary areas of specialization often come up in discussion: frontend and backend development. While both are crucial components of creating functional and user-friendly websites and applications, they serve distinct purposes and require different skill sets. In this comprehensive guide, we’ll explore the fundamental differences between frontend and backend development, helping you understand their roles, technologies, and how they work together to create the digital experiences we interact with daily.
What is Frontend Development?
Frontend development, also known as client-side development, focuses on creating the user interface and user experience of a website or application. It’s the part of web development that users directly interact with through their browsers. Frontend developers are responsible for designing and implementing the visual elements, layouts, and interactive features that make a website or app engaging and easy to use.
Key Responsibilities of Frontend Developers:
- Designing and implementing user interfaces
- Ensuring responsive design for various screen sizes and devices
- Creating interactive elements and animations
- Optimizing website performance for speed and efficiency
- Ensuring cross-browser compatibility
- Implementing accessibility features for users with disabilities
Essential Frontend Technologies:
- HTML (Hypertext Markup Language): The foundation of web content structure
- CSS (Cascading Style Sheets): Used for styling and layout of web pages
- JavaScript: Enables interactive and dynamic content on the client-side
- Frontend Frameworks and Libraries: Such as React, Vue.js, and Angular
- CSS Preprocessors: Like Sass and Less for more efficient styling
- Version Control Systems: Git for managing code changes and collaboration
What is Backend Development?
Backend development, also referred to as server-side development, involves working on the server side of web applications. It focuses on the logic, database interactions, server configuration, and application programming interfaces (APIs) that power the frontend. Backend developers ensure that data is processed, stored, and delivered efficiently to the frontend.
Key Responsibilities of Backend Developers:
- Designing and implementing server-side logic
- Creating and managing databases
- Developing APIs for frontend communication
- Ensuring data security and user authentication
- Optimizing server performance and scalability
- Integrating with external services and APIs
Essential Backend Technologies:
- Server-side Programming Languages: Such as Python, Java, Ruby, PHP, or Node.js
- Database Management Systems: Like MySQL, PostgreSQL, MongoDB, or Oracle
- Web Servers: Apache, Nginx, or Microsoft IIS
- APIs and Web Services: RESTful APIs, GraphQL, or SOAP
- Server-side Frameworks: Django, Ruby on Rails, Express.js, or Laravel
- Cloud Platforms: AWS, Google Cloud Platform, or Microsoft Azure
Key Differences Between Frontend and Backend Development
Now that we’ve outlined the basics of frontend and backend development, let’s dive deeper into the key differences between these two areas of web development:
1. User Interaction
Frontend: Directly interacts with users through browsers or mobile apps. It’s responsible for what users see and how they interact with the application.
Backend: Operates behind the scenes, processing requests, managing data, and serving information to the frontend. Users don’t directly interact with backend components.
2. Programming Languages
Frontend: Primarily uses HTML, CSS, and JavaScript. Additional frontend frameworks and libraries like React, Angular, or Vue.js are also commonly used.
Backend: Utilizes a wide range of programming languages such as Python, Java, Ruby, PHP, or Node.js. The choice often depends on the specific requirements of the project and the developer’s expertise.
3. Focus Areas
Frontend: Concentrates on user experience (UX), user interface (UI) design, visual appeal, and client-side performance optimization.
Backend: Focuses on server-side logic, database management, security, and scalability of the application.
4. Execution Environment
Frontend: Code is executed in the user’s web browser or mobile app.
Backend: Code runs on web servers, which can be physical machines or cloud-based services.
5. Data Handling
Frontend: Retrieves and displays data from the backend, often storing small amounts of data locally for improved performance (e.g., browser cache or local storage).
Backend: Manages large-scale data storage, retrieval, and processing. It interacts with databases and ensures data integrity and security.
6. Security Concerns
Frontend: Implements client-side security measures like input validation and secure communication with the backend. However, it’s generally considered less secure as code is visible to users.
Backend: Responsible for core security features such as user authentication, authorization, data encryption, and protection against various types of attacks (e.g., SQL injection, cross-site scripting).
7. Performance Optimization
Frontend: Focuses on optimizing load times, minimizing network requests, and ensuring smooth user interactions. Techniques include code splitting, lazy loading, and efficient DOM manipulation.
Backend: Concentrates on server response times, database query optimization, caching strategies, and efficient use of server resources to handle high traffic loads.
8. Scalability Considerations
Frontend: Scalability mainly involves ensuring the application works well across different devices and screen sizes (responsive design).
Backend: Deals with scaling the application to handle increased user loads, often involving techniques like load balancing, database sharding, and horizontal scaling of server infrastructure.
The Intersection: Full-Stack Development
While frontend and backend development are distinct areas, many developers choose to specialize in both, becoming what is known as full-stack developers. Full-stack development involves understanding and working with both client-side and server-side technologies, allowing developers to build complete web applications from start to finish.
Full-stack developers have a broader skill set that includes:
- Frontend technologies (HTML, CSS, JavaScript, and frontend frameworks)
- Backend programming languages and frameworks
- Database management and design
- Server configuration and deployment
- Version control and project management
While being a full-stack developer can be advantageous, especially for smaller projects or startups, larger organizations often prefer specialists who can dive deep into either frontend or backend development.
Choosing Between Frontend and Backend Development
If you’re considering a career in web development, you might be wondering whether to focus on frontend or backend development. Here are some factors to consider:
Choose Frontend Development If:
- You have a keen eye for design and user experience
- You enjoy working on visual elements and creating interactive interfaces
- You’re interested in the latest design trends and user interface technologies
- You like seeing immediate results of your work in the browser
- You enjoy problem-solving related to user interactions and browser compatibility
Choose Backend Development If:
- You’re interested in working with data and databases
- You enjoy solving complex logical problems
- You’re passionate about system architecture and scalability
- You’re interested in security and data protection
- You like working on the core functionality that powers applications
The Importance of Communication Between Frontend and Backend
While frontend and backend development are separate disciplines, effective communication between these two areas is crucial for successful web development projects. Here’s why:
1. API Design and Implementation
Frontend and backend developers need to collaborate closely on designing and implementing APIs (Application Programming Interfaces). These APIs serve as the bridge between the frontend and backend, defining how data is exchanged between the two.
Example of a simple API endpoint in Node.js with Express:
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// Fetch users from database
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
];
res.json(users);
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
2. Data Formatting and Structure
Both frontend and backend developers need to agree on the format and structure of data being exchanged. This ensures that the frontend can correctly interpret and display the data received from the backend.
3. Performance Optimization
Collaboration between frontend and backend teams is essential for optimizing overall application performance. This might involve decisions about caching strategies, data pagination, or implementing real-time updates using technologies like WebSockets.
4. Security Considerations
Frontend and backend developers must work together to implement robust security measures. This includes proper handling of user authentication, secure data transmission, and protection against common web vulnerabilities.
5. Feature Implementation
When implementing new features, frontend and backend developers need to coordinate their efforts. This ensures that the necessary backend functionality is in place to support new frontend features and vice versa.
The Future of Frontend and Backend Development
As web technologies continue to evolve, the landscape of frontend and backend development is constantly changing. Here are some trends shaping the future of web development:
1. Serverless Architecture
Serverless computing is gaining popularity, blurring the lines between frontend and backend development. With serverless platforms, developers can focus more on writing code and less on managing server infrastructure.
2. JAMstack
The JAMstack (JavaScript, APIs, and Markup) approach to web development is growing, emphasizing static site generation and decoupling the frontend from the backend.
3. Progressive Web Apps (PWAs)
PWAs are becoming more prevalent, combining the best of web and mobile apps. This trend requires frontend developers to have a deeper understanding of performance optimization and offline capabilities.
4. AI and Machine Learning Integration
Both frontend and backend developers will increasingly need to work with AI and machine learning technologies, integrating intelligent features into web applications.
5. WebAssembly
WebAssembly is enabling high-performance code to run in web browsers, potentially changing how we think about frontend development and browser capabilities.
Conclusion
Understanding the differences between frontend and backend development is crucial for anyone looking to enter the field of web development or seeking to collaborate effectively in a development team. While frontend development focuses on creating engaging user interfaces and experiences, backend development powers the core functionality and data management of web applications.
Both areas require unique skill sets and offer exciting challenges. Whether you choose to specialize in frontend, backend, or pursue full-stack development, the key to success lies in continuous learning and adapting to new technologies and best practices.
As the web continues to evolve, the synergy between frontend and backend development remains critical in creating robust, efficient, and user-friendly web applications. By appreciating the distinct roles and the importance of collaboration between these two areas, developers can contribute to building the next generation of innovative web solutions.