Front-End vs Back-End Development: Understanding the Key Differences
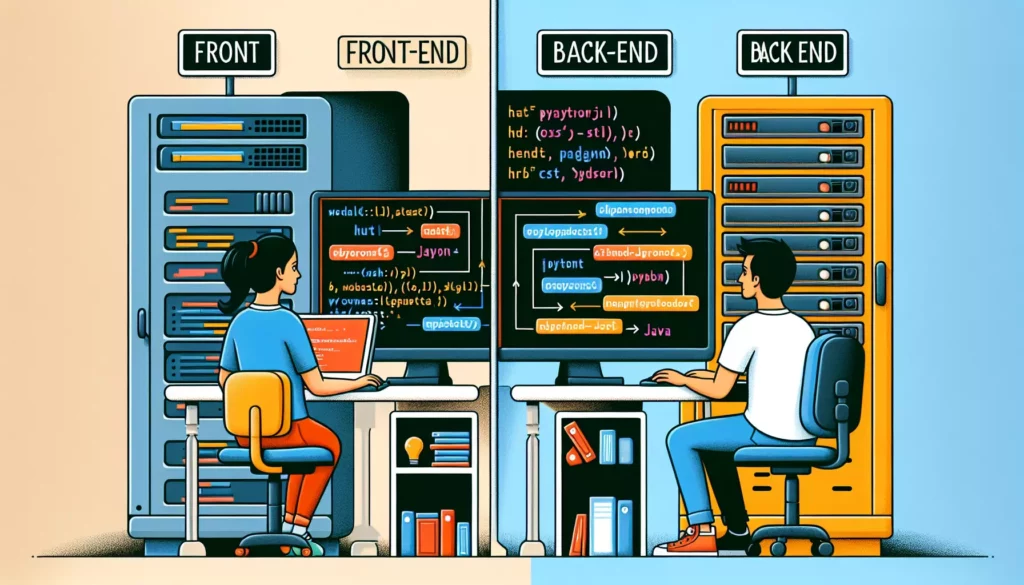
In the vast landscape of web development, two primary domains stand out: front-end and back-end development. While both are crucial for creating functional and user-friendly websites and applications, they serve different purposes and require distinct skill sets. This comprehensive guide will delve into the intricacies of front-end and back-end development, highlighting their differences, similarities, and the roles they play in the world of coding.
What is Front-End Development?
Front-end development, also known as client-side development, focuses on creating the user interface and user experience of a website or application. It’s the part of the website that users directly interact with, including the layout, design, and interactive elements.
Key Responsibilities of Front-End Developers
- Implementing visual elements that users see and interact with
- Ensuring website responsiveness and compatibility across different devices and browsers
- Optimizing site performance for speed and efficiency
- Creating and maintaining user-friendly interfaces
- Collaborating with designers and back-end developers
Essential Front-End Technologies
Front-end developers typically work with the following technologies:
- HTML (Hypertext Markup Language): The backbone of web content structure
- CSS (Cascading Style Sheets): Used for styling and layout
- JavaScript: Enables interactive and dynamic content
- Frameworks and libraries like React, Angular, or Vue.js
- Responsive design techniques
- Version control systems like Git
Example of Front-End Code
Here’s a simple example of HTML, CSS, and JavaScript working together to create a button that changes color when clicked:
<!-- HTML -->
<button id="colorButton">Click me!</button>
<!-- CSS -->
<style>
#colorButton {
background-color: blue;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
</style>
<!-- JavaScript -->
<script>
document.getElementById("colorButton").addEventListener("click", function() {
this.style.backgroundColor = "red";
});
</script>
What is Back-End Development?
Back-end development, also referred to as server-side development, focuses on the behind-the-scenes functionality of web applications. It involves working with servers, databases, and application logic to ensure that everything on the front-end works smoothly.
Key Responsibilities of Back-End Developers
- Building and maintaining the server-side of web applications
- Creating and managing databases
- Developing APIs (Application Programming Interfaces)
- Ensuring server, application, and database communicate efficiently
- Implementing security measures and data protection
- Optimizing the application for speed and scalability
Essential Back-End Technologies
Back-end developers typically work with:
- Server-side programming languages like Python, Java, Ruby, or PHP
- Databases such as MySQL, MongoDB, or PostgreSQL
- Server technologies like Node.js, Apache, or Nginx
- APIs and web services
- Cloud platforms like AWS, Google Cloud, or Azure
- Version control systems
Example of Back-End Code
Here’s a simple example of a Python function that could be part of a back-end API to retrieve user data from a database:
import mysql.connector
def get_user_data(user_id):
try:
connection = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
cursor = connection.cursor()
query = "SELECT * FROM users WHERE id = %s"
cursor.execute(query, (user_id,))
user_data = cursor.fetchone()
return user_data
except mysql.connector.Error as error:
print(f"Error retrieving user data: {error}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
Key Differences Between Front-End and Back-End Development
Now that we’ve explored the basics of both front-end and back-end development, let’s highlight the key differences between these two domains:
1. Focus and Visibility
- Front-End: Focuses on the visual and interactive aspects that users directly engage with. It’s the “face” of the application.
- Back-End: Deals with the behind-the-scenes functionality, data management, and server operations. Users don’t directly interact with or see this part.
2. Programming Languages
- Front-End: Primarily uses HTML, CSS, and JavaScript, along with various frameworks and libraries built on these technologies.
- Back-End: Utilizes a wide range of languages such as Python, Java, Ruby, PHP, C#, and others, depending on the specific requirements of the project.
3. Tools and Frameworks
- Front-End: Employs tools like React, Angular, Vue.js, Bootstrap, and Sass to enhance development efficiency and capabilities.
- Back-End: Uses frameworks and tools like Django (Python), Ruby on Rails, Express.js (Node.js), and Spring (Java) to streamline server-side development.
4. Databases
- Front-End: Generally doesn’t interact directly with databases, although modern front-end frameworks may include some data management features.
- Back-End: Works extensively with databases, both relational (like MySQL) and non-relational (like MongoDB), to store, retrieve, and manage data.
5. Performance Optimization
- Front-End: Focuses on optimizing load times, minimizing HTTP requests, and ensuring smooth user interactions.
- Back-End: Concentrates on server response times, database query optimization, and efficient data processing.
6. Security Concerns
- Front-End: Deals with client-side security issues like Cross-Site Scripting (XSS) prevention and securing API calls.
- Back-End: Handles more critical security aspects such as authentication, data encryption, protection against SQL injection, and overall system security.
7. Scalability Considerations
- Front-End: Focuses on creating responsive designs that work across various devices and screen sizes.
- Back-End: Deals with scaling the application to handle increased loads, often involving concepts like load balancing and distributed systems.
The Intersection: Full-Stack Development
While front-end and back-end development are distinct domains, there’s a growing demand for developers who can work on both sides of web development. These professionals are known as full-stack developers.
What is Full-Stack Development?
Full-stack development involves working with both the front-end and back-end of a web application. A full-stack developer is proficient in:
- Front-end technologies (HTML, CSS, JavaScript, etc.)
- Back-end programming (e.g., Python, Java, Node.js)
- Database management
- Server configuration
- API development and integration
Full-stack developers have a comprehensive understanding of how all the parts of a web application work together, allowing them to oversee projects from conception to completion.
Advantages of Full-Stack Development
- Versatility in handling various aspects of web development
- Better understanding of how different parts of an application interact
- Ability to work on smaller projects independently
- Enhanced problem-solving skills due to broader knowledge
- Valuable for startups and small teams where resources are limited
Choosing Your Path: Front-End, Back-End, or Full-Stack?
For those embarking on a career in web development, choosing between front-end, back-end, or full-stack development can be challenging. Here are some factors to consider:
Front-End Development Might Be for You If:
- You have a keen eye for design and user experience
- You enjoy working on visual aspects and creating intuitive interfaces
- You’re interested in the latest design trends and user interaction patterns
- You like seeing immediate results of your work in the browser
Back-End Development Might Be for You If:
- You enjoy working with data and solving complex logical problems
- You’re interested in system architecture and database design
- You like the idea of building the “engine” that powers applications
- You have a strong interest in security and performance optimization
Full-Stack Development Might Be for You If:
- You want a comprehensive understanding of web development
- You enjoy variety in your work and learning multiple technologies
- You’re interested in overseeing entire projects from start to finish
- You’re aiming for versatility in your career options
The Role of AlgoCademy in Your Development Journey
Whether you’re leaning towards front-end, back-end, or full-stack development, platforms like AlgoCademy play a crucial role in helping you develop the necessary skills and knowledge. Here’s how AlgoCademy can assist you in your journey:
1. Comprehensive Curriculum
AlgoCademy offers a wide range of courses covering various aspects of programming, from basic syntax to advanced algorithms. This comprehensive approach ensures that you have a solid foundation, regardless of which development path you choose.
2. Interactive Coding Tutorials
The platform provides hands-on coding experiences through interactive tutorials. This practical approach is crucial for both front-end and back-end development, allowing you to apply theoretical knowledge in real-world scenarios.
3. Focus on Algorithmic Thinking
AlgoCademy places a strong emphasis on algorithmic thinking and problem-solving skills. These are fundamental abilities that benefit both front-end and back-end developers, enhancing your capacity to create efficient and optimized code.
4. Preparation for Technical Interviews
With a focus on preparing learners for interviews at major tech companies, AlgoCademy helps you develop the skills and confidence needed to excel in technical interviews, whether you’re aiming for a front-end, back-end, or full-stack position.
5. AI-Powered Assistance
The AI-powered features of AlgoCademy provide personalized guidance and feedback, helping you identify areas for improvement and accelerating your learning process in your chosen development path.
6. Diverse Learning Resources
By offering a variety of resources, AlgoCademy caters to different learning styles and preferences. This diverse approach ensures that whether you’re visual learner or prefer hands-on practice, you’ll find resources that suit your learning style.
Conclusion: Bridging the Gap Between Front-End and Back-End
While front-end and back-end development are distinct domains with their own unique challenges and skill sets, understanding both sides of web development can significantly enhance your capabilities as a developer. Whether you choose to specialize in one area or pursue full-stack development, having a comprehensive understanding of how web applications work from end to end will make you a more effective and versatile professional.
As you embark on your journey in web development, remember that platforms like AlgoCademy are invaluable resources. They provide structured learning paths, hands-on experience, and the guidance needed to navigate the complex world of coding. Whether you’re building beautiful, responsive user interfaces or crafting robust server-side applications, the skills you develop through dedicated learning and practice will set you on the path to success in the ever-evolving field of web development.
Ultimately, the choice between front-end, back-end, or full-stack development depends on your interests, strengths, and career goals. Whichever path you choose, remember that continuous learning and adaptability are key in the fast-paced world of technology. Embrace the challenges, stay curious, and let your passion for coding guide you as you create the digital experiences of tomorrow.