Why You’re Watching Coding Tutorials but Still Can’t Build Anything
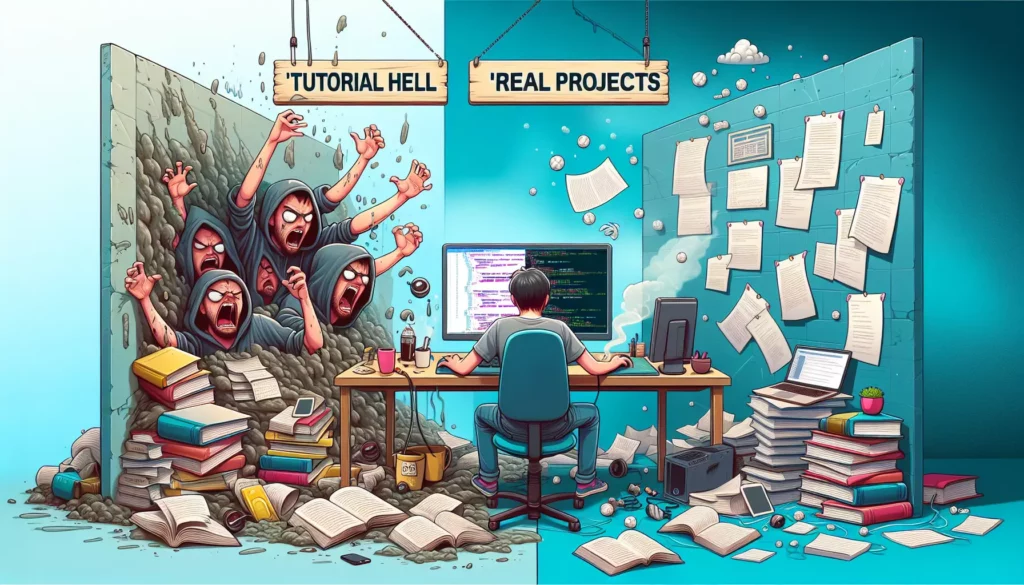
The Tutorial Trap: Why Watching Isn’t the Same as Building
You’ve spent countless hours watching coding tutorials. Your YouTube history is filled with “Learn JavaScript in 10 Hours” and “Build a Full-Stack App in a Weekend.” You’ve bookmarked dozens of courses on Udemy and followed countless programming influencers.
Yet, when you sit down to build something on your own, you freeze. The blank screen mocks you. Your fingers hover uncertainly over the keyboard. Suddenly, all that knowledge seems to evaporate.
If this sounds familiar, you’re caught in what I call the “tutorial trap” – and you’re not alone. This phenomenon affects beginners and intermediate programmers alike, creating a frustrating cycle of consumption without creation.
In this post, we’ll explore why simply watching coding tutorials rarely translates to actual coding ability, and more importantly, how to break free from this cycle to become a confident, capable developer who can build real projects.
Passive Learning vs. Active Building: Understanding the Difference
The core problem with relying on tutorials is that they provide a passive learning experience. When you watch someone else code, your brain operates in a different mode than when you’re solving problems yourself.
Consider these fundamental differences:
- Tutorial watching activates recognition memory (“I’ve seen this before”)
- Building projects requires recall memory (“I need to produce this knowledge myself”)
This distinction is crucial. Recognition is far easier than recall – it’s the difference between being able to recognize a face (easy) versus describing a face to a sketch artist (difficult).
When tutorial creators explain concepts and write code, they make it look effortless. They know exactly which files to modify, which functions to call, and how to fix errors that pop up. This creates an “illusion of competence” – you feel like you understand because everything makes sense when someone explains it.
But programming isn’t about understanding explanations; it’s about solving problems when no one is there to guide you.
The False Comfort of Tutorial Completionism
Many aspiring developers fall into the trap of “tutorial completionism” – the belief that if they just finish one more course or watch one more video series, they’ll finally be ready to build on their own.
This mindset is particularly dangerous because:
- It creates a false sense of progress (you’re completing things, after all)
- It postpones the discomfort of independent problem-solving
- It can become an endless cycle as new technologies and tutorials constantly emerge
Each completed tutorial gives you a dopamine hit – a sense of accomplishment without the struggle of independent creation. This can become addictive, leading to what I call “tutorial dependency syndrome.”
The hard truth is that no amount of passive learning will prepare you completely for building your own projects. There will always be a gap between following along and creating independently.
The Copy-Paste Syndrome: Why Code Mimicry Fails
Another common pattern among tutorial-watchers is the tendency to copy code without truly understanding it. This approach might help you complete the tutorial project, but it does little to build your independent coding skills.
Here’s a typical scenario: You follow a React tutorial to build a to-do app. The instructor writes:
const [todos, setTodos] = useState([]);
const addTodo = (text) => {
const newTodos = [...todos, { text, completed: false, id: Date.now() }];
setTodos(newTodos);
}
You copy this code, and it works! But do you truly understand what the spread operator does here? Could you explain why we’re using Date.now()
for the ID? Do you grasp the principles of immutability that inform how React state updates work?
Without this deeper understanding, you’ll struggle when trying to build something slightly different from the tutorial example. You’ve memorized specific solutions rather than internalized the underlying principles.
The “Tutorial Bubble” and Real-World Coding
Tutorials exist in a carefully constructed bubble designed to minimize friction and maximize learning efficiency. This is both their strength and their limitation.
In the tutorial world:
- Projects are perfectly scoped for teaching specific concepts
- All required technologies and versions are pre-selected
- Common errors are anticipated and addressed
- The path from start to finish is clearly mapped
In the real world:
- You must define project scope yourself (often the hardest part)
- You need to choose between competing technologies and versions
- You’ll face unexpected errors with minimal guidance
- The path forward is rarely clear and requires constant decision-making
This disconnect creates a shock when you move from tutorials to independent building. Suddenly, you’re not just coding – you’re making architectural decisions, debugging unexpected issues, and navigating ambiguity.
The Missing Skills That Tutorials Don’t Teach
Beyond the code itself, there are crucial meta-skills that most tutorials don’t explicitly teach:
1. Problem Decomposition
In tutorials, the big problem is already broken down into manageable steps. In real development, you need to take a vague idea like “I want to build a recipe app” and decompose it into specific technical tasks:
- Design a database schema for recipes and ingredients
- Create a form component for recipe submission
- Implement search functionality with filters
- Build a responsive recipe display component
This ability to break down problems is often more valuable than knowing specific syntax.
2. Effective Debugging
Tutorial projects rarely go wrong in unexpected ways. When they do, the instructor immediately knows what’s wrong. In contrast, independent coding involves spending hours debugging issues that no tutorial prepared you for.
Learning to read error messages, use debugging tools, and systematically isolate problems is a skill developed through practice, not passive watching.
3. Reading Documentation
Tutorials pre-digest documentation for you, presenting only the relevant parts in an accessible way. Building independently requires combing through official docs, GitHub issues, and Stack Overflow posts to find solutions.
This skill – knowing how to find and interpret technical information – is essential but rarely explicitly taught.
4. Making Technical Decisions
Should you use Redux or Context API? CSS modules or styled-components? MongoDB or PostgreSQL? In tutorials, these decisions are made for you. In real projects, you must weigh tradeoffs and make architectural choices that affect the entire project.
The Emotional Journey: Why Building Is Psychologically Harder
Beyond the technical aspects, there’s a significant psychological dimension to the tutorial-to-building gap.
Tutorials offer a structured, guided experience with regular validation. Building independently means confronting:
- Uncertainty: Not knowing if your approach will work
- Frustration: Spending hours on problems that seem simple
- Impostor syndrome: Questioning your abilities when you get stuck
- Decision fatigue: Making countless choices without clear “right answers”
This emotional component explains why many developers retreat to the comfort of tutorials when independent building gets tough. It’s not just about technical knowledge – it’s about developing the resilience to push through the uncomfortable phases of creation.
Breaking the Cycle: From Tutorial Consumer to Project Builder
Now that we understand the problem, let’s focus on solutions. How can you transform from a passive tutorial consumer to an active project builder?
1. Embrace the “Modification First” Approach
Instead of jumping straight from tutorials to building from scratch, start by modifying tutorial projects. After completing a tutorial:
- Add a new feature not covered in the tutorial
- Change the styling completely
- Refactor the code to use a different approach
- Replace a library used with an alternative
This creates a middle ground between following instructions and building independently. You have a working codebase to start with, but you’re forced to understand it well enough to modify it successfully.
For example, if you’ve built a to-do app in a tutorial, try adding categories, due dates, or priority levels without guidance.
2. Practice Active Learning During Tutorials
Transform how you consume tutorials by adopting active learning techniques:
- Code before watching: Try to implement a feature before seeing the solution
- Pause and predict: Regularly pause videos and predict what code comes next
- Take handwritten notes: Summarize concepts in your own words
- Verbalize explanations: Explain what the code does out loud as if teaching someone
These techniques force your brain to engage more deeply with the material, moving from recognition to recall.
3. Build Deliberately Simplified Projects
When starting independent projects, scope is everything. Many beginners fail because they attempt projects that are too complex.
Instead, start with deliberately simplified versions of your project ideas:
- Want to build a social media app? Start with just the user profile page
- Dream of creating an e-commerce platform? Begin with just the product listing
- Planning a blog? First build only the single post view
This approach lets you experience the full development cycle (planning, building, debugging, deploying) without getting overwhelmed by scope.
4. Embrace the “Build in Public” Mindset
One of the most powerful ways to transition from tutorial-watching to building is to commit to building in public. This means:
- Sharing your progress on Twitter, LinkedIn, or GitHub
- Documenting your learning journey through blog posts
- Joining communities where you can showcase your work
Public accountability provides motivation during difficult phases and often leads to valuable feedback from experienced developers.
5. Develop a Debugging Mindset
Instead of seeing errors as failures, reframe them as the primary way you learn. Develop a systematic approach to debugging:
- Read the error message carefully (don’t just scan it)
- Check the line number and surrounding code
- Verify your assumptions with console.log() or debugger statements
- Search for the error message online with context
- Break down the problem into smaller parts to isolate the issue
The more comfortable you become with debugging, the less intimidating independent building becomes.
The Project-Based Learning Framework
To make the transition from tutorials to projects more structured, consider adopting this framework for your next learning endeavor:
Phase 1: Guided Learning (20% of your time)
- Complete a focused tutorial on the main technology you need
- Take active notes on key concepts and patterns
- Build the tutorial project alongside the instructor
Phase 2: Guided Exploration (30% of your time)
- Modify the tutorial project with new features
- Rebuild the same project from memory without following along
- Experiment with alternative approaches to the same problem
Phase 3: Scaffolded Building (50% of your time)
- Design a simple original project using similar technologies
- Break it down into small, manageable tasks
- Build incrementally, seeking help only after attempting solutions
- Document your process and learnings
This framework gradually shifts the balance from consumption to creation, building your confidence along the way.
Practical Examples: From Tutorial to Real Project
Let’s see how this might work in practice with a common learning path:
Learning React: The Tutorial Trap Way
- Watch a 12-hour React course on YouTube
- Complete a Udemy course on React hooks
- Follow a tutorial to build a Netflix clone
- Start another course on React with TypeScript
- Watch videos on React performance optimization
- Never actually build anything independently
Learning React: The Project-Based Way
- Complete one focused React fundamentals course
- Modify the course project to add two new features
- Build a simple weather app with React (minimal features, but complete)
- Share your weather app on GitHub and get feedback
- Add one advanced feature to your weather app (like location detection)
- Build a slightly more complex project based on what you’ve learned
The second approach involves less content consumption but much more active learning and building, leading to genuine skill development.
Common Obstacles and How to Overcome Them
Even with the best strategies, you’ll face obstacles in your journey from tutorial consumer to project builder. Here’s how to handle the most common ones:
1. The Blank Canvas Paralysis
Obstacle: You don’t know where to start when facing an empty project.
Solution: Start with structure, not code. Create the basic files and folders for your project. Set up a simple “Hello World” version that just renders on screen. Breaking the initial inertia is often the hardest part.
2. The “I Don’t Know Enough Yet” Myth
Obstacle: You feel you need to learn more before starting your project.
Solution: Adopt a “learn as you build” mindset. Start with what you know, and when you hit a knowledge gap, learn just enough to solve that specific problem. This targeted learning is far more effective than broad pre-learning.
3. The Perfectionism Trap
Obstacle: You want your code to be as polished as what you see in tutorials.
Solution: Embrace the concept of the “ugly first draft.” Give yourself permission to write messy, inefficient code for the first version. You can refactor once the functionality works.
4. Tutorial Withdrawal
Obstacle: You feel uncomfortable without the guidance of a tutorial.
Solution: Create your own “micro-tutorials” by breaking your project into small tasks and researching just those specific elements. Instead of “How to build a blog with React,” search for “How to create a comment form in React.”
5. The Comparison Trap
Obstacle: Your projects don’t look as impressive as tutorials or other developers’ work.
Solution: Remember that tutorials show the final, polished product, not the messy process. Also, most tutorial projects have been built and refined many times by the instructor. Your first independent project will never match this – and that’s perfectly normal.
Beyond Tutorials: Building a Sustainable Learning Process
The ultimate goal isn’t just to break free from tutorial dependency for one project – it’s to develop a sustainable, long-term approach to learning and building.
Create a Learning Flywheel
The most effective developers create what I call a “learning flywheel” – a self-reinforcing cycle that builds momentum over time:
- Learn the minimum needed to start building
- Build something using what you learned
- Encounter problems you don’t know how to solve
- Research specific solutions to those problems
- Implement the solutions, deepening your understanding
- Reflect on what you learned and identify knowledge gaps
- Repeat the cycle with increasingly complex projects
This approach creates a virtuous cycle where building and learning reinforce each other, rather than being separate activities.
Balanced Learning Diet
Just as a balanced diet is crucial for physical health, a balanced learning diet is essential for developer growth. Aim for:
- 20% guided learning (tutorials, courses, books)
- 40% building projects (applying what you’ve learned)
- 20% reading code (studying open-source projects, reviewing others’ code)
- 10% teaching others (explaining concepts solidifies understanding)
- 10% reflection (reviewing your progress and adjusting your approach)
This balance ensures you’re not just consuming content but actively processing and applying it in different ways.
The Spaced Repetition Approach to Coding Skills
One of the most effective techniques for moving knowledge from passive recognition to active recall is spaced repetition – returning to concepts at increasing intervals.
Apply this to your coding journey by:
- Learning a concept (e.g., React hooks)
- Implementing it in a simple project immediately
- Coming back to it in a different project 2-3 days later
- Using it again in a more complex way 1-2 weeks later
- Challenging yourself to use it in an advanced scenario a month later
This approach aligns with how our brains form long-term memories and builds the neural pathways needed for fluent recall when building projects.
From Consumer to Creator: The Mindset Shift
Perhaps the most important transformation isn’t technical but psychological – shifting from seeing yourself as a content consumer to identifying as a creator and problem-solver.
This identity shift manifests in how you approach learning:
Consumer Mindset | Creator Mindset |
---|---|
“I need to finish this course before I can build anything” | “I’ll learn enough to start, then learn more as I build” |
“I don’t know how to do this yet” | “I don’t know how to do this YET, but I can figure it out” |
“This error means I’m doing something wrong” | “This error is teaching me something important” |
“My code doesn’t look like the tutorial” | “There are many ways to solve this problem” |
“I completed another tutorial” | “I built something that works” |
Notice how the creator mindset embraces challenges, values output over input, and focuses on problem-solving rather than perfect replication.
Case Study: How I Broke Free from Tutorial Hell
Let me share a personal story about my own journey from tutorial addiction to independent building.
After six months of JavaScript tutorials, I could explain closures, promises, and the event loop – but I couldn’t build a simple app without following step-by-step instructions. I was stuck in tutorial hell.
My breakthrough came when I set a strict rule: for every hour of tutorials, I would spend two hours building. At first, it was incredibly uncomfortable. I stared at empty files, wrote clumsy code, and spent hours debugging simple problems.
But gradually, something changed. I started recognizing patterns. Problems that once seemed insurmountable became manageable. My debugging skills improved dramatically. Most importantly, I developed the confidence that I could figure things out without external guidance.
The key wasn’t learning more – it was applying what I already knew, even imperfectly. Each small success built momentum until building became more comfortable than passively watching.
The 30-Day Tutorial Detox Challenge
If you’re ready to break free from tutorial dependency, I challenge you to try this 30-day “tutorial detox” program:
Week 1: Minimal Input, Maximum Output
- Limit yourself to 30 minutes of tutorials per day
- Spend at least 1 hour building each day
- Start a simple project using technologies you’ve already learned
- Document your daily progress and challenges
Week 2: Strategic Problem-Solving
- Continue with 30 minutes maximum tutorial time
- Increase building time to 90 minutes daily
- When stuck, write down the specific problem before searching for solutions
- Try to solve each problem for at least 20 minutes before seeking help
Week 3: Building Momentum
- Reduce tutorials to only 20 minutes of targeted learning
- Maintain 90+ minutes of building time
- Add one new feature to your project each day, no matter how small
- Share your progress with at least one other person
Week 4: Independence
- Tutorials only as needed for specific problems
- 2+ hours of building time daily
- Complete your project to a sharable state (doesn’t need to be perfect)
- Publish your project and share what you learned
This structured approach gradually weans you off tutorial dependency while building the confidence and skills needed for independent development.
Conclusion: The Builder’s Path Forward
The gap between watching tutorials and building projects isn’t primarily about knowledge – it’s about practice, mindset, and the development of meta-skills that only come through active creation.
Remember these key principles as you move forward:
- Start small but start building as soon as possible
- Embrace the struggle – it’s where the most valuable learning happens
- Build a support system of other learners and more experienced developers
- Celebrate progress, not perfection
- Trust the process – building skills takes time and consistent effort
The path from tutorial consumer to confident builder isn’t easy, but it’s immensely rewarding. Each project you complete, no matter how small or imperfect, represents real growth in your capabilities.
So close that tutorial tab (after finishing this article, of course), open your code editor, and start building. Your future self – the one who can confidently create rather than just consume – will thank you.
What small project will you start building today? Share your commitment in the comments, and let’s hold each other accountable for creating, not just consuming.