From Nutella to Algorithms: A Tasty Journey into Coding on the Beaches of Tenerife
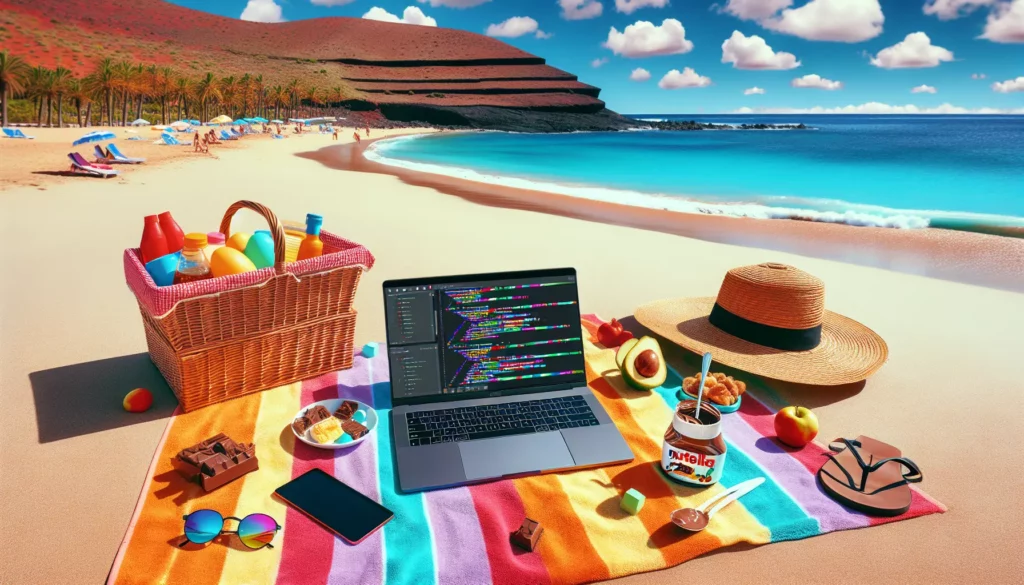
Welcome, fellow coding enthusiasts and curious minds! Today, we’re embarking on a unique adventure that combines the sweetness of Nutella, the sunny shores of Tenerife, and the exciting world of programming. Buckle up as we explore how these seemingly unrelated topics can come together to create a delicious coding experience that’s as rich and satisfying as your favorite chocolate-hazelnut spread.
The AlgoCademy Approach: Blending Fun and Learning
Before we dive into our Nutella-infused coding journey, let’s take a moment to appreciate the philosophy behind AlgoCademy. This innovative platform is revolutionizing the way we approach coding education and programming skills development. By providing interactive tutorials, comprehensive resources, and AI-powered assistance, AlgoCademy is making the path from beginner to interview-ready professional smoother than ever.
Just as Nutella transforms a simple piece of toast into a gourmet treat, AlgoCademy takes the sometimes daunting world of coding and turns it into an accessible, enjoyable experience. With a focus on algorithmic thinking and problem-solving, learners can develop practical skills that are crucial for success in the tech industry, particularly when aiming for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
Coding with a View: Why Tenerife?
Now, you might be wondering, “Why Tenerife?” Well, imagine learning to code while surrounded by pristine beaches, breathtaking landscapes, and a relaxed island atmosphere. Tenerife, the largest of Spain’s Canary Islands, offers the perfect backdrop for a unique coding retreat. The island’s diverse environments – from sandy shores to lush forests and even a volcano – mirror the varied landscapes of programming languages and paradigms we’ll explore.
Just as Tenerife attracts visitors from all over the world, the field of programming brings together people from diverse backgrounds, united by their passion for problem-solving and innovation. So, let’s set up our laptops on a sunny beach and start our coding adventure!
The Nutella Connection: Sweet Analogies for Coding Concepts
You might be surprised to learn that Nutella can teach us a lot about programming concepts. Let’s explore some sweet analogies that will make coding principles stick in your mind like Nutella to the roof of your mouth!
1. Variables: The Jars of Our Code
Think of variables in programming as jars of Nutella. Just as you can store different amounts of Nutella in jars of various sizes, variables allow us to store different types and amounts of data in our code. Let’s see how we might declare a Nutella-themed variable in Python:
nutella_grams = 200
jar_size = "medium"
is_delicious = True
print(f"This {jar_size} jar contains {nutella_grams}g of Nutella. Is it delicious? {is_delicious}")
In this example, we’ve created three variables: a number (integer), a string, and a boolean. Just like different Nutella jars can hold various amounts and types of spreads, our variables can hold different types of data.
2. Functions: The Recipe for Nutella Magic
If variables are like jars of Nutella, then functions are like recipes for creating delicious Nutella-based treats. Functions allow us to package a set of instructions that we can use repeatedly, just like how a recipe lets us create the same delicious snack over and over. Let’s write a simple function that calculates how many sandwiches we can make with a given amount of Nutella:
def calculate_sandwiches(nutella_grams, grams_per_sandwich):
return nutella_grams // grams_per_sandwich
nutella_in_jar = 400
grams_needed = 20
sandwiches = calculate_sandwiches(nutella_in_jar, grams_needed)
print(f"You can make {sandwiches} sandwiches with {nutella_in_jar}g of Nutella!")
This function takes two parameters: the amount of Nutella we have and how much we need for each sandwich. It then calculates and returns the number of sandwiches we can make. Just like how a recipe can be adjusted for different quantities, our function can be used with various amounts of Nutella.
3. Loops: Spreading Nutella Efficiently
Imagine you’re making Nutella sandwiches for a crowd on Tenerife’s beautiful Playa de Las Teresitas. You wouldn’t want to write out the instructions for each sandwich individually. This is where loops come in handy! Loops in programming allow us to repeat actions efficiently, just like how you’d use the same motion to spread Nutella on multiple slices of bread.
Let’s use a for loop to make our sandwich-making process more efficient:
def make_nutella_sandwich():
print("Spread Nutella on bread")
print("Add banana slices")
print("Close sandwich")
print("Enjoy your Nutella banana sandwich!")
number_of_sandwiches = 5
for i in range(number_of_sandwiches):
print(f"Making sandwich #{i+1}")
make_nutella_sandwich()
print("--------------------")
This code will efficiently “make” five Nutella banana sandwiches, demonstrating how loops can save us time and effort in programming, just as they do in sandwich making!
Tenerife-Inspired Coding Projects
Now that we’ve covered some basic programming concepts using our Nutella analogy, let’s apply these skills to some Tenerife-inspired coding projects. These projects will not only help reinforce what we’ve learned but also give us a taste of real-world application development.
Project 1: Tenerife Weather Predictor
Tenerife is known for its microclimates, which can make weather prediction challenging and interesting. Let’s create a simple weather prediction program using conditional statements:
def predict_weather(temperature, humidity, wind_speed):
if temperature > 25 and humidity < 60 and wind_speed < 15:
return "Perfect beach day in Tenerife!"
elif temperature > 20 and humidity < 70:
return "Good day for hiking in Teide National Park."
elif wind_speed > 20:
return "Windy day - great for surfing at El Médano!"
else:
return "Enjoy a museum day or visit the Loro Parque."
# Example usage
today_temp = 28
today_humidity = 55
today_wind = 10
forecast = predict_weather(today_temp, today_humidity, today_wind)
print(f"Today's forecast: {forecast}")
This simple program uses conditional statements to predict activities based on weather conditions, showcasing how we can use programming to solve real-world problems.
Project 2: Nutella Recipe Calculator
Let’s create a more complex program that calculates ingredient quantities for a Nutella-based recipe, adjusting for the number of servings:
class NutellaRecipe:
def __init__(self, name, base_servings, ingredients):
self.name = name
self.base_servings = base_servings
self.ingredients = ingredients
def calculate_ingredients(self, desired_servings):
factor = desired_servings / self.base_servings
adjusted_ingredients = {}
for ingredient, amount in self.ingredients.items():
adjusted_ingredients[ingredient] = amount * factor
return adjusted_ingredients
def print_recipe(self, desired_servings):
print(f"Recipe for {self.name} (Servings: {desired_servings})")
adjusted = self.calculate_ingredients(desired_servings)
for ingredient, amount in adjusted.items():
print(f"{ingredient}: {amount:.2f}")
# Define a Nutella banana smoothie recipe
smoothie = NutellaRecipe("Nutella Banana Smoothie", 2, {
"Nutella": 60, # in grams
"Banana": 1, # in pieces
"Milk": 250, # in ml
"Ice cubes": 4 # in pieces
})
# Calculate and print recipe for 6 servings
smoothie.print_recipe(6)
This program demonstrates the use of classes, methods, and dictionaries to create a flexible recipe calculator. It’s perfect for when you’re planning a Nutella-themed party on the beaches of Tenerife!
Advancing Your Coding Skills: From Nutella to Algorithms
As we progress from our Nutella-inspired basics to more advanced concepts, it’s important to remember that mastering programming is a journey. Just as perfecting a Nutella recipe takes practice, becoming proficient in coding requires dedication and consistent effort.
Data Structures: Organizing Our Nutella Pantry
In programming, data structures are ways of organizing and storing data. Think of them as different ways to arrange your Nutella jars in a pantry. Let’s explore a few common data structures:
1. Lists (Arrays)
Lists are like shelves in your pantry where you can store multiple items in a specific order. In Python, we can create a list of Nutella-based snacks like this:
nutella_snacks = ["Nutella sandwich", "Nutella crepe", "Nutella smoothie", "Nutella ice cream"]
# Accessing items
print(nutella_snacks[0]) # Outputs: Nutella sandwich
# Adding an item
nutella_snacks.append("Nutella pancakes")
# Removing an item
nutella_snacks.remove("Nutella crepe")
print(nutella_snacks)
2. Dictionaries (Hash Tables)
Dictionaries are like labeled jars in your pantry. Each item (value) is associated with a unique label (key). Let’s use a dictionary to store Nutella recipes:
nutella_recipes = {
"sandwich": ["bread", "Nutella"],
"smoothie": ["Nutella", "banana", "milk", "ice"],
"crepe": ["crepe", "Nutella", "strawberries"]
}
# Accessing recipes
print(nutella_recipes["smoothie"])
# Adding a new recipe
nutella_recipes["fondue"] = ["Nutella", "cream", "fruits for dipping"]
# Updating a recipe
nutella_recipes["sandwich"].append("banana slices")
print(nutella_recipes)
Algorithms: The Secret Recipes of Programming
Algorithms are like the secret recipes that make our code efficient and effective. They are step-by-step procedures for solving problems or performing tasks. Let’s look at a simple sorting algorithm to arrange our Nutella snacks alphabetically:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
nutella_snacks = ["Nutella ice cream", "Nutella sandwich", "Nutella crepe", "Nutella smoothie"]
sorted_snacks = bubble_sort(nutella_snacks)
print("Sorted Nutella snacks:", sorted_snacks)
This bubble sort algorithm demonstrates how we can systematically solve problems in programming. As you advance in your coding journey, you’ll encounter more complex algorithms for searching, sorting, and optimizing data.
Bringing It All Together: A Tenerife Nutella Coding Challenge
Now that we’ve explored various programming concepts through the lens of Nutella and Tenerife, let’s challenge ourselves with a more complex project that brings everything together. We’ll create a Tenerife Nutella Food Truck simulator!
import random
class NutellaFoodTruck:
def __init__(self):
self.menu = {
"Nutella Sandwich": 5,
"Nutella Crepe": 7,
"Nutella Smoothie": 6,
"Nutella Ice Cream": 4
}
self.inventory = {item: 20 for item in self.menu}
self.cash = 100
def display_menu(self):
print("\nMenu:")
for item, price in self.menu.items():
print(f"{item}: €{price}")
def take_order(self):
self.display_menu()
order = input("What would you like to order? ").title()
if order in self.menu:
if self.inventory[order] > 0:
self.inventory[order] -= 1
self.cash += self.menu[order]
print(f"Enjoy your {order}!")
else:
print(f"Sorry, we're out of {order}.")
else:
print("Sorry, we don't have that item.")
def restock(self):
for item in self.inventory:
restock_amount = random.randint(5, 10)
self.inventory[item] += restock_amount
self.cash -= restock_amount # Assume €1 cost per item to restock
print("Inventory restocked!")
def daily_report(self):
print("\nDaily Report:")
print(f"Cash: €{self.cash}")
print("Inventory:")
for item, quantity in self.inventory.items():
print(f"{item}: {quantity}")
def run_food_truck():
truck = NutellaFoodTruck()
days = 7
for day in range(1, days + 1):
print(f"\n--- Day {day} ---")
customers = random.randint(5, 15)
for _ in range(customers):
truck.take_order()
truck.restock()
truck.daily_report()
run_food_truck()
This Nutella Food Truck simulator incorporates many of the concepts we’ve discussed:
- Classes and objects to represent the food truck
- Dictionaries to store the menu and inventory
- Functions to handle various operations like taking orders and restocking
- Loops to simulate multiple days of operation
- Conditional statements to check inventory and validate orders
- Random number generation to simulate customer visits and restocking
By running this simulation, you can see how all these programming concepts come together to create a more complex and interactive program.
Conclusion: Your Coding Journey Continues
As we wrap up our Nutella-infused, Tenerife-inspired coding adventure, remember that this is just the beginning of your programming journey. The concepts we’ve explored – from variables and functions to data structures and algorithms – form the foundation of computer science and software development.
Just as Tenerife offers a diverse landscape to explore, the world of programming is vast and varied. There’s always more to learn, new languages to discover, and exciting projects to undertake. Whether you’re building web applications, developing mobile apps, or diving into data science, the skills you’ve begun to develop here will serve you well.
Remember the AlgoCademy approach: blend learning with fun, tackle problems step-by-step, and never stop exploring. As you continue your coding education, challenge yourself with new projects, collaborate with other developers, and don’t be afraid to get creative with your coding – who knows, you might just come up with the next big Nutella-inspired app!
So, as you enjoy your virtual (or real) Nutella treat on the sunny beaches of Tenerife, take a moment to appreciate how far you’ve come in your coding journey. The world of programming is your oyster, or should we say, your jar of Nutella – rich, rewarding, and full of possibilities. Keep coding, keep learning, and most importantly, keep enjoying the sweet success of solving problems through programming!