From Emoji to Algorithm: Translating Everyday Symbols into Code Concepts
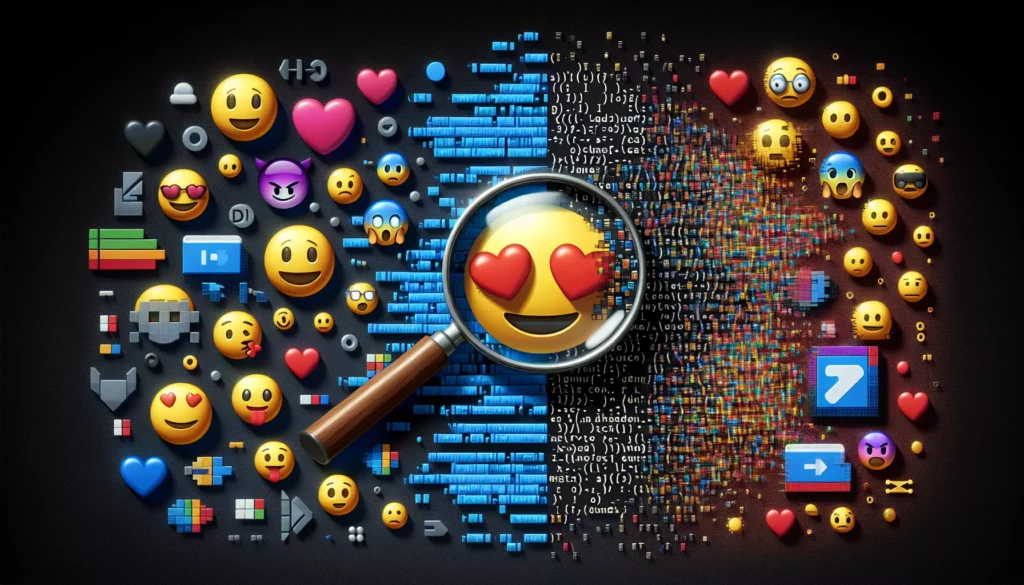
In our increasingly digital world, we’re surrounded by symbols that convey meaning at a glance. From the ubiquitous emojis in our text messages to the icons on our smartphone screens, these visual shorthand elements have become an integral part of our daily communication. But what if we told you that these familiar symbols could be your gateway to understanding complex coding concepts?
Welcome to a journey where we’ll explore how everyday symbols can be translated into fundamental programming ideas. This approach not only makes coding more accessible but also demonstrates how the logical thinking behind programming is already present in our daily lives. Whether you’re a complete beginner or looking to reinforce your coding knowledge, this analogy-driven exploration will help you connect the dots between the familiar and the technical.
1. The Smiley Face Emoji: Functions and Reusability
Let’s start with one of the most recognizable symbols in digital communication: the smiley face emoji 😊. In the world of coding, this cheerful icon can represent the concept of functions.
Why the Connection?
Just as a smiley face emoji conveys a complete emotion with a single symbol, a function in programming encapsulates a set of instructions that perform a specific task. You don’t need to describe your happiness in detail every time; you simply use the emoji. Similarly, once a function is defined, you can call it whenever you need to perform that task, without rewriting the code.
Coding Example:
def smile():
print("😊 Hello, World!")
# Using the function
smile()
smile()
smile()
In this Python example, we’ve defined a function called smile()
that prints a greeting with a smiley face. We can use this function multiple times without rewriting the print statement, just like you can use the smiley emoji multiple times in a conversation without explaining its meaning each time.
2. The Traffic Light: Conditional Statements
The familiar traffic light system with its red, yellow, and green signals is an excellent analogy for conditional statements in programming.
Why the Connection?
Traffic lights guide our actions based on specific conditions. If the light is red, we stop; if it’s green, we go; if it’s yellow, we prepare to stop. This decision-making process mirrors how conditional statements work in programming.
Coding Example:
def traffic_light(color):
if color == "red":
return "Stop!"
elif color == "yellow":
return "Prepare to stop"
elif color == "green":
return "Go!"
else:
return "Invalid color"
print(traffic_light("red")) # Output: Stop!
print(traffic_light("green")) # Output: Go!
This code demonstrates how conditional statements (if
, elif
, else
) in Python can mimic the decision-making process of a traffic light.
3. The Shopping Cart Icon: Data Structures
The shopping cart icon, often seen in e-commerce websites, can be a great way to understand the concept of data structures, particularly lists or arrays.
Why the Connection?
A shopping cart holds multiple items, just like a list in programming can contain multiple elements. You can add items to your cart, remove them, or check what’s inside – operations that are analogous to common list operations in programming.
Coding Example:
shopping_cart = []
# Adding items to the cart
shopping_cart.append("Laptop")
shopping_cart.append("Headphones")
shopping_cart.append("Mouse")
print("Cart contents:", shopping_cart)
# Removing an item
shopping_cart.remove("Headphones")
print("Updated cart:", shopping_cart)
# Checking if an item is in the cart
if "Laptop" in shopping_cart:
print("Laptop is in the cart")
This Python code demonstrates how a list can be used to represent a shopping cart, with operations like adding items (append
), removing items (remove
), and checking for items (in
operator).
4. The Recycling Symbol: Loops and Iteration
The recycling symbol, with its three arrows forming a triangle, is a perfect representation of loops in programming.
Why the Connection?
The recycling process is continuous and repetitive, much like a loop in programming. Each arrow in the symbol can represent a step in the loop, and the circular nature of the symbol reflects how loops repeat until a certain condition is met.
Coding Example:
def recycle(items):
for item in items:
print(f"Recycling {item}...")
# Simulate recycling process
print(f"{item} has been recycled!")
print("All items have been recycled!")
recyclables = ["Paper", "Plastic", "Glass"]
recycle(recyclables)
This code uses a for
loop to iterate through a list of recyclable items, simulating the recycling process for each item.
5. The Puzzle Piece: Object-Oriented Programming
A puzzle piece is an excellent symbol to represent the concept of object-oriented programming (OOP) and how different components fit together to create a complete picture.
Why the Connection?
Each puzzle piece has its own unique shape and part of the overall image, yet it connects with other pieces to form a larger picture. Similarly, in OOP, objects are individual units with their own properties and methods, but they interact with other objects to create complex programs.
Coding Example:
class PuzzlePiece:
def __init__(self, shape, image_part):
self.shape = shape
self.image_part = image_part
def connect(self, other_piece):
print(f"Connecting {self.shape} piece with {other_piece.shape} piece")
def display(self):
print(f"This piece shows {self.image_part} of the image")
# Creating puzzle pieces
corner_piece = PuzzlePiece("corner", "top-left sky")
edge_piece = PuzzlePiece("edge", "cloud")
# Using the pieces
corner_piece.display()
corner_piece.connect(edge_piece)
This example demonstrates how classes in OOP can be used to create objects (puzzle pieces) with their own properties and methods, and how these objects can interact with each other.
6. The Magnifying Glass: Searching and Algorithms
The magnifying glass icon, often used to represent search functionality, can be a great way to introduce the concept of searching algorithms in programming.
Why the Connection?
Just as a magnifying glass helps us find small details in a larger image, search algorithms in programming help us find specific elements within large datasets. The process of moving the magnifying glass over an area is similar to how search algorithms traverse through data.
Coding Example:
def linear_search(arr, target):
for i, item in enumerate(arr):
if item == target:
return f"Found {target} at index {i}"
return f"{target} not found in the list"
# List of items to search through
items = ["book", "pen", "laptop", "notebook", "pencil"]
# Searching for an item
print(linear_search(items, "laptop"))
print(linear_search(items, "phone"))
This code implements a simple linear search algorithm, which is like moving a magnifying glass over each item in a list until the desired item is found.
7. The Gear Icon: Functions and Processes
The gear or cog icon, often used to represent settings or processes, can be an excellent analogy for functions and processes in programming.
Why the Connection?
Gears work together in a machine to perform complex tasks, much like functions in a program work together to achieve a larger goal. Each gear has a specific role, just as each function in a program has a specific purpose.
Coding Example:
def gear_a(x):
return x * 2
def gear_b(x):
return x + 5
def gear_c(x):
return x ** 2
def machine_process(input_value):
result = gear_a(input_value)
result = gear_b(result)
result = gear_c(result)
return result
# Using the machine process
input_value = 3
output = machine_process(input_value)
print(f"Input: {input_value}, Output: {output}")
In this example, each function (gear_a
, gear_b
, gear_c
) represents a gear in a machine. The machine_process
function combines these “gears” to process the input, similar to how gears work together in a real machine.
8. The Lock Icon: Encryption and Data Security
The lock icon, commonly used to represent security in digital interfaces, can be a great way to introduce concepts of encryption and data security in programming.
Why the Connection?
Just as a lock secures physical objects, encryption algorithms secure digital data. The process of locking and unlocking with a key is analogous to the encryption and decryption processes in programming.
Coding Example:
import base64
def encrypt(message, key):
encoded = base64.b64encode(message.encode()).decode()
encrypted = ""
for i, char in enumerate(encoded):
key_char = key[i % len(key)]
encrypted += chr((ord(char) + ord(key_char)) % 256)
return base64.b64encode(encrypted.encode()).decode()
def decrypt(encrypted, key):
decoded = base64.b64decode(encrypted).decode()
decrypted = ""
for i, char in enumerate(decoded):
key_char = key[i % len(key)]
decrypted += chr((ord(char) - ord(key_char)) % 256)
return base64.b64decode(decrypted).decode()
# Using the encryption
message = "Hello, World!"
key = "secretkey"
encrypted = encrypt(message, key)
print("Encrypted:", encrypted)
decrypted = decrypt(encrypted, key)
print("Decrypted:", decrypted)
This example demonstrates a simple encryption and decryption process. While this is not a secure encryption method for real-world use, it illustrates the concept of using a key to “lock” (encrypt) and “unlock” (decrypt) data.
9. The Calendar Icon: Time Complexity and Scheduling
The calendar icon can be used to introduce the concept of time complexity in algorithms and scheduling in programming.
Why the Connection?
A calendar helps us manage time and schedule tasks efficiently. Similarly, in programming, we need to consider how long our algorithms take to run (time complexity) and how to schedule tasks efficiently, especially in concurrent programming.
Coding Example:
import time
def measure_time(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.5f} seconds to run")
return result
return wrapper
@measure_time
def linear_search(arr, target):
for item in arr:
if item == target:
return True
return False
@measure_time
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return True
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return False
# Create a large sorted list
large_list = list(range(1000000))
# Search for a number
linear_search(large_list, 999999)
binary_search(large_list, 999999)
This example compares the time complexity of linear search and binary search algorithms. The measure_time
decorator acts like a stopwatch, measuring how long each function takes to run, similar to how we might use a calendar to track the duration of tasks.
10. The Chain Link: Data Structures (Linked Lists)
The chain link symbol can be an excellent representation of linked lists, a fundamental data structure in programming.
Why the Connection?
Each link in a chain connects to the next, forming a sequence. This is exactly how a linked list works in programming: each node in the list contains data and a reference (or “link”) to the next node.
Coding Example:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def display(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
print(" -> ".join(map(str, elements)))
# Using the LinkedList
chain = LinkedList()
chain.append("Link 1")
chain.append("Link 2")
chain.append("Link 3")
chain.display()
This example demonstrates how a linked list can be implemented in Python. Each Node
in the list is like a link in a chain, connected to the next node.
Conclusion: Bridging the Gap Between Everyday Symbols and Coding Concepts
As we’ve explored in this journey from emojis to algorithms, the world of programming is not as disconnected from our daily lives as it might initially seem. By drawing parallels between familiar symbols and complex coding concepts, we can demystify programming and make it more accessible to learners of all levels.
These analogies serve as mental bridges, connecting the known to the unknown. They provide a foundation upon which deeper understanding can be built. As you continue your coding journey, try to find more such connections in your everyday life. You might be surprised at how many programming concepts are reflected in the world around you.
Remember, the goal of platforms like AlgoCademy is not just to teach you how to code, but to help you think like a programmer. By developing this mindset, you’ll be better equipped to solve problems, create efficient solutions, and ultimately, prepare for technical interviews at top tech companies.
So the next time you see a smiley face emoji, a traffic light, or a shopping cart icon, take a moment to reflect on the programming concept it represents. This practice will reinforce your learning and help you see the world through a programmer’s eyes. Happy coding!