Why You Understand The Code But Can’t Write It From Scratch
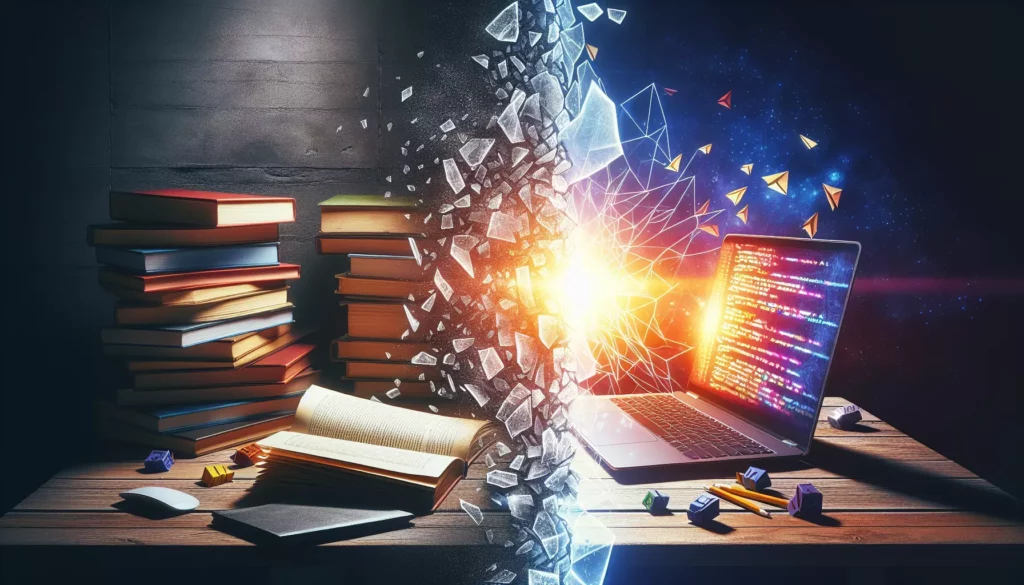
The Coding Comprehension Paradox: Understanding vs. Creating
Have you ever found yourself nodding along while reading someone else’s code, thinking “This makes perfect sense!” — only to freeze when faced with a blank editor and asked to create similar functionality from scratch? This phenomenon is incredibly common, even among experienced developers. You’re not alone in this struggle, and there’s actually solid cognitive science behind why understanding code and generating it are such different mental processes.
This disconnect between comprehension and creation is what I call the “Coding Comprehension Paradox,” and it affects programmers at all levels. In this post, we’ll explore why recognizing patterns in existing code is fundamentally different from conjuring solutions from thin air, and more importantly, how you can bridge this gap in your own development journey.
Recognition vs. Recall: The Fundamental Cognitive Difference
At its core, this problem stems from how our brains process information. Cognitive psychologists distinguish between two types of memory retrieval: recognition and recall.
Recognition memory is your ability to identify information you’ve previously encountered. When reading code, you’re essentially saying, “Yes, I recognize this pattern. I know what a for-loop does. I understand how this function transforms data.”
Recall memory, on the other hand, requires you to retrieve information from memory without external cues. Writing code from scratch demands recall — you must summon syntax, algorithms, and patterns with minimal prompting.
This distinction explains why you might perfectly understand every line of a complex algorithm when reading it, but struggle to reproduce even a simplified version when staring at an empty file. Recognition is simply easier for our brains than recall.
Think of it like the difference between recognizing a friend’s face in a crowd (easy) versus trying to draw their portrait from memory (much harder). Both involve the same information, but the mental processes are vastly different.
The Four Stages of Code Comprehension
To better understand this paradox, let’s break down the journey from reading code to writing it:
1. Passive Familiarity
At this stage, code looks familiar. You recognize syntax, understand what individual lines do, and can follow the general flow. This is where many developers get stuck thinking they “understand” the code.
For example, you might look at this JavaScript snippet and understand it perfectly:
const users = [
{ name: 'Alice', age: 25, active: true },
{ name: 'Bob', age: 30, active: false },
{ name: 'Charlie', age: 35, active: true }
];
const activeUsers = users.filter(user => user.active);
console.log(activeUsers);
You recognize the array, the objects within it, the filter method, and the arrow function. It all makes sense when you read it.
2. Active Comprehension
Here, you can not only read the code but also explain it to someone else, predict its output, and identify potential edge cases or bugs. You understand not just what the code does, but why it’s written that way.
Using our example above, at this stage you could explain that the code filters an array of user objects to include only those with the “active” property set to true, and you might even point out that the code doesn’t handle cases where the “active” property might be missing.
3. Adaptation Ability
At this level, you can take existing code and modify it to suit different requirements. You can change parameters, add features, or fix bugs. This is where many professional developers operate day-to-day.
For instance, you could adapt the previous example to filter users who are both active and over a certain age:
const activeAdultUsers = users.filter(user => user.active && user.age >= 18);
console.log(activeAdultUsers);
4. Generation Capability
This is the ability to create similar functionality from scratch without referencing existing code. It requires deep internalization of patterns, principles, and problem-solving approaches.
At this stage, if asked to “write code that filters a collection based on certain criteria,” you could produce a solution without looking at examples.
The gap between stages 3 and 4 is where most developers experience the frustration of understanding but not being able to create.
Why Understanding Code Doesn’t Automatically Enable Creation
Several factors contribute to the disconnect between comprehension and creation:
The Illusion of Competence
When we read well-written code, its clarity can create an illusion that we’ve mastered the concepts. Psychologists call this “fluency bias” — when information feels easy to process, we assume we’ve learned it thoroughly. But true learning requires active engagement, not just passive reading.
Think about learning a foreign language: understanding a sentence when you hear it is much easier than constructing grammatically correct sentences yourself. The same principle applies to code.
Working Memory Limitations
Writing code from scratch requires juggling multiple concepts simultaneously in your working memory:
- Syntax details
- Algorithmic approach
- Variable naming and management
- Program flow and structure
- Edge cases and error handling
Our working memory has limited capacity (typically 4-7 items). When reading code, the structure is provided for you, reducing cognitive load. When writing code, you must manage all these elements yourself, which can quickly overwhelm your mental resources.
The Missing Context
Existing code provides context and constraints that guide understanding. It’s like having the border and corner pieces of a puzzle already assembled. Without this framework, the blank editor represents infinite possibilities, which can be paralyzing.
Additionally, professional codebases often contain layers of context that aren’t immediately visible:
- Architecture decisions made before this code was written
- Team conventions and patterns
- Historical reasons for certain approaches
- Implicit knowledge about the problem domain
When trying to write similar code, you may be missing this crucial context.
The Gap Between Conceptual and Procedural Knowledge
Understanding code (conceptual knowledge) is different from knowing the steps to create it (procedural knowledge). This is similar to understanding how a car works versus knowing how to build one.
You might perfectly understand what a binary search tree is and how it functions, but implementing one requires procedural knowledge of:
- How to define the node structure
- How to write insertion logic
- How to implement traversal algorithms
- How to handle edge cases
Each of these steps requires its own procedural knowledge, creating a much higher barrier to creation than comprehension.
The Role of Experience and Pattern Recognition
Experienced developers seem to magically produce code because they’ve internalized common patterns and solutions. This pattern recognition develops through repeated exposure and practice.
When a senior developer writes code “from scratch,” they’re actually assembling pre-existing mental patterns they’ve built over years. They’re not creating entirely new solutions each time.
Mental Models and Chunking
Experts use what psychologists call “chunking” to overcome working memory limitations. Instead of thinking about individual lines of code, they think in higher-level patterns or “chunks.”
For example, an experienced developer doesn’t see this:
for (let i = 0; i < array.length; i++) {
if (array[i].property === value) {
return array[i];
}
}
return null;
They see "find first matching element in array" as a single concept or chunk. This mental compression allows them to hold more complex ideas in working memory.
As you gain experience, you develop more sophisticated chunks, enabling you to think at higher levels of abstraction. This is why experienced developers can write complex functionality quickly — they're not thinking about each line, but about larger conceptual units.
The Importance of Deliberate Practice
Building these mental models requires deliberate practice — not just reading or writing code, but consciously analyzing patterns and internalizing them. This is why simply reading more code won't necessarily improve your ability to write it.
Research in expertise development shows that the quality of practice matters more than quantity. Effective practice involves:
- Working at the edge of your abilities
- Receiving immediate feedback
- Reflecting on what worked and what didn't
- Deliberately practicing difficult components
This type of focused practice builds the neural pathways needed for effective recall when writing code from scratch.
Bridging the Gap: Strategies to Improve Your Code Creation Skills
Now for the practical part: how do you move from understanding code to confidently writing it yourself? Here are proven strategies to bridge this gap:
1. Implement Active Reading Techniques
Don't just passively read code. Engage with it actively:
- Predict before you read: Before looking at a function's implementation, try to predict how it might be coded based on its name and purpose.
- Explain in your own words: After reading a section of code, close the file and explain what it does aloud, as if teaching someone else.
- Question the implementation: Ask yourself "Why did they choose this approach instead of alternatives?"
- Identify patterns: Consciously note recurring patterns and give them names in your mind.
This active engagement transforms passive recognition into knowledge you can recall later.
2. Practice Incremental Writing
Instead of trying to write complex functionality from scratch, build up incrementally:
- Start with the simplest possible version that works
- Add one feature or handle one edge case at a time
- Test each increment before moving to the next
For example, if building a user authentication system, start with just the basic login function before adding password validation, security features, and error handling.
// Step 1: Basic function structure
function loginUser(username, password) {
// Just return true for now
return true;
}
// Step 2: Add simple validation
function loginUser(username, password) {
if (!username || !password) {
return false;
}
return true;
}
// Step 3: Add mock database check
function loginUser(username, password) {
if (!username || !password) {
return false;
}
// Mock database check
const validUser = { username: 'testuser', password: 'password123' };
return username === validUser.username && password === validUser.password;
}
This incremental approach prevents the blank-page paralysis and builds your confidence as you see progress.
3. Use the "Code Reconstruction" Technique
This powerful learning method involves:
- Study a piece of code until you understand it thoroughly
- Close the file and wait at least an hour (preferably a day)
- Try to rewrite the same functionality from memory
- Compare your version with the original and analyze differences
This technique bridges the gap between recognition and recall, forcing your brain to retrieve the patterns rather than just recognize them.
4. Build a Personal Code Snippet Library
Create your own library of code patterns that you understand and can adapt:
- Organize snippets by functionality (data transformation, API calls, validation, etc.)
- Add comments explaining how each snippet works and when to use it
- Include examples of how the snippet can be modified for different uses
This library becomes a bridge between reading others' code and writing your own, giving you starting points that you can customize.
5. Practice Deliberate Imitation
Artists learn by copying masters, and programmers can use the same approach:
- Find well-written code that accomplishes something similar to your goal
- Study how it's structured and why certain decisions were made
- Write your own version, deliberately applying the patterns you observed
- Gradually modify and expand on these patterns to make them your own
This isn't plagiarism when done as a learning exercise — it's how expertise has been developed for centuries in creative fields.
6. Implement the "Read-Cover-Recall-Check" Method
This technique comes from effective study methods:
- Read: Study a code example thoroughly
- Cover: Hide the code
- Recall: Write as much as you can remember
- Check: Compare your version with the original
This approach strengthens the neural pathways needed for recall, making it easier to write similar code in the future.
The Power of Breaking Down Problems
One of the biggest challenges in writing code from scratch is the overwhelming complexity of the whole problem. Expert developers don't tackle entire problems at once — they break them down into manageable components.
The Decomposition Technique
When faced with a complex coding task:
- Identify the major components or steps required
- Break each component into smaller, more manageable sub-problems
- Continue breaking down until each piece feels approachable
- Implement each small piece independently
- Integrate the pieces into a complete solution
For example, if building a contact form with validation and submission:
- Component 1: HTML form structure
- Sub-task: Create input fields
- Sub-task: Style the form
- Component 2: Form validation
- Sub-task: Validate email format
- Sub-task: Check required fields
- Sub-task: Display error messages
- Component 3: Form submission
- Sub-task: Prevent default submit behavior
- Sub-task: Collect form data
- Sub-task: Send data to server
- Sub-task: Handle response
By tackling each sub-task individually, the formerly overwhelming project becomes a series of manageable challenges.
The Pseudocode Bridge
Pseudocode serves as a bridge between your understanding of a problem and the actual code implementation. Before writing real code:
- Write out the logic in plain English or informal pseudocode
- Review and refine your approach
- Gradually translate each line of pseudocode into actual code
For example:
// Pseudocode
// 1. Get all users from database
// 2. Filter out inactive users
// 3. Sort remaining users by last login date
// 4. Return the first 10 users
// Actual implementation
async function getRecentActiveUsers() {
// 1. Get all users from database
const allUsers = await database.getUsers();
// 2. Filter out inactive users
const activeUsers = allUsers.filter(user => user.isActive);
// 3. Sort remaining users by last login date
const sortedUsers = activeUsers.sort((a, b) =>
new Date(b.lastLogin) - new Date(a.lastLogin)
);
// 4. Return the first 10 users
return sortedUsers.slice(0, 10);
}
This approach makes the transition from understanding to implementation more gradual and manageable.
Overcoming Perfectionism and Analysis Paralysis
Many developers struggle to write code from scratch because they aim for perfection on the first try. This perfectionism leads to analysis paralysis — the inability to start because you can't immediately see the perfect solution.
Embrace the Iterative Process
Professional developers rarely write perfect code on the first attempt. Instead, they:
- Write a "good enough" first version that works
- Refactor to improve structure and readability
- Optimize for performance if necessary
- Add tests to ensure reliability
Give yourself permission to follow this same process. Your first attempt doesn't need to be perfect — it just needs to work well enough to build upon.
The 15-Minute Rule
When stuck on a problem, follow the 15-minute rule:
- Try to solve the problem on your own for 15 minutes
- If still stuck, look for hints or examples (not complete solutions)
- Try again with this new information for another 15 minutes
- If still stuck, look at a solution, but then close it and implement your own version
This structured approach prevents both giving up too quickly and wasting hours when you need guidance.
Learning to Think Like a Programmer
Beyond specific techniques, developing a "programmer's mindset" is crucial for bridging the understanding-creation gap.
Cultivate Algorithmic Thinking
Practice describing processes as step-by-step algorithms in your daily life. For example, think about the algorithm for making a sandwich or finding the fastest route to work. This trains your brain to break problems into sequential steps.
Develop Pattern Recognition
Actively look for patterns in code you read and problems you solve. Creating a "pattern vocabulary" helps you recognize when similar solutions can be applied to new problems.
Common patterns to recognize include:
- Iteration patterns (map, filter, reduce)
- Recursion patterns
- State management patterns
- Data transformation patterns
- Error handling patterns
Build Mental Models
Develop clear mental models of how programming concepts work. For example, don't just memorize how to use array methods — understand how they work internally.
Strong mental models allow you to reason about code even when you don't remember the exact syntax or implementation details.
From Understanding to Creating: A Progressive Path
Let's put everything together into a progressive learning path that takes you from understanding code to confidently creating it:
Stage 1: Active Comprehension (1-2 weeks)
- Find high-quality code examples in your target language/framework
- Practice active reading techniques with these examples
- Write summaries explaining how the code works and why certain decisions were made
- Create diagrams or flowcharts to visualize the code's operation
Stage 2: Guided Modification (2-3 weeks)
- Take working code examples and modify them to add new features
- Fix intentionally broken code
- Rewrite existing functionality using different approaches
- Practice the "code reconstruction" technique
Stage 3: Scaffolded Creation (3-4 weeks)
- Use pseudocode to plan solutions before coding
- Build solutions incrementally, testing each step
- Use your snippet library as a starting point
- Implement solutions with the help of documentation and references
Stage 4: Independent Creation (Ongoing)
- Set progressively more challenging projects for yourself
- Build complete applications from scratch
- Contribute to open source projects
- Teach others to solidify your understanding
This gradual progression builds the neural pathways needed to move from understanding to creation, without the frustration of trying to leap directly from reading code to writing complex applications from scratch.
Common Obstacles and How to Overcome Them
As you work to bridge the gap between understanding and creating code, you'll likely encounter some common obstacles:
Syntax Frustration
Problem: You understand the logic but get stuck on syntax details.
Solution: Create syntax cheat sheets for your most-used language features. Use an IDE with good autocomplete features. Remember that even experienced developers regularly look up syntax — it's not a sign of weakness.
The Blank Editor Problem
Problem: You freeze when facing an empty file, unsure where to start.
Solution: Start by writing comments outlining what you want to do. Create a standard template for projects or files that you can use as a starting point. Begin with the part you're most confident about, even if it's not the logical first step.
Comparing Yourself to Others
Problem: You get discouraged seeing others write code quickly and confidently.
Solution: Remember that you're seeing their finished performance, not their learning process. Most developers struggle when learning new concepts. Focus on your progress compared to your past self, not compared to others.
Tutorial Dependency
Problem: You can follow tutorials but struggle to create similar projects independently.
Solution: After completing a tutorial, immediately build a similar project with different requirements. Start with small modifications to the tutorial project, then gradually create more independent work.
The Importance of Deliberate Practice
Throughout this article, I've mentioned "deliberate practice" several times. This concept, popularized by psychologist K. Anders Ericsson, is crucial for moving from understanding to mastery.
Deliberate practice isn't just writing more code — it's practicing with specific intent to improve. Effective deliberate practice in programming includes:
- Focus on specific skills: Instead of building a complete app, spend time mastering particular techniques (e.g., practice writing recursive functions or implementing specific design patterns)
- Immediate feedback: Test your code frequently and analyze what works and what doesn't
- Working at the edge of your ability: Choose challenges that push you just beyond your current comfort zone
- Reflection: Regularly review your code and approach to identify patterns and areas for improvement
This type of focused practice accelerates the development of the neural connections needed for effective code creation.
Conclusion: Understanding and Creating Are Different Skills
The gap between understanding code and being able to write it from scratch is natural and affects developers at all levels. It's not a reflection of your intelligence or potential as a programmer — it's simply a result of how our brains process and retrieve information.
By recognizing that comprehension and creation are different cognitive skills requiring different types of practice, you can take a more structured approach to developing your coding abilities. The strategies outlined in this article provide a path from passive understanding to active creation:
- Practice active reading to deepen comprehension
- Use techniques like code reconstruction to bridge recognition and recall
- Break down complex problems into manageable components
- Build incrementally rather than expecting perfection from the start
- Create a personal library of patterns and snippets
- Engage in deliberate practice focused on specific skills
Remember that even experienced developers rarely write complex code from scratch without references, planning, or iterations. The goal isn't to memorize every syntax detail or algorithm but to develop the problem-solving mindset and pattern recognition that allows you to approach new challenges confidently.
With consistent practice and patience, you'll gradually close the gap between what you can understand and what you can create, building both your skills and your confidence as a developer.
What strategies have helped you bridge the gap between understanding and creating code? Share your experiences in the comments below!