From Blueprint to Code: How to Design and Build Projects from Scratch
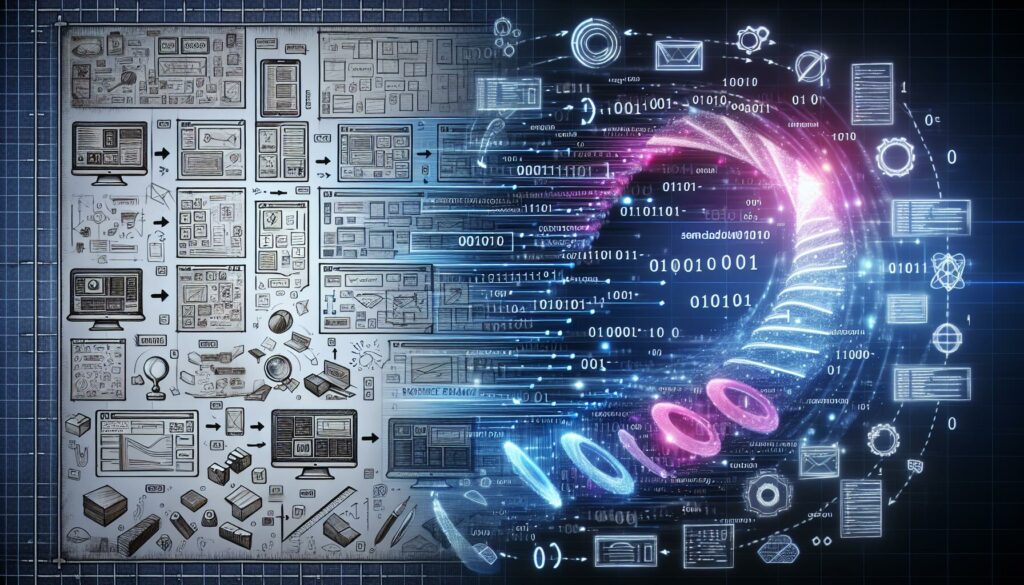
In the world of software development, the journey from a mere idea to a fully functional application can be both exciting and challenging. Whether you’re a seasoned programmer or just starting your coding journey, understanding how to design and build projects from scratch is an essential skill. This comprehensive guide will walk you through the process of transforming your ideas into tangible, working software, covering everything from initial planning to final deployment.
1. Conceptualization and Planning
Every great project starts with a solid idea and a well-thought-out plan. This initial phase is crucial for setting the foundation of your project and ensuring its success.
1.1. Define Your Project’s Purpose and Goals
Begin by clearly articulating what your project aims to achieve. Ask yourself:
- What problem does this project solve?
- Who is the target audience?
- What are the key features and functionalities?
Write down your answers to these questions, as they will guide your decision-making throughout the development process.
1.2. Create a Project Outline
Develop a high-level outline of your project. This should include:
- Main components or modules
- Key features and their priorities
- Potential challenges and risks
- Timeline and milestones
This outline will serve as your roadmap, helping you stay focused and organized as you progress.
1.3. Research and Gather Resources
Before diving into development, it’s crucial to research and gather the necessary resources:
- Explore similar existing projects for inspiration and to avoid reinventing the wheel
- Identify potential technologies, frameworks, and libraries that could be useful
- Collect relevant documentation, tutorials, and learning materials
2. Designing the Architecture
With a clear plan in place, it’s time to design the architecture of your project. This step involves making crucial decisions about the structure and organization of your code.
2.1. Choose Your Tech Stack
Select the technologies you’ll use for your project. Consider factors such as:
- Programming languages (e.g., Python, JavaScript, Java)
- Frameworks (e.g., React, Django, Spring)
- Databases (e.g., MySQL, MongoDB, PostgreSQL)
- Hosting platforms (e.g., AWS, Heroku, DigitalOcean)
Your choice should align with your project requirements, your team’s expertise, and scalability needs.
2.2. Design the System Architecture
Sketch out the high-level architecture of your system. This may include:
- Frontend and backend components
- Database schema
- API endpoints
- Third-party integrations
Use diagrams or flowcharts to visualize the relationships between different components.
2.3. Plan Your Data Models
Design your data models and relationships. This involves:
- Identifying the main entities in your system
- Defining attributes for each entity
- Establishing relationships between entities
- Creating a database schema or object model
For example, if you’re building a blog application, you might have models for User, Post, and Comment, with relationships between them.
3. Setting Up the Development Environment
Before you start coding, it’s essential to set up a proper development environment. This ensures consistency and efficiency throughout the development process.
3.1. Install Necessary Tools
Install all the required software and tools for your project:
- Integrated Development Environment (IDE) or code editor (e.g., Visual Studio Code, PyCharm)
- Version control system (e.g., Git)
- Package managers (e.g., npm, pip)
- Database management tools
- API testing tools (e.g., Postman)
3.2. Set Up Version Control
Initialize a Git repository for your project and set up a remote repository on a platform like GitHub or GitLab. This allows you to:
- Track changes in your code
- Collaborate with others
- Revert to previous versions if needed
- Manage different features or experiments using branches
3.3. Configure Your Development Environment
Set up your development environment to match your project requirements:
- Configure your IDE or editor with appropriate plugins and settings
- Set up virtual environments for Python projects
- Configure environment variables for sensitive information
- Set up linters and code formatters for consistent code style
4. Implementing Core Functionality
With your environment set up, it’s time to start implementing the core functionality of your project.
4.1. Start with a Minimum Viable Product (MVP)
Begin by implementing the most basic version of your project that still provides value. This approach allows you to:
- Validate your core concept quickly
- Get early feedback
- Identify and address fundamental issues early in the development process
4.2. Follow Best Practices and Design Patterns
As you code, adhere to best practices and utilize appropriate design patterns:
- Write clean, readable, and well-documented code
- Follow the DRY (Don’t Repeat Yourself) principle
- Implement proper error handling and logging
- Use design patterns like MVC (Model-View-Controller) or MVVM (Model-View-ViewModel) where appropriate
4.3. Implement Key Features Incrementally
Build your project feature by feature, starting with the most critical functionalities:
- Implement basic data models and database interactions
- Create core business logic
- Develop API endpoints or service layers
- Build user interfaces or frontend components
Here’s a simple example of implementing a basic API endpoint using Express.js:
const express = require("express");
const app = express();
const port = 3000;
app.get("/api/hello", (req, res) => {
res.json({ message: "Hello, World!" });
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
5. Testing and Quality Assurance
As you build your project, it’s crucial to implement a robust testing strategy to ensure the quality and reliability of your code.
5.1. Write Unit Tests
Create unit tests for individual components and functions. This helps you:
- Verify that each part of your code works as expected
- Catch bugs early in the development process
- Refactor with confidence
Here’s an example of a simple unit test using Jest:
function sum(a, b) {
return a + b;
}
test("adds 1 + 2 to equal 3", () => {
expect(sum(1, 2)).toBe(3);
});
5.2. Implement Integration Tests
Write integration tests to ensure different parts of your application work together correctly:
- Test API endpoints
- Verify database interactions
- Check third-party integrations
5.3. Perform Manual Testing
Conduct thorough manual testing to catch issues that automated tests might miss:
- Test user flows and interactions
- Check for usability issues
- Verify cross-browser and cross-device compatibility
6. Optimization and Performance Tuning
Once your core functionality is in place and tested, focus on optimizing your project for better performance.
6.1. Profile Your Code
Use profiling tools to identify performance bottlenecks in your code. Look for:
- Slow database queries
- Inefficient algorithms
- Memory leaks
- Unnecessary computations
6.2. Optimize Database Performance
Improve database performance through techniques such as:
- Indexing frequently queried fields
- Optimizing complex queries
- Implementing caching mechanisms
- Using database-specific optimization features
6.3. Implement Caching
Use caching to reduce the load on your server and improve response times:
- Implement in-memory caching for frequently accessed data
- Use Content Delivery Networks (CDNs) for static assets
- Implement API response caching where appropriate
6.4. Optimize Frontend Performance
Improve the performance of your frontend:
- Minimize and compress assets (CSS, JavaScript, images)
- Implement lazy loading for images and components
- Use code splitting to reduce initial load times
- Optimize rendering performance
7. Security Considerations
Security should be a top priority throughout the development process. Implement security best practices to protect your application and user data.
7.1. Implement Authentication and Authorization
Secure your application with robust authentication and authorization mechanisms:
- Use secure password hashing algorithms (e.g., bcrypt)
- Implement JWT (JSON Web Tokens) for stateless authentication
- Use OAuth for third-party authentication
- Implement role-based access control (RBAC)
7.2. Protect Against Common Vulnerabilities
Safeguard your application against common security threats:
- Prevent SQL injection by using parameterized queries
- Implement CSRF (Cross-Site Request Forgery) protection
- Use Content Security Policy (CSP) headers
- Sanitize user inputs to prevent XSS (Cross-Site Scripting) attacks
7.3. Secure Data Transmission
Ensure that data is transmitted securely:
- Use HTTPS for all communications
- Implement proper SSL/TLS configuration
- Encrypt sensitive data in transit and at rest
7.4. Regular Security Audits
Conduct regular security audits and penetration testing to identify and address vulnerabilities:
- Use automated security scanning tools
- Perform manual code reviews focused on security
- Stay updated on security best practices and emerging threats
8. Documentation and Knowledge Sharing
Proper documentation is crucial for the long-term success and maintainability of your project.
8.1. Write Comprehensive Documentation
Create detailed documentation for your project, including:
- README file with project overview and setup instructions
- API documentation
- Code comments explaining complex logic
- Architecture and design documents
- Deployment and configuration guides
8.2. Create User Guides
If your project has a user interface, create user guides or help documentation:
- Step-by-step tutorials for common tasks
- FAQ section
- Troubleshooting guides
8.3. Maintain a Change Log
Keep a detailed change log to track updates and changes to your project:
- Document new features
- List bug fixes
- Note any breaking changes or deprecations
9. Deployment and Continuous Integration/Continuous Deployment (CI/CD)
Set up a robust deployment process to ensure smooth and reliable releases of your project.
9.1. Choose a Hosting Platform
Select an appropriate hosting platform based on your project requirements:
- Cloud platforms (e.g., AWS, Google Cloud, Azure)
- Platform-as-a-Service (PaaS) providers (e.g., Heroku, DigitalOcean App Platform)
- Self-hosted solutions
9.2. Set Up CI/CD Pipelines
Implement CI/CD pipelines to automate your build, test, and deployment processes:
- Use tools like Jenkins, GitLab CI, or GitHub Actions
- Automate unit and integration tests
- Perform static code analysis and linting
- Automate deployment to staging and production environments
9.3. Implement Monitoring and Logging
Set up monitoring and logging to track the health and performance of your application:
- Use application performance monitoring (APM) tools
- Implement centralized logging
- Set up alerts for critical issues
- Monitor server resources and application metrics
10. Maintenance and Iteration
Once your project is live, the work doesn’t stop. Continuous maintenance and iteration are key to long-term success.
10.1. Regular Updates and Patches
Keep your project up-to-date and secure:
- Regularly update dependencies to patch security vulnerabilities
- Fix bugs as they are discovered
- Refactor code to improve maintainability
10.2. Gather and Analyze Feedback
Continuously gather feedback from users and stakeholders:
- Implement user feedback mechanisms
- Analyze usage data and metrics
- Conduct user surveys or interviews
10.3. Plan and Implement New Features
Based on feedback and project goals, plan and implement new features:
- Prioritize feature requests
- Plan development sprints or iterations
- Continuously improve user experience
Conclusion
Designing and building projects from scratch is a complex but rewarding process. By following this comprehensive guide, you’ll be well-equipped to take your ideas from concept to reality. Remember that software development is an iterative process, and even after your initial release, there’s always room for improvement and innovation.
As you embark on your project journey, keep in mind the importance of planning, best practices, and continuous learning. Embrace challenges as opportunities to grow your skills and create better software. With dedication and the right approach, you can turn your blueprints into powerful, efficient, and user-friendly applications that make a real impact.
Happy coding, and may your projects be successful and impactful!