From Blank Screen to Full Solution: How to Build Confidence in Starting New Problems
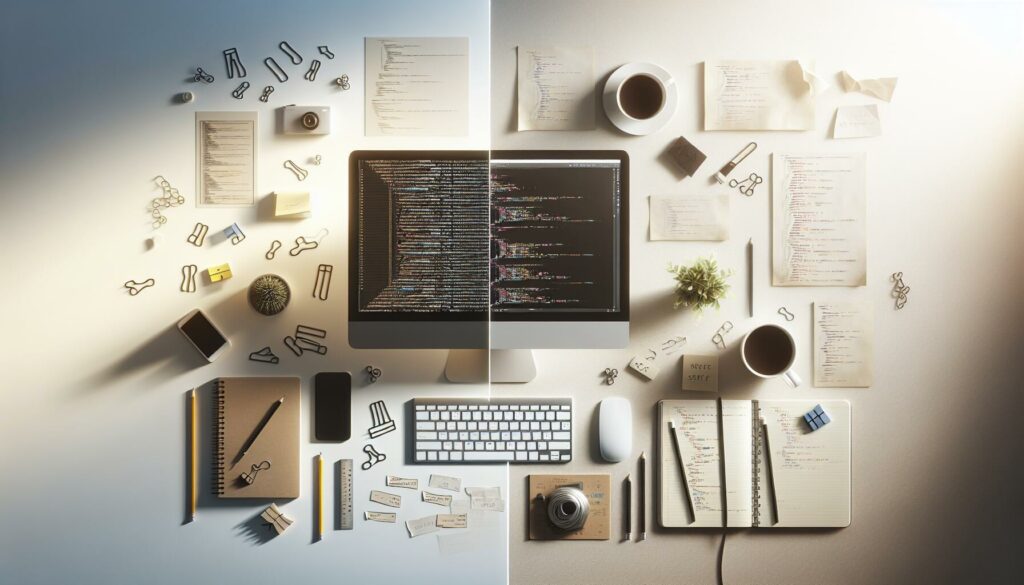
As aspiring programmers and seasoned developers alike, we’ve all faced that daunting moment: staring at a blank screen, cursor blinking, with a new coding problem looming before us. The challenge of transforming that empty canvas into a fully-fledged solution can be overwhelming, especially when tackling unfamiliar territory. But fear not! In this comprehensive guide, we’ll explore effective strategies to build your confidence and equip you with the tools to approach new problems with enthusiasm and skill.
1. Embrace the Learning Mindset
Before diving into any new problem, it’s crucial to adopt the right mindset. Remember, every challenge is an opportunity to learn and grow. Here’s how to cultivate a positive approach:
- Reframe the challenge: Instead of viewing the problem as a test of your abilities, see it as a chance to expand your knowledge and skills.
- Celebrate small victories: Acknowledge each step forward, no matter how small. This builds momentum and motivation.
- Learn from failures: Mistakes are not setbacks; they’re valuable lessons. Analyze what went wrong and use that insight to improve.
2. Break Down the Problem
Large, complex problems can be intimidating. The key is to break them down into smaller, manageable pieces. This approach, often called “divide and conquer,” makes the task less daunting and more approachable.
Steps to break down a problem:
- Read the problem statement carefully, multiple times if necessary.
- Identify the main components or requirements of the problem.
- List out the sub-problems or individual tasks that need to be solved.
- Prioritize these sub-problems based on their dependencies and complexity.
- Tackle each sub-problem one at a time.
For example, if you’re tasked with creating a simple to-do list application, you might break it down like this:
- Create a data structure to store tasks
- Implement a function to add new tasks
- Develop a function to mark tasks as complete
- Design a user interface to display tasks
- Add functionality to remove tasks
3. Start with Pseudocode
Before jumping into actual coding, it’s often helpful to outline your solution in pseudocode. This high-level description of your algorithm allows you to focus on the logic without getting bogged down in syntax details.
Here’s an example of pseudocode for adding a new task to our to-do list:
FUNCTION addTask(taskDescription):
CREATE new task object with description and completed status (false)
ADD task object to tasks list
DISPLAY confirmation message
END FUNCTION
Writing pseudocode helps you organize your thoughts and provides a roadmap for your actual code implementation.
4. Research and Gather Resources
Don’t hesitate to seek out information and resources when facing a new problem. This doesn’t mean copying solutions, but rather learning concepts and techniques that can help you solve the problem.
Useful resources include:
- Official documentation for the programming language or framework you’re using
- Online coding platforms like Stack Overflow for specific questions
- Tutorial websites and coding blogs
- Video tutorials on platforms like YouTube or Coursera
- Coding books and e-books
Remember, the goal is to understand the concepts and apply them to your specific problem, not to find a ready-made solution.
5. Start Small and Iterate
Once you have a plan and some resources, it’s time to start coding. Begin with the simplest part of your solution and build from there. This approach, known as incremental development, allows you to see progress quickly and helps maintain motivation.
Steps for incremental development:
- Implement the simplest functionality first
- Test your code to ensure it works as expected
- Refine and optimize your solution
- Add the next piece of functionality
- Repeat steps 2-4 until your solution is complete
For our to-do list example, you might start by implementing the function to add tasks, then move on to displaying tasks, and so on.
6. Use Version Control
As you work on your solution, it’s important to track your progress and have the ability to revert changes if needed. This is where version control systems like Git come in handy. They allow you to:
- Save different versions of your code
- Experiment with new features without fear of breaking existing code
- Collaborate with others more easily
- Track the history of your project
If you’re new to Git, here’s a simple workflow to get started:
git init # Initialize a new Git repository
git add . # Stage all files for commit
git commit -m "Initial commit" # Commit your changes
# ... make some changes to your code ...
git add . # Stage the new changes
git commit -m "Added new feature X" # Commit the new changes
7. Practice Debugging Techniques
Encountering errors is an inevitable part of coding. Developing strong debugging skills will boost your confidence in tackling new problems. Here are some effective debugging strategies:
- Use print statements: Add print statements to your code to track variable values and program flow.
- Utilize breakpoints: If your IDE supports it, use breakpoints to pause execution and inspect your program’s state.
- Read error messages carefully: Error messages often provide valuable clues about what’s going wrong and where.
- Use a debugger: Learn to use debugging tools provided by your programming environment.
- Rubber duck debugging: Explain your code line-by-line to an inanimate object (or a patient friend). Often, the act of explaining helps you spot the issue.
Here’s a simple example of using print statements for debugging:
def calculate_average(numbers):
print(f"Input: {numbers}") # Debug print
total = sum(numbers)
print(f"Sum: {total}") # Debug print
count = len(numbers)
print(f"Count: {count}") # Debug print
average = total / count
print(f"Average: {average}") # Debug print
return average
result = calculate_average([1, 2, 3, 4, 5])
print(f"Final result: {result}")
8. Seek Feedback and Collaborate
Don’t underestimate the power of collaboration and feedback. Engaging with other developers can provide new perspectives, help you spot errors, and introduce you to better solutions. Here’s how to leverage collaboration:
- Join coding communities: Participate in online forums, Discord servers, or local meetups.
- Pair programming: Work with a partner to solve problems together.
- Code reviews: Ask peers or mentors to review your code and provide feedback.
- Contribute to open-source projects: This exposes you to different coding styles and collaborative workflows.
Remember, even experienced developers seek help and feedback. It’s a sign of wisdom, not weakness.
9. Reflect and Document
After solving a problem, take time to reflect on your process and document your solution. This practice reinforces your learning and creates a valuable resource for future reference.
Reflection questions:
- What was the most challenging part of this problem?
- What new concepts or techniques did I learn?
- How could I improve my approach next time?
- Are there any parts of my solution that could be optimized?
Consider maintaining a coding journal or blog where you document your problem-solving processes and solutions. This not only helps solidify your understanding but can also be a great addition to your portfolio.
10. Embrace Continuous Learning
The field of programming is constantly evolving, with new languages, frameworks, and best practices emerging regularly. To build lasting confidence in your problem-solving abilities, commit to continuous learning:
- Set learning goals: Identify areas where you want to improve and set specific, achievable goals.
- Follow industry trends: Stay updated with the latest developments in your field.
- Take online courses: Platforms like Coursera, edX, and Udacity offer a wide range of programming courses.
- Read technical books and blogs: Deepen your understanding of programming concepts and best practices.
- Attend conferences and workshops: These events provide opportunities to learn from experts and network with other developers.
Remember, every expert was once a beginner. Consistent practice and learning will gradually build your skills and confidence.
Conclusion
Transforming a blank screen into a full solution is a journey that becomes easier and more enjoyable with practice and the right mindset. By breaking down problems, starting small, leveraging resources, and continuously learning, you’ll build the confidence to tackle any coding challenge that comes your way.
Remember, the key is to start. Don’t let the fear of the blank screen paralyze you. With each problem you solve, your skills grow, and your confidence builds. Embrace the process, celebrate your progress, and keep coding!
As you continue your coding journey, platforms like AlgoCademy can be invaluable resources. With interactive tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy can help you progress from beginner-level coding to tackling complex technical interviews. Remember, every line of code you write is a step towards mastery.
So, the next time you face a blank screen, take a deep breath, apply these strategies, and dive in with confidence. You’ve got this!