From Artist to Programmer: Leveraging Creative Skills in Coding
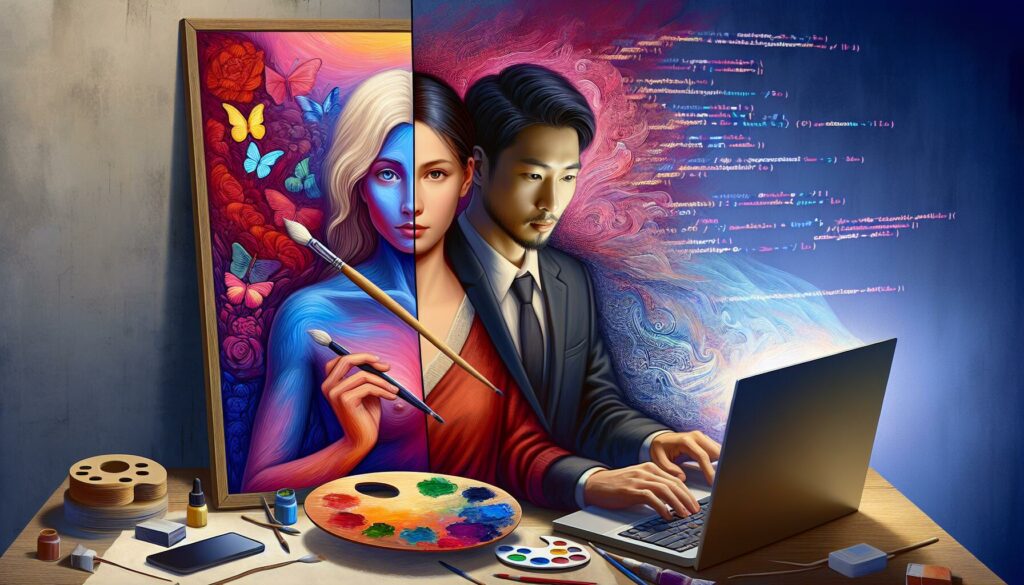
In today’s rapidly evolving digital landscape, the lines between art and technology are increasingly blurred. As a result, many artists are finding themselves drawn to the world of programming, discovering that their creative skills can be leveraged to produce innovative coding solutions. This transition from artist to programmer is not only possible but can lead to unique and valuable contributions in the tech industry. In this article, we’ll explore how artistic abilities can translate into programming prowess and why the combination of creativity and coding can be a powerful force in the digital realm.
The Artistic Mindset in Programming
At first glance, art and programming might seem like polar opposites. One is often perceived as free-flowing and emotionally driven, while the other is thought to be logical and structured. However, upon closer examination, these two disciplines share more commonalities than one might expect.
1. Problem-Solving and Creative Thinking
Artists are natural problem-solvers. When creating a piece of art, they constantly make decisions about composition, color, form, and technique to achieve their desired outcome. This process of creative problem-solving is directly applicable to programming, where developers must find innovative solutions to complex challenges.
For example, when a programmer encounters a bug or needs to optimize code performance, they must think creatively to identify the root cause and develop an effective solution. This often involves looking at the problem from multiple angles, much like an artist considering various perspectives when composing a painting.
2. Attention to Detail
Artists are trained to notice subtle nuances in color, texture, and form. This keen eye for detail translates well to programming, where precision is crucial. In coding, a single misplaced character can cause an entire program to malfunction. The meticulous nature of artists can be a significant asset when it comes to writing clean, error-free code and debugging complex systems.
3. Embracing Iteration and Refinement
The artistic process often involves multiple iterations and refinements before a piece is considered complete. Similarly, in programming, code is rarely perfect on the first try. Developers frequently refactor and optimize their code to improve functionality and efficiency. The artistic mindset of continuous improvement and willingness to revise work aligns perfectly with the iterative nature of software development.
Translating Artistic Skills to Coding Practices
Now that we’ve established the parallels between artistic thinking and programming, let’s explore how specific artistic skills can be applied to various aspects of coding.
1. Visual Design and User Interface Development
Artists with a background in visual design have a natural advantage when it comes to creating user interfaces (UI) and user experience (UX) designs. Their understanding of color theory, layout, and visual hierarchy can be directly applied to crafting intuitive and aesthetically pleasing interfaces.
For instance, a painter turned front-end developer might use their knowledge of color harmony to create a visually appealing color scheme for a website:
<style>
:root {
--primary-color: #3498db;
--secondary-color: #e74c3c;
--accent-color: #f1c40f;
--background-color: #ecf0f1;
--text-color: #2c3e50;
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
.button-primary {
background-color: var(--primary-color);
color: white;
}
.button-secondary {
background-color: var(--secondary-color);
color: white;
}
.highlight {
color: var(--accent-color);
}
</style>
This CSS example demonstrates how an artist’s understanding of color relationships can be applied to create a cohesive and visually appealing color palette for a web application.
2. Storytelling and User Experience Design
Artists who work in narrative mediums, such as writers or filmmakers, can apply their storytelling skills to create engaging user experiences. They understand how to guide a user through a journey, building tension and release, and creating emotional connections. This can be particularly valuable in game development or interactive web experiences.
For example, a writer turned game developer might structure the narrative flow of a game using object-oriented programming concepts:
class StoryNode {
constructor(content, choices) {
this.content = content;
this.choices = choices;
}
displayContent() {
console.log(this.content);
this.displayChoices();
}
displayChoices() {
this.choices.forEach((choice, index) => {
console.log(`${index + 1}. ${choice.text}`);
});
}
makeChoice(choiceIndex) {
return this.choices[choiceIndex].nextNode;
}
}
const introNode = new StoryNode(
"You find yourself at a crossroads in a dark forest...",
[
{ text: "Take the path to the left", nextNode: leftPathNode },
{ text: "Take the path to the right", nextNode: rightPathNode },
]
);
// Game loop
let currentNode = introNode;
while (currentNode) {
currentNode.displayContent();
const choice = getUserChoice(); // Implement this function to get user input
currentNode = currentNode.makeChoice(choice);
}
This JavaScript code demonstrates how storytelling concepts can be translated into a programmatic structure, allowing for the creation of interactive narratives in games or applications.
3. Music and Audio Programming
Musicians and sound artists can bring their expertise to audio programming and sound design in software development. Their understanding of rhythm, harmony, and sound synthesis can be applied to create immersive audio experiences in games, multimedia applications, or even in developing audio processing software.
For instance, a musician might use their knowledge of sound synthesis to create a simple synthesizer in code:
import numpy as np
import sounddevice as sd
def generate_sine_wave(freq, duration, sample_rate=44100):
t = np.linspace(0, duration, int(sample_rate * duration), False)
return np.sin(2 * np.pi * freq * t)
def play_note(frequency, duration):
wave = generate_sine_wave(frequency, duration)
sd.play(wave, samplerate=44100)
sd.wait()
# Play a C major scale
notes = [261.63, 293.66, 329.63, 349.23, 392.00, 440.00, 493.88, 523.25]
for note in notes:
play_note(note, 0.5)
This Python code demonstrates how musical knowledge can be applied to create a simple synthesizer that plays a C major scale. Musicians can build upon this foundation to create more complex audio applications or sound design tools.
4. Sculpture and 3D Modeling
Sculptors and 3D artists can apply their spatial reasoning skills and understanding of form to 3D modeling and computer graphics programming. Their ability to visualize and manipulate objects in three-dimensional space is invaluable in fields such as game development, virtual reality, and computer-aided design (CAD).
For example, a sculptor might use their knowledge of 3D forms to create a simple 3D rendering engine:
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
def create_cube():
vertices = np.array([
[0, 0, 0], [1, 0, 0], [1, 1, 0], [0, 1, 0],
[0, 0, 1], [1, 0, 1], [1, 1, 1], [0, 1, 1]
])
edges = [
(0, 1), (1, 2), (2, 3), (3, 0),
(4, 5), (5, 6), (6, 7), (7, 4),
(0, 4), (1, 5), (2, 6), (3, 7)
]
return vertices, edges
def rotate_3d(vertices, angle_x, angle_y, angle_z):
Rx = np.array([
[1, 0, 0],
[0, np.cos(angle_x), -np.sin(angle_x)],
[0, np.sin(angle_x), np.cos(angle_x)]
])
Ry = np.array([
[np.cos(angle_y), 0, np.sin(angle_y)],
[0, 1, 0],
[-np.sin(angle_y), 0, np.cos(angle_y)]
])
Rz = np.array([
[np.cos(angle_z), -np.sin(angle_z), 0],
[np.sin(angle_z), np.cos(angle_z), 0],
[0, 0, 1]
])
R = np.dot(Rz, np.dot(Ry, Rx))
return np.dot(vertices, R.T)
vertices, edges = create_cube()
rotated_vertices = rotate_3d(vertices, np.pi/4, np.pi/4, 0)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
for edge in edges:
ax.plot3D(rotated_vertices[edge, 0], rotated_vertices[edge, 1], rotated_vertices[edge, 2], 'b')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
This Python code demonstrates how a sculptor’s understanding of 3D forms and spatial relationships can be applied to create a simple 3D rendering engine that generates and rotates a cube in 3D space.
The Power of Interdisciplinary Thinking
The fusion of artistic creativity and programming skills can lead to innovative solutions and unique approaches to problem-solving in the tech industry. This interdisciplinary thinking brings several advantages:
1. Enhanced User-Centric Design
Artists-turned-programmers often have a deep understanding of human perception and emotional responses. This insight can be invaluable when designing user interfaces and experiences that are not only functional but also emotionally engaging and intuitive to use.
2. Novel Approaches to Problem-Solving
The diverse background of an artist can bring fresh perspectives to coding challenges. They may approach problems from unconventional angles, leading to innovative solutions that might not occur to those with a purely technical background.
3. Bridging the Gap Between Technical and Non-Technical Teams
Artists who become programmers can serve as valuable intermediaries between technical and non-technical team members. Their ability to communicate in both “languages” can facilitate better collaboration and understanding across different departments.
4. Pushing the Boundaries of Digital Art
With their combined artistic vision and technical skills, artist-programmers are well-positioned to create cutting-edge digital art experiences. This could include interactive installations, generative art, or innovative digital storytelling platforms.
Challenges and Learning Curve
While the transition from artist to programmer can be incredibly rewarding, it’s not without its challenges. Artists venturing into the world of coding may face several hurdles:
1. Learning the Technical Foundations
Programming requires a solid understanding of computer science concepts, algorithms, and data structures. Artists may need to invest significant time and effort in learning these foundational elements.
2. Adapting to Logical Thinking
While artists are creative problem-solvers, they may need to adapt to the more structured and logical thinking required in programming. This can involve learning to break down problems into smaller, manageable steps and thinking in terms of algorithms and data flow.
3. Keeping Up with Rapid Technological Changes
The tech industry evolves at a breakneck pace, with new languages, frameworks, and tools constantly emerging. Artists transitioning to programming need to develop a habit of continuous learning to stay current with the latest technologies.
4. Balancing Creativity with Technical Constraints
Artists may sometimes find it challenging to reconcile their creative vision with technical limitations or performance considerations. Learning to find creative solutions within these constraints is an important skill to develop.
Resources for Artists Transitioning to Programming
For artists interested in exploring the world of programming, there are numerous resources available to help bridge the gap:
1. Online Coding Courses
Platforms like Codecademy, freeCodeCamp, and Coursera offer a wide range of programming courses, many of which are designed for beginners and can be particularly appealing to those with creative backgrounds.
2. Creative Coding Communities
Communities like Processing, OpenFrameworks, and p5.js are specifically designed for artists and designers interested in coding. These platforms provide tools and resources that make it easier for creatives to start programming visual and interactive projects.
3. Art-Focused Coding Bootcamps
Some coding bootcamps offer programs specifically tailored to artists and designers, focusing on areas like front-end development, UX/UI design, or creative technology.
4. Books on Creative Coding
Books like “Generative Design” by Hartmut Bohnacker, “Code as Creative Medium” by Golan Levin and Tega Brain, and “The Nature of Code” by Daniel Shiffman offer insights into the intersection of art and programming.
5. Open Source Projects
Participating in open source projects can provide hands-on experience and exposure to real-world coding practices. Many projects welcome contributions from individuals with diverse backgrounds, including artists.
Conclusion
The journey from artist to programmer is a transformative experience that can lead to unique and valuable contributions in the tech industry. By leveraging their creative skills, artists can bring fresh perspectives, innovative solutions, and enhanced user-centric design to the world of coding.
While the learning curve may be steep, the potential rewards are significant. Artist-programmers are well-positioned to push the boundaries of digital creativity, bridge the gap between technical and non-technical teams, and create truly innovative digital experiences.
As the lines between art and technology continue to blur, the demand for individuals who can seamlessly blend creativity with technical skills will only grow. For artists willing to embrace the challenge, the world of programming offers a canvas of unlimited possibilities, where their unique vision and creative problem-solving abilities can truly shine.
Whether you’re an artist curious about coding or a programmer looking to tap into your creative side, remember that the fusion of these two disciplines can lead to extraordinary outcomes. The future of technology is not just about functionality; it’s about creating meaningful, engaging, and beautiful experiences. And who better to lead this charge than those who have mastered both the brush and the keyboard?