Floyd-Warshall Algorithm: A Comprehensive Guide for Coding Interviews
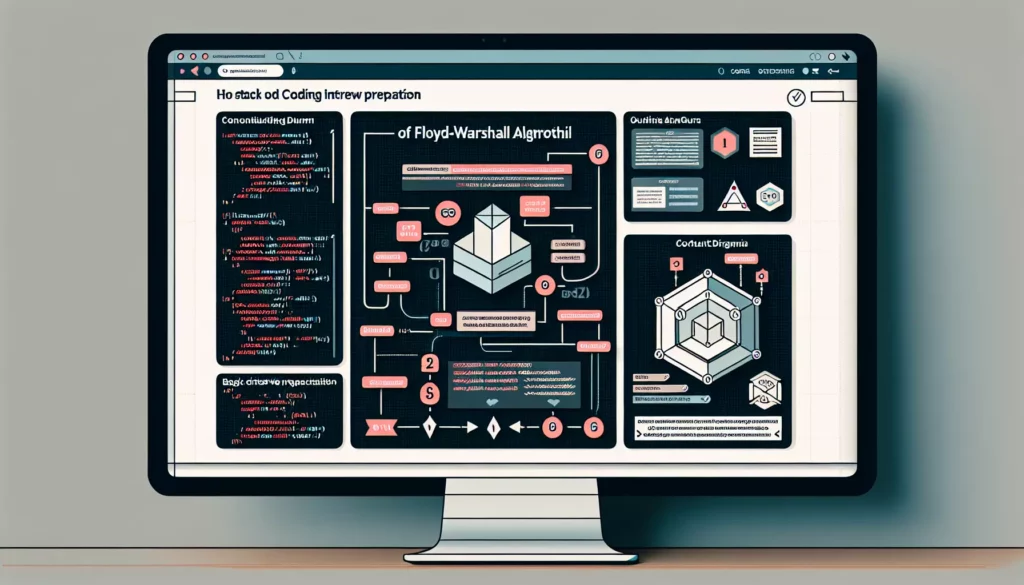
In the world of computer science and algorithmic problem-solving, the Floyd-Warshall algorithm stands out as a powerful tool for solving the all-pairs shortest path problem in weighted graphs. As you prepare for coding interviews, especially those with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding this algorithm can give you a significant edge. In this comprehensive guide, we’ll dive deep into the Floyd-Warshall algorithm, exploring its concepts, implementation, time complexity, and practical applications.
Table of Contents
- Introduction to the Floyd-Warshall Algorithm
- How the Floyd-Warshall Algorithm Works
- Implementing the Floyd-Warshall Algorithm
- Time and Space Complexity Analysis
- Real-world Applications
- Optimizations and Variations
- Interview Tips and Common Questions
- Practice Problems
- Conclusion
1. Introduction to the Floyd-Warshall Algorithm
The Floyd-Warshall algorithm, named after Robert Floyd and Stephen Warshall, is a graph analysis algorithm used to find the shortest paths between all pairs of vertices in a weighted graph. It’s particularly useful when you need to compute the shortest distances between every pair of nodes in a graph, making it a go-to solution for various problems in network routing, transportation systems, and more.
Key characteristics of the Floyd-Warshall algorithm include:
- It works on weighted graphs, including those with negative edge weights (but no negative cycles).
- It can detect the presence of negative cycles in the graph.
- It’s an example of dynamic programming, building up the solution incrementally.
- It has a time complexity of O(V³), where V is the number of vertices in the graph.
2. How the Floyd-Warshall Algorithm Works
The Floyd-Warshall algorithm operates on the principle of dynamic programming. It iteratively improves the shortest path estimates between all pairs of vertices by considering the possibility of going through an intermediate vertex.
Here’s a step-by-step breakdown of how the algorithm works:
- Initialization: Create a distance matrix D where D[i][j] represents the shortest known distance from vertex i to vertex j. Initialize this matrix with the direct edge weights from the input graph. If there’s no direct edge between i and j, set D[i][j] to infinity.
- Iterative Improvement: For each vertex k (as an intermediate vertex), and for each pair of vertices (i, j):
- Check if the path from i to j through k is shorter than the current known shortest path from i to j.
- If it is, update D[i][j] with this new shorter distance.
- Repeat: Repeat step 2 for all vertices k = 1 to V, where V is the total number of vertices.
- Result: After all iterations, D[i][j] will contain the shortest distance from vertex i to vertex j.
The key insight here is that at each step, we’re considering whether going through a new intermediate vertex k can provide a shorter path between any two vertices i and j.
3. Implementing the Floyd-Warshall Algorithm
Let’s implement the Floyd-Warshall algorithm in Python. This implementation will work with a weighted graph represented as an adjacency matrix.
def floyd_warshall(graph):
V = len(graph)
dist = [row[:] for row in graph] # Create a copy of the input graph
# Iterate through all possible intermediate vertices
for k in range(V):
# For each pair of vertices (i, j)
for i in range(V):
for j in range(V):
# If vertex k is on the shortest path from i to j,
# update the value of dist[i][j]
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
return dist
# Example usage
INF = float('inf')
graph = [
[0, 5, INF, 10],
[INF, 0, 3, INF],
[INF, INF, 0, 1],
[INF, INF, INF, 0]
]
result = floyd_warshall(graph)
# Print the shortest distance matrix
for row in result:
print(row)
In this implementation:
- We start by creating a copy of the input graph to store our distance matrix.
- We then iterate through all possible intermediate vertices k.
- For each k, we check if going through k provides a shorter path for any pair of vertices i and j.
- If a shorter path is found, we update our distance matrix.
- After all iterations, the distance matrix contains the shortest paths between all pairs of vertices.
4. Time and Space Complexity Analysis
Understanding the time and space complexity of the Floyd-Warshall algorithm is crucial for assessing its applicability to different problem sizes and constraints.
Time Complexity
The time complexity of the Floyd-Warshall algorithm is O(V³), where V is the number of vertices in the graph. This cubic time complexity comes from the three nested loops in the algorithm:
- The outermost loop iterates V times (for each potential intermediate vertex).
- For each iteration of the outermost loop, we have two inner loops that each iterate V times.
- This results in V * V * V = V³ operations.
While this cubic time complexity makes the algorithm less suitable for very large graphs, it’s highly efficient for small to medium-sized graphs, especially when you need to find all pairs of shortest paths.
Space Complexity
The space complexity of the Floyd-Warshall algorithm is O(V²). This comes from the distance matrix we create to store the shortest distances between all pairs of vertices. The matrix has V rows and V columns, resulting in V² space usage.
It’s worth noting that the algorithm can be implemented with O(V²) space complexity without needing any additional data structures beyond the input graph and the output distance matrix.
Comparison with Other Algorithms
When comparing Floyd-Warshall with other shortest path algorithms:
- Dijkstra’s Algorithm: Dijkstra’s has a better time complexity of O((V+E)log V) for sparse graphs when finding single-source shortest paths. However, to find all pairs of shortest paths, you’d need to run Dijkstra’s V times, resulting in O(V(V+E)log V), which can be worse than Floyd-Warshall for dense graphs.
- Bellman-Ford Algorithm: Bellman-Ford has a time complexity of O(VE) for single-source shortest paths and can handle negative edge weights. For all pairs, running it V times would result in O(V²E), which is generally worse than Floyd-Warshall.
- Johnson’s Algorithm: For sparse graphs, Johnson’s algorithm, which combines Dijkstra’s and Bellman-Ford, can be more efficient for all-pairs shortest paths with a time complexity of O(V²log V + VE).
5. Real-world Applications
The Floyd-Warshall algorithm finds applications in various real-world scenarios where understanding the relationships or distances between all pairs of entities in a system is crucial. Here are some notable applications:
1. Network Routing
In computer networks, the Floyd-Warshall algorithm can be used to determine the most efficient routing paths between all pairs of nodes. This is particularly useful in:
- Optimizing data packet routing in telecommunications networks
- Finding the shortest path for information flow in distributed systems
- Calculating the minimum latency between all pairs of servers in a data center
2. Transportation Systems
The algorithm is valuable in planning and optimizing transportation networks:
- Finding the shortest routes between all pairs of cities in a road network
- Optimizing flight paths in airline route planning
- Calculating minimum travel times in public transit systems
3. Graph Theory and Analysis
In graph theory and network analysis, Floyd-Warshall is used for:
- Computing the transitive closure of a graph
- Detecting cycles in a graph, including negative cycles
- Analyzing the connectivity and centrality of nodes in social networks
4. Computer Graphics
In computer graphics and game development:
- Pathfinding in game maps or 3D environments
- Calculating minimum distances for character movement in strategy games
5. Operations Research
In operations research and logistics:
- Solving the all-pairs shortest path problem in supply chain optimization
- Minimizing transportation costs in logistics networks
6. Optimizations and Variations
While the basic Floyd-Warshall algorithm is powerful, there are several optimizations and variations that can enhance its performance or extend its capabilities:
1. Path Reconstruction
One common extension is to not only compute the shortest distances but also to reconstruct the actual paths. This can be done by maintaining an additional matrix to keep track of the intermediate vertices:
def floyd_warshall_with_path(graph):
V = len(graph)
dist = [row[:] for row in graph]
next = [[j if dist[i][j] != float('inf') else None for j in range(V)] for i in range(V)]
for k in range(V):
for i in range(V):
for j in range(V):
if dist[i][k] + dist[k][j] < dist[i][j]:
dist[i][j] = dist[i][k] + dist[k][j]
next[i][j] = next[i][k]
return dist, next
def reconstruct_path(next, u, v):
if next[u][v] is None:
return []
path = [u]
while u != v:
u = next[u][v]
path.append(u)
return path
2. Early Termination for Negative Cycles
If the graph potentially contains negative cycles, you can modify the algorithm to detect them early and terminate:
def floyd_warshall_negative_cycle(graph):
V = len(graph)
dist = [row[:] for row in graph]
for k in range(V):
for i in range(V):
for j in range(V):
if dist[i][k] + dist[k][j] < dist[i][j]:
dist[i][j] = dist[i][k] + dist[k][j]
if i == j and dist[i][j] < 0:
return "Negative cycle detected"
return dist
3. Space Optimization
For very large graphs, you can optimize space usage by using only two rows of the distance matrix at a time:
def floyd_warshall_space_optimized(graph):
V = len(graph)
dist = [row[:] for row in graph]
for k in range(V):
next_dist = [row[:] for row in dist]
for i in range(V):
for j in range(V):
next_dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
dist = next_dist
return dist
4. Parallel Implementation
For large graphs, a parallel implementation can significantly speed up the computation. The innermost loop of the algorithm can be parallelized:
import multiprocessing
def parallel_floyd_warshall(graph):
V = len(graph)
dist = [row[:] for row in graph]
def update_row(args):
i, k = args
for j in range(V):
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
with multiprocessing.Pool() as pool:
for k in range(V):
pool.map(update_row, [(i, k) for i in range(V)])
return dist
5. Johnson’s Algorithm for Sparse Graphs
For sparse graphs, Johnson’s algorithm can be more efficient. It uses a combination of Bellman-Ford and Dijkstra’s algorithms:
import heapq
def johnsons_algorithm(graph):
V = len(graph)
# Add a new vertex
for i in range(V):
graph[i].append(0)
graph.append([0] * (V + 1))
# Run Bellman-Ford
h = bellman_ford(graph, V)
if h is None:
return "Negative cycle detected"
# Reweight the edges
for i in range(V + 1):
for j in range(V + 1):
if graph[i][j] != float('inf'):
graph[i][j] += h[i] - h[j]
# Run Dijkstra's for each vertex
dist = [[float('inf')] * V for _ in range(V)]
for i in range(V):
dijkstras(graph, i, dist[i])
# Adjust the distances
for i in range(V):
for j in range(V):
dist[i][j] += h[j] - h[i]
return dist
def bellman_ford(graph, s):
V = len(graph)
dist = [float('inf')] * V
dist[s] = 0
for _ in range(V - 1):
for u in range(V):
for v in range(V):
if graph[u][v] != float('inf'):
dist[v] = min(dist[v], dist[u] + graph[u][v])
# Check for negative cycles
for u in range(V):
for v in range(V):
if graph[u][v] != float('inf') and dist[u] + graph[u][v] < dist[v]:
return None
return dist
def dijkstras(graph, s, dist):
pq = [(0, s)]
dist[s] = 0
while pq:
d, u = heapq.heappop(pq)
if d > dist[u]:
continue
for v in range(len(graph)):
if graph[u][v] != float('inf'):
new_dist = dist[u] + graph[u][v]
if new_dist < dist[v]:
dist[v] = new_dist
heapq.heappush(pq, (new_dist, v))
7. Interview Tips and Common Questions
When preparing for coding interviews, especially for top tech companies, it’s crucial to not only understand the Floyd-Warshall algorithm but also be ready to discuss its nuances and applications. Here are some tips and common questions you might encounter:
Tips for Discussing Floyd-Warshall in Interviews
- Understand the trade-offs: Be prepared to discuss when Floyd-Warshall is preferred over other shortest path algorithms like Dijkstra’s or Bellman-Ford.
- Know the limitations: Understand that Floyd-Warshall doesn’t work with negative cycles and be ready to explain why.
- Think about optimizations: Consider how you might optimize the algorithm for specific scenarios (e.g., sparse graphs, memory constraints).
- Real-world applications: Have a few concrete examples of where Floyd-Warshall is used in real-world systems.
- Code implementation: Be able to implement the basic algorithm without referencing external resources.
Common Interview Questions
- Q: What is the time and space complexity of the Floyd-Warshall algorithm?
A: The time complexity is O(V³) and the space complexity is O(V²), where V is the number of vertices in the graph. - Q: How does Floyd-Warshall handle negative edge weights?
A: Floyd-Warshall can handle negative edge weights, but it cannot handle negative cycles. If there’s a negative cycle, the algorithm will not produce correct results. - Q: Can Floyd-Warshall be used to detect negative cycles? How?
A: Yes, if after running the algorithm, any diagonal element in the distance matrix is negative, it indicates the presence of a negative cycle. - Q: How would you modify Floyd-Warshall to reconstruct the shortest paths?
A: You can maintain an additional matrix to keep track of the intermediate vertices used in the shortest paths. This allows for path reconstruction after the algorithm completes. - Q: In what scenarios would you choose Floyd-Warshall over Dijkstra’s algorithm?
A: Floyd-Warshall is preferred when you need all-pairs shortest paths, especially in dense graphs, or when there are negative edge weights (but no negative cycles). Dijkstra’s is better for single-source shortest paths in non-negative weighted graphs. - Q: How would you optimize Floyd-Warshall for sparse graphs?
A: For sparse graphs, Johnson’s algorithm, which combines Bellman-Ford and Dijkstra’s algorithms, is often more efficient. It has a better time complexity for sparse graphs. - Q: Can Floyd-Warshall be parallelized? How?
A: Yes, the inner loop of Floyd-Warshall can be parallelized. Each calculation of dist[i][j] in a given iteration is independent and can be computed in parallel.
8. Practice Problems
To solidify your understanding of the Floyd-Warshall algorithm and prepare for coding interviews, here are some practice problems you can work on:
- All-Pairs Shortest Path: Implement the basic Floyd-Warshall algorithm to find the shortest distances between all pairs of vertices in a weighted graph.
- Path Reconstruction: Modify the Floyd-Warshall algorithm to not only find the shortest distances but also reconstruct the actual paths between all pairs of vertices.
- Transitive Closure: Use Floyd-Warshall to find the transitive closure of a directed graph. The transitive closure of a graph is a graph where there’s an edge between vertices i and j if there’s a path from i to j in the original graph.
- Negative Cycle Detection: Implement a version of Floyd-Warshall that can detect the presence of negative cycles in the graph.
- Maximum Capacity Paths: Given a graph where edge weights represent capacities (e.g., bandwidth in a network), use a modified Floyd-Warshall to find the maximum capacity path between all pairs of vertices.
- Minimum Vertex Paths: Given an unweighted graph, use Floyd-Warshall to find paths between all pairs of vertices that use the minimum number of intermediate vertices.
- Regular Expression Matching: Use Floyd-Warshall to solve the regular expression matching problem for patterns without Kleene star.
- Graph Diameter: Use Floyd-Warshall to find the diameter of a graph (the maximum shortest path distance between any two vertices).
- Arbitrage Detection: Given a graph of currency exchange rates, use Floyd-Warshall to detect arbitrage opportunities.
- Minimax Paths: In a graph where edge weights represent costs or risks, find paths between all pairs of vertices that minimize the maximum edge weight along the path.
For each of these problems, try to:
- Implement the solution from scratch
- Analyze the time and space complexity of your solution
- Consider edge cases and how to handle them
- Think about potential optimizations or variations of the problem
Remember, the key to mastering algorithms like Floyd-Warshall is consistent practice and understanding how to apply the core concepts to different problem scenarios.
9. Conclusion
The Floyd-Warshall algorithm is a powerful tool in the arsenal of any software engineer or computer scientist. Its ability to solve the all-pairs shortest path problem efficiently makes it invaluable in various applications, from network routing to operations research.
Key takeaways from this guide include:
- Understanding the core principle of the Floyd-Warshall algorithm: iteratively improving path estimates by considering intermediate vertices.
- Recognizing the algorithm’s strengths (handling negative weights, finding all-pairs shortest paths) and limitations (cubic time complexity, inability to handle negative cycles).
- Being able to implement the basic algorithm and its variations, such as path reconstruction and negative cycle detection.
- Knowing when to apply Floyd-Warshall versus other shortest path algorithms like Dijkstra’s or Bellman-Ford.
- Appreciating the algorithm’s applications in real-world scenarios, from computer networking to transportation systems.
As you prepare for coding interviews, especially with top tech companies, remember that understanding algorithms like Floyd-Warshall goes beyond mere implementation. Being able to discuss its complexities, trade-offs, and applications demonstrates a deep understanding of algorithmic problem-solving.
Continue to practice implementing the algorithm, solving related problems, and thinking about how you might optimize or adapt it for different scenarios. This comprehensive understanding will not only serve you well in interviews but also in your career as a software engineer, where efficient algorithm selection and implementation can make a significant impact on system performance and capabilities.
Keep exploring, keep practicing, and remember that mastering algorithms like Floyd-Warshall is a journey of continuous learning and application. Good luck with your interview preparation and future coding challenges!