Finding the Least Common Denominator: A Comprehensive Guide for Programmers
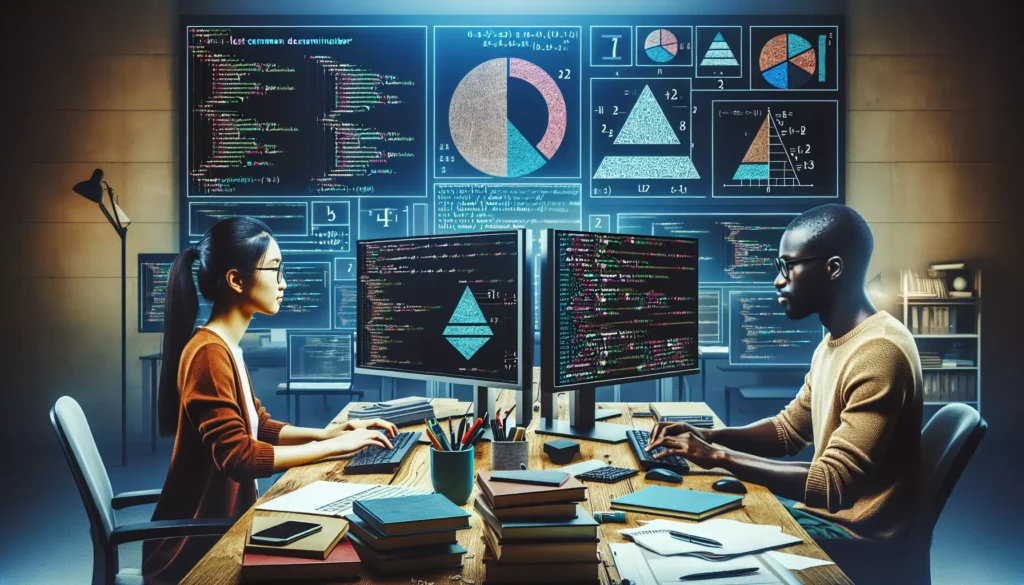
Understanding the least common denominator (LCD) is fundamental for many computational tasks, from fraction operations to algorithm optimization. While it might seem like a basic mathematical concept, its applications in programming are widespread and significant. In this comprehensive guide, we’ll explore what the least common denominator is, how to calculate it efficiently, and how to implement it in code with various programming languages.
What is the Least Common Denominator?
The least common denominator (LCD) is the smallest positive number that is divisible by all denominators in a set of fractions. It’s closely related to the least common multiple (LCM) of the denominators.
For example, if we have fractions 1/4 and 3/6, the LCD would be 12, as it’s the smallest number that both 4 and 6 can divide into evenly.
Understanding the LCD is crucial for:
- Adding or subtracting fractions
- Comparing fraction sizes
- Simplifying complex fraction operations
- Solving certain types of algorithms efficiently
The Relationship Between LCD and LCM
The least common denominator of a set of fractions is actually the least common multiple (LCM) of their denominators. This relationship simplifies our approach: to find the LCD of fractions, we compute the LCM of their denominators.
Calculating the Least Common Denominator
There are several methods to calculate the LCD (or LCM):
Method 1: Prime Factorization
This method involves breaking down each denominator into its prime factors, then taking the highest power of each prime factor that appears.
For example, to find the LCD of 12 and 18:
- 12 = 2² × 3
- 18 = 2 × 3²
- Take the highest power of each: 2² and 3²
- Multiply them: 2² × 3² = 4 × 9 = 36
Therefore, the LCD of 12 and 18 is 36.
Method 2: Using GCD (Greatest Common Divisor)
A more efficient approach uses the relationship between LCM and GCD:
LCM(a, b) = (a × b) / GCD(a, b)
This formula can be extended for multiple numbers by finding the LCM of the first two numbers, then finding the LCM of that result and the next number, and so on.
Method 3: Brute Force
A simple but less efficient approach is to start with the largest denominator and increment until we find a number divisible by all denominators.
Implementing LCD Calculation in Code
Let’s implement these methods in various programming languages:
Python Implementation
import math
from functools import reduce
# Method 1: Using GCD
def lcm_of_two_numbers(a, b):
return a * b // math.gcd(a, b)
def find_lcd(denominators):
return reduce(lcm_of_two_numbers, denominators)
# Example usage
fractions_denominators = [4, 6, 8]
print(f"The LCD of {fractions_denominators} is {find_lcd(fractions_denominators)}")
# Method 2: Brute force approach
def find_lcd_brute_force(denominators):
max_denom = max(denominators)
lcd = max_denom
while True:
if all(lcd % d == 0 for d in denominators):
return lcd
lcd += max_denom
# Example usage
print(f"Using brute force, the LCD is {find_lcd_brute_force(fractions_denominators)}")
JavaScript Implementation
// Helper function to find GCD using Euclidean algorithm
function gcd(a, b) {
while (b) {
let t = b;
b = a % b;
a = t;
}
return a;
}
// Method 1: Using GCD
function lcm(a, b) {
return (a * b) / gcd(a, b);
}
function findLCD(denominators) {
return denominators.reduce((acc, curr) => lcm(acc, curr));
}
// Example usage
const fractionDenominators = [4, 6, 8];
console.log(`The LCD of ${fractionDenominators} is ${findLCD(fractionDenominators)}`);
// Method 2: Brute force approach
function findLCDBruteForce(denominators) {
const maxDenom = Math.max(...denominators);
let lcd = maxDenom;
while (true) {
if (denominators.every(d => lcd % d === 0)) {
return lcd;
}
lcd += maxDenom;
}
}
// Example usage
console.log(`Using brute force, the LCD is ${findLCDBruteForce(fractionDenominators)}`);
Java Implementation
import java.util.Arrays;
public class LeastCommonDenominator {
// Helper method to find GCD using Euclidean algorithm
public static int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
// Method 1: Using GCD
public static int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
public static int findLCD(int[] denominators) {
int result = denominators[0];
for (int i = 1; i < denominators.length; i++) {
result = lcm(result, denominators[i]);
}
return result;
}
// Method 2: Brute force approach
public static int findLCDBruteForce(int[] denominators) {
int maxDenom = Arrays.stream(denominators).max().getAsInt();
int lcd = maxDenom;
while (true) {
boolean isDivisible = true;
for (int d : denominators) {
if (lcd % d != 0) {
isDivisible = false;
break;
}
}
if (isDivisible) {
return lcd;
}
lcd += maxDenom;
}
}
public static void main(String[] args) {
int[] fractionDenominators = {4, 6, 8};
System.out.println("The LCD of " + Arrays.toString(fractionDenominators) +
" is " + findLCD(fractionDenominators));
System.out.println("Using brute force, the LCD is " +
findLCDBruteForce(fractionDenominators));
}
}
Time and Space Complexity Analysis
Understanding the efficiency of our LCD algorithms is important for optimizing performance:
GCD-based Method
- Time Complexity: O(n log m), where n is the number of denominators and m is the maximum value among them. The Euclidean algorithm for GCD runs in O(log m) time.
- Space Complexity: O(1), as we only need a constant amount of space regardless of input size.
Brute Force Method
- Time Complexity: O(n × m), where n is the number of denominators and m is the LCD. This can be much slower than the GCD-based method.
- Space Complexity: O(1)
Practical Applications of LCD in Programming
1. Fraction Operations
The most direct application is implementing fraction arithmetic:
class Fraction {
private int numerator;
private int denominator;
public Fraction(int numerator, int denominator) {
this.numerator = numerator;
this.denominator = denominator;
simplify();
}
private void simplify() {
int gcd = gcd(Math.abs(numerator), denominator);
numerator /= gcd;
denominator /= gcd;
}
private int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
private int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
public Fraction add(Fraction other) {
int lcd = lcm(this.denominator, other.denominator);
int newNumerator = this.numerator * (lcd / this.denominator) +
other.numerator * (lcd / other.denominator);
return new Fraction(newNumerator, lcd);
}
@Override
public String toString() {
return numerator + "/" + denominator;
}
}
2. Solving Linear Diophantine Equations
LCD is useful in solving equations of the form ax + by = c, where a, b, c are integers and x, y are unknown integers.
3. Time Scheduling Problems
When calculating intervals or frequencies, LCD can help find the common cycle time.
4. Memory Management
In systems with multiple memory blocks of different sizes, LCD can help determine efficient allocation strategies.
Common Pitfalls and Optimization Tips
Potential Issues
- Integer Overflow: When calculating (a × b) / gcd(a, b), the intermediate product might overflow. A safer implementation is:
int lcm(int a, int b) { return a / gcd(a, b) * b; // Divide first to prevent overflow }
- Zero Handling: Be careful with inputs containing zero. The LCD is undefined if any denominator is zero.
- Negative Numbers: Ensure your GCD function handles negative numbers correctly.
Optimization Tips
- Binary GCD Algorithm: For very large numbers, consider using the binary GCD algorithm (Stein’s algorithm), which avoids division operations.
- Prime Factorization Caching: If you’re repeatedly calculating LCDs for the same set of numbers, consider caching prime factorizations.
- Early Termination: In the brute force method, you can often add optimization checks to terminate early.
Advanced Topic: Finding LCD for Rational Numbers
When working with rational numbers (fractions), finding the LCD becomes more complex. We need to:
- Express each rational number as an irreducible fraction
- Find the LCD of all denominators
class Rational {
private int numerator;
private int denominator;
// Constructor and simplify method as before
public static int findLCDForRationals(Rational[] rationals) {
int[] denominators = new int[rationals.length];
for (int i = 0; i < rationals.length; i++) {
denominators[i] = rationals[i].denominator;
}
return findLCD(denominators);
}
// findLCD implementation as before
}
Testing Your LCD Implementation
Proper testing is essential to ensure your LCD function works correctly. Here’s a testing approach:
// Test cases for LCD function
function testLCDFunction() {
const testCases = [
{ input: [2, 3], expected: 6 },
{ input: [4, 6], expected: 12 },
{ input: [5, 7, 11], expected: 385 },
{ input: [2, 4, 8], expected: 8 },
{ input: [3, 9, 21], expected: 63 }
];
for (const testCase of testCases) {
const result = findLCD(testCase.input);
console.assert(
result === testCase.expected,
`For input ${testCase.input}, expected ${testCase.expected} but got ${result}`
);
}
console.log("All tests passed!");
}
testLCDFunction();
Conclusion
The least common denominator is a fundamental mathematical concept with numerous applications in programming. Whether you’re implementing fraction arithmetic, solving scheduling problems, or optimizing memory allocation, understanding how to efficiently calculate the LCD can significantly improve your algorithmic solutions.
By mastering the relationship between LCD and LCM, and implementing efficient algorithms for their computation, you’ll add a valuable tool to your programming toolkit. Remember to consider the potential pitfalls, especially when dealing with large numbers or special cases like zeros and negative values.
As you continue to develop your programming skills, these fundamental mathematical concepts will serve as building blocks for more complex algorithms and solutions. Practice implementing LCD calculations in different programming languages and explore how they can be applied to solve real-world problems.
Happy coding!