Final Checklist Before Your Coding Interview Day: 100 Essential Points to Remember
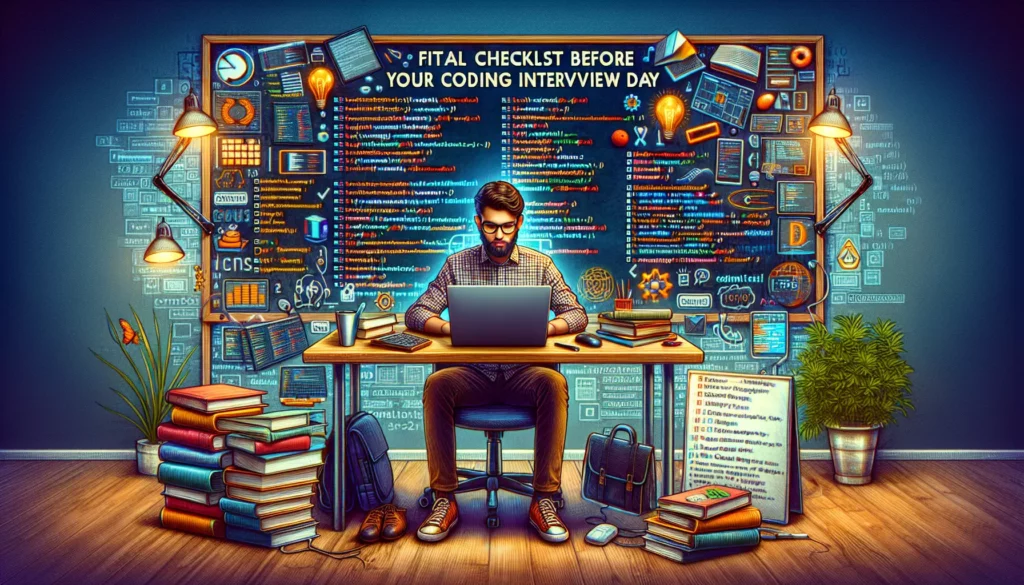
Preparing for a coding interview can be an intense and nerve-wracking experience. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, thorough preparation is key to success. To help you feel confident and ready on the big day, we’ve compiled a comprehensive checklist of 100 essential points to remember before your coding interview. Let’s dive in!
Technical Preparation
1. Data Structures
- Review arrays and their time complexities
- Understand linked lists (singly and doubly)
- Master stack and queue implementations
- Know how to use hash tables effectively
- Refresh your knowledge of trees (binary, AVL, Red-Black)
- Practice with graphs (directed and undirected)
- Understand heaps (min-heap and max-heap)
- Review tries for string-related problems
2. Algorithms
- Master sorting algorithms (quicksort, mergesort, heapsort)
- Understand searching algorithms (binary search, depth-first search, breadth-first search)
- Practice dynamic programming problems
- Review greedy algorithms
- Understand divide and conquer strategies
- Master recursion and when to use it
- Know how to implement backtracking algorithms
- Understand the basics of graph algorithms (Dijkstra’s, Bellman-Ford)
3. Time and Space Complexity
- Review Big O notation
- Understand time complexity analysis
- Practice space complexity analysis
- Know common time complexities (O(1), O(log n), O(n), O(n log n), O(n^2))
- Be able to optimize solutions for better time/space complexity
4. Problem-Solving Strategies
- Practice breaking down complex problems into smaller steps
- Learn to identify patterns in problems
- Develop a systematic approach to problem-solving
- Practice explaining your thought process out loud
- Learn to ask clarifying questions when needed
5. Coding Practice
- Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Practice writing clean, readable code
- Get comfortable with whiteboard coding
- Time yourself while solving problems
- Review and understand multiple solutions to each problem
Language-Specific Preparation
6. Choose Your Primary Language
- Decide on the programming language you’ll use in the interview
- Ensure you’re comfortable with its syntax and features
- Review language-specific best practices and conventions
7. Language Fundamentals
- Review basic syntax and control structures
- Understand object-oriented programming concepts (if applicable)
- Know how to work with arrays and strings efficiently
- Understand memory management in your chosen language
- Review common built-in functions and libraries
8. Data Structure Implementations
- Know how to implement basic data structures in your chosen language
- Understand language-specific optimizations for data structures
- Be familiar with the standard library’s data structure implementations
Behavioral Preparation
9. Company Research
- Research the company’s history and mission
- Understand the company’s products or services
- Read recent news or press releases about the company
- Familiarize yourself with the company’s culture and values
10. Prepare Your Personal Pitch
- Craft a concise introduction about yourself
- Highlight relevant experience and skills
- Practice delivering your pitch confidently
11. Behavioral Questions
- Prepare answers for common behavioral questions
- Use the STAR method (Situation, Task, Action, Result) for structuring responses
- Have specific examples ready to illustrate your skills and experiences
- Practice answering questions about teamwork and conflict resolution
12. Questions for the Interviewer
- Prepare thoughtful questions about the role and company
- Ask about team dynamics and project management methodologies
- Inquire about growth opportunities within the company
Interview Day Preparation
13. Logistics
- Confirm the interview time and format (in-person or virtual)
- Know the exact location or video conferencing details
- Plan your route and arrival time (if in-person)
- Test your internet connection and equipment (if virtual)
- Have a backup plan for technical issues (if virtual)
14. What to Bring
- Bring multiple copies of your resume
- Carry a notepad and pen
- Bring a water bottle
- Have your ID ready (if required for building access)
15. Dress Code
- Research the company’s dress code
- Choose appropriate attire (usually business casual for tech interviews)
- Ensure your clothes are clean and wrinkle-free
16. Mental Preparation
- Get a good night’s sleep before the interview
- Eat a healthy meal before the interview
- Practice relaxation techniques to manage anxiety
- Remind yourself of your strengths and accomplishments
- Arrive early to allow time to compose yourself
During the Interview
17. Communication
- Greet the interviewer professionally
- Maintain good eye contact
- Speak clearly and at a moderate pace
- Listen actively and ask for clarification when needed
- Think out loud while solving problems
18. Problem-Solving Approach
- Read the problem carefully and ask clarifying questions
- Discuss your approach before starting to code
- Consider edge cases and potential optimizations
- Write clean, well-commented code
- Test your solution with sample inputs
19. Handling Difficult Questions
- Stay calm if you encounter a challenging problem
- Break down the problem into smaller steps
- Communicate your thought process, even if you’re unsure
- Ask for hints if you’re completely stuck
- Be open to feedback and suggestions from the interviewer
20. Closing the Interview
- Ask your prepared questions about the role and company
- Express your enthusiasm for the position
- Thank the interviewer for their time
- Ask about the next steps in the hiring process
Post-Interview
21. Follow-Up
- Send a thank-you email within 24 hours
- Reflect on your performance and areas for improvement
- Follow up if you haven’t heard back within the specified timeframe
22. Continuous Improvement
- Continue practicing coding problems regularly
- Stay updated with industry trends and technologies
- Network with other professionals in your field
- Consider contributing to open-source projects
- Keep refining your skills and expanding your knowledge
Conclusion
Preparing for a coding interview is a challenging but rewarding process. By following this comprehensive checklist, you’ll be well-equipped to tackle both the technical and non-technical aspects of your interview. Remember, practice and preparation are key to success. Stay confident, be yourself, and showcase your problem-solving skills.
Good luck with your upcoming interview! With thorough preparation and the right mindset, you’ll be well on your way to landing your dream job in the tech industry.