Figma Technical Interview Prep: A Comprehensive Guide
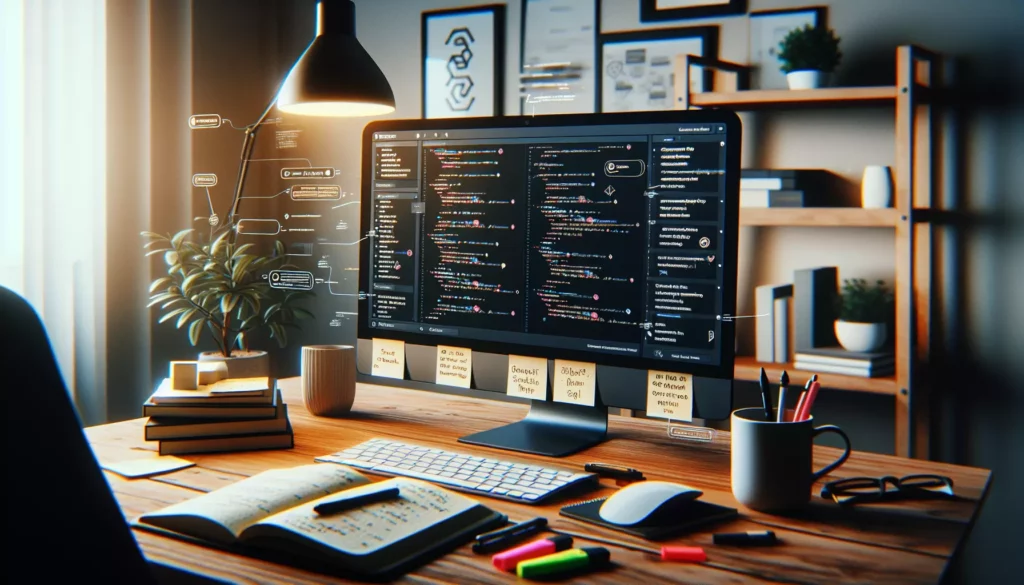
Are you gearing up for a technical interview at Figma? As one of the leading design tools in the industry, Figma attracts top talent, and their interview process is known to be rigorous. This comprehensive guide will walk you through everything you need to know to ace your Figma technical interview, from understanding the company culture to mastering the specific skills they’re looking for.
Table of Contents
- Understanding Figma
- The Figma Interview Process
- Key Technical Skills to Master
- Common Coding Challenges
- System Design Questions
- Behavioral Questions
- Tips and Tricks for Success
- Additional Resources
- Conclusion
Understanding Figma
Before diving into the interview prep, it’s crucial to understand what Figma is and what they value. Figma is a cloud-based design tool that enables collaborative interface design. It’s used by designers, developers, and other stakeholders to create, prototype, and collaborate on user interfaces and user experiences.
Key aspects of Figma’s culture and technology include:
- Collaboration: Figma emphasizes real-time collaboration, allowing multiple users to work on the same design simultaneously.
- Web-based: Unlike many traditional design tools, Figma runs in the browser, making it accessible across different platforms.
- Performance: Figma prides itself on being fast and responsive, even with complex designs.
- Plugins and extensibility: Figma has a robust plugin ecosystem, allowing developers to extend its functionality.
Understanding these core aspects will help you align your responses and demonstrate your fit with Figma’s mission and values during the interview.
The Figma Interview Process
The Figma interview process typically consists of several stages:
- Initial Screening: This is usually a phone call with a recruiter to discuss your background and interest in the role.
- Technical Phone Screen: A 45-60 minute call with an engineer, involving coding questions and technical discussions.
- Take-home Assignment: Some roles may require a take-home coding assignment to assess your skills in a less pressured environment.
- On-site Interviews: A series of interviews (now often conducted virtually) including:
- Coding interviews
- System design interview
- Behavioral interviews
- Final Decision: The hiring team meets to discuss your performance and make a decision.
Each stage is designed to evaluate different aspects of your skills and fit for the role. Let’s dive deeper into what you need to prepare for each part of the process.
Key Technical Skills to Master
To excel in a Figma technical interview, you should be well-versed in the following areas:
1. JavaScript Proficiency
As Figma is built primarily with JavaScript (and TypeScript), a strong command of JS is crucial. Focus on:
- ES6+ features
- Asynchronous programming (Promises, async/await)
- Functional programming concepts
- Object-oriented programming in JavaScript
Here’s a simple example of modern JavaScript you might be expected to understand and write:
const fetchUserData = async (userId) => {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const userData = await response.json();
return userData;
} catch (error) {
console.error('Error fetching user data:', error);
return null;
}
};
// Usage
fetchUserData(123).then(data => {
if (data) {
console.log('User data:', data);
} else {
console.log('Failed to fetch user data');
}
});
2. Web Technologies
Given Figma’s web-based nature, you should be familiar with:
- HTML5 and CSS3
- DOM manipulation
- Web APIs (e.g., Canvas, WebGL)
- Browser rendering and performance optimization
3. React and State Management
Figma uses React for its user interface. Knowledge of React and state management solutions like Redux or MobX can be beneficial. Be prepared to discuss:
- React component lifecycle
- Hooks (useState, useEffect, useContext, etc.)
- State management patterns
- Performance optimization in React applications
4. TypeScript
While not always required, familiarity with TypeScript can be a significant advantage. Understand:
- Type annotations and interfaces
- Generics
- Union and intersection types
- Type inference
5. Data Structures and Algorithms
As with many tech interviews, a solid grasp of data structures and algorithms is essential. Focus on:
- Arrays and strings
- Hash tables
- Trees and graphs
- Dynamic programming
- Time and space complexity analysis
6. System Design and Architecture
For more senior roles, you’ll need to demonstrate your ability to design scalable systems. Key areas include:
- Distributed systems
- Caching strategies
- Database design
- API design
- Real-time collaboration architectures
Common Coding Challenges
During your Figma interview, you may encounter coding challenges that test your problem-solving skills and coding ability. Here are some types of problems you might face:
1. String Manipulation
Given Figma’s text handling capabilities, string manipulation questions are common. For example:
// Problem: Implement a function that reverses words in a sentence
function reverseWords(sentence) {
return sentence.split(' ').map(word =>
word.split('').reverse().join('')
).join(' ');
}
console.log(reverseWords("Hello Figma")); // Output: "olleH amgiF"
2. Array Transformations
Figma deals with layers and objects, which are often represented as arrays. You might encounter questions like:
// Problem: Flatten a nested array
function flattenArray(arr) {
return arr.reduce((flat, toFlatten) =>
flat.concat(Array.isArray(toFlatten) ? flattenArray(toFlatten) : toFlatten),
[]);
}
console.log(flattenArray([1, [2, [3, 4], 5]])); // Output: [1, 2, 3, 4, 5]
3. Tree Traversal
Understanding tree structures is crucial for working with Figma’s layer hierarchy. You might be asked to implement tree traversal algorithms:
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
function inorderTraversal(root) {
const result = [];
function traverse(node) {
if (node) {
traverse(node.left);
result.push(node.value);
traverse(node.right);
}
}
traverse(root);
return result;
}
// Usage
const root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
console.log(inorderTraversal(root)); // Output: [4, 2, 5, 1, 3]
4. Implementing Design Patterns
You might be asked to implement or explain common design patterns. For instance, the Observer pattern is relevant to Figma’s real-time collaboration features:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
removeObserver(observer) {
const index = this.observers.indexOf(observer);
if (index > -1) {
this.observers.splice(index, 1);
}
}
notifyObservers(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log('Received update:', data);
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers('New design change');
System Design Questions
For senior roles, you’ll likely face system design questions. These assess your ability to architect complex systems. Some potential topics include:
1. Designing a Real-time Collaboration System
You might be asked to design a system that allows multiple users to edit a document simultaneously, similar to Figma’s core functionality. Key points to consider:
- Conflict resolution strategies (Operational Transformation vs. Conflict-free Replicated Data Types)
- WebSocket implementation for real-time updates
- Scalability concerns for handling many concurrent users
- Data persistence and synchronization
2. Implementing a Plugin System
Figma’s extensibility is a key feature. You might need to design a plugin architecture that allows third-party developers to extend Figma’s functionality. Consider:
- Sandboxing for security
- API design for plugin developers
- Performance implications of running third-party code
- Versioning and compatibility
3. Scaling Vector Graphics Rendering
Given Figma’s focus on vector graphics, you might be asked how you’d design a system to efficiently render complex vector illustrations. Think about:
- Efficient data structures for storing vector paths
- Rendering optimizations (e.g., canvas vs. SVG)
- Caching strategies for improved performance
- Handling zoom and pan operations efficiently
Behavioral Questions
Figma, like many tech companies, values not just technical skills but also how well you work in a team and align with their culture. Be prepared for behavioral questions such as:
- “Tell me about a time when you had to work on a challenging project with tight deadlines.”
- “How do you approach learning new technologies or skills?”
- “Describe a situation where you had to collaborate with a difficult team member.”
- “How do you ensure the quality of your code in a fast-paced environment?”
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
Tips and Tricks for Success
To maximize your chances of success in a Figma technical interview, keep these tips in mind:
- Practice coding without an IDE: Figma interviews often involve coding on a whiteboard or in a simple text editor. Practice coding without the help of autocomplete or syntax highlighting.
- Communicate your thought process: Explain your approach as you solve problems. Interviewers are interested in how you think, not just the final solution.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification on problems. This shows engagement and helps you avoid misunderstandings.
- Know Figma’s product: Use Figma before your interview. Understanding the product will help you relate your answers to real-world scenarios.
- Be prepared to optimize: After solving a problem, be ready to discuss how you could optimize it for better performance or scalability.
- Stay updated: Figma is at the forefront of design technology. Stay informed about the latest trends in web technologies and design tools.
- Practice collaborative problem-solving: Given Figma’s focus on collaboration, be prepared to work through problems with your interviewer, not just present solutions.
Additional Resources
To further prepare for your Figma technical interview, consider exploring these resources:
- Figma Developer Documentation: Familiarize yourself with Figma’s API and plugin development to understand their technical ecosystem.
- LeetCode: Practice coding problems, especially those related to JavaScript and web development.
- System Design Primer: A GitHub repository with resources for learning about large-scale system design.
- React Documentation: Brush up on your React skills with the official React documentation.
- TypeScript Handbook: If you’re not familiar with TypeScript, the official handbook is a great place to start.
- Web Performance Optimization: Learn about optimizing web applications with resources like Google’s Web Fundamentals.
Conclusion
Preparing for a Figma technical interview requires a blend of strong JavaScript skills, understanding of web technologies, and knowledge of system design principles. By focusing on these areas and practicing regularly, you’ll be well-equipped to showcase your abilities and land that dream role at Figma.
Remember, the key to success is not just about having the right answers, but also demonstrating your problem-solving process, your ability to collaborate, and your passion for creating great user experiences. With thorough preparation and the right mindset, you’ll be ready to tackle whatever challenges the Figma interview process throws your way.
Good luck with your interview preparation, and may your journey with Figma be a successful one!