Exploring Voice and Speech Recognition Technologies: Building Voice-Activated Applications
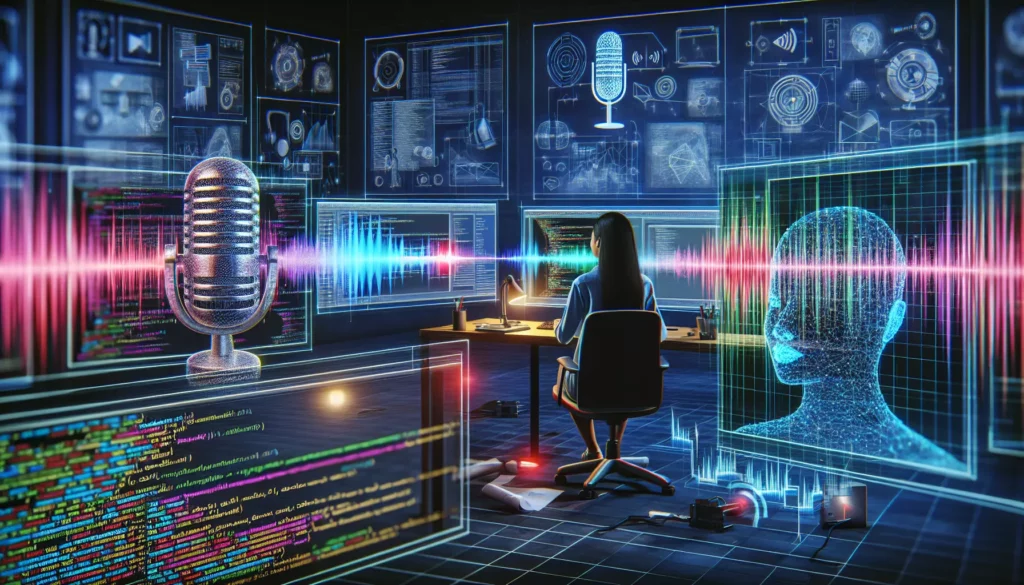
In the ever-evolving landscape of technology, voice and speech recognition have emerged as powerful tools that are revolutionizing the way we interact with our devices. From virtual assistants like Siri and Alexa to voice-controlled smart home devices, these technologies are becoming increasingly prevalent in our daily lives. As a programmer, understanding and implementing voice and speech recognition can open up exciting possibilities for creating innovative applications. In this comprehensive guide, we’ll explore the world of voice and speech recognition technologies and learn how to create applications that can process and respond to voice commands.
Understanding Voice and Speech Recognition
Before diving into the implementation details, it’s essential to understand the basics of voice and speech recognition technologies.
What is Voice Recognition?
Voice recognition, also known as speaker recognition, is the technology that identifies and authenticates individual speakers based on their unique vocal characteristics. This technology is primarily used for security purposes, such as voice-based authentication systems.
What is Speech Recognition?
Speech recognition, on the other hand, focuses on converting spoken words into text. This technology is used in various applications, including voice assistants, transcription services, and voice-controlled devices.
How Do These Technologies Work?
Both voice and speech recognition technologies rely on complex algorithms and machine learning models to process and interpret audio input. Here’s a simplified overview of the process:
- Audio Input: The system captures audio input through a microphone.
- Preprocessing: The audio signal is cleaned and normalized to remove background noise and enhance the relevant features.
- Feature Extraction: The system extracts key features from the audio, such as frequency, pitch, and rhythm.
- Pattern Matching: The extracted features are compared against pre-trained models or datasets.
- Decision Making: Based on the pattern matching results, the system makes a decision or generates an output (e.g., transcribed text or speaker identification).
Popular Libraries and APIs for Voice and Speech Recognition
To implement voice and speech recognition in your applications, you can leverage various libraries and APIs. Here are some popular options:
1. Google Speech-to-Text API
Google’s Speech-to-Text API is a powerful cloud-based solution that offers high accuracy and support for multiple languages. It’s easy to integrate into applications and provides real-time transcription capabilities.
2. Mozilla DeepSpeech
DeepSpeech is an open-source speech-to-text engine developed by Mozilla. It uses deep learning techniques and can be run locally, making it suitable for applications that require offline functionality.
3. CMU Sphinx
CMU Sphinx is a group of speech recognition systems developed by Carnegie Mellon University. It offers both offline and online recognition capabilities and supports multiple languages.
4. Web Speech API
For web-based applications, the Web Speech API provides a standardized way to implement speech recognition and synthesis in web browsers. It’s supported by most modern browsers and is easy to use with JavaScript.
Creating a Voice-Activated Application: Step-by-Step Guide
Now that we have an understanding of the technologies and available tools, let’s walk through the process of creating a simple voice-activated application. In this example, we’ll build a web-based voice calculator using JavaScript and the Web Speech API.
Step 1: Set Up the HTML Structure
First, let’s create the basic HTML structure for our voice calculator:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Voice Calculator</title>
<style>
/* Add your CSS styles here */
</style>
</head>
<body>
<div id="calculator">
<input type="text" id="result" readonly>
<button id="startVoice">Start Voice Input</button>
<p id="status"></p>
</div>
<script src="voice-calculator.js"></script>
</body>
</html>
Step 2: Implement the Voice Recognition Logic
Next, let’s create the JavaScript file (voice-calculator.js) to implement the voice recognition and calculation logic:
// Check if the browser supports speech recognition
if ("webkitSpeechRecognition" in window) {
const recognition = new webkitSpeechRecognition();
const startButton = document.getElementById("startVoice");
const result = document.getElementById("result");
const status = document.getElementById("status");
recognition.continuous = false;
recognition.lang = "en-US";
startButton.addEventListener("click", () => {
recognition.start();
status.textContent = "Listening...";
});
recognition.onresult = (event) => {
const transcript = event.results[0][0].transcript;
status.textContent = `You said: ${transcript}`;
try {
// Evaluate the mathematical expression
const calculatedResult = eval(transcript);
result.value = calculatedResult;
} catch (error) {
result.value = "Error: Invalid expression";
}
};
recognition.onerror = (event) => {
status.textContent = `Error: ${event.error}`;
};
recognition.onend = () => {
status.textContent = "Voice recognition ended";
};
} else {
alert("Your browser doesn't support speech recognition. Please try a different browser.");
}
This code sets up the speech recognition functionality using the Web Speech API. When the user clicks the “Start Voice Input” button, the application listens for voice input, transcribes it, and attempts to evaluate it as a mathematical expression.
Step 3: Enhance the User Experience
To make the application more user-friendly, you can add features like:
- Visual feedback during voice input
- Error handling for unsupported browsers or microphone access issues
- A list of supported voice commands or example expressions
Step 4: Test and Refine
Test your application thoroughly with various voice inputs and mathematical expressions. Refine the recognition accuracy by adding custom logic to handle common speech patterns or mathematical terms.
Advanced Topics in Voice and Speech Recognition
As you become more comfortable with basic voice recognition, you can explore more advanced topics to enhance your applications:
1. Natural Language Processing (NLP)
Integrating NLP techniques can help your application better understand and interpret user intent. Libraries like Natural.js or cloud-based services like Google Cloud Natural Language API can be valuable for this purpose.
2. Custom Wake Words
Implement custom wake words to activate your voice application, similar to “Hey Siri” or “Okay Google”. This can be achieved using techniques like keyword spotting or always-on audio processing.
3. Multi-language Support
Expand your application’s reach by supporting multiple languages. Many speech recognition APIs offer multi-language capabilities, allowing you to cater to a global audience.
4. Voice Authentication
Implement voice-based user authentication for added security in your applications. This involves creating and comparing voice prints of users.
5. Emotion Recognition
Analyze the emotional content of speech to provide more personalized responses. This can be achieved using machine learning models trained on emotional speech datasets.
Challenges and Considerations
While voice and speech recognition technologies offer exciting possibilities, there are several challenges and considerations to keep in mind:
1. Privacy and Security
Handling voice data requires careful consideration of privacy and security implications. Ensure that you comply with relevant data protection regulations and implement proper security measures to protect user information.
2. Accuracy and Reliability
Speech recognition accuracy can vary depending on factors like background noise, accents, and speech patterns. Consider implementing fallback mechanisms or alternative input methods to handle cases where voice recognition fails.
3. Performance Optimization
For applications that require real-time processing, optimizing performance is crucial. Consider using lightweight models or edge computing solutions for faster response times.
4. Accessibility
While voice interfaces can improve accessibility for some users, they may present challenges for others. Ensure that your application provides alternative interaction methods to accommodate all users.
Future Trends in Voice and Speech Recognition
As technology continues to advance, we can expect several exciting developments in the field of voice and speech recognition:
1. Improved Accuracy and Context Understanding
Advancements in machine learning and natural language processing will lead to more accurate and context-aware voice recognition systems.
2. Edge Computing for Voice Processing
As edge devices become more powerful, we’ll see more voice processing happening locally, reducing latency and improving privacy.
3. Integration with Other Technologies
Voice recognition will increasingly be integrated with other technologies like augmented reality, virtual reality, and IoT devices, creating more immersive and intuitive user experiences.
4. Personalized Voice Experiences
AI-driven voice assistants will become more personalized, adapting to individual users’ speech patterns, preferences, and behaviors.
Conclusion
Voice and speech recognition technologies have opened up a new frontier in human-computer interaction. By creating applications that can process and respond to voice commands, developers can build more intuitive, accessible, and engaging user experiences. As we’ve explored in this guide, implementing voice recognition in your applications is becoming increasingly accessible thanks to powerful APIs and libraries.
Whether you’re building a simple voice-controlled calculator or a complex voice-driven assistant, the key to success lies in understanding the underlying technologies, choosing the right tools, and continuously refining your implementation based on user feedback and technological advancements.
As you embark on your journey into voice and speech recognition development, remember that practice and experimentation are crucial. Start with simple projects, gradually increase complexity, and don’t hesitate to explore the cutting-edge developments in this rapidly evolving field. With dedication and creativity, you can harness the power of voice technology to create truly innovative and impactful applications.
So, what are you waiting for? It’s time to give your applications a voice and let them listen to the world around them!