Exploring Union-Find Data Structures: A Comprehensive Guide
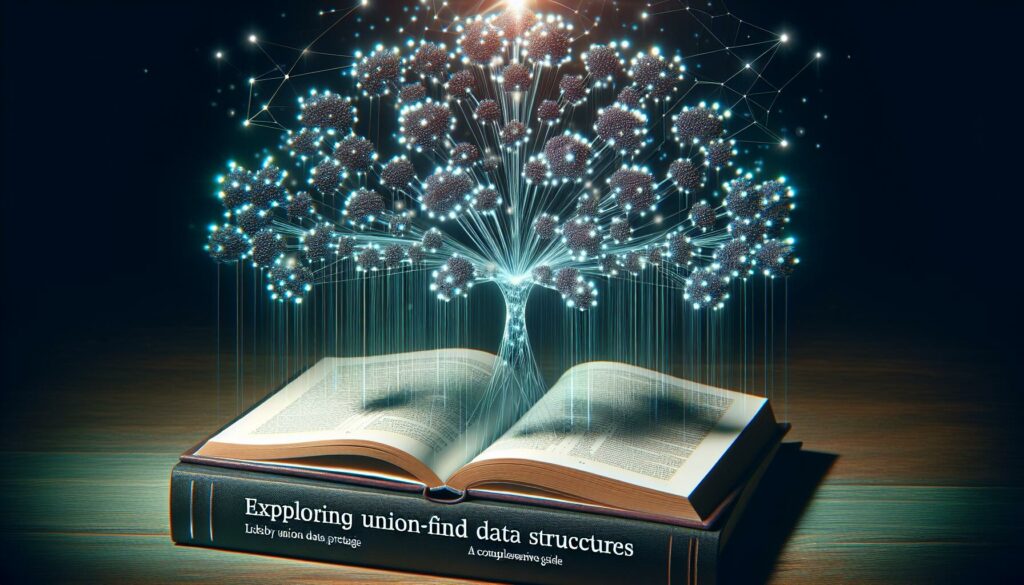
In the world of computer science and algorithm design, efficient data structures play a crucial role in solving complex problems. One such powerful data structure is the Union-Find, also known as the Disjoint Set data structure. This article will dive deep into the Union-Find data structure, exploring its concepts, implementation, and various applications in solving real-world problems.
What is a Union-Find Data Structure?
The Union-Find data structure is a tree-based data structure that efficiently keeps track of a partition of a set of elements into disjoint subsets. It provides two primary operations:
- Find: Determine which subset a particular element belongs to.
- Union: Join two subsets into a single subset.
These operations are essential for solving problems related to connectivity, such as finding connected components in a graph or determining whether two elements are in the same set.
Basic Implementation of Union-Find
Let’s start with a basic implementation of the Union-Find data structure in Python:
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
root_x = self.find(x)
root_y = self.find(y)
if root_x != root_y:
if self.rank[root_x] < self.rank[root_y]:
self.parent[root_x] = root_y
elif self.rank[root_x] > self.rank[root_y]:
self.parent[root_y] = root_x
else:
self.parent[root_y] = root_x
self.rank[root_x] += 1
This implementation uses two key optimizations:
- Path Compression: In the
find
method, we recursively set each node’s parent to the root of the tree, flattening the structure. - Union by Rank: In the
union
method, we attach the tree with a smaller rank to the root of the tree with a larger rank, keeping the tree balanced.
Time Complexity Analysis
The time complexity of Union-Find operations with path compression and union by rank is nearly constant time, specifically O(α(n)), where α(n) is the inverse Ackermann function. For all practical values of n, α(n) is at most 4, making these operations essentially constant time.
- Find operation: O(α(n))
- Union operation: O(α(n))
Applications of Union-Find
Union-Find data structures have numerous applications in various domains of computer science and real-world problem-solving. Let’s explore some of the most common use cases:
1. Connected Components in Graphs
One of the primary applications of Union-Find is finding connected components in an undirected graph. This is useful in social network analysis, image processing, and network connectivity problems.
Example: Finding the number of connected components in a graph
def count_connected_components(n, edges):
uf = UnionFind(n)
for u, v in edges:
uf.union(u, v)
components = set()
for i in range(n):
components.add(uf.find(i))
return len(components)
# Example usage
n = 5
edges = [(0, 1), (1, 2), (3, 4)]
print(count_connected_components(n, edges)) # Output: 2
2. Kruskal’s Algorithm for Minimum Spanning Tree
Union-Find is a key component in Kruskal’s algorithm for finding the minimum spanning tree of a weighted, undirected graph. This has applications in network design, clustering, and image segmentation.
def kruskal_mst(n, edges):
uf = UnionFind(n)
edges.sort(key=lambda x: x[2]) # Sort edges by weight
mst = []
for u, v, weight in edges:
if uf.find(u) != uf.find(v):
uf.union(u, v)
mst.append((u, v, weight))
return mst
# Example usage
n = 4
edges = [(0, 1, 10), (0, 2, 6), (0, 3, 5), (1, 3, 15), (2, 3, 4)]
print(kruskal_mst(n, edges))
# Output: [(2, 3, 4), (0, 3, 5), (0, 1, 10)]
3. Cycle Detection in Graphs
Union-Find can be used to detect cycles in undirected graphs efficiently. This is useful in various graph algorithms and in detecting conflicts in systems.
def has_cycle(n, edges):
uf = UnionFind(n)
for u, v in edges:
if uf.find(u) == uf.find(v):
return True
uf.union(u, v)
return False
# Example usage
n = 3
edges = [(0, 1), (1, 2), (2, 0)]
print(has_cycle(n, edges)) # Output: True
4. Percolation Theory
Union-Find is used in studying percolation systems, which model the flow of fluids through porous materials. This has applications in physics, materials science, and complex systems analysis.
5. Image Segmentation
In image processing, Union-Find can be used for efficient image segmentation algorithms, grouping similar pixels together to identify objects or regions in an image.
6. Dynamic Connectivity
Union-Find is ideal for solving dynamic connectivity problems, where the connectivity between elements changes over time. This is useful in network management and distributed systems.
Advanced Techniques and Optimizations
While the basic Union-Find implementation with path compression and union by rank is highly efficient, there are several advanced techniques and optimizations that can further improve performance in specific scenarios:
1. Weighted Quick Union
This optimization keeps track of the size of each tree and always attaches the smaller tree to the root of the larger tree. This ensures that the tree depth remains logarithmic in the worst case.
class WeightedQuickUnionUF:
def __init__(self, n):
self.parent = list(range(n))
self.size = [1] * n
def find(self, x):
while x != self.parent[x]:
x = self.parent[x]
return x
def union(self, x, y):
root_x = self.find(x)
root_y = self.find(y)
if root_x != root_y:
if self.size[root_x] < self.size[root_y]:
self.parent[root_x] = root_y
self.size[root_y] += self.size[root_x]
else:
self.parent[root_y] = root_x
self.size[root_x] += self.size[root_y]
2. Path Splitting
Path splitting is an alternative to path compression that can be more efficient in some cases. Instead of directly linking all nodes to the root, it makes every other node in the path point to its grandparent.
def find(self, x):
while x != self.parent[x]:
self.parent[x] = self.parent[self.parent[x]]
x = self.parent[x]
return x
3. Path Halving
Path halving is similar to path splitting but even simpler. It makes every node point to its grandparent.
def find(self, x):
while x != self.parent[x]:
self.parent[x] = self.parent[self.parent[x]]
x = self.parent[self.parent[x]]
return x
4. Iterative Path Compression
For languages that don’t handle deep recursion well, an iterative approach to path compression can be used:
def find(self, x):
root = x
while root != self.parent[root]:
root = self.parent[root]
while x != root:
next_x = self.parent[x]
self.parent[x] = root
x = next_x
return root
5. Optimized Union by Rank
Instead of using a separate rank array, we can store the rank information in the parent array itself, using negative values to represent ranks:
class OptimizedUnionFind:
def __init__(self, n):
self.parent = [-1] * n
def find(self, x):
if self.parent[x] < 0:
return x
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
root_x = self.find(x)
root_y = self.find(y)
if root_x != root_y:
if self.parent[root_x] > self.parent[root_y]:
root_x, root_y = root_y, root_x
self.parent[root_x] += self.parent[root_y]
self.parent[root_y] = root_x
Practical Examples and Problem Solving
Let’s explore some practical examples and problem-solving scenarios where Union-Find data structures can be effectively applied:
1. Friend Circles
Problem: Given a group of people and their friendships, determine the number of friend circles.
def find_friend_circles(friendships):
n = len(friendships)
uf = UnionFind(n)
for i in range(n):
for j in range(i+1, n):
if friendships[i][j] == 1:
uf.union(i, j)
circles = set()
for i in range(n):
circles.add(uf.find(i))
return len(circles)
# Example usage
friendships = [
[1, 1, 0],
[1, 1, 0],
[0, 0, 1]
]
print(find_friend_circles(friendships)) # Output: 2
2. Redundant Connection
Problem: In a graph that started as a tree, one extra edge was added. Find that edge.
def find_redundant_connection(edges):
n = len(edges)
uf = UnionFind(n + 1)
for u, v in edges:
if uf.find(u) == uf.find(v):
return [u, v]
uf.union(u, v)
return []
# Example usage
edges = [[1,2], [1,3], [2,3]]
print(find_redundant_connection(edges)) # Output: [2, 3]
3. Number of Islands II
Problem: Given a 2D matrix initially filled with water, and a list of land positions, find the number of islands after each land position is added.
def num_islands2(m, n, positions):
uf = UnionFind(m * n)
grid = [[0] * n for _ in range(m)]
count = 0
result = []
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
for r, c in positions:
if grid[r][c] == 1:
result.append(count)
continue
grid[r][c] = 1
count += 1
for dr, dc in directions:
nr, nc = r + dr, c + dc
if 0 <= nr < m and 0 <= nc < n and grid[nr][nc] == 1:
root1 = uf.find(r * n + c)
root2 = uf.find(nr * n + nc)
if root1 != root2:
uf.union(root1, root2)
count -= 1
result.append(count)
return result
# Example usage
m, n = 3, 3
positions = [[0,0], [0,1], [1,2], [2,1]]
print(num_islands2(m, n, positions)) # Output: [1, 1, 2, 3]
Performance Considerations and Trade-offs
When working with Union-Find data structures, it’s important to consider the following performance aspects and trade-offs:
1. Memory Usage
The basic Union-Find implementation uses O(n) memory, where n is the number of elements. This can be a concern for very large datasets. In some cases, you might need to use more memory-efficient representations or external storage for large-scale problems.
2. Initialization Cost
The initialization of a Union-Find data structure takes O(n) time. For applications where you need to create many small Union-Find instances, this initialization cost can become significant.
3. Balancing Find and Union Operations
Different optimizations can favor either find or union operations. Path compression optimizes finds at the cost of slightly slower unions, while union by rank ensures balanced trees but might result in slightly slower finds. Choose the optimization that best suits your specific use case.
4. Iterative vs. Recursive Implementations
Recursive implementations of path compression are elegant but can lead to stack overflow for very deep trees. Iterative implementations are generally safer but might be slightly slower for shallow trees.
5. Dynamic vs. Static Sets
The basic Union-Find structure works well for a fixed number of elements. If you need to add or remove elements dynamically, you might need to use more complex data structures or periodically rebuild the Union-Find structure.
Conclusion
Union-Find data structures are powerful tools for solving a wide range of problems related to set partitioning and connectivity. Their near-constant time complexity for both find and union operations makes them highly efficient for large-scale problems.
By understanding the core concepts, implementations, and optimizations of Union-Find, you can effectively apply this data structure to solve complex problems in areas such as graph theory, network analysis, and image processing. As with any algorithm or data structure, it’s crucial to consider the specific requirements of your problem and choose the most appropriate implementation and optimizations.
As you continue your journey in algorithm design and problem-solving, keep the Union-Find data structure in your toolkit. Its simplicity, efficiency, and versatility make it an invaluable asset for tackling a wide array of computational challenges.