Exploring the Software Behind Self-Driving Cars: A Deep Dive into Autonomous Vehicle Programming
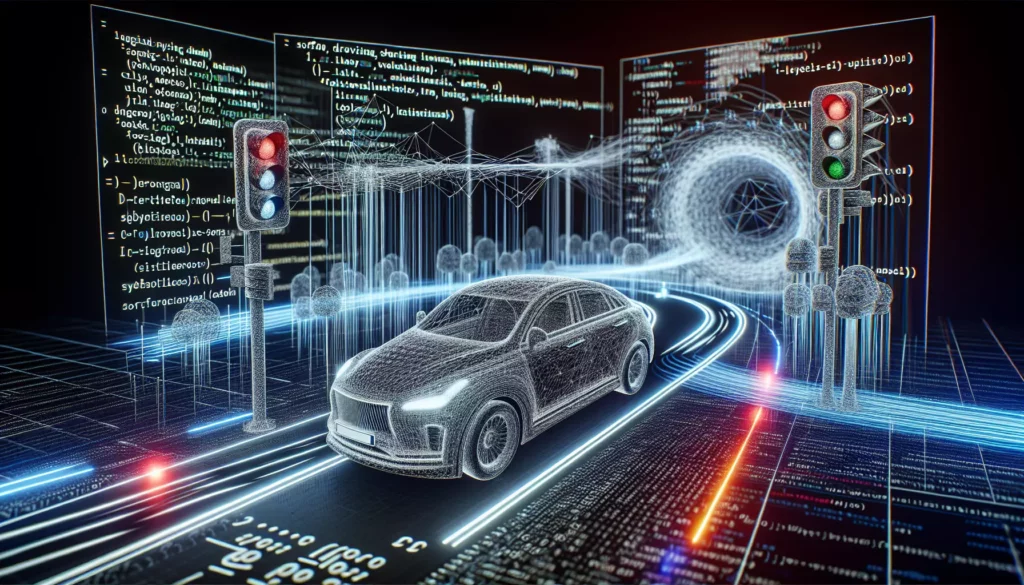
As we stand on the cusp of a transportation revolution, autonomous vehicles are no longer a distant dream but a rapidly approaching reality. The software powering these self-driving marvels is a complex tapestry of algorithms, machine learning models, and real-time decision-making systems. In this comprehensive guide, we’ll explore the intricate world of autonomous vehicle programming, unraveling the layers of code that enable cars to navigate our roads without human intervention.
The Foundation of Autonomous Vehicle Software
At its core, the software behind self-driving cars is designed to replicate and enhance human driving capabilities. This involves several key components:
- Perception
- Localization and Mapping
- Path Planning
- Control Systems
- Decision Making
Each of these components relies on sophisticated algorithms and data processing techniques. Let’s delve into each one to understand how they contribute to the overall functionality of autonomous vehicles.
1. Perception: The Eyes and Ears of Self-Driving Cars
Perception systems are responsible for gathering and interpreting data from the vehicle’s environment. This is achieved through a combination of sensors, including:
- Cameras
- LiDAR (Light Detection and Ranging)
- Radar
- Ultrasonic sensors
The software processes the raw data from these sensors to create a comprehensive understanding of the vehicle’s surroundings. This involves:
Object Detection and Classification
Using computer vision algorithms, the system identifies and categorizes objects in the environment. This includes other vehicles, pedestrians, traffic signs, and road markings.
A typical object detection algorithm might use a convolutional neural network (CNN) architecture. Here’s a simplified example of how you might define a CNN in Python using TensorFlow:
import tensorflow as tf
def create_cnn_model():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(224, 224, 3)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
return model
cnn_model = create_cnn_model()
cnn_model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Sensor Fusion
To create a robust and accurate perception of the environment, data from multiple sensors must be combined. This process, known as sensor fusion, helps overcome the limitations of individual sensors and provides a more complete picture of the surroundings.
Here’s a conceptual example of how sensor fusion might be implemented:
def sensor_fusion(camera_data, lidar_data, radar_data):
# Combine data from different sensors
fused_data = {
'objects': [],
'distances': [],
'velocities': []
}
# Process camera data for object recognition
objects = process_camera_data(camera_data)
fused_data['objects'] = objects
# Use LiDAR data for precise distance measurements
distances = process_lidar_data(lidar_data)
fused_data['distances'] = distances
# Use radar data for velocity measurements
velocities = process_radar_data(radar_data)
fused_data['velocities'] = velocities
return fused_data
def process_camera_data(camera_data):
# Image processing and object recognition
pass
def process_lidar_data(lidar_data):
# Point cloud processing for distance measurements
pass
def process_radar_data(radar_data):
# Doppler effect analysis for velocity measurements
pass
2. Localization and Mapping: Knowing Where You Are
For an autonomous vehicle to navigate effectively, it needs to know its precise location and have an accurate map of its environment. This is achieved through a combination of technologies:
Global Positioning System (GPS)
GPS provides a rough estimate of the vehicle’s location, but it’s not accurate enough for autonomous driving on its own.
Inertial Measurement Units (IMU)
IMUs use accelerometers and gyroscopes to measure the vehicle’s movement and orientation.
Simultaneous Localization and Mapping (SLAM)
SLAM algorithms allow the vehicle to build a map of its environment while simultaneously determining its location within that map. This is crucial for operating in areas where high-precision maps may not be available.
Here’s a simplified example of how a basic SLAM algorithm might be structured:
import numpy as np
class SLAM:
def __init__(self):
self.map = np.zeros((1000, 1000)) # Initialize empty map
self.position = np.array([500, 500]) # Start at center
self.orientation = 0 # Initial orientation (in radians)
def update(self, sensor_data, control_input):
# Predict new position based on control input
self.predict_position(control_input)
# Update map based on sensor data
self.update_map(sensor_data)
# Correct position estimate based on map matching
self.correct_position()
def predict_position(self, control_input):
# Update position based on control input (e.g., wheel encoders)
delta_position = self.calculate_movement(control_input)
self.position += delta_position
def update_map(self, sensor_data):
# Update map based on new sensor readings
for reading in sensor_data:
x, y = self.calculate_map_position(reading)
self.map[x, y] = 1 # Mark as occupied
def correct_position(self):
# Correct position estimate by matching sensor data to map
# This would involve complex algorithms like particle filters
pass
def calculate_movement(self, control_input):
# Convert control input to position change
pass
def calculate_map_position(self, sensor_reading):
# Convert sensor reading to map coordinates
pass
3. Path Planning: Charting the Course
Once the vehicle knows where it is and what’s around it, it needs to plan a safe and efficient route to its destination. Path planning algorithms consider various factors:
- Traffic rules and regulations
- Road conditions
- Other vehicles and obstacles
- Efficiency (shortest route, least fuel consumption, etc.)
Path planning typically involves multiple levels of planning:
Route Planning
This high-level planning determines the overall route from the current location to the destination, often using graph-based algorithms like A* or Dijkstra’s algorithm.
Behavior Planning
This intermediate level decides on maneuvers like lane changes, turns, and merges based on the current traffic situation and route plan.
Trajectory Planning
This low-level planning generates the precise path the vehicle should follow, considering vehicle dynamics and ensuring a smooth, safe ride.
Here’s a simplified example of how a basic path planning algorithm might be implemented:
import heapq
def astar(graph, start, goal):
def heuristic(node):
# Estimate distance to goal (e.g., Euclidean distance)
pass
def get_neighbors(node):
# Return list of neighboring nodes
pass
frontier = [(0, start)]
came_from = {}
cost_so_far = {start: 0}
while frontier:
current_cost, current = heapq.heappop(frontier)
if current == goal:
break
for next in get_neighbors(current):
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
# Reconstruct path
path = []
current = goal
while current != start:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
4. Control Systems: Executing the Plan
Once a path is planned, the control systems take over to execute it. This involves translating high-level commands into specific instructions for the vehicle’s actuators (steering, acceleration, braking).
Control systems in autonomous vehicles often use advanced techniques like Model Predictive Control (MPC) to anticipate future states and optimize vehicle behavior.
Here’s a simplified example of a basic control system:
class VehicleController:
def __init__(self):
self.current_speed = 0
self.current_steering_angle = 0
def update(self, target_speed, target_steering_angle):
# Adjust speed
speed_error = target_speed - self.current_speed
acceleration = self.calculate_acceleration(speed_error)
self.current_speed += acceleration
# Adjust steering
steering_error = target_steering_angle - self.current_steering_angle
steering_adjustment = self.calculate_steering_adjustment(steering_error)
self.current_steering_angle += steering_adjustment
return acceleration, self.current_steering_angle
def calculate_acceleration(self, speed_error):
# PID controller for speed
kp = 0.1 # Proportional gain
ki = 0.01 # Integral gain
kd = 0.05 # Derivative gain
# In a real implementation, we would keep track of past errors
# for integral and derivative terms
return kp * speed_error
def calculate_steering_adjustment(self, steering_error):
# Simple proportional control for steering
kp = 0.1
return kp * steering_error
5. Decision Making: The Brain of the Operation
At the heart of autonomous vehicle software is the decision-making system. This component integrates information from all other systems to make real-time decisions about vehicle behavior.
Decision-making in autonomous vehicles often employs techniques from artificial intelligence and machine learning, including:
- Rule-based systems
- Probabilistic methods (e.g., Bayesian networks)
- Reinforcement learning
- Deep learning models
Here’s a conceptual example of a decision-making system using a simple rule-based approach:
class DecisionMaker:
def __init__(self):
self.safety_distance = 10 # meters
def make_decision(self, perception_data, vehicle_state):
if self.emergency_detected(perception_data):
return self.emergency_stop()
if self.obstacle_ahead(perception_data):
return self.avoid_obstacle(perception_data)
if self.lane_change_needed(perception_data, vehicle_state):
return self.initiate_lane_change()
return self.continue_current_path()
def emergency_detected(self, perception_data):
# Check for emergency situations
pass
def obstacle_ahead(self, perception_data):
# Check if there's an obstacle within safety distance
pass
def lane_change_needed(self, perception_data, vehicle_state):
# Determine if a lane change is necessary
pass
def emergency_stop(self):
return {'action': 'stop', 'deceleration': -9.8} # Max braking
def avoid_obstacle(self, perception_data):
# Calculate evasive maneuver
pass
def initiate_lane_change(self):
# Plan and execute lane change
pass
def continue_current_path(self):
return {'action': 'maintain', 'speed': 0, 'steering': 0}
Challenges in Autonomous Vehicle Programming
Developing software for autonomous vehicles comes with numerous challenges:
1. Safety and Reliability
Ensuring the safety of passengers, pedestrians, and other road users is paramount. The software must be extremely reliable and able to handle unexpected situations.
2. Ethical Decision Making
Autonomous vehicles may encounter situations where they need to make ethical decisions, such as choosing between two harmful outcomes. Programming these ethical considerations is a complex challenge.
3. Handling Edge Cases
Real-world driving involves countless edge cases and unusual situations. The software must be able to handle these rare but critical scenarios.
4. Real-time Performance
The software must process vast amounts of data and make decisions in real-time, often within milliseconds.
5. Adaptability
Autonomous vehicles must adapt to different driving conditions, weather, and traffic patterns.
6. Cybersecurity
As connected systems, autonomous vehicles must be protected against potential cyber attacks.
The Future of Autonomous Vehicle Programming
As technology advances, we can expect to see several trends in autonomous vehicle programming:
1. Advanced AI and Machine Learning
More sophisticated AI models will be developed to handle complex decision-making and improve adaptability to new situations.
2. Improved Sensor Technology
As sensors become more advanced and cost-effective, software will need to evolve to make better use of the increased data resolution and accuracy.
3. Vehicle-to-Everything (V2X) Communication
Autonomous vehicles will increasingly communicate with other vehicles, infrastructure, and pedestrians, requiring new software frameworks to handle this interconnected ecosystem.
4. Standardization and Regulation
As the industry matures, we can expect to see more standardization in software architectures and stricter regulations governing autonomous vehicle software.
5. Human-AI Collaboration
Future systems may focus more on collaborative control between humans and AI, rather than full autonomy in all situations.
Conclusion
The software behind autonomous vehicles represents one of the most complex and exciting areas of modern programming. It combines elements of computer vision, machine learning, control theory, and real-time systems to create vehicles that can navigate our roads safely and efficiently.
As we continue to develop and refine these systems, we’re not just programming cars – we’re reshaping the future of transportation. The challenges are significant, but the potential benefits in terms of safety, efficiency, and accessibility are enormous.
For aspiring programmers and computer scientists, the field of autonomous vehicle software offers a wealth of opportunities to work on cutting-edge technology that has the potential to change the world. Whether you’re interested in machine learning, computer vision, control systems, or ethical AI, there’s a place for you in this rapidly evolving field.
As we move forward, the key to success will be not just in writing efficient code, but in creating systems that can understand and interact with the complex, unpredictable world of human drivers and pedestrians. It’s a challenge that will require not just technical skill, but also creativity, empathy, and a deep understanding of human behavior.
The road ahead for autonomous vehicle programming is long and winding, but it’s also filled with excitement and potential. As we continue to push the boundaries of what’s possible, we’re not just programming vehicles – we’re programming the future of mobility itself.