Exploring the Edmonds-Karp Algorithm: A Comprehensive Guide
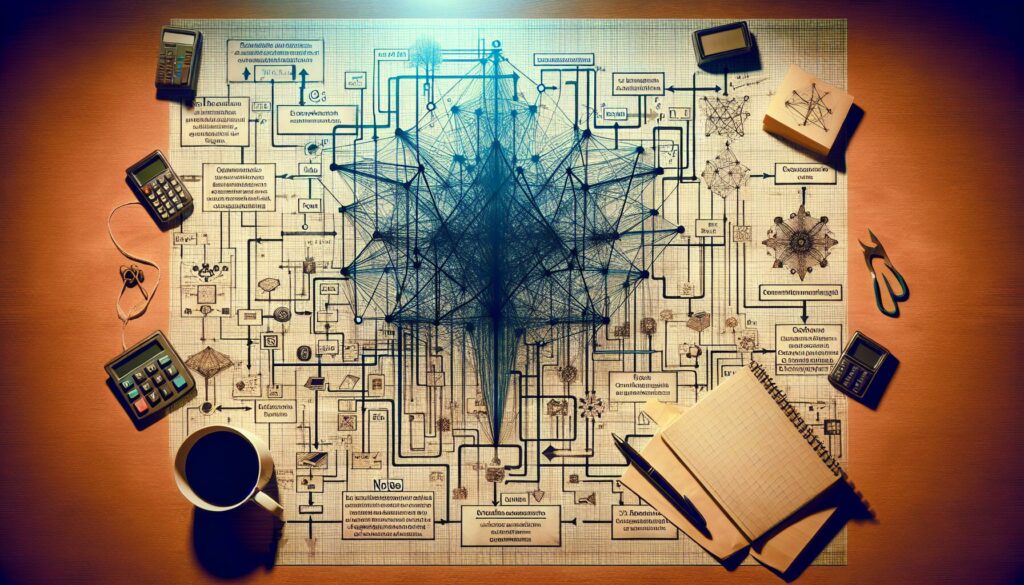
In the world of computer science and network optimization, the Edmonds-Karp algorithm stands as a powerful tool for solving maximum flow problems. This algorithm, an implementation of the Ford-Fulkerson method, has significant applications in various fields, from transportation networks to computer networking. In this comprehensive guide, we’ll dive deep into the Edmonds-Karp algorithm, exploring its concepts, implementation, and real-world applications.
Table of Contents
- Introduction to the Edmonds-Karp Algorithm
- Background and Context
- How the Edmonds-Karp Algorithm Works
- Implementation in Python
- Time Complexity Analysis
- Real-world Applications
- Comparison with Other Flow Algorithms
- Optimization Techniques
- Challenges and Limitations
- Future Directions and Research
- Conclusion
1. Introduction to the Edmonds-Karp Algorithm
The Edmonds-Karp algorithm, developed by Jack Edmonds and Richard Karp in 1972, is a specific implementation of the Ford-Fulkerson method for computing the maximum flow in a flow network. It addresses the maximum flow problem, which involves finding the greatest amount of flow that can be pushed from a source node to a sink node in a given network.
What sets the Edmonds-Karp algorithm apart is its use of breadth-first search (BFS) to find augmenting paths. This choice guarantees a polynomial time complexity, making it more efficient than the general Ford-Fulkerson method, which can have exponential time complexity in certain cases.
2. Background and Context
To fully appreciate the Edmonds-Karp algorithm, it’s essential to understand the context in which it was developed and the problems it aims to solve.
The Maximum Flow Problem
The maximum flow problem is a fundamental problem in network optimization. Given a network represented as a directed graph with capacities on its edges, the goal is to find the maximum flow from a designated source node to a sink node, subject to the capacity constraints of the edges.
Ford-Fulkerson Method
The Ford-Fulkerson method, introduced in 1956, provides a general approach to solving the maximum flow problem. It works by repeatedly finding augmenting paths from the source to the sink and pushing flow along these paths until no more augmenting paths can be found.
However, the Ford-Fulkerson method doesn’t specify how to find these augmenting paths, which can lead to inefficient implementations in certain cases.
Enter Edmonds-Karp
The Edmonds-Karp algorithm improves upon the Ford-Fulkerson method by specifying that augmenting paths should be found using breadth-first search. This seemingly simple modification has profound implications for the algorithm’s performance and guarantees.
3. How the Edmonds-Karp Algorithm Works
Let’s break down the Edmonds-Karp algorithm step by step:
- Initialization: Start with zero flow on all edges of the network.
- Find an Augmenting Path: Use BFS to find the shortest path (in terms of the number of edges) from the source to the sink in the residual graph.
- Augment Flow: If an augmenting path is found, push the maximum possible flow along this path. This flow is equal to the minimum residual capacity among all edges in the path.
- Update Residual Graph: Update the residual capacities of the edges along the augmenting path.
- Repeat: Continue steps 2-4 until no more augmenting paths can be found.
The key insight of the Edmonds-Karp algorithm is the use of BFS to find augmenting paths. This ensures that the algorithm always finds the shortest augmenting path available, which leads to its polynomial time complexity.
The Residual Graph
The concept of a residual graph is crucial to understanding the Edmonds-Karp algorithm. The residual graph represents the remaining capacity in the network after some flow has been pushed. It includes both forward edges (with remaining capacity) and backward edges (allowing flow to be “undone” if necessary).
4. Implementation in Python
Let’s implement the Edmonds-Karp algorithm in Python. We’ll use an adjacency matrix to represent the graph for simplicity, though in practice, an adjacency list might be more efficient for sparse graphs.
from collections import deque
def edmonds_karp(graph, source, sink):
n = len(graph) # Number of nodes
flow = 0
while True:
# Find an augmenting path using BFS
parent = [-1] * n
parent[source] = -2
q = deque([source])
while q and parent[sink] == -1:
u = q.popleft()
for v in range(n):
if parent[v] == -1 and graph[u][v] > 0:
parent[v] = u
q.append(v)
# If no augmenting path is found, we're done
if parent[sink] == -1:
break
# Compute the bottleneck capacity
path_flow = float('inf')
v = sink
while v != source:
u = parent[v]
path_flow = min(path_flow, graph[u][v])
v = u
# Update the residual graph
v = sink
while v != source:
u = parent[v]
graph[u][v] -= path_flow
graph[v][u] += path_flow
v = u
flow += path_flow
return flow
# Example usage
graph = [
[0, 16, 13, 0, 0, 0],
[0, 0, 10, 12, 0, 0],
[0, 4, 0, 0, 14, 0],
[0, 0, 9, 0, 0, 20],
[0, 0, 0, 7, 0, 4],
[0, 0, 0, 0, 0, 0]
]
source = 0
sink = 5
max_flow = edmonds_karp(graph, source, sink)
print(f"The maximum flow is: {max_flow}")
This implementation follows the steps outlined earlier. It uses a breadth-first search to find augmenting paths, computes the bottleneck capacity, and updates the residual graph accordingly.
5. Time Complexity Analysis
One of the key advantages of the Edmonds-Karp algorithm is its guaranteed polynomial time complexity. Let’s break down the analysis:
- The algorithm performs at most O(VE) augmentations, where V is the number of vertices and E is the number of edges in the graph.
- Each BFS takes O(E) time.
- Therefore, the overall time complexity is O(VE^2).
This polynomial time complexity is a significant improvement over the potential exponential time complexity of the general Ford-Fulkerson method.
Why O(VE) Augmentations?
The key to understanding the O(VE) bound on the number of augmentations lies in the algorithm’s use of BFS to find shortest augmenting paths. With each augmentation, at least one edge in the residual network becomes saturated. Moreover, the length of the shortest augmenting path must increase after at most V augmentations. Since the longest possible path has length V-1, there can be at most O(VE) augmentations in total.
6. Real-world Applications
The Edmonds-Karp algorithm, and maximum flow algorithms in general, have numerous practical applications across various domains:
1. Transportation Networks
Optimizing the flow of vehicles, goods, or people through road networks, railway systems, or airline routes.
2. Computer Networking
Managing data flow in communication networks, optimizing bandwidth allocation, and solving network congestion issues.
3. Bipartite Matching
Solving assignment problems, such as matching job applicants to positions or students to courses.
4. Image Segmentation
In computer vision, maximum flow algorithms can be used for image segmentation tasks, separating foreground from background.
5. Sports Scheduling
Determining if a sports league’s remaining schedule allows for a particular team to achieve a certain standing.
6. Project Management
Analyzing project networks to identify bottlenecks and optimize resource allocation.
7. Comparison with Other Flow Algorithms
While the Edmonds-Karp algorithm is a significant improvement over the basic Ford-Fulkerson method, it’s not the only algorithm for solving maximum flow problems. Let’s compare it with some other notable algorithms:
Dinic’s Algorithm
Dinic’s algorithm improves upon Edmonds-Karp by using level graphs and blocking flows. It has a time complexity of O(V^2E), which is better than Edmonds-Karp for dense graphs.
Push-Relabel Algorithm
The Push-Relabel algorithm takes a different approach, working locally on vertices rather than finding augmenting paths. It has a time complexity of O(V^2E) or O(V^3), depending on the implementation.
MPM Algorithm
The MPM (Malhotra, Pramodh-Kumar, and Maheshwari) algorithm combines ideas from Dinic’s algorithm and the Push-Relabel method. It achieves a time complexity of O(V^3).
The choice of algorithm often depends on the specific characteristics of the problem and the graph structure. Edmonds-Karp remains popular due to its simplicity and guaranteed polynomial time complexity.
8. Optimization Techniques
While the basic Edmonds-Karp algorithm is already efficient, there are several optimization techniques that can improve its performance in practice:
1. Early Termination
If we know an upper bound on the maximum flow, we can terminate the algorithm early once this bound is reached.
2. Capacity Scaling
By initially considering only high-capacity edges and gradually including lower-capacity edges, we can potentially reduce the number of augmentations needed.
3. Edge Ordering
Maintaining a specific order of edges when performing BFS can lead to finding better augmenting paths more quickly.
4. Graph Compression
For graphs with many degree-2 vertices, we can compress paths of such vertices into single edges with combined capacities.
5. Using Adjacency Lists
Implementing the graph using adjacency lists instead of an adjacency matrix can improve performance for sparse graphs.
from collections import defaultdict, deque
def edmonds_karp_optimized(graph, source, sink):
def bfs():
visited = [False] * len(graph)
queue = deque([(source, float('inf'))])
visited[source] = True
while queue:
u, flow = queue.popleft()
if u == sink:
return flow
for v, cap in graph[u].items():
if not visited[v] and cap > 0:
visited[v] = True
queue.append((v, min(flow, cap)))
parent[v] = u
return 0
flow = 0
parent = [-1] * len(graph)
while True:
path_flow = bfs()
if path_flow == 0:
break
flow += path_flow
v = sink
while v != source:
u = parent[v]
graph[u][v] -= path_flow
graph[v][u] += path_flow
v = u
return flow
# Example usage
graph = defaultdict(lambda: defaultdict(int))
graph[0][1] = 16
graph[0][2] = 13
graph[1][2] = 10
graph[1][3] = 12
graph[2][1] = 4
graph[2][4] = 14
graph[3][2] = 9
graph[3][5] = 20
graph[4][3] = 7
graph[4][5] = 4
source, sink = 0, 5
max_flow = edmonds_karp_optimized(graph, source, sink)
print(f"The maximum flow is: {max_flow}")
This optimized version uses adjacency lists (implemented with nested defaultdicts) and incorporates the early termination optimization within the BFS function.
9. Challenges and Limitations
While the Edmonds-Karp algorithm is powerful and widely applicable, it’s important to be aware of its limitations and challenges:
1. Memory Usage
For very large graphs, the memory required to store the residual graph can be substantial.
2. Performance on Dense Graphs
While polynomial, the O(VE^2) time complexity can still be prohibitive for extremely large, dense graphs.
3. Floating-Point Precision
When dealing with non-integer capacities, floating-point precision issues can arise, potentially leading to inaccuracies in the final flow value.
4. Dynamic Graphs
The algorithm is designed for static graphs. Adapting it to handle dynamically changing graphs efficiently is challenging.
5. Multiple Sources and Sinks
While it’s possible to modify the algorithm to handle multiple sources and sinks, doing so efficiently can be non-trivial.
10. Future Directions and Research
The field of network flow algorithms continues to evolve, with ongoing research in several directions:
1. Parallelization
Developing efficient parallel implementations of maximum flow algorithms, including Edmonds-Karp, to leverage modern multi-core and distributed computing architectures.
2. Approximation Algorithms
For extremely large graphs where exact solutions are impractical, research into fast approximation algorithms for maximum flow continues.
3. Integration with Machine Learning
Exploring ways to combine traditional flow algorithms with machine learning techniques for better prediction and optimization in dynamic network scenarios.
4. Quantum Algorithms
Investigating potential quantum algorithms for solving maximum flow problems, which could offer significant speedups over classical algorithms.
5. Application-Specific Optimizations
Developing specialized variants of flow algorithms optimized for specific types of networks or application domains.
11. Conclusion
The Edmonds-Karp algorithm stands as a cornerstone in the field of network flow algorithms. Its elegant use of breadth-first search to find augmenting paths not only guarantees polynomial time complexity but also provides a clear and intuitive approach to solving maximum flow problems.
As we’ve explored in this comprehensive guide, the algorithm’s applications span a wide range of fields, from transportation and networking to image processing and project management. Its efficiency and versatility make it a valuable tool in any computer scientist’s or software engineer’s toolkit.
While more advanced algorithms have been developed since Edmonds-Karp, its fundamental principles continue to influence modern approaches to network optimization. As research in this field progresses, we can expect to see new innovations building upon the solid foundation laid by algorithms like Edmonds-Karp.
Whether you’re preparing for technical interviews, working on network optimization problems, or simply expanding your algorithmic knowledge, a deep understanding of the Edmonds-Karp algorithm is invaluable. Its blend of graph theory, optimization, and algorithmic thinking encapsulates many of the core principles that drive computer science and software engineering forward.
As you continue your journey in algorithm study and implementation, remember that algorithms like Edmonds-Karp are not just theoretical constructs, but powerful tools with real-world impact. They represent the kind of innovative thinking and problem-solving skills that are at the heart of computer science and that continue to shape our increasingly networked world.