Exploring Serverless Architecture: Building Applications Without Managing Server Infrastructure
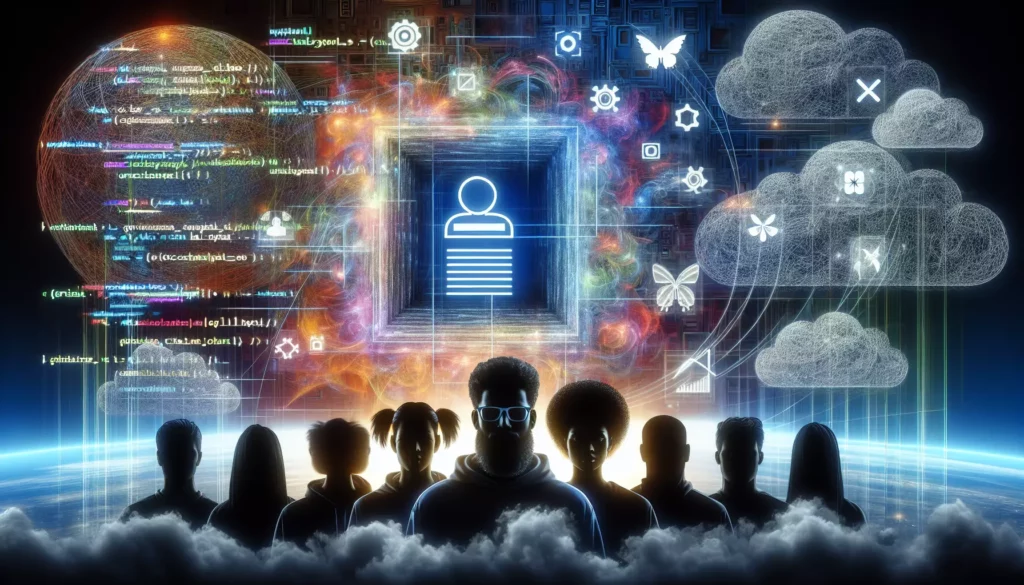
In the ever-evolving landscape of software development, serverless architecture has emerged as a game-changing paradigm. This approach allows developers to build and run applications without the need to manage server infrastructure directly. Services like AWS Lambda and Azure Functions have made it possible to focus on writing code and delivering value, rather than worrying about server maintenance and scaling. In this comprehensive guide, we’ll explore the world of serverless architecture, its benefits, challenges, and how to get started with popular serverless platforms.
What is Serverless Architecture?
Serverless architecture, despite its name, doesn’t mean there are no servers involved. Instead, it refers to a cloud computing execution model where the cloud provider dynamically manages the allocation and provisioning of servers. A serverless application runs in stateless compute containers that are event-triggered, ephemeral (may last for one invocation), and fully managed by the cloud provider.
Key characteristics of serverless architecture include:
- No server management: Developers don’t need to worry about server provisioning, maintenance, updates, or scaling.
- Pay-per-execution: You’re charged based on the number of executions rather than pre-purchased compute capacity.
- Auto-scaling: The platform automatically scales your application in response to demand.
- Event-driven: Serverless functions are typically triggered by events, such as HTTP requests, database changes, or messaging events.
Benefits of Serverless Architecture
Adopting a serverless approach offers several advantages:
- Reduced operational costs: Pay only for the compute time you consume, rather than for idle servers.
- Improved developer productivity: Focus on writing code instead of managing infrastructure.
- Automatic scaling: Handle varying workloads without manual intervention.
- Faster time to market: Rapidly develop and deploy applications without infrastructure concerns.
- Enhanced flexibility: Easily integrate with other cloud services and third-party APIs.
Popular Serverless Platforms
Several cloud providers offer serverless computing services. Let’s look at two of the most popular options:
AWS Lambda
AWS Lambda is Amazon’s serverless compute service. It allows you to run code without provisioning or managing servers. Key features include:
- Support for multiple programming languages (Node.js, Python, Java, C#, Go, and more)
- Automatic scaling and high availability
- Integration with other AWS services
- Pay-per-use pricing model
Azure Functions
Microsoft’s Azure Functions is another popular serverless computing service. It offers:
- Support for various programming languages (C#, JavaScript, Python, PowerShell, and more)
- Seamless integration with Azure services
- Flexible scaling options
- Pay-per-execution pricing
Building a Serverless Application
Let’s walk through the process of creating a simple serverless application using AWS Lambda. We’ll create a function that responds to HTTP requests and returns a greeting message.
Step 1: Set up an AWS account
If you don’t already have an AWS account, sign up at aws.amazon.com. You’ll need to provide credit card information, but AWS offers a free tier that includes 1 million free Lambda requests per month.
Step 2: Create a Lambda function
- Navigate to the AWS Lambda console
- Click “Create function”
- Choose “Author from scratch”
- Give your function a name (e.g., “HelloWorldFunction”)
- Select a runtime (e.g., Node.js 14.x)
- Click “Create function”
Step 3: Write the function code
In the Lambda function editor, replace the default code with the following:
exports.handler = async (event) => {
const name = event.queryStringParameters && event.queryStringParameters.name || 'World';
const response = {
statusCode: 200,
body: JSON.stringify({ message: `Hello, ${name}!` }),
};
return response;
};
This function checks for a ‘name’ query parameter and uses it in the greeting. If no name is provided, it defaults to ‘World’.
Step 4: Configure an API Gateway trigger
- In the Lambda function configuration, click “Add trigger”
- Select “API Gateway” as the trigger type
- Choose “Create a new API”
- Select “HTTP API” and “Open” security
- Click “Add”
Step 5: Test your function
After the API Gateway is created, you’ll see a URL for your function. You can test it by visiting this URL in a web browser or using a tool like curl:
curl "https://your-api-id.execute-api.your-region.amazonaws.com/default/HelloWorldFunction?name=Alice"
You should receive a response like:
{"message":"Hello, Alice!"}
Best Practices for Serverless Development
As you dive deeper into serverless development, keep these best practices in mind:
- Keep functions small and focused: Each function should do one thing well. This improves maintainability and reduces cold start times.
- Use environment variables: Store configuration values as environment variables rather than hardcoding them in your function.
- Implement proper error handling: Ensure your functions handle errors gracefully and provide meaningful error messages.
- Optimize for cold starts: Minimize the use of heavy dependencies and consider using provisioned concurrency for frequently accessed functions.
- Monitor and log extensively: Use cloud provider monitoring tools and implement comprehensive logging to troubleshoot issues.
- Consider security: Implement proper authentication and authorization. Use the principle of least privilege when granting permissions to your functions.
- Test thoroughly: Implement unit tests, integration tests, and end-to-end tests for your serverless applications.
Challenges of Serverless Architecture
While serverless offers many benefits, it’s important to be aware of potential challenges:
- Cold starts: When a function hasn’t been used for a while, it may take longer to start up, leading to increased latency.
- Vendor lock-in: Serverless applications often rely heavily on cloud provider-specific services, which can make it difficult to switch providers.
- Limited execution duration: Most serverless platforms have a maximum execution time for functions, which can be problematic for long-running tasks.
- Debugging and monitoring: Debugging distributed serverless applications can be more complex than traditional monolithic applications.
- State management: Serverless functions are stateless by design, which can complicate applications that need to maintain state.
Advanced Serverless Concepts
As you become more comfortable with basic serverless development, you may want to explore more advanced concepts:
Serverless Frameworks
Serverless frameworks can simplify the development and deployment of serverless applications. Some popular options include:
- Serverless Framework: A multi-provider framework that supports AWS, Azure, Google Cloud, and more.
- AWS SAM (Serverless Application Model): A framework specifically for building serverless applications on AWS.
- Claudia.js: A deployment tool that simplifies serverless development for Node.js applications.
Event-Driven Architecture
Serverless functions are often used in event-driven architectures. This involves designing systems where functions are triggered by events from various sources, such as:
- Database changes
- File uploads
- Message queue events
- IoT device signals
Understanding event-driven design patterns can help you build more scalable and responsive serverless applications.
Serverless Databases
Traditional databases can be challenging to use in serverless architectures due to connection management and scaling issues. Serverless databases address these challenges. Some options include:
- Amazon DynamoDB
- Azure Cosmos DB
- Google Cloud Firestore
- FaunaDB
Serverless GraphQL APIs
GraphQL is becoming increasingly popular for building flexible APIs. Serverless platforms offer ways to build and deploy GraphQL APIs without managing servers. For example:
- AWS AppSync
- Azure Static Web Apps with Azure Functions
- Apollo Server with serverless functions
Real-World Serverless Use Cases
Serverless architecture can be applied to a wide range of applications. Here are some common use cases:
- Web and mobile backends: Build scalable APIs for web and mobile applications without managing servers.
- Data processing: Use serverless functions to process data in real-time, such as image resizing or log analysis.
- IoT backends: Handle data from IoT devices and trigger actions based on incoming data.
- Chatbots and virtual assistants: Build conversational interfaces that can scale to handle varying loads.
- Scheduled tasks and cron jobs: Run periodic tasks without maintaining a dedicated server.
- CI/CD pipelines: Automate parts of your development workflow using serverless functions.
The Future of Serverless
As serverless technology continues to evolve, we can expect to see:
- Improved cold start times: Cloud providers are working on reducing cold start latency.
- Better developer tools: More sophisticated debugging and monitoring tools for serverless applications.
- Increased adoption in enterprise: As serverless matures, more large organizations will adopt it for critical applications.
- Edge computing integration: Serverless functions running at edge locations for reduced latency.
- Standardization: Efforts to create standards that reduce vendor lock-in and improve portability.
Conclusion
Serverless architecture represents a significant shift in how we build and deploy applications. By abstracting away server management, it allows developers to focus on writing code and delivering value. While it comes with its own set of challenges, the benefits of reduced operational overhead, improved scalability, and potential cost savings make it an attractive option for many use cases.
As you continue your journey in software development, exploring serverless architecture can open up new possibilities and efficiencies in your projects. Whether you’re building a simple API or a complex, event-driven system, serverless platforms like AWS Lambda and Azure Functions provide powerful tools to bring your ideas to life without the burden of server management.
Remember, the key to success with serverless is to start small, understand the principles, and gradually build more complex applications as you become comfortable with the paradigm. Happy coding, and welcome to the serverless revolution!