Exploring Programming for Wearable Devices: A Comprehensive Guide to Developing Apps for Smartwatches
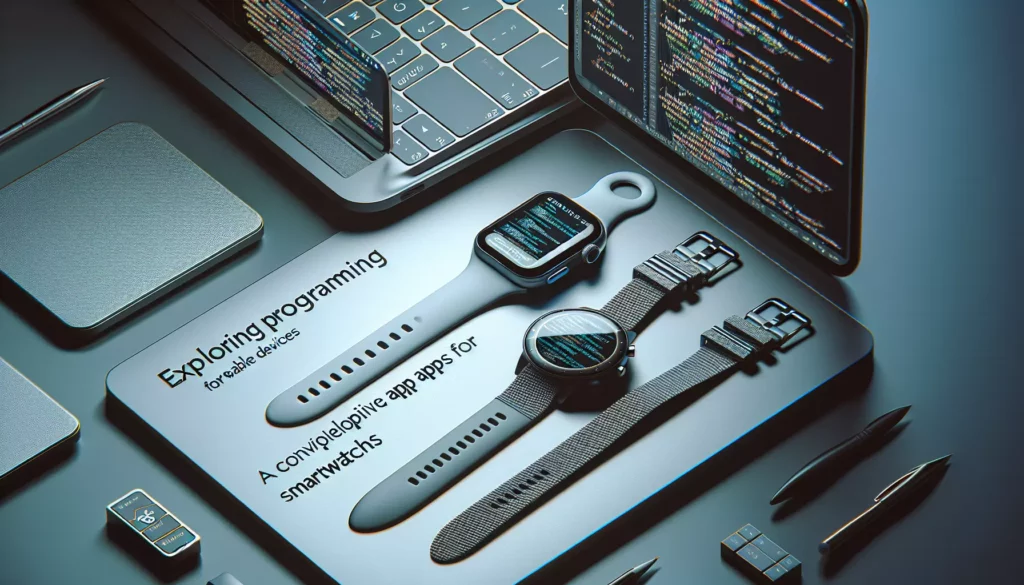
In the ever-evolving landscape of technology, wearable devices have emerged as a fascinating frontier for developers. Among these, smartwatches stand out as particularly intriguing platforms, offering unique challenges and opportunities for app creation. This comprehensive guide will delve into the world of programming for wearable devices, with a specific focus on developing apps for smartwatches using popular platforms like Wear OS and watchOS.
Table of Contents
- Introduction to Wearable Device Programming
- Understanding Wearable Platforms: Wear OS and watchOS
- Getting Started with Smartwatch App Development
- Design Principles for Wearable Apps
- Developing for Wear OS
- Developing for watchOS
- Testing and Debugging Wearable Apps
- Implementing Advanced Features
- Challenges in Wearable App Development
- Future Trends in Wearable Technology
- Conclusion
1. Introduction to Wearable Device Programming
Wearable devices, particularly smartwatches, have revolutionized the way we interact with technology. These compact, powerful devices offer a unique set of capabilities that can enhance users’ daily lives. As a programmer, venturing into wearable app development opens up exciting possibilities for creating innovative, user-centric applications.
Wearable programming differs significantly from traditional mobile or desktop development. The constrained screen size, limited battery life, and unique interaction models present both challenges and opportunities for developers. Understanding these nuances is crucial for creating successful wearable applications.
2. Understanding Wearable Platforms: Wear OS and watchOS
Two major platforms dominate the smartwatch market: Google’s Wear OS and Apple’s watchOS. Let’s explore each of these platforms in detail:
Wear OS
Wear OS, formerly known as Android Wear, is Google’s operating system for smartwatches. It’s designed to work with Android smartphones and offers a wide range of features:
- Compatibility with a variety of hardware from different manufacturers
- Integration with Google services like Google Assistant, Google Fit, and Google Pay
- Support for both round and square watch faces
- A growing ecosystem of third-party apps
watchOS
watchOS is Apple’s proprietary operating system for the Apple Watch. Key features include:
- Tight integration with iOS devices
- Access to Apple’s ecosystem, including Siri, Apple Pay, and HealthKit
- Emphasis on health and fitness tracking
- A curated App Store specifically for Apple Watch apps
3. Getting Started with Smartwatch App Development
Before diving into development, it’s essential to set up your development environment. Here’s what you’ll need for each platform:
For Wear OS Development:
- Android Studio
- Java or Kotlin programming skills
- Wear OS emulator or a physical Wear OS device
For watchOS Development:
- Xcode
- Swift programming skills
- watchOS simulator or a physical Apple Watch
Once you have your environment set up, familiarize yourself with the platform-specific SDKs and documentation. Both Google and Apple provide extensive resources for developers looking to create apps for their respective platforms.
4. Design Principles for Wearable Apps
Designing for wearables requires a different approach compared to mobile or desktop applications. Here are some key principles to keep in mind:
Simplicity is Key
Given the limited screen real estate, focus on providing essential information and functionality. Avoid cluttered interfaces and complex navigation structures.
Glanceable Information
Users typically interact with smartwatches for short periods. Design your app to provide quick, easily digestible information at a glance.
Leverage Platform-Specific Features
Take advantage of unique hardware features like heart rate sensors, accelerometers, and GPS to enhance your app’s functionality.
Consistent with Platform Guidelines
Adhere to the design guidelines provided by Google and Apple to ensure your app feels native to the platform.
5. Developing for Wear OS
Wear OS development is based on Android development principles, with some specific considerations for wearable devices. Here’s a basic example of creating a simple Wear OS app:
<?xml version="1.0" encoding="utf-8"?>
<androidx.wear.widget.BoxInsetLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="@dimen/box_inset_layout_padding"
tools:context=".MainActivity"
tools:deviceIds="wear">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="@dimen/inner_frame_layout_padding"
app:layout_boxedEdges="all">
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
</FrameLayout>
</androidx.wear.widget.BoxInsetLayout>
This XML layout uses the BoxInsetLayout
, which is specific to Wear OS and ensures that your content is properly displayed on both round and square watch faces.
In your activity, you can then interact with this layout:
import android.os.Bundle
import android.support.wearable.activity.WearableActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : WearableActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Enables Always-on
setAmbientEnabled()
// Example of setting the text view
text.text = "Hello, Wear OS!"
}
}
This Kotlin code sets up a basic Wear OS activity and demonstrates how to interact with the UI elements defined in the layout.
6. Developing for watchOS
watchOS development is based on iOS development principles but tailored for the Apple Watch. Here’s a basic example of creating a simple watchOS app using SwiftUI:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, watchOS!")
.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
@main
struct MyWatchApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
This SwiftUI code creates a simple watchOS app that displays “Hello, watchOS!” on the screen. SwiftUI makes it easy to create interfaces that adapt to different Apple Watch sizes and shapes.
7. Testing and Debugging Wearable Apps
Testing wearable apps presents unique challenges due to the small form factor and specific use cases. Here are some key considerations:
Emulators and Simulators
Both Android Studio and Xcode provide emulators/simulators for testing your apps without physical devices. While these are useful for initial development, they can’t fully replicate the experience of using a physical device.
Physical Device Testing
Whenever possible, test your app on actual smartwatches. This allows you to test factors like battery consumption, performance on real hardware, and the actual user experience of interacting with a small touchscreen.
Performance Profiling
Use the profiling tools provided by Android Studio and Xcode to monitor your app’s performance, particularly in terms of battery usage and responsiveness.
Accessibility Testing
Ensure your app is usable with accessibility features like VoiceOver (for watchOS) or TalkBack (for Wear OS).
8. Implementing Advanced Features
Once you’ve mastered the basics, you can start exploring more advanced features of smartwatch development:
Complications
Complications are small elements on a watch face that display information from apps. Implementing complications allows your app to provide quick, glanceable information directly on the watch face.
Background Tasks
Both Wear OS and watchOS support background tasks, allowing your app to perform operations even when it’s not actively running. This is particularly useful for fitness tracking or notification-based apps.
Sensor Data
Leverage the various sensors available on smartwatches, such as heart rate monitors, accelerometers, and GPS, to create more interactive and context-aware applications.
Voice Commands
Implement voice command functionality to make your app easier to use on the small screen of a smartwatch.
9. Challenges in Wearable App Development
Developing for wearables comes with its own set of challenges:
Battery Life
Smartwatches have small batteries, so it’s crucial to optimize your app’s power consumption. Avoid constant background processes and minimize network requests.
Limited Screen Real Estate
The small screen size requires careful UI design. Prioritize the most important information and functions.
Input Methods
Traditional input methods like keyboards are impractical on smartwatches. Consider alternative input methods like voice commands or predefined responses.
Connectivity
Many smartwatches rely on a connection to a smartphone. Design your app to gracefully handle situations where this connection may be intermittent or unavailable.
10. Future Trends in Wearable Technology
As wearable technology continues to evolve, several trends are shaping the future of smartwatch development:
Health and Fitness Integration
Smartwatches are increasingly being used for health monitoring and fitness tracking. Future apps may integrate more deeply with health data and provide more sophisticated analysis.
AI and Machine Learning
On-device machine learning could enable more personalized experiences and smarter, context-aware applications.
Augmented Reality
While still in its early stages for wearables, AR could open up new possibilities for smartwatch interactions.
Improved Battery Life and Performance
As hardware improves, developers will have more resources to work with, enabling more complex and feature-rich applications.
11. Conclusion
Programming for wearable devices, particularly smartwatches, offers an exciting opportunity to create innovative, user-centric applications. By understanding the unique constraints and possibilities of these devices, developers can create apps that truly enhance users’ daily lives.
Whether you’re developing for Wear OS or watchOS, the key is to focus on simplicity, glanceability, and leveraging the unique features of smartwatches. As wearable technology continues to evolve, the potential for creative and impactful applications will only grow.
Remember, the best wearable apps are those that provide value while seamlessly integrating into the user’s life. As you embark on your journey into wearable app development, keep the user experience at the forefront of your design and development process.
Happy coding, and may your wearable apps make a positive impact in the exciting world of smartwatch technology!