Exploring NP-Complete Problems: The Ultimate Challenge in Computer Science
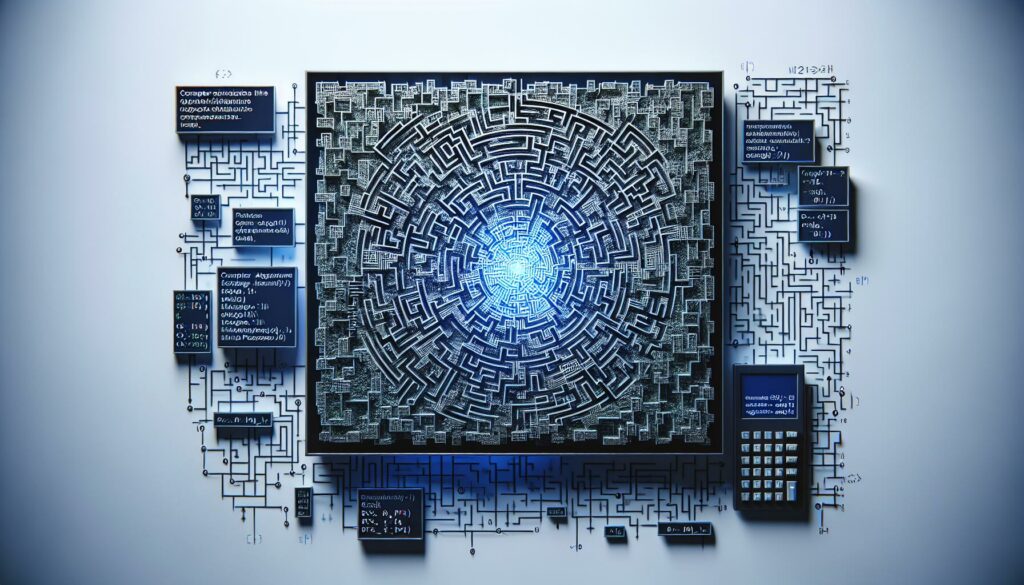
In the vast realm of computer science and algorithmic complexity, few concepts are as intriguing and challenging as NP-complete problems. These computational puzzles have captivated researchers, programmers, and theorists for decades, standing as a testament to the limits of efficient computation. In this comprehensive exploration, we’ll dive deep into the world of NP-complete problems, unraveling their significance, characteristics, and impact on modern computing.
What Are NP-Complete Problems?
NP-complete problems represent a class of computational challenges that are notoriously difficult to solve efficiently. The term “NP” stands for “nondeterministic polynomial time,” which refers to the time complexity of verifying a solution to these problems. In essence, NP-complete problems are decision problems for which:
- A solution can be verified quickly (in polynomial time)
- No known algorithm can solve the problem efficiently (in polynomial time) for all instances
These problems are at the heart of computational complexity theory and have far-reaching implications for various fields, including computer science, mathematics, and even cryptography.
The Importance of NP-Complete Problems
Understanding NP-complete problems is crucial for several reasons:
- Theoretical Significance: They represent a fundamental boundary in computational complexity, helping us understand the limits of efficient computation.
- Practical Applications: Many real-world optimization problems are NP-complete, affecting areas like scheduling, resource allocation, and network design.
- Algorithmic Research: The quest for efficient solutions to NP-complete problems drives innovation in algorithm design and heuristics.
- Cryptography: The hardness of certain NP-complete problems forms the basis for many cryptographic systems.
Characteristics of NP-Complete Problems
NP-complete problems share several key characteristics:
- Decision Problems: They are typically framed as yes-or-no questions.
- Verifiability: Given a potential solution, it can be verified quickly (in polynomial time).
- Hardness: They are at least as hard as any problem in NP.
- Reducibility: Any problem in NP can be reduced to an NP-complete problem in polynomial time.
Famous Examples of NP-Complete Problems
Let’s explore some of the most well-known NP-complete problems:
1. The Traveling Salesman Problem (TSP)
The TSP asks: “Given a list of cities and the distances between each pair of cities, what is the shortest possible route that visits each city exactly once and returns to the starting city?”
This problem has applications in logistics, planning, and microchip manufacturing. While it’s easy to verify a given solution, finding the optimal route becomes exponentially more difficult as the number of cities increases.
2. Boolean Satisfiability Problem (SAT)
SAT is the problem of determining if there exists an interpretation that satisfies a given Boolean formula. For example:
(x1 OR x2) AND (NOT x1 OR x3) AND (NOT x2 OR NOT x3)
The challenge is to find values for x1, x2, and x3 that make the entire formula true, or determine that no such values exist.
3. Graph Coloring Problem
This problem asks whether the vertices of a given graph can be colored using at most k colors, such that no two adjacent vertices share the same color. It has applications in scheduling, register allocation in compilers, and even solving Sudoku puzzles.
4. Subset Sum Problem
Given a set of integers and a target sum, the subset sum problem asks whether there exists a subset of the integers that adds up exactly to the target sum. This problem is related to various optimization and decision-making scenarios.
Approaches to Solving NP-Complete Problems
While no efficient algorithm is known to solve NP-complete problems in general, several approaches are used to tackle them in practice:
1. Approximation Algorithms
These algorithms aim to find a solution that is guaranteed to be within a certain factor of the optimal solution. For example, there are approximation algorithms for the Traveling Salesman Problem that can find routes within a factor of 1.5 of the optimal route length.
2. Heuristics and Metaheuristics
Heuristic methods use problem-specific knowledge to guide the search for a good (but not necessarily optimal) solution. Metaheuristics like genetic algorithms, simulated annealing, and ant colony optimization provide general frameworks for developing problem-solving strategies.
3. Parameterized Algorithms
These algorithms exploit the structure of the input to solve problems efficiently when certain parameters are small, even if the overall input size is large.
4. Special Case Solutions
For some NP-complete problems, efficient algorithms exist for special cases or restricted inputs. Identifying these cases can lead to practical solutions for specific scenarios.
The P vs NP Problem
At the heart of the study of NP-complete problems lies one of the most famous unsolved problems in computer science: the P vs NP problem. This question asks whether every problem whose solution can be quickly verified (NP) can also be solved quickly (P).
If P = NP were true, it would mean that there exist efficient algorithms for all NP-complete problems, revolutionizing fields from cryptography to optimization. However, most researchers believe that P ≠NP, implying that NP-complete problems are inherently hard and cannot be solved efficiently in general.
Implications and Applications
The study of NP-complete problems has profound implications across various domains:
1. Algorithm Design
Understanding the nature of NP-complete problems guides algorithm designers in developing practical approaches for hard problems, even when optimal solutions are out of reach.
2. Cryptography
Many cryptographic systems rely on the assumed hardness of certain NP-complete problems. For instance, public-key cryptography often depends on the difficulty of factoring large numbers, a problem believed to be NP-intermediate (neither in P nor NP-complete, but still in NP).
3. Artificial Intelligence
Many problems in AI, such as certain types of constraint satisfaction and planning problems, are NP-complete. This understanding helps in developing appropriate heuristics and approximation methods.
4. Optimization
Real-world optimization problems in logistics, scheduling, and resource allocation are often NP-complete. Techniques developed for these problems have wide-ranging industrial applications.
Coding Challenge: Subset Sum Problem
To better understand NP-complete problems, let’s implement a solution to the Subset Sum Problem. We’ll use a dynamic programming approach, which is efficient for small to medium-sized inputs but still exhibits exponential time complexity in the worst case.
def subset_sum(numbers, target_sum):
n = len(numbers)
dp = [[False for _ in range(target_sum + 1)] for _ in range(n + 1)]
# Initialize base case: empty subset can achieve sum 0
for i in range(n + 1):
dp[i][0] = True
# Fill the dp table
for i in range(1, n + 1):
for j in range(1, target_sum + 1):
if j < numbers[i-1]:
dp[i][j] = dp[i-1][j]
else:
dp[i][j] = dp[i-1][j] or dp[i-1][j-numbers[i-1]]
# Reconstruct the subset if a solution exists
if dp[n][target_sum]:
subset = []
i, j = n, target_sum
while i > 0 and j > 0:
if dp[i][j] != dp[i-1][j]:
subset.append(numbers[i-1])
j -= numbers[i-1]
i -= 1
return True, subset[::-1]
else:
return False, []
# Example usage
numbers = [3, 34, 4, 12, 5, 2]
target_sum = 9
exists, subset = subset_sum(numbers, target_sum)
if exists:
print(f"Subset with sum {target_sum} exists: {subset}")
else:
print(f"No subset with sum {target_sum} exists.")
This implementation uses dynamic programming to solve the Subset Sum Problem. It builds a table where dp[i][j]
represents whether it’s possible to achieve a sum of j using the first i numbers. The algorithm then reconstructs the actual subset if a solution exists.
Conclusion
NP-complete problems represent some of the most challenging and intriguing puzzles in computer science. Their study has led to profound insights into the nature of computation and has driven the development of numerous algorithmic techniques and heuristics.
As aspiring computer scientists and programmers, understanding NP-complete problems is crucial. It not only helps in recognizing the inherent difficulty of certain problems but also guides us in developing practical approaches to tackle them. Whether you’re designing algorithms, working on optimization problems, or delving into artificial intelligence, the concepts surrounding NP-completeness will invariably shape your approach to problem-solving.
While the ultimate resolution of the P vs NP problem remains one of the great open questions in mathematics and computer science, the journey of exploring NP-complete problems continues to yield valuable insights and practical applications. As you progress in your coding education and skills development, keep these challenging problems in mind – they represent the frontiers of computational thinking and the endless possibilities that lie in the realm of algorithm design and problem-solving.